The Science Behind Efficient Algorithms: Unlocking the Power of Optimal Problem-Solving
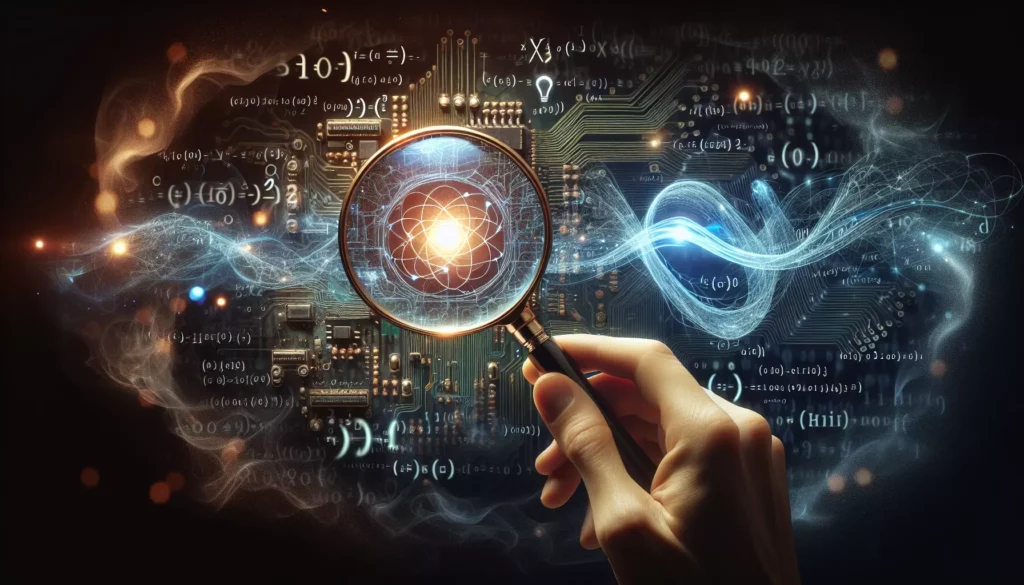
In the world of computer science and programming, algorithms are the unsung heroes that power everything from simple sorting tasks to complex machine learning models. As we dive deeper into the digital age, understanding the science behind efficient algorithms becomes increasingly crucial. This comprehensive guide will explore the fundamental principles, techniques, and real-world applications of algorithm efficiency, providing you with the knowledge to optimize your code and solve problems more effectively.
What Are Algorithms and Why Do They Matter?
Before we delve into the intricacies of algorithm efficiency, let’s start with the basics. An algorithm is a step-by-step procedure or formula for solving a problem or accomplishing a task. In the context of computer science, algorithms are the foundation of all software and are essential for processing data, making decisions, and performing calculations.
The importance of algorithms cannot be overstated. They are the building blocks of computer programs and are responsible for:
- Sorting and searching large datasets
- Optimizing resource allocation
- Enabling artificial intelligence and machine learning
- Facilitating communication in computer networks
- Powering graphics rendering and image processing
As the scale and complexity of computational problems grow, the efficiency of algorithms becomes paramount. An efficient algorithm can mean the difference between a task completing in milliseconds versus hours, or between a program running smoothly on a smartphone versus requiring a supercomputer.
The Foundations of Algorithm Efficiency
Algorithm efficiency is typically measured in terms of two primary resources: time and space. Time complexity refers to how long an algorithm takes to run, while space complexity deals with how much memory it requires. Let’s explore these concepts in more detail:
Time Complexity
Time complexity is expressed using Big O notation, which describes the upper bound of an algorithm’s running time in relation to the input size. Common time complexities include:
- O(1) – Constant time
- O(log n) – Logarithmic time
- O(n) – Linear time
- O(n log n) – Linearithmic time
- O(n^2) – Quadratic time
- O(2^n) – Exponential time
Understanding these complexities is crucial for predicting how an algorithm will perform as the input size increases. For example, an O(n) algorithm will scale linearly with input size, while an O(n^2) algorithm will become increasingly slower for larger inputs.
Space Complexity
Space complexity refers to the amount of memory an algorithm uses in relation to the input size. Like time complexity, it’s also expressed using Big O notation. Common space complexities include:
- O(1) – Constant space
- O(n) – Linear space
- O(n^2) – Quadratic space
Efficient algorithms strive to minimize both time and space complexity, but often there are trade-offs between the two. For instance, an algorithm might use more memory to achieve faster execution times, or vice versa.
Key Techniques for Designing Efficient Algorithms
Now that we understand the basics of algorithm efficiency, let’s explore some key techniques used to design and optimize algorithms:
1. Divide and Conquer
The divide and conquer approach involves breaking a problem into smaller subproblems, solving them independently, and then combining the results. This technique is the basis for many efficient algorithms, including:
- Merge Sort (O(n log n) time complexity)
- Quick Sort (O(n log n) average-case time complexity)
- Binary Search (O(log n) time complexity)
Here’s a simple example of a binary search implementation in Python:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15]
result = binary_search(sorted_array, 7)
print(f"Element found at index: {result}")
2. Dynamic Programming
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It’s particularly useful for optimization problems and can significantly reduce time complexity by storing and reusing intermediate results. Famous examples include:
- Fibonacci sequence calculation
- Longest Common Subsequence
- Knapsack problem
Here’s an example of using dynamic programming to calculate Fibonacci numbers efficiently:
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Example usage
n = 10
result = fibonacci(n)
print(f"The {n}th Fibonacci number is: {result}")
3. Greedy Algorithms
Greedy algorithms make the locally optimal choice at each step, hoping to find a global optimum. While not always guaranteed to find the best overall solution, greedy algorithms are often simple to implement and can be very efficient. Examples include:
- Dijkstra’s algorithm for finding the shortest path in a graph
- Huffman coding for data compression
- Kruskal’s algorithm for minimum spanning trees
4. Hashing
Hashing is a technique that allows for O(1) average-case time complexity for insertion, deletion, and lookup operations. It’s widely used in database indexing, caching systems, and for implementing data structures like hash tables. Here’s a simple example of using a hash table in Python:
class SimpleHashTable:
def __init__(self, size):
self.size = size
self.table = [[] for _ in range(size)]
def _hash(self, key):
return hash(key) % self.size
def insert(self, key, value):
index = self._hash(key)
for item in self.table[index]:
if item[0] == key:
item[1] = value
return
self.table[index].append([key, value])
def get(self, key):
index = self._hash(key)
for item in self.table[index]:
if item[0] == key:
return item[1]
raise KeyError(key)
# Example usage
ht = SimpleHashTable(10)
ht.insert("apple", 5)
ht.insert("banana", 7)
print(ht.get("apple")) # Output: 5
Real-World Applications of Efficient Algorithms
The impact of efficient algorithms extends far beyond academic computer science. Let’s explore some real-world applications where algorithm efficiency makes a significant difference:
1. Search Engines
Search engines like Google rely on highly efficient algorithms to crawl, index, and rank billions of web pages. The PageRank algorithm, for instance, uses iterative methods to efficiently calculate the importance of web pages based on their link structure.
2. Financial Trading
In high-frequency trading, algorithms process market data and execute trades in microseconds. The efficiency of these algorithms can mean the difference between profit and loss in rapidly changing markets.
3. Route Planning
Navigation apps use algorithms like A* search or variants of Dijkstra’s algorithm to find the shortest or fastest route between two points. The efficiency of these algorithms is crucial for providing real-time navigation assistance.
4. Machine Learning and AI
Many machine learning algorithms, such as gradient descent for neural networks or decision tree algorithms, rely on efficient implementations to process large datasets and train models in reasonable timeframes.
5. Compression Algorithms
Data compression algorithms like JPEG for images or MP3 for audio use sophisticated techniques to reduce file sizes while maintaining quality. The efficiency of these algorithms directly impacts storage requirements and transmission speeds.
Challenges and Future Directions in Algorithm Efficiency
As we continue to push the boundaries of computing, new challenges and opportunities arise in the field of algorithm efficiency:
1. Quantum Computing
The advent of quantum computing presents both challenges and opportunities for algorithm design. Quantum algorithms like Shor’s algorithm for factoring large numbers promise exponential speedups over classical algorithms for certain problems.
2. Big Data and Scalability
With the explosion of data in the digital age, algorithms must be designed to handle enormous datasets efficiently. This has led to the development of distributed algorithms and frameworks like MapReduce for processing data across clusters of computers.
3. Energy Efficiency
As computing becomes more ubiquitous and energy concerns grow, there’s an increasing focus on designing algorithms that are not just time and space efficient, but also energy efficient.
4. Approximation Algorithms
For many NP-hard problems, finding exact solutions efficiently is impractical. Approximation algorithms that can quickly find near-optimal solutions are becoming increasingly important in practical applications.
5. Online and Streaming Algorithms
In scenarios where data arrives in a stream or where decisions must be made in real-time without access to all information, online and streaming algorithms are crucial. These algorithms must be designed to make efficient decisions with partial information.
Conclusion: The Ongoing Quest for Efficiency
The science behind efficient algorithms is a fascinating and ever-evolving field at the heart of computer science. As we’ve explored in this article, understanding and implementing efficient algorithms is crucial for solving complex problems, handling large-scale data, and pushing the boundaries of what’s computationally possible.
From the foundational concepts of time and space complexity to advanced techniques like dynamic programming and the challenges of quantum computing, the quest for more efficient algorithms continues to drive innovation in technology and beyond.
As you continue your journey in programming and computer science, remember that mastering efficient algorithms is not just about writing faster code—it’s about developing a problem-solving mindset that can tackle the most challenging computational problems of our time. Whether you’re optimizing a database query, developing a machine learning model, or creating the next breakthrough in quantum computing, the principles of algorithm efficiency will be your guide to creating elegant, powerful, and impactful solutions.
Keep exploring, keep optimizing, and never stop seeking the most efficient path to solving problems. The future of technology depends on the continuous improvement and innovation in algorithmic efficiency, and you have the power to be a part of that exciting journey.