The Art of Problem Solving in Coding Interviews: Mastering Algorithmic Thinking
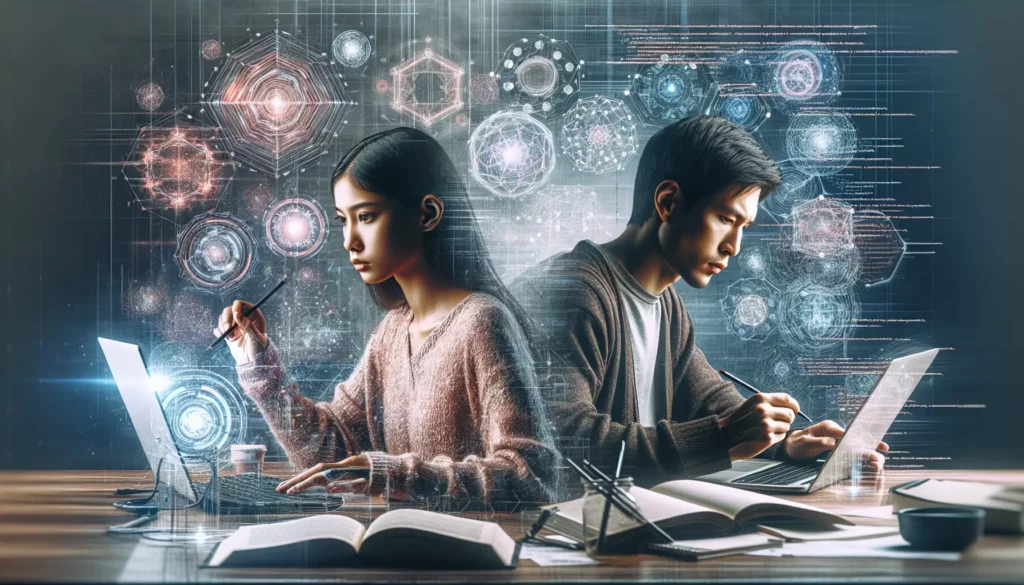
In the competitive landscape of tech recruitment, coding interviews have become a crucial gateway for aspiring developers seeking positions at top-tier companies. These interviews, particularly those conducted by FAANG (Facebook, Amazon, Apple, Netflix, Google) and other tech giants, are designed to assess not just a candidate’s coding prowess but their problem-solving abilities and algorithmic thinking. This article delves deep into the art of problem-solving in coding interviews, providing you with strategies, insights, and practical tips to excel in these high-stakes situations.
Understanding the Nature of Coding Interviews
Before we dive into problem-solving techniques, it’s essential to understand what coding interviews entail and why they’re structured the way they are.
The Purpose of Coding Interviews
Coding interviews serve multiple purposes:
- Assess technical skills and coding ability
- Evaluate problem-solving and analytical thinking
- Gauge communication skills and ability to work under pressure
- Determine cultural fit and potential for growth within the company
Common Interview Formats
Coding interviews can take various forms:
- Whiteboard Coding: Solving problems on a whiteboard, focusing on high-level problem-solving rather than syntactical correctness.
- Online Coding Platforms: Solving problems in real-time using online IDEs like HackerRank or LeetCode.
- Take-home Assignments: Completing a coding project within a given timeframe, often used to assess real-world coding skills.
- Pair Programming: Collaborating with an interviewer to solve a problem, demonstrating teamwork and communication skills.
The Problem-Solving Framework
Regardless of the interview format, a structured approach to problem-solving can significantly improve your performance. Here’s a framework you can follow:
1. Understand the Problem
The first step is to ensure you have a clear understanding of the problem at hand. This involves:
- Carefully reading the problem statement
- Identifying the input and expected output
- Clarifying any ambiguities with the interviewer
- Considering edge cases and constraints
For example, if given a problem to find the longest palindromic substring in a string, you might ask:
- Should the solution be case-sensitive?
- Are we considering only alphanumeric characters?
- What should be returned if there are multiple palindromes of the same length?
2. Plan Your Approach
Once you understand the problem, it’s time to devise a strategy. This step involves:
- Brainstorming potential solutions
- Considering different algorithms and data structures
- Evaluating the time and space complexity of each approach
- Choosing the most efficient solution given the constraints
Let’s say you’re solving the “Two Sum” problem. You might consider:
- A brute force approach using nested loops (O(n^2) time complexity)
- Sorting the array and using two pointers (O(n log n) time complexity)
- Using a hash map for constant-time lookups (O(n) time complexity)
3. Implement the Solution
With a plan in place, start implementing your solution:
- Write clean, readable code
- Use meaningful variable names
- Break down the problem into smaller functions if necessary
- Comment your code to explain your thought process
Here’s an example implementation of the Two Sum problem using a hash map approach:
def two_sum(nums, target):
num_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_map:
return [num_map[complement], i]
num_map[num] = i
return [] # No solution found
4. Test Your Solution
After implementation, it’s crucial to test your solution:
- Start with simple test cases
- Move on to edge cases (empty input, large numbers, etc.)
- Verify the correctness of your output
- Analyze the time and space complexity of your implementation
For the Two Sum problem, you might test:
# Test cases
print(two_sum([2, 7, 11, 15], 9)) # Expected: [0, 1]
print(two_sum([3, 2, 4], 6)) # Expected: [1, 2]
print(two_sum([3, 3], 6)) # Expected: [0, 1]
print(two_sum([1, 2, 3, 4], 10)) # Expected: []
5. Optimize and Refine
If time allows, look for ways to optimize your solution:
- Can you reduce the time or space complexity?
- Are there any redundant operations you can eliminate?
- Can you make the code more readable or efficient?
In our Two Sum example, the hash map approach is already optimal in terms of time complexity. However, you might discuss potential trade-offs between time and space complexity with the interviewer.
Essential Problem-Solving Techniques
To excel in coding interviews, it’s crucial to be familiar with a range of problem-solving techniques. Here are some key approaches:
1. Divide and Conquer
This technique involves breaking down a complex problem into smaller, more manageable subproblems. It’s particularly useful for problems involving sorting, searching, and optimization.
Example: Merge Sort algorithm
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
2. Dynamic Programming
Dynamic Programming (DP) is a method for solving complex problems by breaking them down into simpler subproblems. It’s especially useful for optimization problems and problems with overlapping subproblems.
Example: Fibonacci sequence using DP
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
3. Greedy Algorithms
Greedy algorithms make locally optimal choices at each step with the hope of finding a global optimum. They’re often used for optimization problems but don’t always guarantee the best solution.
Example: Coin change problem (assuming coins of denominations 1, 5, 10, 25)
def coin_change(amount):
coins = [25, 10, 5, 1]
result = []
for coin in coins:
while amount >= coin:
result.append(coin)
amount -= coin
return result
4. Sliding Window
The sliding window technique is used to perform operations on a specific window size of an array or string. It’s particularly useful for problems involving subarrays or substrings.
Example: Finding the maximum sum subarray of size k
def max_subarray_sum(arr, k):
if len(arr) < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(len(arr) - k):
window_sum = window_sum - arr[i] + arr[i + k]
max_sum = max(max_sum, window_sum)
return max_sum
5. Two Pointers
The two pointers technique uses two pointers to iterate through the data structure in a single pass. It’s often used for problems involving arrays or linked lists.
Example: Reversing a string in-place
def reverse_string(s):
left, right = 0, len(s) - 1
while left < right:
s[left], s[right] = s[right], s[left]
left += 1
right -= 1
return s
Common Pitfalls and How to Avoid Them
Even with a solid problem-solving framework and knowledge of various techniques, there are common pitfalls that candidates often fall into during coding interviews. Being aware of these can help you navigate the interview process more successfully.
1. Rushing to Code
Pitfall: Jumping straight into coding without fully understanding the problem or planning your approach.
How to Avoid:
- Take time to thoroughly understand the problem
- Discuss your approach with the interviewer before coding
- Use pseudocode to outline your solution
2. Overlooking Edge Cases
Pitfall: Failing to consider and handle edge cases, leading to incomplete or incorrect solutions.
How to Avoid:
- Explicitly ask about and consider edge cases (empty input, negative numbers, etc.)
- Include edge case handling in your initial implementation
- Test your solution with edge cases before declaring it complete
3. Poor Time Management
Pitfall: Spending too much time on one part of the problem, leaving insufficient time for implementation or optimization.
How to Avoid:
- Set mental time limits for each phase of problem-solving
- Communicate with the interviewer about your time allocation
- Be prepared to move on with a suboptimal solution if time is running out
4. Neglecting Communication
Pitfall: Focusing solely on coding without explaining your thought process to the interviewer.
How to Avoid:
- Think out loud as you work through the problem
- Explain your reasoning for choosing certain approaches
- Ask for feedback and clarification when needed
5. Ignoring Space Complexity
Pitfall: Focusing only on time complexity and neglecting space complexity in your solutions.
How to Avoid:
- Consider both time and space complexity when devising solutions
- Discuss trade-offs between time and space with the interviewer
- Be prepared to optimize for space if required
Advanced Problem-Solving Strategies
As you progress in your interview preparation, it’s beneficial to familiarize yourself with more advanced problem-solving strategies. These can give you an edge when tackling complex problems.
1. Recursion and Backtracking
Recursion is a powerful technique where a function calls itself to solve a problem. Backtracking is an algorithmic technique that considers all possible configurations and removes those that fail to satisfy the constraints.
Example: Generating all permutations of a string
def generate_permutations(s):
def backtrack(start):
if start == len(s):
result.append(''.join(s))
for i in range(start, len(s)):
s[start], s[i] = s[i], s[start]
backtrack(start + 1)
s[start], s[i] = s[i], s[start] # backtrack
result = []
backtrack(0)
return result
# Usage
print(generate_permutations(list("abc")))
2. Graph Algorithms
Understanding graph algorithms is crucial for many complex problems. Key algorithms include Depth-First Search (DFS), Breadth-First Search (BFS), Dijkstra’s algorithm, and Union-Find.
Example: Implementing DFS for a graph
from collections import defaultdict
class Graph:
def __init__(self):
self.graph = defaultdict(list)
def add_edge(self, u, v):
self.graph[u].append(v)
def dfs_util(self, v, visited):
visited.add(v)
print(v, end=' ')
for neighbor in self.graph[v]:
if neighbor not in visited:
self.dfs_util(neighbor, visited)
def dfs(self, v):
visited = set()
self.dfs_util(v, visited)
# Usage
g = Graph()
g.add_edge(0, 1)
g.add_edge(0, 2)
g.add_edge(1, 2)
g.add_edge(2, 0)
g.add_edge(2, 3)
g.add_edge(3, 3)
print("DFS starting from vertex 2:")
g.dfs(2)
3. Bit Manipulation
Bit manipulation techniques can lead to highly efficient solutions for certain problems, particularly those involving binary operations or optimizing space usage.
Example: Checking if a number is a power of 2
def is_power_of_two(n):
return n > 0 and (n & (n - 1)) == 0
# Usage
print(is_power_of_two(16)) # True
print(is_power_of_two(18)) # False
4. Mathematical Approaches
Some problems can be solved more efficiently using mathematical principles rather than purely algorithmic approaches.
Example: Finding the nth Fibonacci number using matrix exponentiation
def matrix_multiply(a, b):
return [
[a[0][0]*b[0][0] + a[0][1]*b[1][0], a[0][0]*b[0][1] + a[0][1]*b[1][1]],
[a[1][0]*b[0][0] + a[1][1]*b[1][0], a[1][0]*b[0][1] + a[1][1]*b[1][1]]
]
def matrix_power(matrix, n):
if n == 1:
return matrix
if n % 2 == 0:
half = matrix_power(matrix, n // 2)
return matrix_multiply(half, half)
return matrix_multiply(matrix, matrix_power(matrix, n - 1))
def fibonacci(n):
if n <= 1:
return n
base = [[1, 1], [1, 0]]
result = matrix_power(base, n - 1)
return result[0][0]
# Usage
print(fibonacci(100)) # Efficiently calculates the 100th Fibonacci number
Preparing for Coding Interviews
Now that we’ve covered various problem-solving techniques and strategies, let’s discuss how to effectively prepare for coding interviews.
1. Practice Regularly
Consistent practice is key to improving your problem-solving skills:
- Solve problems on platforms like LeetCode, HackerRank, or AlgoCademy
- Aim to solve at least one problem daily
- Focus on understanding and implementing different algorithms and data structures
2. Study Core Computer Science Concepts
Ensure you have a solid understanding of fundamental CS concepts:
- Data Structures (Arrays, Linked Lists, Trees, Graphs, Hash Tables, etc.)
- Algorithms (Sorting, Searching, Graph algorithms, Dynamic Programming, etc.)
- Time and Space Complexity Analysis
- Object-Oriented Programming principles
3. Mock Interviews
Simulate real interview conditions to improve your performance:
- Practice with a friend or use online platforms that offer mock interviews
- Time yourself to get used to solving problems under pressure
- Practice explaining your thought process out loud
4. Review and Reflect
After solving problems or completing mock interviews:
- Review your solutions and compare them with other efficient approaches
- Reflect on areas where you struggled and focus on improving those skills
- Keep a log of problems you’ve solved and revisit them periodically
5. Stay Updated
The tech industry is constantly evolving:
- Keep up with new programming languages and frameworks
- Stay informed about industry trends and new technologies
- Participate in coding competitions or hackathons to challenge yourself
Conclusion
Mastering the art of problem-solving in coding interviews is a journey that requires dedication, practice, and a structured approach. By understanding the nature of coding interviews, adopting a solid problem-solving framework, and familiarizing yourself with various techniques and strategies, you can significantly improve your performance and confidence.
Remember that the goal of these interviews is not just to arrive at a correct solution, but to demonstrate your thought process, problem-solving skills, and ability to communicate effectively. Even if you don’t immediately solve a problem, showing a logical approach and the ability to learn and adapt can leave a positive impression on interviewers.
As you prepare, leverage resources like AlgoCademy that provide interactive coding tutorials, AI-powered assistance, and step-by-step guidance. These tools can help you progress from beginner-level coding to confidently tackling technical interviews at major tech companies.
With consistent practice and the right mindset, you can develop the skills and confidence needed to excel in coding interviews and take a significant step towards your dream role in the tech industry. Good luck with your interview preparation!