Interactive Challenge: Can You Beat This Coding Puzzle?
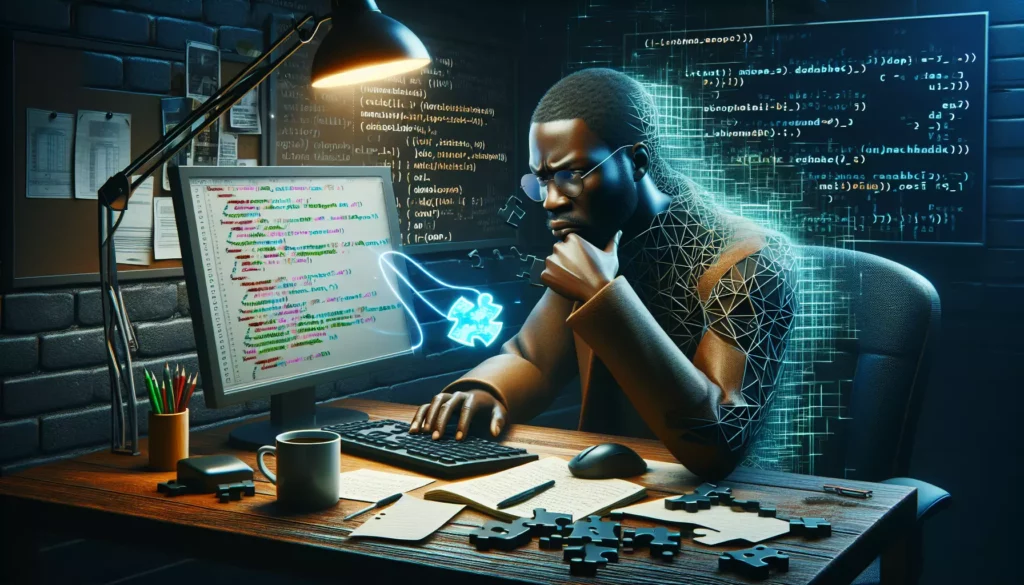
Welcome, coding enthusiasts and aspiring programmers! Today, we’re diving into an exciting interactive challenge that will put your algorithmic thinking and problem-solving skills to the test. As part of our ongoing mission at AlgoCademy to provide top-notch coding education and help you develop essential programming skills, we’ve crafted a coding puzzle that’s sure to get your synapses firing.
Before we jump into the challenge, let’s briefly discuss why exercises like these are crucial for your growth as a programmer, especially if you’re aiming to land a position at one of the FAANG (Facebook, Amazon, Apple, Netflix, Google) companies or other tech giants.
Why Coding Puzzles Matter
Coding puzzles and algorithmic challenges are more than just fun brain teasers. They play a vital role in developing the kind of analytical thinking and problem-solving skills that are highly valued in the tech industry. Here’s why they’re so important:
- Sharpening Algorithmic Thinking: These puzzles force you to think about efficiency and optimal solutions, which is crucial when dealing with large-scale systems.
- Improving Problem-Solving Skills: By tackling diverse challenges, you learn to approach problems from different angles and develop a toolkit of problem-solving strategies.
- Interview Preparation: Many tech companies, especially FAANG, use coding challenges as part of their interview process to assess candidates’ abilities.
- Enhancing Code Quality: Regular practice with coding puzzles often leads to writing cleaner, more efficient code in your day-to-day work.
- Boosting Confidence: As you solve more puzzles, you’ll gain confidence in your ability to tackle unknown problems, which is invaluable in a real-world programming environment.
Now that we understand the importance of these challenges, let’s dive into today’s puzzle!
The Coding Puzzle: Balanced Brackets
Our challenge today focuses on a classic problem that often appears in coding interviews: determining whether a string of brackets is balanced. This problem tests your ability to work with stacks, a fundamental data structure, and your understanding of string manipulation.
Problem Statement
Given a string containing just the characters ‘(‘, ‘)’, ‘{‘, ‘}’, ‘[‘ and ‘]’, determine if the input string is valid. An input string is valid if:
- Open brackets must be closed by the same type of brackets.
- Open brackets must be closed in the correct order.
- Every close bracket has a corresponding open bracket of the same type.
Examples
- Input: “()” – Output: true
- Input: “()[]{}” – Output: true
- Input: “(]” – Output: false
- Input: “([)]” – Output: false
- Input: “{[]}” – Output: true
The Challenge
Your task is to write a function that takes a string of brackets as input and returns true if the string is valid according to the rules above, and false otherwise. Here’s a template to get you started:
function isValid(s: string): boolean {
// Your code here
}
Hints
If you’re stuck, here are a few hints to guide you:
- Consider using a stack data structure to keep track of opening brackets.
- Think about what happens when you encounter a closing bracket.
- Remember to handle the case where there might be closing brackets without corresponding opening brackets.
Solution Walkthrough
Now, let’s walk through a solution to this problem. Remember, there are multiple ways to solve this, but we’ll focus on an efficient approach using a stack.
Step 1: Understanding the Approach
The key to solving this problem is to use a stack to keep track of opening brackets. As we iterate through the string, we’ll follow these rules:
- If we encounter an opening bracket, we push it onto the stack.
- If we encounter a closing bracket, we check if the stack is empty (which would mean we have a closing bracket without a corresponding opening bracket). If it’s not empty, we pop the top element from the stack and check if it matches the current closing bracket.
- After processing all characters, the stack should be empty for a valid string.
Step 2: Implementing the Solution
Here’s an implementation of the solution in TypeScript:
function isValid(s: string): boolean {
const stack: string[] = [];
const bracketPairs: { [key: string]: string } = {
')': '(',
'}': '{',
']': '['
};
for (let char of s) {
if (!bracketPairs[char]) {
// It's an opening bracket, push to stack
stack.push(char);
} else {
// It's a closing bracket
if (stack.length === 0 || stack.pop() !== bracketPairs[char]) {
return false;
}
}
}
// The string is valid if the stack is empty at the end
return stack.length === 0;
}
Step 3: Explaining the Code
Let’s break down the solution:
- We initialize an empty stack to store opening brackets.
- We create an object
bracketPairs
that maps closing brackets to their corresponding opening brackets. This will help us quickly check if brackets match. - We iterate through each character in the input string:
- If the character is not in
bracketPairs
(i.e., it’s an opening bracket), we push it onto the stack. - If it’s a closing bracket, we check two conditions:
- Is the stack empty? If so, we have a closing bracket without an opening bracket, so we return false.
- Does the top of the stack (which we pop) match the corresponding opening bracket for our current closing bracket? If not, we return false.
Step 4: Time and Space Complexity
Let’s analyze the efficiency of our solution:
- Time Complexity: O(n), where n is the length of the input string. We iterate through the string once.
- Space Complexity: O(n) in the worst case, where the string contains only opening brackets, and we push all of them onto the stack.
Testing Your Solution
To ensure your solution works correctly, it’s crucial to test it with various inputs. Here are some test cases you can use:
console.log(isValid("()")); // Should output: true
console.log(isValid("()[]{}")); // Should output: true
console.log(isValid("(]")); // Should output: false
console.log(isValid("([)]")); // Should output: false
console.log(isValid("{[]}")); // Should output: true
console.log(isValid("")); // Should output: true (empty string is considered valid)
console.log(isValid("(((")); // Should output: false
console.log(isValid(")))")); // Should output: false
console.log(isValid("({[]})")); // Should output: true
Extending the Challenge
If you’ve successfully implemented the solution and want to push yourself further, here are some ways to extend the challenge:
- Handle Different Bracket Types: Modify your function to handle additional types of brackets or parentheses, such as < and >, or even custom bracket pairs.
- Identify the Position of the Error: Instead of just returning a boolean, modify your function to return the index of the first bracket that causes the string to be invalid.
- Optimize for Space: Can you solve this problem without using additional data structures? (Hint: This is possible but may increase time complexity)
- Balance with Other Characters: Modify the function to work with strings that contain other characters besides brackets. Ignore non-bracket characters but still check if the brackets are balanced.
Conclusion
Congratulations on tackling this coding puzzle! Whether you solved it on your own, followed along with the solution, or are still working on it, you’ve taken an important step in developing your programming skills. Remember, the key to mastering these challenges is consistent practice and a willingness to learn from each attempt.
At AlgoCademy, we believe that interactive challenges like these are fundamental to your growth as a programmer. They not only prepare you for technical interviews at top tech companies but also enhance your problem-solving abilities and algorithmic thinking, which are invaluable skills in any programming career.
As you continue your coding journey, we encourage you to:
- Practice regularly with diverse coding challenges
- Analyze and understand multiple solutions to the same problem
- Focus on optimizing both time and space complexity in your solutions
- Collaborate with peers and learn from their approaches
- Utilize AlgoCademy’s resources, including our AI-powered assistance and step-by-step guidance, to enhance your learning
Remember, every puzzle you solve brings you one step closer to achieving your programming goals, whether that’s acing a FAANG interview or becoming a more proficient developer overall. Keep coding, keep learning, and most importantly, enjoy the process!
Stay tuned for more interactive challenges, coding tutorials, and programming insights from AlgoCademy. Happy coding!