What to Expect in a Coding Interview: A Day in the Life
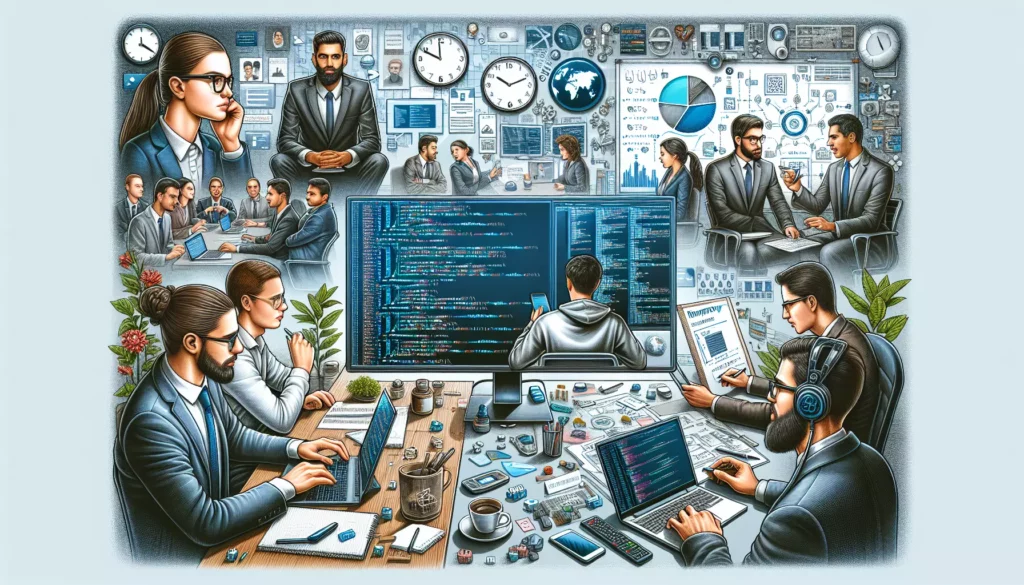
For many aspiring software engineers, the coding interview is a pivotal moment in their career journey. It’s the gateway to landing that dream job at a top tech company, often referred to as FAANG (Facebook/Meta, Amazon, Apple, Netflix, Google) or similar high-profile organizations. But what exactly happens during a coding interview? What should you expect, and how can you prepare? In this comprehensive guide, we’ll walk you through a typical day in the life of a coding interview candidate, providing insights, tips, and strategies to help you succeed.
The Night Before: Setting the Stage for Success
Your coding interview experience actually begins the night before. Here’s what you should do to ensure you’re in the best possible state of mind:
- Get a good night’s sleep: Aim for 7-8 hours of rest. A well-rested mind is crucial for problem-solving.
- Prepare your materials: Gather everything you’ll need, including a notepad, pen, and a bottle of water.
- Review your resume: Refresh your memory on the projects and experiences you’ve listed.
- Light review of key concepts: Skim through your notes on data structures and algorithms, but avoid cramming.
- Set multiple alarms: Ensure you wake up on time, leaving room for unexpected delays.
The Morning of: Getting in the Right Mindset
The day has arrived. Here’s how to start it right:
- Wake up early: Give yourself plenty of time to get ready without rushing.
- Eat a nutritious breakfast: Fuel your brain with a balanced meal.
- Dress appropriately: Even for virtual interviews, dressing professionally can boost your confidence.
- Do a quick tech check: Ensure your computer, internet connection, and any required software are working properly.
- Practice deep breathing or meditation: Take a few minutes to center yourself and calm your nerves.
Arriving at the Interview: First Impressions Matter
Whether you’re interviewing in-person or virtually, these tips apply:
- Arrive early: Aim to be 10-15 minutes early for in-person interviews, or log in 5 minutes early for virtual ones.
- Greet everyone politely: From the receptionist to your interviewer, be courteous to everyone you encounter.
- Have a confident posture: Stand or sit up straight, make eye contact, and offer a firm handshake (if in-person).
- Be prepared to small talk: Your interviewer might start with casual conversation to help you relax.
The Coding Interview Begins: What to Expect
While every company has its unique approach, most coding interviews follow a similar structure:
1. Introduction and Icebreaker (5-10 minutes)
The interviewer will likely introduce themselves and give you a brief overview of the interview process. They might ask about your background or interest in the company. This is your chance to make a good first impression and build rapport.
2. Technical Questions (10-15 minutes)
Before diving into coding, the interviewer might ask some technical questions to gauge your knowledge. These could include:
- Explaining key concepts like object-oriented programming, data structures, or algorithms
- Discussing the time and space complexity of different algorithms
- Describing your approach to solving a particular type of problem
3. The Coding Challenge (30-45 minutes)
This is the core of the interview. You’ll be presented with one or more coding problems to solve. Here’s what typically happens:
- Problem presentation: The interviewer will describe a problem, often related to data structures or algorithms.
- Clarification: You should ask questions to ensure you fully understand the problem and requirements.
- Brainstorming: Discuss your approach before coding. The interviewer wants to see your thought process.
- Coding: You’ll write code to solve the problem, either on a whiteboard, shared document, or coding platform.
- Testing: Run through test cases to verify your solution works correctly.
- Optimization: Discuss potential improvements or alternative approaches.
Example Coding Challenge
Here’s an example of a coding challenge you might encounter:
Problem: Given an array of integers, find two numbers such that they add up to a specific target number.
Function Signature:
def two_sum(nums: List[int], target: int) -> List[int]:
# Your code here
Example:
Input: nums = [2, 7, 11, 15], target = 9
Output: [0, 1] (because nums[0] + nums[1] = 2 + 7 = 9)
A possible solution might look like this:
def two_sum(nums: List[int], target: int) -> List[int]:
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return [] # No solution found
Remember, the interviewer is not just looking for the correct answer, but also evaluating your problem-solving approach, coding style, and ability to explain your thought process.
4. System Design Discussion (if applicable, 15-20 minutes)
For more senior positions or certain roles, you might be asked to discuss system design. This could involve:
- Designing a scalable architecture for a given application
- Discussing trade-offs between different design choices
- Explaining how you would handle specific challenges (e.g., load balancing, caching)
5. Behavioral Questions (10-15 minutes)
Towards the end of the interview, you might be asked behavioral questions to assess your soft skills and cultural fit. These could include:
- “Tell me about a time you faced a challenging technical problem. How did you solve it?”
- “How do you handle disagreements with team members?”
- “Describe a project you’re particularly proud of and why.”
6. Your Questions for the Interviewer (5-10 minutes)
At the end of the interview, you’ll usually have the opportunity to ask questions. This is your chance to learn more about the company and demonstrate your genuine interest. Some good questions to ask include:
- “What does a typical day look like for someone in this role?”
- “Can you tell me about the team I’d be working with?”
- “What are the biggest challenges facing the department/company right now?”
- “How does the company support professional development and learning?”
After the Interview: Next Steps
Once the interview is over, your day isn’t quite done:
- Thank the interviewer: Express your appreciation for their time and reaffirm your interest in the position.
- Send a follow-up email: Within 24 hours, send a brief thank-you email, reiterating your interest and perhaps mentioning a specific point from the interview.
- Reflect on the experience: Take notes on what went well and areas for improvement.
- Follow up if necessary: If you don’t hear back within the timeframe they specified, it’s okay to send a polite inquiry about the status of your application.
Common Challenges and How to Handle Them
Even with thorough preparation, you might encounter some challenges during your coding interview. Here’s how to handle common situations:
1. Getting Stuck on a Problem
If you find yourself stuck, don’t panic. Here’s what to do:
- Think out loud: Verbalize your thought process. The interviewer might provide hints or guidance.
- Break the problem down: Try to solve a simpler version of the problem first.
- Review the constraints: Sometimes, revisiting the problem statement can provide new insights.
- Ask for help: It’s okay to ask for a hint if you’re truly stuck. This shows you can collaborate and seek assistance when needed.
2. Nervousness or Anxiety
It’s natural to feel nervous, but excessive anxiety can hinder your performance. Try these techniques:
- Deep breathing: Take slow, deep breaths to calm your nerves.
- Positive self-talk: Remind yourself of your preparation and past successes.
- Focus on the problem: Immersing yourself in the task at hand can help reduce anxiety.
3. Technical Issues (for Virtual Interviews)
If you encounter technical problems during a virtual interview:
- Stay calm: Technical issues are often beyond your control. How you handle them can demonstrate your problem-solving skills.
- Have a backup plan: Be prepared with alternative contact methods (phone number, alternate video platform).
- Communicate clearly: If you’re experiencing issues, let the interviewer know immediately.
4. Time Management
Managing your time effectively during the interview is crucial:
- Ask about time constraints: Clarify how much time you have for each section of the interview.
- Prioritize: Focus on the most critical parts of the problem first.
- Keep an eye on the clock: Pace yourself, but don’t let time pressure overwhelm you.
Tips for Success: Mastering the Coding Interview
To increase your chances of success in a coding interview, keep these tips in mind:
1. Practice, Practice, Practice
Regular practice is key to performing well in coding interviews. Here’s how to make the most of your preparation:
- Use platforms like AlgoCademy: Take advantage of interactive coding tutorials and AI-powered assistance to sharpen your skills.
- Solve diverse problems: Practice a wide range of problem types to broaden your problem-solving toolkit.
- Time yourself: Get comfortable solving problems under time constraints.
- Mock interviews: Practice with friends or use online platforms that simulate real interview conditions.
2. Communicate Effectively
Your ability to communicate your thoughts clearly is just as important as your coding skills:
- Think out loud: Explain your thought process as you work through the problem.
- Ask clarifying questions: Ensure you fully understand the problem before diving into a solution.
- Explain your decisions: Justify why you chose a particular approach or data structure.
3. Know Your Fundamentals
A solid grasp of computer science fundamentals is crucial:
- Data Structures: Be comfortable with arrays, linked lists, stacks, queues, trees, graphs, and hash tables.
- Algorithms: Understand common algorithms for searching, sorting, and graph traversal.
- Time and Space Complexity: Be able to analyze the efficiency of your solutions.
- Object-Oriented Programming: Understand key OOP concepts like encapsulation, inheritance, and polymorphism.
4. Write Clean, Readable Code
Even under pressure, strive to write code that’s clean and easy to understand:
- Use meaningful variable names: Choose names that clearly indicate the purpose of each variable.
- Keep your code modular: Break down complex problems into smaller, manageable functions.
- Comment your code: Add brief comments to explain complex logic or non-obvious decisions.
- Follow consistent formatting: Use proper indentation and maintain a consistent coding style.
5. Be Prepared to Optimize
Often, interviewers will ask you to improve your initial solution:
- Start with a working solution: It’s better to have a less efficient solution that works than no solution at all.
- Analyze your solution: Be prepared to discuss the time and space complexity of your code.
- Consider edge cases: Think about how your solution handles extreme or unusual inputs.
- Be open to feedback: If the interviewer suggests an optimization, be receptive and try to incorporate their ideas.
Conclusion: Embracing the Challenge
A coding interview can be a daunting experience, but it’s also an opportunity to showcase your skills, learn, and potentially launch an exciting new chapter in your career. By understanding what to expect, preparing thoroughly, and approaching the interview with the right mindset, you can turn this challenge into a stepping stone towards your goals.
Remember, the purpose of a coding interview is not just to test your technical skills, but also to evaluate how you approach problems, communicate your thoughts, and collaborate with others. These are all crucial skills for a successful career in software development.
As you prepare for your coding interviews, leverage resources like AlgoCademy to hone your skills, practice regularly, and build your confidence. With dedication and the right approach, you can navigate the coding interview process successfully and open doors to exciting opportunities in the tech industry.
Good luck with your coding interviews, and remember: every interview, regardless of the outcome, is a valuable learning experience that brings you one step closer to your dream job in tech!