The Ethics of Coding: What Developers Should Know
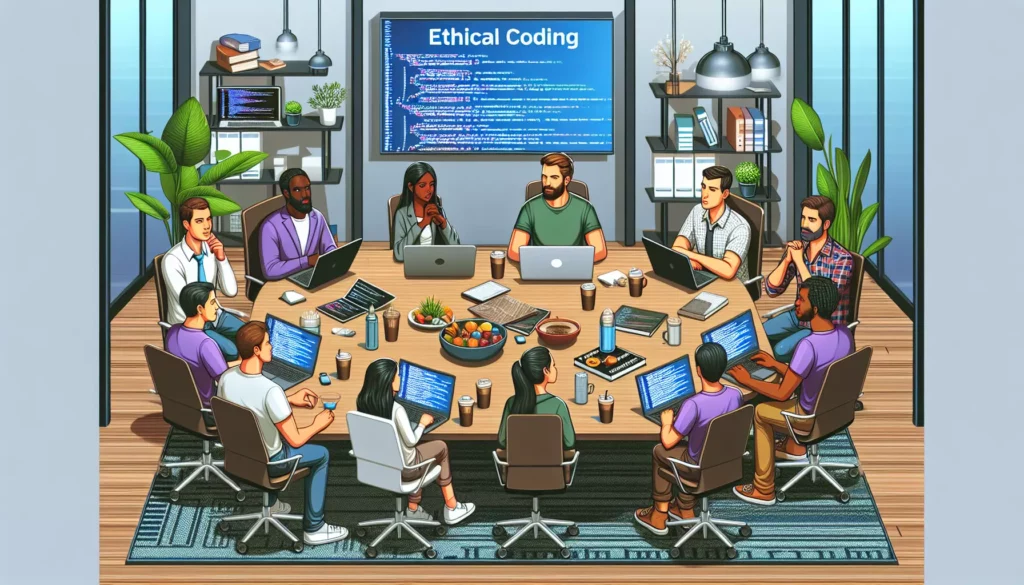
In the rapidly evolving world of technology, coding has become an essential skill that powers our digital landscape. From mobile apps to complex artificial intelligence systems, code is the backbone of our modern society. However, as developers, we have a responsibility that extends beyond just writing functional code. We must consider the ethical implications of our work and how it impacts individuals, communities, and society as a whole. In this comprehensive guide, we’ll explore the ethics of coding and what every developer should know to navigate this crucial aspect of their profession.
1. Understanding the Importance of Ethics in Coding
Ethics in coding is not just a theoretical concept; it has real-world consequences that can affect millions of people. As developers, we hold significant power in shaping the digital world, and with that power comes great responsibility. Here’s why ethics in coding matters:
- Impact on Society: The code we write can influence how people interact, access information, and make decisions.
- Privacy Concerns: With the increasing amount of personal data being collected and processed, ethical coding practices are crucial for protecting user privacy.
- Fairness and Equality: Algorithms and systems we develop can perpetuate or combat biases and discrimination.
- Safety and Security: Ethical coding practices are essential for creating secure systems that protect users from harm.
- Trust in Technology: Ethical coding helps maintain public trust in the technologies we create and use daily.
2. Key Ethical Principles for Developers
To navigate the complex landscape of coding ethics, developers should be familiar with several key principles:
2.1 Transparency
Transparency involves being open about how your code works, its limitations, and potential impacts. This includes:
- Clearly documenting your code and its functionality
- Disclosing the use of algorithms and AI in user-facing applications
- Being honest about the capabilities and limitations of your software
2.2 Privacy and Data Protection
Respecting user privacy and protecting personal data should be a top priority:
- Implement strong data encryption and security measures
- Collect only necessary data and provide clear opt-in/opt-out options
- Adhere to data protection regulations like GDPR and CCPA
2.3 Fairness and Non-discrimination
Ensure your code and algorithms treat all users fairly:
- Test for and mitigate algorithmic bias
- Consider diverse user groups in your design and development process
- Avoid reinforcing societal stereotypes or prejudices
2.4 Accountability
Take responsibility for the code you write and its consequences:
- Implement proper error handling and logging
- Be prepared to explain and justify your coding decisions
- Establish clear lines of responsibility within development teams
2.5 Safety and Reliability
Prioritize the safety and reliability of your code:
- Conduct thorough testing, including edge cases and potential misuse scenarios
- Implement fail-safes and error recovery mechanisms
- Regularly update and maintain your code to address vulnerabilities
3. Ethical Challenges in Different Coding Domains
Different areas of software development present unique ethical challenges. Let’s explore some specific domains and the ethical considerations they entail:
3.1 Artificial Intelligence and Machine Learning
AI and ML systems pose significant ethical challenges due to their ability to make autonomous decisions and learn from data:
- Bias in Training Data: Ensure diverse and representative training data to avoid perpetuating societal biases.
- Explainability: Strive for transparent AI systems that can explain their decision-making processes.
- Human Oversight: Implement human-in-the-loop systems for critical decisions.
Example of addressing bias in machine learning:
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import confusion_matrix, classification_report
# Load and preprocess the data
data = pd.read_csv("employee_data.csv")
X = data.drop(["employee_id", "promotion", "gender", "race"], axis=1)
y = data["promotion"]
# Split the data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Scale the features
scaler = StandardScaler()
X_train_scaled = scaler.fit_transform(X_train)
X_test_scaled = scaler.transform(X_test)
# Train the model
model = LogisticRegression()
model.fit(X_train_scaled, y_train)
# Make predictions
y_pred = model.predict(X_test_scaled)
# Evaluate the model
print(confusion_matrix(y_test, y_pred))
print(classification_report(y_test, y_pred))
# Check for bias
for feature in ["gender", "race"]:
print(f"\nPromotion rate by {feature}:")
print(data.groupby(feature)["promotion"].mean())
This code snippet demonstrates how to check for bias in a machine learning model for employee promotions. It includes steps to evaluate the model’s performance and examine promotion rates across different demographic groups.
3.2 Web Development and User Interface Design
Web developers and UI designers must consider:
- Accessibility: Ensure websites and applications are usable by people with disabilities.
- Dark Patterns: Avoid deceptive UI practices that manipulate user behavior.
- Data Collection: Be transparent about data collection and provide easy opt-out options.
Example of implementing accessible web design:
<!-- HTML -->
<button aria-label="Close menu" class="close-button">
<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 24 24" width="24" height="24">
<path d="M19 6.41L17.59 5 12 10.59 6.41 5 5 6.41 10.59 12 5 17.59 6.41 19 12 13.41 17.59 19 19 17.59 13.41 12z"/>
</svg>
</button>
/* CSS */
.close-button {
background: none;
border: none;
cursor: pointer;
padding: 8px;
}
.close-button:focus {
outline: 2px solid #007bff;
outline-offset: 2px;
}
/* For users who prefer reduced motion */
@media (prefers-reduced-motion: reduce) {
* {
animation: none !important;
transition: none !important;
}
}
This code demonstrates accessible design practices by using ARIA labels, ensuring keyboard navigability, and respecting user preferences for reduced motion.
3.3 Cybersecurity and Ethical Hacking
Security professionals face unique ethical challenges:
- Responsible Disclosure: Follow proper channels when reporting vulnerabilities.
- Privacy vs. Security: Balance security measures with user privacy rights.
- Ethical Use of Hacking Skills: Use skills for protection, not exploitation.
3.4 Data Science and Big Data
Data scientists must navigate ethical issues related to:
- Data Privacy: Protect individual privacy when working with large datasets.
- Informed Consent: Ensure data subjects understand how their data will be used.
- Responsible Data Stewardship: Handle data securely and use it only for intended purposes.
4. Implementing Ethical Coding Practices
Now that we’ve explored the principles and challenges, let’s discuss how to implement ethical coding practices in your work:
4.1 Code Reviews with an Ethical Lens
Incorporate ethical considerations into your code review process:
- Include ethics-focused questions in your code review checklist
- Encourage team members to flag potential ethical issues
- Discuss ethical implications of new features or algorithms
4.2 Ethical Impact Assessments
Before starting a new project or implementing a significant feature, conduct an ethical impact assessment:
- Identify potential stakeholders and how they might be affected
- Evaluate possible unintended consequences
- Develop mitigation strategies for identified risks
4.3 Diverse and Inclusive Development Teams
Foster diversity in your development team to bring different perspectives to ethical considerations:
- Actively recruit from underrepresented groups in tech
- Create an inclusive work environment that values diverse viewpoints
- Encourage team members to speak up about ethical concerns
4.4 Continuous Learning and Adaptation
Stay informed about evolving ethical issues in technology:
- Attend conferences and workshops on tech ethics
- Follow thought leaders and organizations focused on ethical technology
- Regularly review and update your ethical coding guidelines
4.5 Ethical Documentation and Transparency
Document your ethical considerations and decision-making processes:
- Include ethical considerations in project documentation
- Clearly communicate the ethical principles guiding your work to stakeholders
- Be transparent about known limitations or potential biases in your systems
5. Case Studies: Ethical Dilemmas in Coding
Let’s examine some real-world case studies that highlight ethical dilemmas in coding:
5.1 The Volkswagen Emissions Scandal
In 2015, it was discovered that Volkswagen had programmed their diesel engines to activate emission controls only during laboratory testing, allowing them to pass emissions tests while polluting heavily during real-world driving.
Ethical Lessons:
- The importance of transparency and honesty in coding practices
- The potential for code to be used for deception and the responsibility of developers to resist such practices
- The far-reaching consequences of unethical coding decisions on public health and trust
5.2 Cambridge Analytica and Facebook Data Misuse
Cambridge Analytica harvested personal data from millions of Facebook profiles without user consent, using it for political advertising purposes.
Ethical Lessons:
- The critical importance of data privacy and user consent
- The potential for data misuse and the need for robust safeguards
- The responsibility of platforms to protect user data from unauthorized access and use
5.3 AI Bias in Criminal Justice Systems
Some AI systems used in criminal justice for risk assessment have been found to exhibit racial bias, potentially leading to unfair treatment of certain groups.
Ethical Lessons:
- The importance of diverse and representative training data in AI systems
- The need for ongoing monitoring and auditing of AI systems for bias
- The potential for algorithms to perpetuate and amplify societal biases
6. Tools and Resources for Ethical Coding
To support your journey in ethical coding, consider these tools and resources:
6.1 Ethical AI Frameworks
6.2 Privacy and Security Tools
- OWASP Top Ten – A powerful awareness document for web application security
- Privacy Patterns – A repository of design solutions for common privacy problems
6.3 Accessibility Tools
6.4 Ethical Coding Communities and Organizations
- IEEE Ethics in Action
- ACM Code of Ethics and Professional Conduct
- ACM Committee on Professional Ethics
7. The Future of Ethical Coding
As technology continues to advance, the importance of ethical coding will only grow. Here are some trends and considerations for the future:
7.1 Emerging Technologies and New Ethical Challenges
As we develop more advanced technologies like quantum computing, brain-computer interfaces, and advanced AI systems, new ethical challenges will emerge. Developers will need to stay informed and adaptable to address these evolving concerns.
7.2 Increased Regulation and Compliance
We can expect to see more regulations around data privacy, AI ethics, and algorithmic transparency. Developers will need to be well-versed in these regulations and incorporate compliance into their coding practices.
7.3 Ethics-by-Design Approaches
Just as we have security-by-design and privacy-by-design, we may see the rise of ethics-by-design approaches, where ethical considerations are built into the software development lifecycle from the very beginning.
7.4 Ethical AI Assistants
As AI becomes more integrated into the development process, we might see the emergence of AI assistants specifically designed to help identify and address ethical issues in code.
7.5 Interdisciplinary Collaboration
Ethical coding will likely require increased collaboration between technologists, ethicists, policymakers, and domain experts to ensure a comprehensive approach to addressing ethical challenges.
Conclusion
The ethics of coding is a complex and evolving field that touches every aspect of software development. As developers, we have the power to shape the digital world, and with that power comes the responsibility to consider the ethical implications of our work. By understanding key ethical principles, implementing ethical coding practices, and staying informed about emerging challenges, we can create technology that not only functions well but also contributes positively to society.
Remember, ethical coding is not a destination but a journey. It requires ongoing learning, reflection, and adaptation. As you continue your development career, make ethical considerations a central part of your coding practice. By doing so, you’ll not only become a better developer but also contribute to a more equitable, safe, and trustworthy digital future for all.
The code you write has the potential to impact millions of lives. Make sure that impact is a positive one. Happy coding, and may your algorithms be both efficient and ethical!