The 7 Habits of Highly Successful Programmers
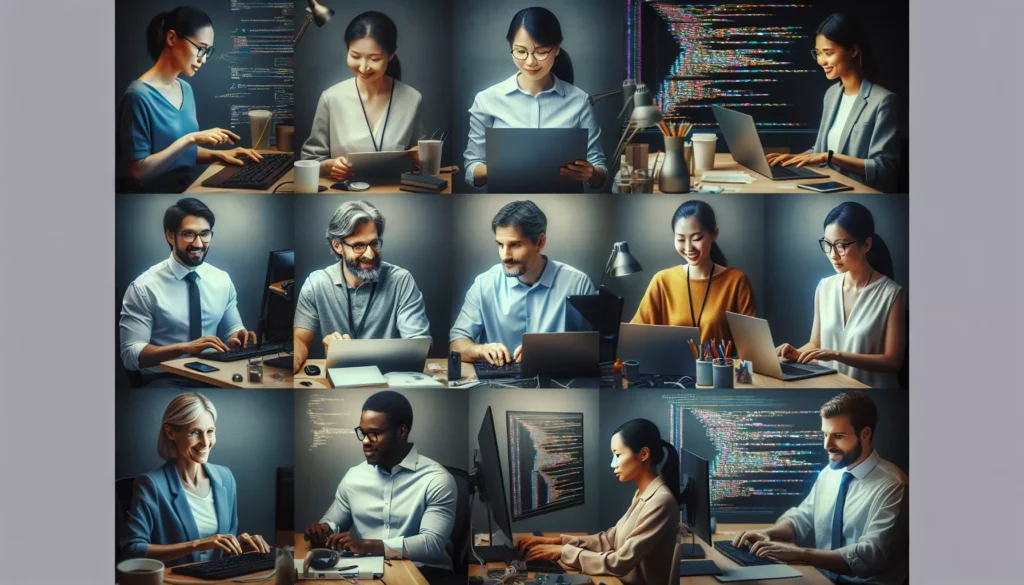
In the fast-paced world of software development, success isn’t just about writing code; it’s about cultivating habits that foster growth, efficiency, and innovation. Whether you’re a seasoned developer or just starting your journey in programming, adopting the right habits can significantly impact your career trajectory. In this comprehensive guide, we’ll explore the seven habits that set highly successful programmers apart from the rest.
1. Continuous Learning
The tech industry is known for its rapid evolution. New languages, frameworks, and methodologies emerge constantly, making continuous learning not just beneficial but essential for programmers who want to stay relevant and competitive.
Why It Matters
- Keeps skills sharp and up-to-date
- Enhances problem-solving abilities
- Opens up new career opportunities
- Fosters innovation and creativity
How to Cultivate This Habit
- Dedicate time daily: Set aside at least 30 minutes each day for learning.
- Diversify your learning sources: Utilize online courses, coding bootcamps, tech blogs, and programming books.
- Practice with personal projects: Apply new concepts by building small projects or contributing to open-source initiatives.
- Attend tech conferences and meetups: Network with other developers and stay informed about industry trends.
Remember, the goal isn’t to know everything but to maintain a growth mindset and adaptability in the face of technological change.
2. Writing Clean and Readable Code
Clean code is not just about aesthetics; it’s about efficiency, maintainability, and collaboration. Successful programmers understand that their code will be read by others (and their future selves) and strive to make it as clear and understandable as possible.
Key Principles of Clean Code
- Meaningful variable and function names
- Consistent formatting and indentation
- DRY (Don’t Repeat Yourself) principle
- SOLID principles for object-oriented design
- Appropriate comments and documentation
Implementing Clean Code Practices
Here’s an example of how to refactor code for better readability:
// Before
function calc(a, b, c) {
return a * b + c;
}
// After
function calculateTotalPrice(basePrice, taxRate, shippingCost) {
const priceWithTax = basePrice * (1 + taxRate);
return priceWithTax + shippingCost;
}
By using descriptive names and breaking down the calculation, the function becomes self-explanatory and easier to maintain.
3. Effective Problem-Solving and Debugging
At its core, programming is about solving problems. Highly successful programmers excel at breaking down complex issues into manageable parts and systematically debugging when things go wrong.
Problem-Solving Strategies
- Understand the problem thoroughly: Before diving into code, ensure you grasp all aspects of the problem.
- Plan before coding: Outline your approach, considering different solutions.
- Break it down: Divide complex problems into smaller, more manageable tasks.
- Use pseudocode: Sketch out the logic before writing actual code.
- Test as you go: Implement incremental testing to catch issues early.
Debugging Techniques
- Use debugging tools effectively (e.g., breakpoints, watch variables)
- Practice rubber duck debugging
- Read error messages carefully
- Isolate the problem through systematic testing
- Keep a debugging journal to track recurrent issues and solutions
4. Version Control Mastery
Version control systems like Git are indispensable tools in modern software development. Mastering version control allows programmers to collaborate effectively, track changes, and maintain code integrity.
Benefits of Version Control Mastery
- Facilitates collaboration in team environments
- Provides a safety net for experimentation
- Enables easy rollback to previous versions
- Helps in tracking changes and understanding code evolution
Essential Git Commands Every Programmer Should Know
git init # Initialize a new Git repository
git clone <url> # Clone a repository
git add <file> # Stage changes
git commit -m "message" # Commit changes
git push # Push changes to remote repository
git pull # Pull changes from remote repository
git branch # List, create, or delete branches
git merge <branch> # Merge branches
git stash # Temporarily store modified, tracked files
Practice these commands regularly to become proficient in version control.
5. Time Management and Productivity
Effective time management is crucial for programmers who often juggle multiple projects, deadlines, and continuous learning. Successful programmers develop strategies to maximize their productivity without burning out.
Time Management Techniques
- Pomodoro Technique: Work in focused 25-minute intervals followed by short breaks.
- Time blocking: Allocate specific time slots for different tasks or projects.
- Eisenhower Matrix: Prioritize tasks based on urgency and importance.
- Task batching: Group similar tasks together to minimize context switching.
Productivity Tools for Programmers
- Project management tools (e.g., Trello, Jira)
- Time tracking apps (e.g., RescueTime, Toggl)
- Code editors with productivity features (e.g., VS Code extensions)
- Note-taking apps for organizing thoughts and ideas (e.g., Notion, Evernote)
Remember, the key is to find a system that works for you and stick to it consistently.
6. Collaboration and Communication Skills
Programming is rarely a solitary activity. Successful programmers excel not just in technical skills but also in their ability to work effectively with others, communicate ideas clearly, and contribute positively to team dynamics.
Enhancing Collaboration
- Actively participate in code reviews
- Use pair programming techniques when appropriate
- Contribute to and maintain documentation
- Be open to feedback and willing to learn from others
Improving Communication
- Practice explaining technical concepts: Use analogies and simple language to make complex ideas accessible.
- Enhance written communication: Write clear, concise emails and documentation.
- Active listening: Pay attention to team members’ ideas and concerns.
- Non-verbal communication: Be aware of body language in meetings and interactions.
Remember, effective communication can often be the difference between a project’s success and failure.
7. Code Optimization and Performance Awareness
While writing functional code is important, highly successful programmers also focus on optimizing their code for better performance. This habit involves understanding the impact of coding decisions on efficiency and scalability.
Key Areas of Code Optimization
- Algorithmic efficiency (time and space complexity)
- Memory management
- Database query optimization
- Front-end performance (load times, rendering efficiency)
Techniques for Performance Improvement
- Profiling: Use tools to identify performance bottlenecks.
- Benchmarking: Compare different implementations to find the most efficient solution.
- Caching: Implement appropriate caching strategies to reduce redundant computations.
- Lazy loading: Load resources only when needed to improve initial load times.
Here’s an example of optimizing a simple function for better performance:
// Before optimization
function findDuplicates(arr) {
let duplicates = [];
for (let i = 0; i < arr.length; i++) {
for (let j = i + 1; j < arr.length; j++) {
if (arr[i] === arr[j] && !duplicates.includes(arr[i])) {
duplicates.push(arr[i]);
}
}
}
return duplicates;
}
// After optimization
function findDuplicates(arr) {
let seen = new Set();
let duplicates = new Set();
for (let num of arr) {
if (seen.has(num)) {
duplicates.add(num);
} else {
seen.add(num);
}
}
return Array.from(duplicates);
}
The optimized version uses a Set for faster lookups and eliminates the nested loop, significantly improving performance for large arrays.
Conclusion
Becoming a highly successful programmer is a journey that extends far beyond just writing code. By cultivating these seven habits – continuous learning, writing clean code, effective problem-solving, version control mastery, time management, collaboration skills, and performance awareness – you’ll set yourself on a path to excellence in the field of software development.
Remember, developing these habits takes time and consistent effort. Start by focusing on one or two areas for improvement and gradually incorporate the others into your daily routine. With persistence and dedication, you’ll find yourself not just keeping up with the rapidly evolving tech landscape but leading the way in innovation and efficiency.
As you progress in your programming career, always keep in mind that these habits are not just about personal success; they contribute to creating better software, more efficient teams, and ultimately, a more innovative and impactful tech industry. Embrace these habits, and watch as they transform not just your code, but your entire approach to software development.