10 Projects to Boost Your Programming Skills
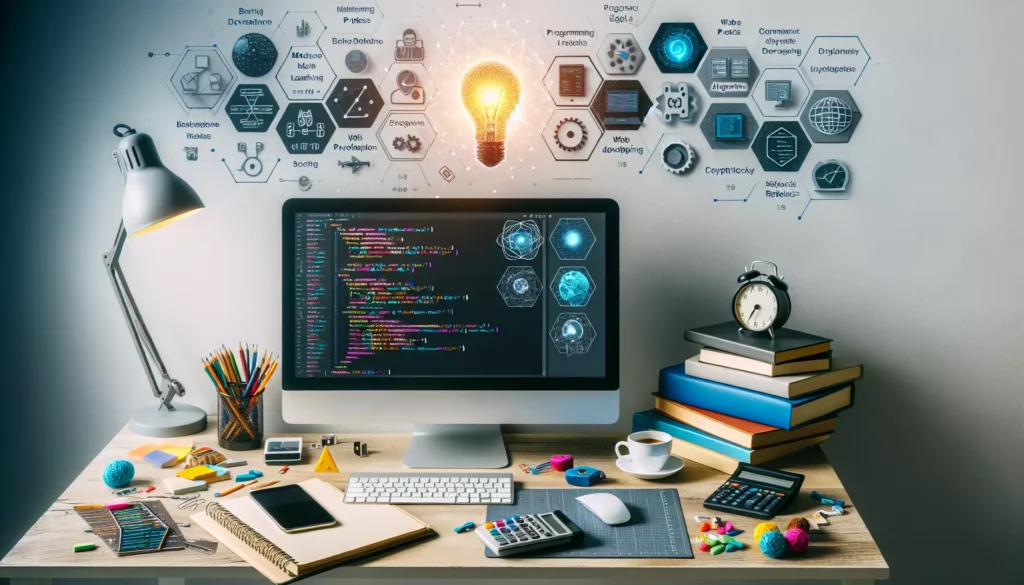
Are you looking to take your programming skills to the next level? Whether you’re a beginner eager to solidify your foundation or an intermediate coder aiming to expand your expertise, engaging in hands-on projects is one of the best ways to enhance your abilities. In this comprehensive guide, we’ll explore 10 exciting projects that will not only challenge you but also significantly boost your programming prowess. These projects are designed to cover a wide range of skills, from algorithmic thinking to practical application development, aligning perfectly with the learning philosophy of platforms like AlgoCademy.
1. Build a Personal Portfolio Website
Creating a personal portfolio website is an excellent starting point for any programmer. This project allows you to showcase your skills while learning or improving your web development abilities.
Key Skills Developed:
- HTML5 and CSS3
- Responsive design
- Basic JavaScript for interactivity
- Version control with Git
Project Outline:
- Design a layout for your portfolio
- Create the HTML structure
- Style your website with CSS
- Add interactivity with JavaScript
- Make it responsive for various screen sizes
- Deploy your website using GitHub Pages or a similar service
This project not only helps you learn web technologies but also provides you with a platform to showcase your other projects and skills to potential employers or clients.
2. Develop a To-Do List Application
A to-do list application is a classic project that covers many fundamental programming concepts. It’s an excellent way to practice working with data structures and basic CRUD (Create, Read, Update, Delete) operations.
Key Skills Developed:
- Data structures (arrays, objects)
- DOM manipulation
- Local storage for data persistence
- Event handling
Project Outline:
- Create a user interface for adding, viewing, and managing tasks
- Implement functionality to add new tasks
- Allow users to mark tasks as complete
- Add the ability to edit and delete tasks
- Implement data persistence using local storage
- Add sorting or filtering options for tasks
This project will give you hands-on experience with fundamental programming concepts and prepare you for more complex application development.
3. Create a Weather App
Building a weather application is an excellent way to learn about working with APIs and asynchronous programming. It’s also a practical project that you can use in your daily life.
Key Skills Developed:
- API integration
- Asynchronous JavaScript (Promises, async/await)
- JSON parsing
- Geolocation
Project Outline:
- Sign up for a weather API (e.g., OpenWeatherMap)
- Create a user interface to display weather information
- Implement geolocation to get the user’s current location
- Fetch weather data from the API based on location
- Parse and display the weather information
- Add features like a 5-day forecast or weather maps
This project will give you valuable experience in working with real-world data and APIs, skills that are crucial in many professional development scenarios.
4. Build a Simple Blockchain
Creating a basic blockchain is an excellent way to understand the fundamental concepts behind this revolutionary technology. It also provides practice in working with cryptographic functions and data structures.
Key Skills Developed:
- Cryptography basics
- Hash functions
- Linked list data structure
- Distributed systems concepts
Project Outline:
- Implement a basic Block class with properties like index, timestamp, data, and hash
- Create a function to calculate the hash of a block
- Implement a Blockchain class to manage the chain of blocks
- Add methods to add new blocks and validate the chain
- Implement a simple proof-of-work algorithm
- Create a basic interface to interact with your blockchain
This project will give you insights into the workings of blockchain technology and improve your understanding of cryptographic concepts.
5. Develop a URL Shortener
A URL shortener is a practical project that involves working with databases, web frameworks, and URL manipulation. It’s a great way to learn about backend development and RESTful API design.
Key Skills Developed:
- Backend development (e.g., Node.js with Express)
- Database management (e.g., MongoDB)
- RESTful API design
- URL parsing and manipulation
Project Outline:
- Set up a backend server using a framework like Express
- Create a database to store long and short URLs
- Implement an algorithm to generate short URLs
- Create API endpoints for shortening URLs and redirecting
- Build a simple frontend for users to input long URLs
- Implement analytics to track clicks on shortened URLs
This project will give you experience in full-stack development and working with databases, essential skills for many programming roles.
6. Create a Chat Application
Building a real-time chat application is an excellent way to learn about websockets and real-time communication in web applications. It’s also a fun project that can be extended with various features.
Key Skills Developed:
- Real-time communication with WebSockets
- Server-side programming
- User authentication
- Frontend frameworks (e.g., React)
Project Outline:
- Set up a server using Node.js and a WebSocket library like Socket.io
- Create a simple chat interface using HTML, CSS, and JavaScript
- Implement real-time message sending and receiving
- Add user authentication to allow users to log in
- Implement private messaging between users
- Add features like emojis, file sharing, or group chats
This project will give you hands-on experience with real-time web applications, a valuable skill in today’s interconnected world.
7. Build a Simple Search Engine
Creating a basic search engine is an excellent way to learn about information retrieval, indexing, and search algorithms. It’s a challenging project that will significantly improve your algorithmic thinking.
Key Skills Developed:
- Text processing and indexing
- Search algorithms (e.g., TF-IDF)
- Data structures for efficient searching
- Web crawling basics
Project Outline:
- Create a simple web crawler to gather text from websites
- Implement text processing to clean and tokenize the text
- Build an inverted index to store word-document relationships
- Implement a basic search algorithm (e.g., TF-IDF)
- Create a simple user interface for searching
- Optimize your search for better performance
This project will give you insights into how search engines work and improve your skills in working with large datasets and complex algorithms.
8. Develop a Simple Machine Learning Model
Creating a basic machine learning model is an excellent way to dive into the world of AI and data science. It’s a great project to understand the fundamentals of machine learning algorithms.
Key Skills Developed:
- Basic machine learning concepts
- Data preprocessing
- Python programming
- Libraries like NumPy, Pandas, and Scikit-learn
Project Outline:
- Choose a simple dataset (e.g., Iris dataset)
- Preprocess and explore the data
- Split the data into training and testing sets
- Implement a simple algorithm (e.g., k-Nearest Neighbors)
- Train the model and make predictions
- Evaluate the model’s performance
This project will introduce you to the basics of machine learning and data science, increasingly important fields in the tech industry.
9. Create a RESTful API
Building a RESTful API is crucial for understanding how modern web applications communicate. This project will help you grasp the concepts of API design and implementation.
Key Skills Developed:
- RESTful API design principles
- Backend framework (e.g., Express.js, Django)
- Database integration
- Authentication and security
Project Outline:
- Choose a domain for your API (e.g., a book library)
- Design your API endpoints following REST principles
- Implement CRUD operations for your resources
- Add database integration to store and retrieve data
- Implement authentication and authorization
- Document your API using tools like Swagger
This project will give you valuable experience in backend development and API design, skills that are in high demand in the industry.
10. Build a Simple Compiler
Creating a simple compiler is an advanced project that will deepen your understanding of programming languages and how they work under the hood. It’s a challenging but rewarding project.
Key Skills Developed:
- Lexical analysis
- Parsing techniques
- Abstract Syntax Trees (ASTs)
- Code generation
Project Outline:
- Define a simple language syntax
- Implement a lexer to tokenize input
- Create a parser to generate an AST
- Implement semantic analysis
- Generate code (e.g., to a stack-based VM)
- Create a simple virtual machine to run the generated code
This project will give you a deep understanding of how programming languages work and significantly improve your problem-solving skills.
Conclusion
Embarking on these projects will not only boost your programming skills but also give you valuable experience in various aspects of software development. From frontend design to backend implementation, from working with APIs to understanding complex algorithms, these projects cover a wide range of skills that are highly valued in the tech industry.
Remember, the key to learning is consistency and practice. Don’t be discouraged if you face challenges along the way – they are part of the learning process. Use resources like AlgoCademy to help you understand the underlying concepts and algorithms needed for these projects. Their interactive coding tutorials and AI-powered assistance can be invaluable as you work through these challenges.
As you complete each project, make sure to document your process, the challenges you faced, and how you overcame them. This documentation will not only help solidify your learning but also serve as a great addition to your portfolio, showcasing your skills and problem-solving abilities to potential employers.
Happy coding, and remember – every line of code you write is a step forward in your programming journey!