The 5 Books Every Programmer Should Read: Essential Reads for Coding Mastery
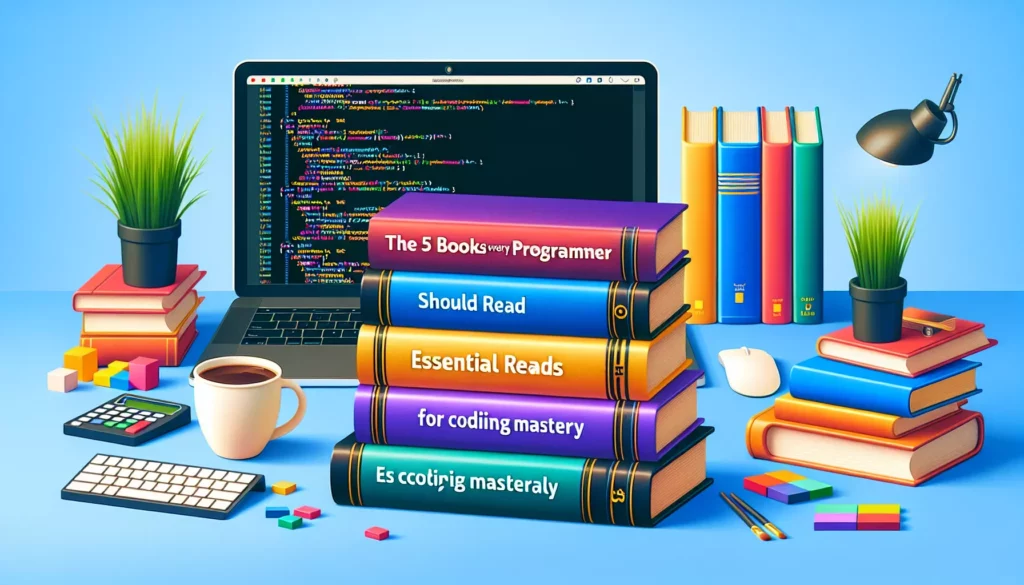
In the ever-evolving world of programming, staying ahead of the curve is crucial. Whether you’re a beginner just starting your coding journey or an experienced developer looking to sharpen your skills, the right books can be invaluable resources. At AlgoCademy, we believe that a well-rounded education in programming goes beyond just writing code. It involves understanding core concepts, best practices, and the philosophy behind great software development.
In this comprehensive guide, we’ll explore the five books that every programmer should read. These books have stood the test of time, offering insights that remain relevant regardless of the latest programming language or framework. They cover a range of topics from algorithmic thinking to clean code practices, and from system design to the human elements of programming.
Let’s dive into these must-read books that will not only improve your coding skills but also change the way you think about programming.
1. “Clean Code: A Handbook of Agile Software Craftsmanship” by Robert C. Martin
“Clean Code” is often considered the bible of writing maintainable, readable, and efficient code. Robert C. Martin, also known as Uncle Bob, presents a comprehensive guide to writing code that is not just functional, but also clean and professional.
Why It’s Essential:
- Code Quality: The book emphasizes the importance of writing code that is easy to read, understand, and maintain. This is crucial in real-world scenarios where multiple developers work on the same codebase.
- Best Practices: It introduces numerous best practices for naming conventions, function design, and code organization that can significantly improve code quality.
- Refactoring Techniques: The book provides practical examples of how to transform “bad” code into “clean” code, offering valuable refactoring techniques.
- Professional Development: It instills a sense of professionalism and craftsmanship in coding, encouraging developers to take pride in their work.
Key Takeaways:
- Meaningful names for variables, functions, and classes are crucial for code readability.
- Functions should be small, do one thing, and operate at a single level of abstraction.
- Comments should be used sparingly and only when necessary to explain intent that cannot be expressed in code.
- Code should be formatted consistently to enhance readability and maintainability.
- Unit tests are an essential part of clean code and should be written with the same care as production code.
Here’s a simple example of how applying clean code principles can improve code quality:
// Before: Unclear and hard to maintain
function calc(a, b, c) {
let result;
if (c == 1) {
result = a + b;
} else if (c == 2) {
result = a - b;
} else {
result = a * b;
}
return result;
}
// After: Clean and easy to understand
function performOperation(firstNumber, secondNumber, operationType) {
switch (operationType) {
case "add":
return firstNumber + secondNumber;
case "subtract":
return firstNumber - secondNumber;
case "multiply":
return firstNumber * secondNumber;
default:
throw new Error("Invalid operation type");
}
}
“Clean Code” is not just about following a set of rules; it’s about adopting a mindset that values clarity, simplicity, and maintainability in code. This book will change the way you approach writing code and help you become a better programmer.
2. “Introduction to Algorithms” by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein
Often referred to as CLRS (after the authors’ last names), “Introduction to Algorithms” is the definitive guide to algorithms and data structures. This comprehensive textbook is widely used in computer science courses and is an invaluable resource for anyone serious about programming.
Why It’s Essential:
- Foundational Knowledge: It provides a deep understanding of fundamental algorithms and data structures, which are the building blocks of efficient software.
- Problem-Solving Skills: The book teaches you how to think algorithmically, improving your ability to solve complex programming problems.
- Performance Analysis: It introduces techniques for analyzing the efficiency of algorithms, crucial for optimizing code performance.
- Breadth of Coverage: From sorting and searching to advanced topics like dynamic programming and graph algorithms, it covers a wide range of algorithmic concepts.
Key Takeaways:
- Understanding time and space complexity is crucial for writing efficient code.
- Different algorithms can solve the same problem with vastly different efficiencies.
- Mastering fundamental data structures (arrays, linked lists, trees, graphs) is essential for effective problem-solving.
- Algorithmic paradigms like divide-and-conquer, dynamic programming, and greedy algorithms are powerful tools for tackling complex problems.
- The ability to analyze and compare algorithms is as important as implementing them.
Here’s a simple example of how understanding algorithms can lead to more efficient code:
// Inefficient approach: O(n^2) time complexity
function findDuplicates(arr) {
let duplicates = [];
for (let i = 0; i < arr.length; i++) {
for (let j = i + 1; j < arr.length; j++) {
if (arr[i] === arr[j] && !duplicates.includes(arr[i])) {
duplicates.push(arr[i]);
}
}
}
return duplicates;
}
// Efficient approach: O(n) time complexity
function findDuplicatesEfficient(arr) {
let seen = new Set();
let duplicates = new Set();
for (let num of arr) {
if (seen.has(num)) {
duplicates.add(num);
} else {
seen.add(num);
}
}
return Array.from(duplicates);
}
While “Introduction to Algorithms” can be mathematically intense at times, it’s an invaluable resource for any programmer looking to deepen their understanding of algorithms and improve their problem-solving skills. The concepts learned from this book will help you write more efficient code and tackle complex programming challenges with confidence.
3. “Design Patterns: Elements of Reusable Object-Oriented Software” by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides
Often referred to as the “Gang of Four” book, “Design Patterns” is a classic text that introduces 23 patterns used to design flexible and maintainable object-oriented software. Although it was published in 1994, its principles remain highly relevant in modern software development.
Why It’s Essential:
- Reusable Solutions: It provides proven solutions to common problems in software design, saving time and reducing errors in development.
- Improved Code Structure: Understanding design patterns helps in creating more organized and scalable code architectures.
- Common Vocabulary: It establishes a shared language among developers, making it easier to communicate complex design ideas.
- Flexibility and Maintainability: The patterns promote loose coupling and high cohesion, leading to more flexible and maintainable software systems.
Key Takeaways:
- Design patterns are not code templates but rather guidelines for solving common design problems.
- Understanding when to apply (and when not to apply) design patterns is as important as knowing the patterns themselves.
- Patterns are categorized into creational, structural, and behavioral patterns, each addressing different aspects of software design.
- Many modern frameworks and libraries incorporate these design patterns, making their understanding crucial for effective use of these tools.
- Design patterns evolve over time, and new patterns emerge as software development practices change.
Here’s a simple example of the Observer pattern, one of the behavioral patterns described in the book:
class Subject {
constructor() {
this.observers = [];
this.state = null;
}
attach(observer) {
this.observers.push(observer);
}
setState(state) {
this.state = state;
this.notifyAllObservers();
}
notifyAllObservers() {
for (let observer of this.observers) {
observer.update(this.state);
}
}
}
class Observer {
update(state) {
// To be implemented by concrete observer classes
}
}
class ConcreteObserver extends Observer {
constructor(name) {
super();
this.name = name;
}
update(state) {
console.log(`${this.name} received update. New state: ${state}`);
}
}
// Usage
let subject = new Subject();
let observer1 = new ConcreteObserver("Observer 1");
let observer2 = new ConcreteObserver("Observer 2");
subject.attach(observer1);
subject.attach(observer2);
subject.setState("New State");
While some argue that certain patterns in the book have become less relevant with modern programming languages and paradigms, the underlying principles of good design that the book teaches remain invaluable. Understanding these patterns will help you recognize common design structures, communicate more effectively with other developers, and create more robust and maintainable software systems.
4. “The Pragmatic Programmer: Your Journey to Mastery” by Andrew Hunt and David Thomas
“The Pragmatic Programmer” is a timeless guide that goes beyond just coding techniques. It offers practical advice on how to approach software development as a craft, emphasizing the importance of critical thinking, continuous learning, and adaptability in the ever-changing world of technology.
Why It’s Essential:
- Holistic Approach: It covers not just coding, but also project management, team dynamics, and personal development aspects of programming.
- Practical Wisdom: The book offers actionable tips and techniques that can be immediately applied to improve one’s programming practices.
- Career Development: It provides insights on how to grow as a professional in the software development field.
- Problem-Solving Mindset: The book encourages a pragmatic approach to problem-solving, emphasizing practicality and efficiency.
Key Takeaways:
- DRY (Don’t Repeat Yourself) principle: Avoid duplication in code, documentation, and processes.
- Orthogonality: Design components that are independent and have a single, well-defined purpose.
- Tracer Bullets: Implement a basic end-to-end functionality early to get quick feedback and guide development.
- Rubber Duck Debugging: Explain your code to an inanimate object (like a rubber duck) to help find bugs and clarify thinking.
- Continuous Learning: Stay current with technology trends and always be learning new tools and techniques.
Here’s an example of applying the DRY principle:
// Before: Violating DRY principle
function calculateAreaSquare(side) {
return side * side;
}
function calculateAreaRectangle(length, width) {
return length * width;
}
function calculateAreaCircle(radius) {
return Math.PI * radius * radius;
}
// After: Applying DRY principle
function calculateArea(shape, ...dimensions) {
switch(shape) {
case "square":
case "rectangle":
return dimensions.reduce((acc, val) => acc * val, 1);
case "circle":
return Math.PI * Math.pow(dimensions[0], 2);
default:
throw new Error("Unsupported shape");
}
}
// Usage
console.log(calculateArea("square", 5));
console.log(calculateArea("rectangle", 4, 6));
console.log(calculateArea("circle", 3));
“The Pragmatic Programmer” is not just about writing better code; it’s about becoming a better programmer. The book’s emphasis on critical thinking, problem-solving, and continuous improvement makes it an essential read for programmers at all levels of experience.
5. “Code Complete: A Practical Handbook of Software Construction” by Steve McConnell
“Code Complete” is a comprehensive guide to software construction that covers everything from planning and design to testing and debugging. It’s often considered one of the most practical and readable books on software development, offering a wealth of best practices and guidelines for writing high-quality code.
Why It’s Essential:
- Comprehensive Coverage: It covers nearly every aspect of software construction, from variables to high-level design.
- Evidence-Based Approach: The book backs up its recommendations with empirical data and research, providing a scientific basis for best practices.
- Language-Agnostic: While it uses examples from various languages, the principles apply across programming languages and paradigms.
- Focus on Quality: It emphasizes techniques for producing high-quality, maintainable code, which is crucial for long-term project success.
Key Takeaways:
- Software construction is the most important activity in software development and deserves more attention than it often receives.
- Good planning and preparation before coding can significantly reduce errors and improve efficiency.
- Code should be written for readability and maintainability, not just for the computer to execute.
- Defensive programming techniques can help create more robust and reliable software.
- Continuous refactoring and code improvement should be part of the development process.
Here’s an example of applying principles from “Code Complete” to improve code quality:
// Before: Hard to read and maintain
function p(d,m,y) {
var r=new Date(y,m-1,d);
var n=new Date();
var diff=n-r;
var a=diff/(1000*60*60*24*365.25);
return Math.floor(a);
}
// After: Applying Code Complete principles
function calculateAge(birthDate) {
const millisecondsPerYear = 1000 * 60 * 60 * 24 * 365.25;
const birthDateTime = new Date(birthDate).getTime();
const currentDateTime = Date.now();
const ageInMilliseconds = currentDateTime - birthDateTime;
const ageInYears = ageInMilliseconds / millisecondsPerYear;
return Math.floor(ageInYears);
}
// Usage
const age = calculateAge("1990-05-15");
console.log(`The person is ${age} years old.`);
“Code Complete” is a treasure trove of software development wisdom. Its comprehensive coverage of software construction practices makes it an invaluable resource for programmers looking to improve the quality of their code and their overall approach to software development.
Conclusion: Building a Strong Foundation for Programming Excellence
These five books represent a wealth of knowledge that can significantly enhance your programming skills and career. Each book offers unique insights:
- “Clean Code” teaches you how to write code that is not just functional, but also maintainable and professional.
- “Introduction to Algorithms” provides the fundamental knowledge of algorithms and data structures necessary for efficient problem-solving.
- “Design Patterns” introduces you to proven solutions for common software design problems, improving your ability to create flexible and maintainable systems.
- “The Pragmatic Programmer” offers practical wisdom on how to approach software development as a craft and grow as a professional.
- “Code Complete” provides comprehensive guidance on all aspects of software construction, emphasizing quality and best practices.
Reading these books is just the beginning. The real value comes from applying the principles and practices they teach in your day-to-day programming work. As you integrate these concepts into your coding habits, you’ll find yourself writing cleaner, more efficient, and more maintainable code.
Remember, becoming an excellent programmer is a journey of continuous learning and improvement. These books provide a strong foundation, but the field of programming is always evolving. Stay curious, keep practicing, and never stop learning.
At AlgoCademy, we believe in the power of combining theoretical knowledge with practical application. That’s why we offer interactive coding tutorials and AI-powered assistance to help you apply these concepts in real-world scenarios. Whether you’re preparing for technical interviews or looking to level up your coding skills, these books, along with our resources, can help you become the programmer you aspire to be.
Happy reading, and happy coding!