From Zero to Hero: A Beginner’s Journey to Coding Mastery
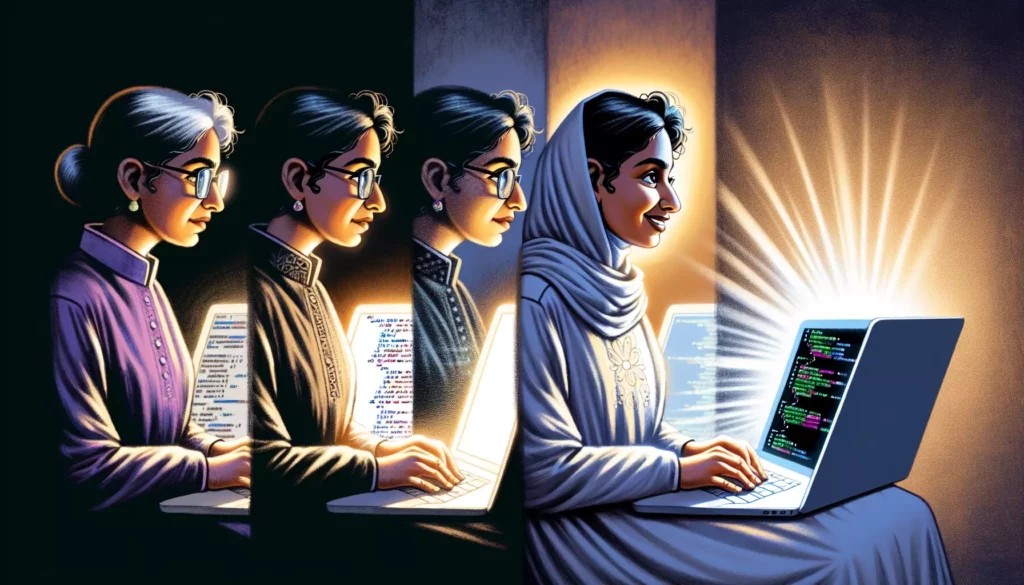
Embarking on a journey to become a coding master can seem daunting, especially if you’re starting from scratch. But fear not! With the right mindset, resources, and dedication, anyone can transform from a complete novice to a confident programmer. In this comprehensive guide, we’ll walk you through the steps to go from zero to hero in your coding journey, with a special focus on how platforms like AlgoCademy can accelerate your learning and prepare you for success in the tech industry.
1. Understanding the Basics: What is Coding?
Before diving into the nitty-gritty of programming, it’s essential to understand what coding actually is. At its core, coding is the process of creating instructions for computers to follow. These instructions, written in various programming languages, allow us to create websites, mobile apps, software, and much more.
Coding involves:
- Problem-solving
- Logical thinking
- Creativity
- Attention to detail
As a beginner, it’s important to realize that coding is a skill that can be learned and improved over time. Don’t be discouraged if things seem confusing at first – every expert was once a beginner!
2. Choosing Your First Programming Language
With numerous programming languages available, choosing your first one can be overwhelming. Here are some popular options for beginners:
- Python: Known for its simplicity and readability, Python is an excellent choice for beginners. It’s versatile and widely used in various fields, including web development, data science, and artificial intelligence.
- JavaScript: If you’re interested in web development, JavaScript is a must-learn language. It’s essential for creating interactive websites and is also used in backend development with Node.js.
- Java: A popular language for building enterprise-level applications, Java is also commonly used in Android app development.
- C++: While more complex, C++ is powerful and widely used in game development and system programming.
For most beginners, Python is an excellent starting point due to its gentle learning curve and wide applicability. Platforms like AlgoCademy often start with Python tutorials to help newcomers grasp fundamental programming concepts.
3. Setting Up Your Development Environment
Once you’ve chosen a language, you’ll need to set up your development environment. This typically involves:
- Installing the programming language on your computer
- Choosing and installing an Integrated Development Environment (IDE) or text editor
- Setting up version control (like Git)
For beginners, using online coding platforms can be a great way to start without the hassle of setting up a local environment. AlgoCademy, for instance, provides an interactive coding environment where you can write and run code directly in your browser.
4. Learning the Fundamentals
Every programming language has fundamental concepts that you need to master. These typically include:
- Variables and data types
- Control structures (if statements, loops)
- Functions and methods
- Basic input/output operations
- Data structures (lists, arrays, dictionaries)
Here’s a simple Python example demonstrating some of these concepts:
# A simple Python program to calculate the area of a rectangle
def calculate_area(length, width):
return length * width
# Get input from the user
length = float(input("Enter the length of the rectangle: "))
width = float(input("Enter the width of the rectangle: "))
# Calculate and display the area
area = calculate_area(length, width)
print(f"The area of the rectangle is: {area}")
This example showcases variables, functions, user input, and basic arithmetic operations. As you progress, you’ll learn more complex concepts and how to apply them in real-world scenarios.
5. Practice, Practice, Practice
The key to mastering coding is consistent practice. Here are some ways to hone your skills:
- Coding challenges: Platforms like AlgoCademy offer daily coding challenges that test your problem-solving skills and help you apply what you’ve learned.
- Personal projects: Start small with projects like a calculator or a simple game, then gradually increase complexity as you improve.
- Open-source contributions: Once you’re comfortable, contributing to open-source projects can provide real-world experience and networking opportunities.
Remember, making mistakes is part of the learning process. Don’t be afraid to experiment and learn from your errors.
6. Understanding Algorithms and Data Structures
As you advance in your coding journey, understanding algorithms and data structures becomes crucial. These form the backbone of efficient programming and are often the focus of technical interviews at major tech companies.
Key areas to study include:
- Array manipulation
- Linked lists
- Stacks and queues
- Trees and graphs
- Sorting and searching algorithms
- Dynamic programming
AlgoCademy’s curriculum is designed to gradually introduce these concepts, providing explanations and practical examples to reinforce your understanding.
7. Developing Problem-Solving Skills
Coding is essentially problem-solving. To excel, you need to develop a systematic approach to tackling coding challenges. Here’s a general problem-solving framework:
- Understand the problem thoroughly
- Break down the problem into smaller, manageable parts
- Plan your approach (pseudocode can be helpful)
- Implement your solution
- Test and debug
- Optimize if necessary
Platforms like AlgoCademy often provide step-by-step guidance for solving complex problems, helping you develop this crucial skill.
8. Learning Object-Oriented Programming (OOP)
Object-Oriented Programming is a programming paradigm that’s widely used in modern software development. It’s based on the concept of “objects” that contain data and code. Understanding OOP principles will make you a more versatile programmer.
Key OOP concepts include:
- Classes and objects
- Inheritance
- Encapsulation
- Polymorphism
Here’s a simple Python example demonstrating a class:
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
def bark(self):
print(f"{self.name} says Woof!")
# Creating an instance of the Dog class
my_dog = Dog("Buddy", 3)
# Using the bark method
my_dog.bark() # Output: Buddy says Woof!
9. Exploring Different Areas of Programming
As you become more comfortable with coding, you might want to explore different specializations. Some popular areas include:
- Web Development: Building websites and web applications using technologies like HTML, CSS, JavaScript, and various frameworks.
- Mobile App Development: Creating applications for iOS and Android devices.
- Data Science and Machine Learning: Analyzing data and creating predictive models using statistical techniques and AI.
- Game Development: Designing and programming video games.
- Cybersecurity: Protecting computer systems and networks from digital attacks.
Platforms like AlgoCademy often offer specialized tracks or modules for these different areas, allowing you to get a taste of various specializations.
10. Building a Portfolio
As you progress in your coding journey, it’s important to showcase your skills. Building a portfolio of projects demonstrates your abilities to potential employers or clients. Here are some tips for creating an impressive coding portfolio:
- Include a variety of projects that demonstrate different skills
- Provide clear documentation for each project
- Include the source code (e.g., on GitHub) and live demos where possible
- Highlight your problem-solving process and any challenges you overcame
Many coding education platforms, including AlgoCademy, encourage students to work on portfolio-worthy projects as part of their learning process.
11. Networking and Community Involvement
Programming isn’t just about writing code – it’s also about being part of a community. Engaging with other developers can accelerate your learning, provide support, and even lead to job opportunities. Here are some ways to get involved:
- Join coding forums and discussion groups
- Attend local meetups or coding events
- Participate in hackathons
- Contribute to open-source projects
- Share your knowledge through blogging or creating tutorials
Many online learning platforms, including AlgoCademy, have community features that allow you to connect with fellow learners and mentors.
12. Preparing for Technical Interviews
As you set your sights on a career in tech, preparing for technical interviews becomes crucial. These interviews often focus on your problem-solving skills, knowledge of algorithms and data structures, and ability to write efficient code.
Key areas to focus on include:
- Time and space complexity analysis
- Common algorithmic patterns (e.g., two-pointers, sliding window)
- System design basics
- Behavioral questions
Platforms like AlgoCademy often provide specialized interview preparation modules, including mock interviews and company-specific question banks, particularly focusing on FAANG (Facebook, Amazon, Apple, Netflix, Google) and other major tech companies.
13. Continuous Learning and Staying Updated
The field of technology is constantly evolving, and as a programmer, it’s crucial to keep your skills up-to-date. Here are some strategies for continuous learning:
- Follow tech blogs and news sites
- Attend webinars and online conferences
- Take advanced courses or specialize in new areas
- Experiment with new technologies and frameworks
- Read books and research papers in your areas of interest
Many coding education platforms, including AlgoCademy, regularly update their content to reflect industry trends and new technologies, helping you stay current in your skills.
14. Leveraging AI-Powered Learning Assistance
As AI technologies advance, they’re increasingly being integrated into coding education. Platforms like AlgoCademy often incorporate AI-powered features to enhance the learning experience:
- Personalized learning paths: AI algorithms can analyze your progress and tailor the curriculum to your strengths and weaknesses.
- Intelligent code analysis: AI can review your code, suggest improvements, and explain errors in natural language.
- Adaptive difficulty: The difficulty of challenges can automatically adjust based on your skill level.
- Natural language explanations: Complex concepts can be explained in simple terms, adapting to your level of understanding.
These AI-powered tools can significantly accelerate your learning process, providing instant feedback and personalized guidance.
15. From Learning to Earning: Transitioning to a Career in Tech
As you near the end of your beginner’s journey and approach coding mastery, it’s time to think about how to transition these skills into a career. Here are some steps to consider:
- Identify your niche: Based on your interests and strengths, decide which area of programming you want to specialize in.
- Polish your resume: Highlight your projects, skills, and any relevant certifications or courses you’ve completed.
- Build your online presence: Create profiles on professional networking sites like LinkedIn and GitHub.
- Apply for internships or entry-level positions: These can provide valuable real-world experience and often lead to full-time positions.
- Prepare for interviews: Practice coding challenges, review fundamental concepts, and prepare for behavioral questions.
- Consider freelancing: Platforms like Upwork or Freelancer can be great for gaining experience and building a client base.
- Never stop learning: The tech industry evolves rapidly, so commit to continuous learning and improvement.
Remember, everyone’s journey is unique. Some may land a job quickly, while others might take a bit longer. The key is to stay persistent, continue improving your skills, and maintain a positive attitude.
Conclusion: Your Coding Journey Awaits
The path from coding novice to programming expert is filled with challenges, exciting discoveries, and rewarding achievements. While it requires dedication and perseverance, the journey is well worth the effort. With the right resources, such as interactive platforms like AlgoCademy, and a commitment to continuous learning, you can transform from a complete beginner to a confident, skilled programmer ready to tackle real-world challenges.
Remember, every expert was once a beginner. Your journey to coding mastery starts with a single line of code. So why wait? Start your coding adventure today, and before you know it, you’ll be well on your way from zero to hero in the world of programming!
Happy coding!