The Ultimate Coding Interview Prep Checklist: Ace Your Technical Interviews
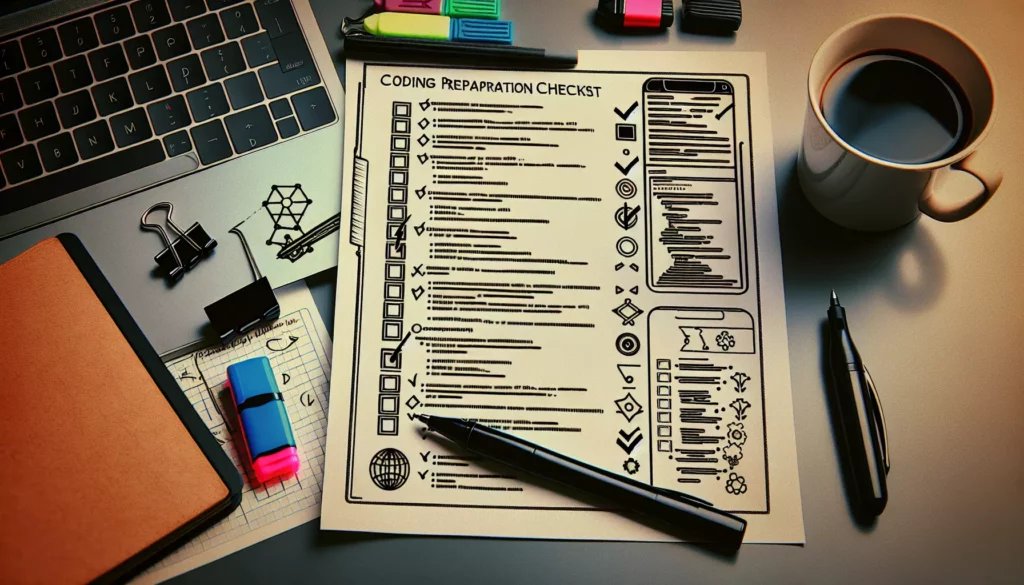
Are you gearing up for coding interviews with top tech companies? Whether you’re aiming for FAANG (Facebook, Amazon, Apple, Netflix, Google) or other prestigious tech firms, proper preparation is key to success. This comprehensive checklist will guide you through the essential steps to ace your technical interviews and land your dream job in software engineering.
Table of Contents
- Understanding the Interview Process
- Mastering Core Computer Science Concepts
- Reviewing Data Structures
- Brushing Up on Algorithms
- Honing Problem-Solving Skills
- Consistent Coding Practice
- System Design and Scalability
- Preparing for Behavioral Questions
- Conducting Mock Interviews
- Researching Target Companies
- Essential Tools and Resources
- Final Preparations Before the Interview
- Post-Interview Steps
- Conclusion
1. Understanding the Interview Process
Before diving into technical preparation, it’s crucial to understand the typical coding interview process at major tech companies:
- Initial Screening: Often a phone or video call with a recruiter to discuss your background and the role.
- Technical Phone Screen: A 45-60 minute interview focusing on coding and problem-solving.
- On-site Interviews: Usually 4-6 rounds covering coding, system design, and behavioral aspects.
- Follow-up: Additional interviews or take-home assignments (less common but possible).
Familiarizing yourself with this process helps you tailor your preparation and manage expectations.
2. Mastering Core Computer Science Concepts
A solid foundation in computer science fundamentals is essential. Review these key areas:
- Time and space complexity analysis (Big O notation)
- Object-oriented programming principles
- Memory management
- Concurrency and multithreading
- Networking basics (TCP/IP, HTTP)
- Database fundamentals (SQL vs. NoSQL, indexing, transactions)
- Operating systems concepts (processes, threads, memory management)
Understanding these concepts will help you approach problems more effectively and discuss solutions in-depth during interviews.
3. Reviewing Data Structures
Proficiency in various data structures is crucial for coding interviews. Make sure you’re comfortable with:
- Arrays and strings
- Linked lists (singly and doubly linked)
- Stacks and queues
- Trees (binary trees, binary search trees, balanced trees)
- Heaps
- Hash tables
- Graphs
- Tries
For each data structure, understand:
- Basic operations and their time complexities
- Common use cases
- Advantages and disadvantages
- Implementation details in your preferred programming language
Here’s a simple example of implementing a stack in Python:
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
def peek(self):
if not self.is_empty():
return self.items[-1]
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
4. Brushing Up on Algorithms
Algorithmic knowledge is a cornerstone of coding interviews. Focus on these key algorithms and techniques:
- Sorting algorithms (Quick Sort, Merge Sort, Heap Sort)
- Searching algorithms (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy algorithms
- Divide and Conquer
- Recursion and backtracking
- Two-pointer technique
- Sliding window
For each algorithm, practice implementing it from scratch and solving related problems. Here’s an example of a binary search implementation in Java:
public class BinarySearch {
public static int binarySearch(int[] arr, int target) {
int left = 0;
int right = arr.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Target not found
}
public static void main(String[] args) {
int[] arr = {1, 3, 5, 7, 9, 11, 13, 15};
int target = 7;
int result = binarySearch(arr, target);
System.out.println(result != -1 ? "Element found at index " + result : "Element not found");
}
}
5. Honing Problem-Solving Skills
Developing strong problem-solving skills is crucial for tackling coding challenges. Follow these steps to improve your approach:
- Understand the problem: Read the question carefully, identify key information, and ask clarifying questions if needed.
- Plan your approach: Break down the problem into smaller steps and consider different solutions.
- Start with a brute-force solution: Begin with a simple, working solution before optimizing.
- Optimize: Analyze your initial solution and look for ways to improve time and space complexity.
- Implement: Write clean, well-structured code to implement your solution.
- Test: Consider edge cases and test your code thoroughly.
- Explain: Practice articulating your thought process and explaining your solution clearly.
Remember, interviewers are often more interested in your problem-solving approach than the final solution itself.
6. Consistent Coding Practice
Regular practice is essential to sharpen your coding skills and build confidence. Here’s how to structure your practice:
- Solve problems daily: Aim for at least one coding problem per day.
- Use platforms like LeetCode, HackerRank, or CodeSignal: These offer a wide range of problems similar to those asked in interviews.
- Time yourself: Practice solving problems within a time limit to simulate interview conditions.
- Review solutions: After solving a problem, study other efficient solutions to learn alternative approaches.
- Focus on weak areas: Identify topics you struggle with and dedicate extra time to improving them.
- Implement data structures and algorithms from scratch: This deepens your understanding and improves your coding skills.
Consider creating a study plan that covers different topics each week, ensuring a well-rounded preparation.
7. System Design and Scalability
For senior roles and at companies like FAANG, system design questions are common. Prepare by:
- Studying distributed systems concepts
- Understanding scalability principles
- Familiarizing yourself with common system architectures
- Practicing designing systems like:
- URL shortener
- Social media feed
- Chat application
- File storage system
- Learning about load balancing, caching, and database sharding
- Exploring microservices architecture
When approaching system design questions, follow this framework:
- Clarify requirements and constraints
- Estimate scale (users, data volume, etc.)
- Design high-level architecture
- Deep dive into core components
- Identify and address bottlenecks
- Discuss trade-offs in your design
8. Preparing for Behavioral Questions
Don’t overlook the importance of behavioral interviews. They assess your soft skills and cultural fit. Prepare for questions like:
- “Tell me about a time when you faced a challenging technical problem.”
- “How do you handle disagreements with team members?”
- “Describe a project you’re proud of and your role in it.”
- “How do you stay updated with new technologies?”
Use the STAR method (Situation, Task, Action, Result) to structure your responses. Practice telling concise, impactful stories about your experiences.
9. Conducting Mock Interviews
Mock interviews are invaluable for realistic practice. Here’s how to make the most of them:
- Find a study buddy or use platforms like Pramp for peer mock interviews
- Simulate real interview conditions (use a whiteboard or shared coding environment)
- Practice thinking out loud and explaining your approach
- Ask for feedback on your problem-solving approach, communication, and code quality
- Alternate between being the interviewer and interviewee to gain different perspectives
Aim to do at least 3-5 mock interviews before your actual interview to build confidence and identify areas for improvement.
10. Researching Target Companies
Tailoring your preparation to specific companies can give you an edge. For each company you’re interviewing with:
- Study their products and technologies
- Read about their company culture and values
- Review recent news and developments
- Understand their interview process and any unique aspects
- Prepare thoughtful questions to ask your interviewers
This research demonstrates your genuine interest and helps you align your responses with the company’s expectations.
11. Essential Tools and Resources
Equip yourself with the right tools and resources for effective preparation:
- Books:
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Elements of Programming Interviews” by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash
- “System Design Interview” by Alex Xu
- Online Platforms:
- LeetCode
- HackerRank
- AlgoExpert
- InterviewBit
- YouTube Channels:
- Back To Back SWE
- Tushar Roy – Coding Made Simple
- Gaurav Sen (for system design)
- Coding Environments:
- Repl.it
- CodePen
- Your preferred IDE
Utilize these resources to supplement your learning and practice.
12. Final Preparations Before the Interview
As your interview date approaches, focus on these final steps:
- Review your resume and be prepared to discuss any project or technology mentioned
- Prepare a short introduction about yourself (often the first question in interviews)
- Get familiar with the video conferencing tool if it’s a remote interview
- Set up your environment: ensure good lighting, a clean background, and a stable internet connection
- Have a pen and paper ready for note-taking
- Prepare questions to ask your interviewers about the role, team, and company
- Get a good night’s sleep and eat a healthy meal before the interview
Being well-prepared will help you feel more confident and perform better during the interview.
13. Post-Interview Steps
After the interview:
- Send a thank-you email to your interviewers within 24 hours
- Reflect on the interview experience and note areas for improvement
- Follow up with the recruiter if you haven’t heard back within the expected timeframe
- Regardless of the outcome, use the experience to refine your preparation for future interviews
Remember, each interview is a learning opportunity, whether you get the job or not.
Conclusion
Preparing for coding interviews, especially for top tech companies, requires dedication, consistent practice, and a structured approach. By following this comprehensive checklist, you’ll be well-equipped to tackle technical interviews with confidence. Remember that interview success is not just about technical knowledge, but also about clear communication, problem-solving skills, and the ability to perform under pressure.
As you work through this checklist, tailor your preparation to your strengths and weaknesses. Focus on steady progress rather than trying to master everything at once. With persistence and the right strategy, you’ll be well on your way to landing your dream job in software engineering.
Good luck with your interviews, and remember that each one is an opportunity to learn and grow as a developer!