The Importance of Big O Notation in Interviews: Mastering Algorithmic Efficiency
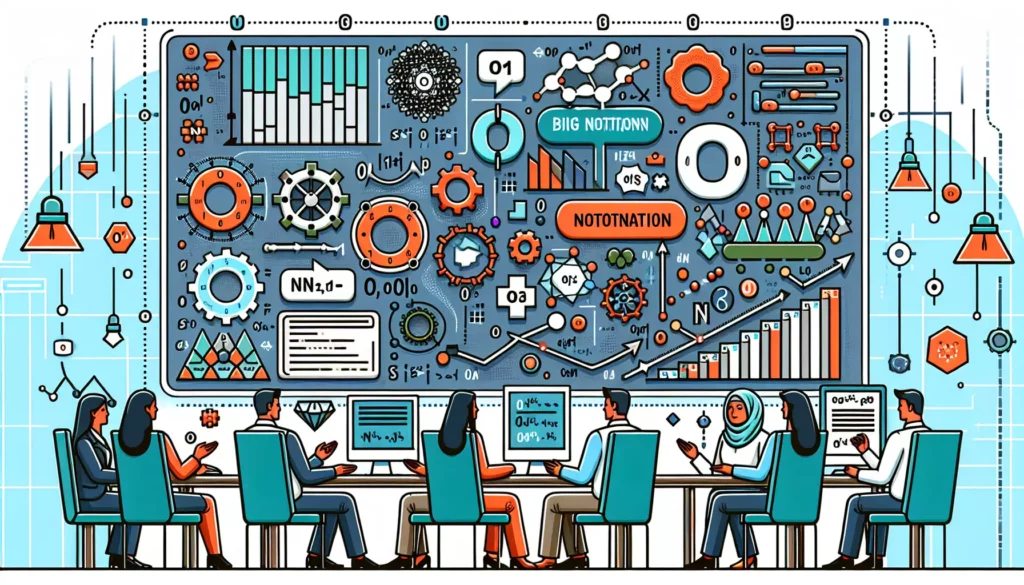
In the competitive landscape of tech interviews, particularly those at major companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), understanding and effectively applying Big O notation is not just beneficial—it’s essential. As a cornerstone of algorithmic analysis, Big O notation provides a standardized way to describe the efficiency of algorithms, making it a crucial topic for any aspiring software engineer. In this comprehensive guide, we’ll delve deep into the world of Big O notation, exploring its significance in technical interviews and providing you with the knowledge and strategies to ace this critical aspect of your coding assessment.
What is Big O Notation?
Before we dive into its importance in interviews, let’s establish a clear understanding of what Big O notation actually is. Big O notation is a mathematical notation used in computer science to describe the performance or complexity of an algorithm. Specifically, it describes the worst-case scenario, or the maximum time an algorithm will take to complete.
The “O” in Big O notation stands for “Order of,” which refers to the order of magnitude of the algorithm’s run time. It provides an upper bound of the complexity in the worst case, helping to quantify performance as the input size becomes arbitrarily large.
Common Big O Notations
Here are some of the most common Big O notations, listed in order of increasing complexity:
- O(1) – Constant time
- O(log n) – Logarithmic time
- O(n) – Linear time
- O(n log n) – Linearithmic time
- O(n^2) – Quadratic time
- O(2^n) – Exponential time
- O(n!) – Factorial time
Why is Big O Notation Important in Interviews?
Understanding Big O notation is crucial in technical interviews for several reasons:
1. Demonstrates Analytical Thinking
By discussing the time and space complexity of your solutions using Big O notation, you demonstrate to interviewers that you can think analytically about your code. This skill is highly valued in software engineering roles, where optimizing for efficiency can have significant impacts on system performance and scalability.
2. Shows Understanding of Efficiency
Big O notation allows you to communicate the efficiency of your algorithms clearly and concisely. This is particularly important when comparing different solutions to a problem, as it provides a standardized way to evaluate trade-offs between time and space complexity.
3. Indicates Problem-Solving Skills
Being able to analyze and improve the efficiency of your algorithms shows that you have strong problem-solving skills. Interviewers are often more interested in your thought process and ability to optimize solutions than in your ability to simply produce working code.
4. Reflects Industry Standards
Big O notation is widely used in the tech industry to discuss algorithm performance. Familiarity with this concept shows that you’re in tune with industry standards and practices.
5. Prepares for Real-World Scenarios
In real-world software development, especially at large tech companies dealing with massive amounts of data, understanding algorithmic efficiency is crucial. Your ability to analyze and optimize algorithms can directly impact the performance and scalability of the systems you’ll work on.
How to Apply Big O Notation in Interviews
Now that we understand why Big O notation is important, let’s explore how to effectively apply it during technical interviews:
1. Analyze Your Solution
After you’ve written your solution to a problem, take a moment to analyze its time and space complexity. Consider the following:
- How many times does your code iterate through the input?
- Are there any nested loops?
- How much additional memory does your solution use?
2. Explain Your Reasoning
When you provide the Big O notation for your solution, explain your reasoning. For example:
“This solution has a time complexity of O(n^2) because there are two nested loops that each iterate through the entire input array of size n. The space complexity is O(1) because we’re only using a constant amount of extra space regardless of the input size.”
3. Consider Edge Cases
Remember that Big O notation represents the worst-case scenario. Consider edge cases that might lead to this worst-case performance and be prepared to discuss them.
4. Discuss Trade-offs
If there are multiple ways to solve the problem, discuss the time and space complexity trade-offs between different approaches. This shows that you can think critically about algorithmic design.
5. Look for Optimization Opportunities
After analyzing your initial solution, consider if there are ways to optimize it. Can you reduce the time complexity by using a different data structure? Can you use techniques like memoization or dynamic programming to improve efficiency?
Common Big O Notations and Their Characteristics
Let’s dive deeper into some of the most common Big O notations you’re likely to encounter in interviews:
O(1) – Constant Time
An algorithm with O(1) complexity performs a constant number of operations, regardless of the input size. These are typically the most efficient algorithms.
Example: Accessing an array element by index.
function getElement(arr, index) {
return arr[index];
}
O(log n) – Logarithmic Time
Logarithmic time complexity is characteristic of algorithms that divide the problem in half at each step. These are very efficient, especially for large inputs.
Example: Binary search on a sorted array.
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
O(n) – Linear Time
Linear time algorithms have a runtime directly proportional to the input size. They typically involve iterating through the input once.
Example: Finding the maximum element in an unsorted array.
function findMax(arr) {
let max = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max) max = arr[i];
}
return max;
}
O(n log n) – Linearithmic Time
This complexity is often seen in efficient sorting algorithms. It’s more efficient than quadratic time but less efficient than linear time.
Example: Merge sort algorithm.
function mergeSort(arr) {
if (arr.length <= 1) return arr;
const mid = Math.floor(arr.length / 2);
const left = arr.slice(0, mid);
const right = arr.slice(mid);
return merge(mergeSort(left), mergeSort(right));
}
function merge(left, right) {
let result = [];
let leftIndex = 0;
let rightIndex = 0;
while (leftIndex < left.length && rightIndex < right.length) {
if (left[leftIndex] < right[rightIndex]) {
result.push(left[leftIndex]);
leftIndex++;
} else {
result.push(right[rightIndex]);
rightIndex++;
}
}
return result.concat(left.slice(leftIndex)).concat(right.slice(rightIndex));
}
O(n^2) – Quadratic Time
Quadratic time algorithms have a runtime that’s proportional to the square of the input size. These are often less efficient and may need optimization for large inputs.
Example: Bubble sort algorithm.
function bubbleSort(arr) {
const n = arr.length;
for (let i = 0; i < n; i++) {
for (let j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// Swap elements
[arr[j], arr[j + 1]] = [arr[j + 1], arr[j]];
}
}
}
return arr;
}
Common Pitfalls and How to Avoid Them
When discussing Big O notation in interviews, be aware of these common pitfalls:
1. Focusing Only on Time Complexity
While time complexity is crucial, don’t forget about space complexity. Sometimes, an algorithm might be time-efficient but memory-intensive, which could be problematic in certain scenarios.
2. Overlooking Hidden Loops
Be careful of hidden loops in your code. For example, certain string or array operations in some programming languages might involve iterating over elements behind the scenes.
3. Misunderstanding Nested Loops
Not all nested loops result in O(n^2) complexity. If the inner loop doesn’t always iterate n times, the complexity might be different.
4. Ignoring Constants and Lower Order Terms
In Big O notation, we typically ignore constants and lower order terms. For example, O(2n) is simplified to O(n), and O(n^2 + n) is simplified to O(n^2).
5. Assuming Best-Case Scenarios
Remember that Big O notation typically represents the worst-case scenario. Don’t base your analysis on the best-case or average-case performance unless specifically asked.
Strategies for Improving Algorithm Efficiency
When faced with an inefficient algorithm during an interview, consider these strategies for improvement:
1. Use Appropriate Data Structures
Choosing the right data structure can significantly impact your algorithm’s efficiency. For example, using a hash table for quick lookups instead of repeatedly searching through an array.
2. Avoid Unnecessary Work
Look for opportunities to reduce redundant computations. This might involve caching results or breaking out of loops early when a condition is met.
3. Divide and Conquer
Consider if the problem can be broken down into smaller subproblems. Techniques like recursion or dynamic programming often lead to more efficient solutions.
4. Space-Time Trade-offs
Sometimes, you can improve time complexity by using more memory, or vice versa. Be prepared to discuss these trade-offs.
5. Preprocessing
If the same operation will be performed multiple times, consider if you can preprocess the data to make subsequent operations more efficient.
Practice Problems
To solidify your understanding of Big O notation and its application in interviews, try analyzing the time and space complexity of solutions to these practice problems:
1. Two Sum
Given an array of integers and a target sum, return indices of two numbers such that they add up to the target.
function twoSum(nums, target) {
const map = new Map();
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (map.has(complement)) {
return [map.get(complement), i];
}
map.set(nums[i], i);
}
return [];
}
2. Reverse a Linked List
Reverse a singly linked list.
function reverseList(head) {
let prev = null;
let current = head;
while (current !== null) {
let nextTemp = current.next;
current.next = prev;
prev = current;
current = nextTemp;
}
return prev;
}
3. Valid Palindrome
Determine if a string is a palindrome, considering only alphanumeric characters and ignoring cases.
function isPalindrome(s) {
s = s.toLowerCase().replace(/[^a-z0-9]/g, '');
let left = 0;
let right = s.length - 1;
while (left < right) {
if (s[left] !== s[right]) return false;
left++;
right--;
}
return true;
}
Conclusion
Mastering Big O notation is a crucial step in your journey to becoming a proficient software engineer and acing technical interviews. It provides a standardized language for discussing algorithm efficiency, demonstrates your analytical thinking skills, and prepares you for real-world scenarios where performance optimization is key.
Remember, the goal isn’t just to memorize Big O notations, but to understand the underlying principles and apply them effectively. As you practice coding problems, make it a habit to analyze the time and space complexity of your solutions. This will not only prepare you for interviews but also make you a more thoughtful and efficient programmer.
Keep in mind that while Big O notation is important, it’s just one aspect of what interviewers are looking for. They also value clean, readable code, good problem-solving skills, and the ability to communicate your thoughts clearly. Combine your understanding of algorithmic efficiency with these other crucial skills, and you’ll be well-prepared to tackle even the most challenging technical interviews.
Happy coding, and best of luck in your interviews!