Story Time: Tales from Coding Interviews
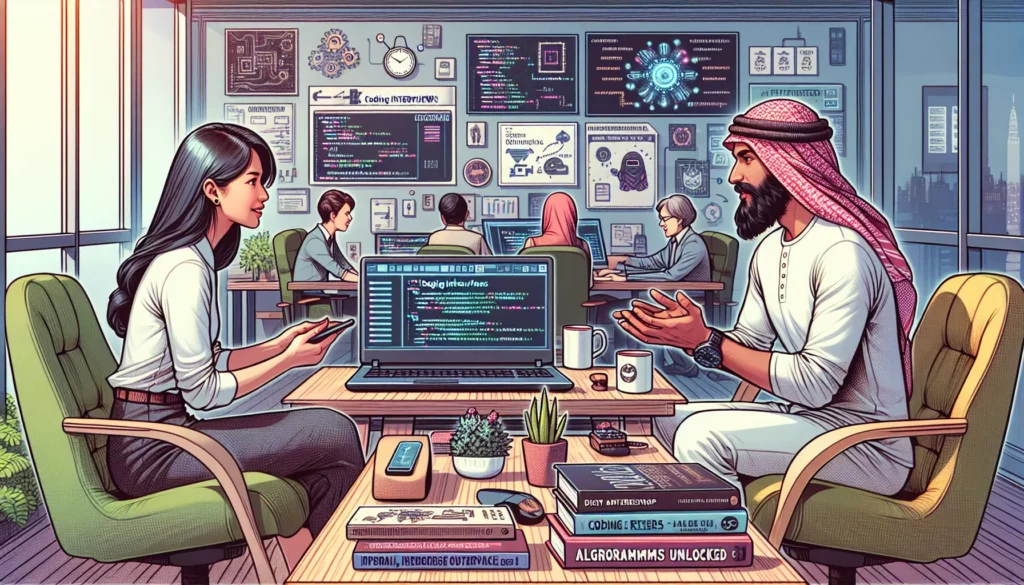
Welcome to AlgoCademy’s collection of coding interview tales! In this blog post, we’ll dive into some of the most memorable, challenging, and sometimes humorous experiences that developers have encountered during technical interviews. These stories not only provide entertainment but also offer valuable insights into the interview process at major tech companies, including the renowned FAANG (Facebook, Amazon, Apple, Netflix, Google) organizations.
Whether you’re a seasoned developer preparing for your next big interview or a coding novice just starting your journey, these tales will give you a glimpse into the world of technical interviews and the importance of solid algorithmic thinking and problem-solving skills.
1. The Unexpected Whiteboard Challenge
Our first story comes from Sarah, a software engineer who interviewed at a major Silicon Valley tech company. She arrived at the interview feeling confident, having spent weeks practicing coding problems and reviewing data structures. However, what happened next caught her completely off guard.
“I walked into the room, and instead of a computer, there was just a giant whiteboard,” Sarah recalls. “The interviewer smiled and said, ‘Today, we’re going to do something a little different. I want you to design a system that can handle millions of users posting and interacting with content in real-time.'”
Sarah’s heart raced as she realized this wasn’t going to be a typical coding challenge. She took a deep breath and started breaking down the problem, discussing potential architectures, data models, and scaling considerations.
“It was nerve-wracking at first,” she admits, “but as I got into it, I actually enjoyed the process. It felt more like a collaborative discussion than a test.”
The lesson here? Be prepared for anything. While coding skills are crucial, interviewers also value your ability to think on your feet and communicate complex ideas effectively.
2. The Bug That Wasn’t
Next, we have a tale from Mike, a backend developer who interviewed at one of the FAANG companies. His challenge was to implement a function that would find the longest palindromic substring in a given string.
Mike confidently wrote his solution, explaining his thought process as he went along. When he finished, the interviewer ran his code through a series of test cases. To Mike’s horror, one of the tests failed.
“I started panicking,” Mike says. “I was sure my algorithm was correct, but I couldn’t figure out why it was failing this particular test case.”
As Mike frantically reviewed his code, the interviewer watched silently. After a few minutes of tense debugging, Mike had a realization.
“Wait a second,” he said, “I think the test case itself might be incorrect.”
He explained his reasoning to the interviewer, who broke into a smile. “Congratulations,” the interviewer said, “You’ve passed the real test. We intentionally included an erroneous test case to see how you would handle it.”
This story highlights the importance of confidence in your solutions and the ability to critically analyze not just your own code, but also the problems presented to you.
3. The Collaborative Coding Challenge
Our third story comes from Alex, a frontend developer who interviewed at a well-known tech startup. Alex was surprised when the interviewer invited them to participate in a pair programming exercise.
“The interviewer said we were going to work together to build a simple React component,” Alex explains. “At first, I was nervous about coding with someone watching, but it turned out to be a great experience.”
As they worked, the interviewer asked questions about Alex’s design choices and suggested alternative approaches. They discussed pros and cons of different implementations and even refactored the code together.
“It felt less like an interview and more like a regular day at work,” Alex says. “I realized they were assessing not just my coding skills, but also how well I could collaborate and communicate with team members.”
This experience underscores the importance of soft skills in technical roles. Being able to work effectively with others is just as crucial as writing good code.
4. The Algorithm That Wouldn’t Quit
Sometimes, the most challenging part of a coding interview isn’t coming up with a solution, but optimizing it. That was the case for Priya, a computer science graduate interviewing for her first job out of college.
Priya’s task was to implement an efficient algorithm for finding the kth largest element in an unsorted array. She quickly came up with a solution using QuickSelect, an algorithm she had recently studied.
“I felt pretty good about my solution,” Priya recalls. “It had an average time complexity of O(n) and worked correctly for all the test cases.”
However, the interviewer wasn’t satisfied. “Can you think of a way to improve the worst-case time complexity?” they asked.
Priya spent the next 20 minutes brainstorming and discussing various approaches with the interviewer. Finally, she had a breakthrough.
“I realized we could use a hybrid approach, combining QuickSelect with a heap-based method for smaller subarrays,” she explains. “This improved the worst-case time complexity to O(n log k) while maintaining the average-case O(n) performance.”
The interviewer was impressed not just with Priya’s final solution, but with her persistence and ability to iterate on her approach.
This story demonstrates that in coding interviews, the journey is often as important as the destination. Interviewers want to see how you approach problems and how you handle challenges along the way.
5. The Unexpected Hardware Question
Our next tale comes from David, a software engineer interviewing for a position at a major tech company known for its innovative hardware products. David had prepared extensively for algorithm and data structure questions, but what he encountered was something entirely different.
“The interviewer placed a computer mouse on the table and said, ‘Explain to me how this works,'” David recounts. “I was completely caught off guard. I’m a software guy, not a hardware engineer!”
Despite his initial panic, David took a deep breath and started breaking down the problem. He talked about the basic components he could see – the buttons, the scroll wheel, and the optical sensor underneath. Then he delved into how these might interact with the computer’s operating system.
“I realized they weren’t looking for a detailed technical explanation of the electronics,” David says. “They wanted to see how I would approach explaining a complex system in simple terms.”
David’s ability to think on his feet and communicate clearly about an unfamiliar topic impressed the interviewer. This experience taught him that technical interviews can sometimes venture beyond pure coding and into broader problem-solving and communication skills.
6. The Real-World Problem Solver
Sometimes, coding interviews can take unexpected turns into real-world problem-solving. That’s what happened to Emma, a full-stack developer interviewing at a startup focused on optimizing urban transportation.
“I was prepared for typical coding challenges,” Emma says, “but instead, the interviewer asked me to design an algorithm to optimize traffic light timings in a busy city intersection.”
Emma was initially overwhelmed by the complexity of the problem. She started by asking clarifying questions about the intersection layout, traffic patterns, and available data sources.
“As I dug into the problem, I realized it was similar to many algorithmic challenges I had practiced,” Emma explains. “It involved graph theory, optimization, and even a bit of machine learning to predict traffic patterns.”
She outlined an approach that combined real-time data analysis with a dynamic programming algorithm to adjust light timings. The interviewer was impressed not just with her technical solution, but with how she related the abstract problem to real-world constraints and considerations.
“This experience taught me the importance of being able to apply algorithmic thinking to practical, messy real-world problems,” Emma reflects.
7. The Debugging Detective
Our next story comes from Carlos, a backend developer who faced an unusual challenge during his interview at a major e-commerce company.
“The interviewer showed me a piece of code and told me it had a bug,” Carlos recalls. “My task was to find and fix the bug, but with a twist – I couldn’t run the code or use any debugging tools. I had to reason through it purely by reading and analyzing the code.”
The code was a complex function handling user authentication and session management. Carlos carefully read through each line, mentally tracing the execution flow and considering edge cases.
“It was like being a detective, looking for clues and following leads,” he says. “After about 15 minutes, I spotted an issue in how the function was handling expired sessions.”
Carlos explained his findings to the interviewer, detailing how the bug could lead to security vulnerabilities. He then proposed a fix and discussed how he would test it in a real-world scenario.
“This experience reinforced for me the importance of being able to read and understand code, not just write it,” Carlos reflects. “It also showed me how critical it is to always be thinking about security and edge cases in authentication systems.”
8. The Algorithmic Time Traveler
Sometimes, coding interviews can take you on a journey through computer science history. That’s what happened to Zoe, a software engineer interviewing at a cutting-edge AI research company.
“The interviewer asked me to implement a sorting algorithm,” Zoe begins. “But here’s the catch – I had to pretend I was a computer scientist in the 1950s, before QuickSort or MergeSort were invented.”
Zoe found herself having to think creatively within historical constraints. She couldn’t rely on her go-to sorting algorithms and had to consider the limitations of 1950s computing hardware.
“I ended up implementing a variation of Bubble Sort with some optimizations,” Zoe explains. “It wasn’t the most efficient algorithm by modern standards, but it was a realistic solution given the constraints.”
The interviewer then engaged Zoe in a discussion about how sorting algorithms have evolved over time and the trade-offs between different approaches.
“This experience taught me the value of understanding the fundamentals and the history of computer science,” Zoe reflects. “It’s not just about knowing the latest algorithms, but understanding why they were developed and how they improve on earlier solutions.”
9. The Whiteboard Warrior
Our next tale comes from Raj, a mobile app developer who faced a daunting whiteboard challenge during his interview at a social media giant.
“The interviewer asked me to implement a simplified version of their news feed algorithm on the whiteboard,” Raj recalls. “I had to design data structures to represent users, posts, and interactions, and then write functions to generate a personalized feed for a given user.”
Raj started by sketching out the data structures and discussing the trade-offs of different representations. He then dove into implementing the core algorithm, talking through his thought process as he wrote the code.
“About halfway through, I realized I had made a mistake in my initial design,” Raj says. “Instead of panicking, I explained my realization to the interviewer and discussed how I would refactor the code to fix it.”
The interviewer appreciated Raj’s ability to recognize and address his own mistakes. They spent the rest of the interview discussing potential optimizations and how the algorithm might scale to millions of users.
“This experience taught me that interviews aren’t just about getting the right answer,” Raj reflects. “They’re about demonstrating your problem-solving process and how you handle challenges along the way.”
10. The Ethical Dilemma
Our final story comes from Maya, a data scientist interviewing at a major tech company. Her interview took an unexpected turn into the realm of ethics and social responsibility.
“The interviewer presented me with a scenario,” Maya begins. “We had developed an AI model that could predict a person’s likelihood of defaulting on a loan with high accuracy. The model used various data points, including some that could be considered sensitive or potentially discriminatory.”
The interviewer asked Maya to discuss the ethical implications of using such a model and how she would approach mitigating potential biases.
“I was caught off guard,” Maya admits. “I had prepared for technical questions about machine learning algorithms, not ethical debates. But as I started discussing the issue, I realized how crucial these considerations are in real-world data science work.”
Maya and the interviewer had a thoughtful discussion about algorithmic bias, data privacy, and the responsibilities of tech companies. They explored techniques for detecting and mitigating bias in AI models and discussed the importance of diverse teams in developing ethical AI systems.
“This experience opened my eyes to the broader impact of our work as technologists,” Maya reflects. “It’s not just about building clever algorithms; it’s about considering the real-world implications of what we create.”
Conclusion: Lessons from the Frontlines of Coding Interviews
These stories from the coding interview trenches offer valuable insights for anyone preparing for technical interviews:
- Expect the unexpected: Interviewers often throw curveballs to see how you handle unfamiliar situations. Stay calm and approach each challenge methodically.
- Communication is key: Your ability to explain your thought process clearly is just as important as your coding skills.
- Embrace collaboration: Many interviews now include pair programming or open-ended discussions. Show that you can work effectively with others.
- Don’t just settle for the first solution: Be prepared to optimize and iterate on your initial approaches.
- Understand the fundamentals: A solid grasp of computer science basics can help you tackle even the most unusual questions.
- Think beyond the code: Consider real-world applications, constraints, and ethical implications of your solutions.
- Stay calm under pressure: Mistakes happen. How you handle and recover from them is what matters.
- Be ready to think on your feet: Practice explaining complex concepts in simple terms, even for topics outside your immediate expertise.
- Consider the bigger picture: Understanding the context and impact of your work is increasingly important in tech roles.
Remember, the goal of these interviews isn’t just to test your coding skills, but to evaluate your problem-solving abilities, your communication skills, and how you approach challenges. By learning from these experiences and practicing regularly, you can improve your chances of success in your next coding interview.
At AlgoCademy, we’re committed to helping you prepare for these challenges. Our platform offers a wide range of coding tutorials, practice problems, and interview preparation resources. We focus on developing not just your coding skills, but also your problem-solving abilities and algorithmic thinking.
Whether you’re aiming for a position at a FAANG company or any other tech organization, remember that each interview is a learning experience. Even if you don’t get the job, you’ll gain valuable insights that will help you in future interviews and in your career as a whole.
Happy coding, and best of luck in your future interviews!