The Most In-Demand Programming Languages: Your Guide to Career Success in Tech
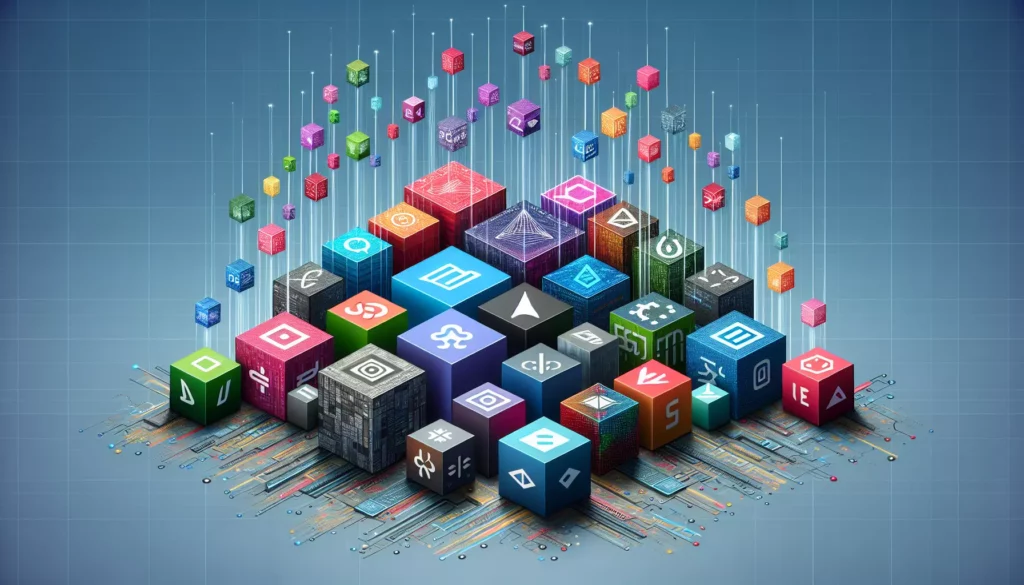
In today’s rapidly evolving tech landscape, staying ahead of the curve is crucial for aspiring developers and seasoned professionals alike. As the demand for skilled programmers continues to soar, understanding which programming languages are most sought-after can be the key to unlocking exciting career opportunities and ensuring long-term success in the field. In this comprehensive guide, we’ll explore the most in-demand programming languages, their applications, and why they’re essential for anyone looking to make their mark in the world of technology.
1. Python: The Jack of All Trades
Python has consistently ranked as one of the most popular and in-demand programming languages for several years, and for good reason. Its versatility, readability, and extensive library support make it an ideal choice for a wide range of applications.
Why Python is in High Demand:
- Data Science and Machine Learning: Python’s robust ecosystem of libraries like NumPy, Pandas, and TensorFlow has made it the go-to language for data analysis, machine learning, and artificial intelligence.
- Web Development: Frameworks like Django and Flask have solidified Python’s position in web development, allowing for rapid prototyping and scalable applications.
- Automation and Scripting: Python’s simplicity makes it perfect for automating repetitive tasks and writing scripts to improve workflow efficiency.
- Game Development: With libraries like Pygame, Python has found its place in game development, particularly for indie developers and educational purposes.
For beginners looking to enter the world of programming, Python offers a gentle learning curve while still providing powerful capabilities. Its syntax emphasizes readability, making it an excellent choice for those new to coding.
# Example of a simple Python function
def greet(name):
return f"Hello, {name}! Welcome to the world of Python."
print(greet("Alice"))
2. JavaScript: Powering the Web and Beyond
JavaScript has long been the backbone of interactive web development, but its influence has expanded far beyond the browser in recent years.
Why JavaScript is Essential:
- Front-end Development: As the primary language for client-side scripting, JavaScript is crucial for creating dynamic and responsive user interfaces.
- Full-stack Development: With Node.js, JavaScript has become a viable option for server-side programming, allowing developers to use a single language across the entire stack.
- Mobile App Development: Frameworks like React Native and Ionic enable developers to create cross-platform mobile applications using JavaScript.
- Desktop Applications: Electron.js has made it possible to build desktop applications using web technologies, expanding JavaScript’s reach even further.
The ubiquity of JavaScript in web development makes it an essential skill for any aspiring developer. Its ecosystem is vast, with countless libraries and frameworks that cater to various needs and preferences.
// Example of an asynchronous JavaScript function using Promises
function fetchData(url) {
return new Promise((resolve, reject) => {
fetch(url)
.then(response => response.json())
.then(data => resolve(data))
.catch(error => reject(error));
});
}
fetchData('https://api.example.com/data')
.then(data => console.log(data))
.catch(error => console.error('Error fetching data:', error));
3. Java: The Enterprise Staple
Despite being one of the older languages on this list, Java remains highly relevant and in-demand, particularly in enterprise environments.
Java’s Enduring Popularity:
- Enterprise Applications: Java’s robustness and scalability make it a preferred choice for building large-scale enterprise systems.
- Android Development: While Kotlin has gained traction, Java is still widely used for Android app development.
- Big Data: Frameworks like Apache Hadoop, which are often used in big data processing, are written in Java.
- Internet of Things (IoT): Java’s “write once, run anywhere” philosophy makes it suitable for IoT applications across various devices.
Java’s strong typing and object-oriented programming principles provide a solid foundation for developers, making it a valuable language to learn for those aiming for roles in large corporations or startups working on complex systems.
// Example of a Java class demonstrating encapsulation
public class BankAccount {
private double balance;
private String accountNumber;
public BankAccount(String accountNumber, double initialBalance) {
this.accountNumber = accountNumber;
this.balance = initialBalance;
}
public void deposit(double amount) {
if (amount > 0) {
balance += amount;
System.out.println("Deposit successful. New balance: " + balance);
} else {
System.out.println("Invalid deposit amount.");
}
}
public void withdraw(double amount) {
if (amount > 0 && amount <= balance) {
balance -= amount;
System.out.println("Withdrawal successful. New balance: " + balance);
} else {
System.out.println("Invalid withdrawal amount or insufficient funds.");
}
}
public double getBalance() {
return balance;
}
public String getAccountNumber() {
return accountNumber;
}
}
4. C++: Performance and Efficiency
C++ continues to be a powerhouse in the programming world, particularly in domains where performance and low-level control are critical.
C++’s Crucial Role:
- Game Development: Many game engines and AAA titles are built using C++ due to its performance capabilities.
- System Programming: Operating systems, device drivers, and embedded systems often rely on C++ for its low-level control and efficiency.
- Financial Systems: High-frequency trading platforms and other performance-critical financial applications frequently use C++.
- Graphics and Simulations: C++’s speed makes it ideal for computationally intensive tasks like 3D rendering and physics simulations.
While C++ has a steeper learning curve compared to some other languages, its performance benefits and wide range of applications make it a valuable skill for developers looking to work on cutting-edge projects.
// Example of a C++ class using templates for generic programming
template <typename T>
class Stack {
private:
std::vector<T> elements;
public:
void push(const T& item) {
elements.push_back(item);
}
T pop() {
if (elements.empty()) {
throw std::out_of_range("Stack is empty");
}
T top = elements.back();
elements.pop_back();
return top;
}
bool isEmpty() const {
return elements.empty();
}
size_t size() const {
return elements.size();
}
};
5. Go: Simplicity and Concurrency
Go, also known as Golang, has gained significant traction in recent years, particularly in the realm of cloud computing and microservices.
Go’s Rising Popularity:
- Cloud and Network Services: Go’s efficient handling of concurrent operations makes it ideal for building scalable network services and cloud infrastructure.
- DevOps and Site Reliability Engineering: Many popular DevOps tools, such as Docker and Kubernetes, are written in Go.
- Microservices: Go’s simplicity and performance characteristics make it well-suited for developing microservices architectures.
- Command-line Tools: Go’s fast compilation and cross-platform support make it an excellent choice for building CLI applications.
Go’s emphasis on simplicity and readability, combined with its powerful concurrency features, has made it increasingly popular among developers and organizations looking to build efficient, scalable systems.
// Example of a Go function demonstrating goroutines and channels
func fetchURLs(urls []string) []string {
ch := make(chan string)
results := []string{}
for _, url := range urls {
go func(url string) {
resp, err := http.Get(url)
if err != nil {
ch <- fmt.Sprintf("Error fetching %s: %v", url, err)
return
}
defer resp.Body.Close()
ch <- fmt.Sprintf("Successfully fetched %s", url)
}(url)
}
for range urls {
results = append(results, <-ch)
}
return results
}
6. TypeScript: JavaScript with Superpowers
TypeScript, a superset of JavaScript, has seen a surge in popularity due to its ability to add static typing to JavaScript projects.
TypeScript’s Growing Influence:
- Large-scale Applications: TypeScript’s type system helps catch errors early and improves code maintainability in large codebases.
- Angular Development: Angular, one of the most popular front-end frameworks, is built with TypeScript, making it a must-know for Angular developers.
- Enhanced Developer Experience: TypeScript’s intelligent code completion and refactoring tools boost developer productivity.
- Cross-platform Development: Like JavaScript, TypeScript can be used for web, mobile, and desktop application development.
For developers already familiar with JavaScript, learning TypeScript can significantly enhance their skills and open up new career opportunities, especially in enterprise environments.
// Example of a TypeScript interface and class
interface Shape {
area(): number;
perimeter(): number;
}
class Circle implements Shape {
constructor(private radius: number) {}
area(): number {
return Math.PI * this.radius ** 2;
}
perimeter(): number {
return 2 * Math.PI * this.radius;
}
}
const circle = new Circle(5);
console.log(`Area: ${circle.area()}`);
console.log(`Perimeter: ${circle.perimeter()}`);
7. Swift: The Apple Ecosystem Language
Swift has become the primary language for developing applications within the Apple ecosystem, including iOS, macOS, watchOS, and tvOS.
Swift’s Importance in Mobile Development:
- iOS App Development: Swift is the preferred language for building native iOS applications, making it essential for mobile developers targeting Apple devices.
- macOS Applications: Swift is also used for developing desktop applications for Mac computers.
- Server-side Development: With frameworks like Vapor, Swift is expanding into server-side development, allowing for full-stack Swift applications.
- Performance and Safety: Swift’s design focuses on performance and safety, making it an attractive option for developing robust applications.
For developers looking to break into the lucrative iOS app market or build applications for Apple platforms, Swift is an indispensable language to learn.
// Example of a Swift protocol and struct
protocol Vehicle {
var numberOfWheels: Int { get }
func start()
func stop()
}
struct Car: Vehicle {
let numberOfWheels: Int = 4
func start() {
print("Car engine started")
}
func stop() {
print("Car engine stopped")
}
}
let myCar = Car()
myCar.start()
print("Number of wheels: \(myCar.numberOfWheels)")
myCar.stop()
8. Rust: Safety and Concurrency
Rust has been gaining traction among developers due to its focus on memory safety and concurrent programming without sacrificing performance.
Rust’s Unique Advantages:
- Systems Programming: Rust is well-suited for low-level system programming, offering a safer alternative to languages like C and C++.
- Web Assembly: Rust’s compatibility with WebAssembly makes it a powerful tool for high-performance web applications.
- Blockchain Development: Many blockchain projects are turning to Rust for its safety guarantees and performance.
- Game Development: Rust’s performance characteristics make it an attractive option for game engines and high-performance game components.
While Rust has a steeper learning curve compared to some other languages on this list, its unique features and growing ecosystem make it a valuable skill for developers looking to work on cutting-edge projects.
// Example of Rust's ownership system and error handling
fn main() {
let s1 = String::from("hello");
let s2 = s1.clone(); // Create a deep copy of s1
println!("s1 = {}, s2 = {}", s1, s2);
let result = divide(10, 2);
match result {
Ok(value) => println!("Result: {}", value),
Err(e) => println!("Error: {}", e),
}
}
fn divide(a: i32, b: i32) -> Result<i32, String> {
if b == 0 {
Err(String::from("Cannot divide by zero"))
} else {
Ok(a / b)
}
}
Choosing the Right Language for Your Career
While the languages listed above are among the most in-demand, it’s important to remember that the best language for you depends on your career goals, interests, and the specific domain you want to work in. Here are some tips for choosing the right programming language to focus on:
- Assess Your Career Goals: Consider the type of projects or industries you’re interested in. For example, if you’re passionate about mobile app development, focusing on Swift (for iOS) or Kotlin/Java (for Android) might be the best path.
- Research Job Market Trends: Look at job postings in your area or desired location to see which languages are most frequently requested by employers.
- Consider Your Background: If you’re coming from a specific field, like data analysis or design, certain languages might align better with your existing skills.
- Start with Versatile Languages: If you’re unsure, beginning with a versatile language like Python or JavaScript can provide a solid foundation and open doors to various specializations.
- Don’t Limit Yourself: While it’s important to develop expertise in one or two languages, being familiar with multiple languages can make you a more well-rounded and adaptable developer.
The Importance of Continuous Learning
In the fast-paced world of technology, the landscape of programming languages is constantly evolving. New languages emerge, existing ones update, and best practices change. To stay competitive in the job market and continue growing as a developer, it’s crucial to adopt a mindset of continuous learning.
Strategies for Staying Up-to-Date:
- Follow Industry Trends: Subscribe to tech blogs, podcasts, and newsletters to stay informed about the latest developments in programming languages and technologies.
- Participate in Coding Communities: Engage with other developers through platforms like GitHub, Stack Overflow, or local meetups to share knowledge and learn from peers.
- Work on Personal Projects: Apply your skills to personal projects or open-source contributions to gain practical experience with new languages and frameworks.
- Take Online Courses: Platforms like Coursera, edX, and Udacity offer courses on various programming languages and computer science topics, often taught by industry experts.
- Attend Conferences and Workshops: Technology conferences and workshops provide opportunities to learn about cutting-edge developments and network with other professionals in the field.
Conclusion: Embracing the Journey of Programming
The world of programming is vast and ever-changing, offering endless opportunities for those willing to learn and adapt. While the languages discussed in this article are currently among the most in-demand, the key to long-term success in the field lies not just in mastering specific languages, but in developing a deep understanding of programming concepts, problem-solving skills, and the ability to learn new technologies quickly.
As you embark on or continue your journey in programming, remember that each language you learn broadens your perspective and enhances your ability to tackle complex problems. Whether you’re just starting out or looking to expand your skill set, focus on building a strong foundation in core programming principles, and don’t be afraid to explore new languages and paradigms.
The most successful developers are those who view coding not just as a skill, but as a craft to be continually honed and refined. By staying curious, embracing challenges, and maintaining a passion for learning, you’ll be well-equipped to thrive in the dynamic and rewarding field of software development.