The Ultimate Guide to Cracking Coding Interviews in 2023
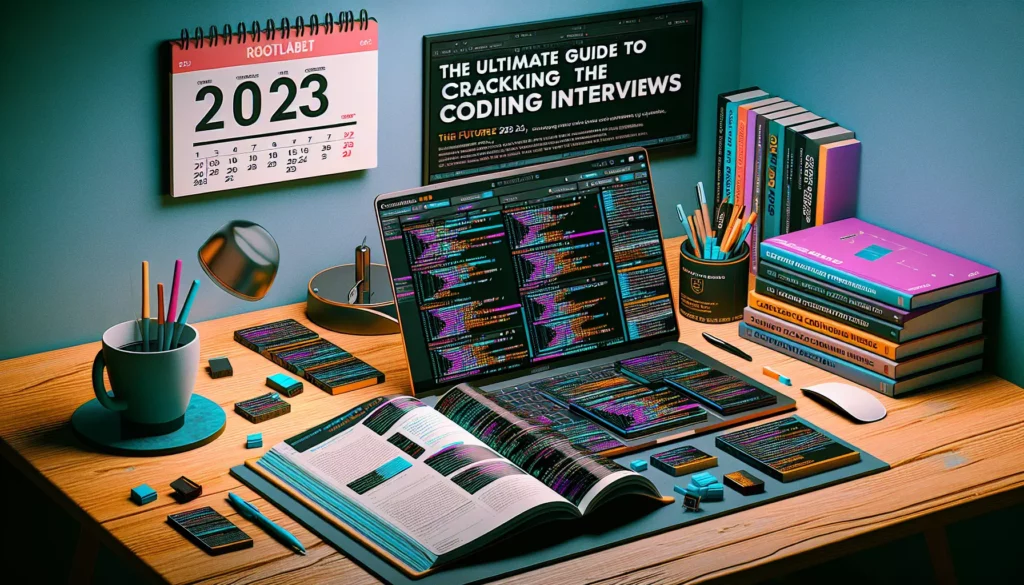
In today’s competitive tech landscape, mastering the art of coding interviews is crucial for landing your dream job at top companies like Google, Amazon, or Facebook. As we navigate through 2023, the interview process continues to evolve, and staying ahead of the curve is more important than ever. This comprehensive guide will equip you with the knowledge, strategies, and resources you need to ace your coding interviews and launch your career in the tech industry.
Table of Contents
- Understanding Coding Interviews
- Essential Skills for Coding Interviews
- Preparation Strategies
- Common Coding Interview Questions and Topics
- Problem-Solving Techniques
- The Importance of Mock Interviews
- Handling Behavioral Questions
- Interview Day: What to Expect and How to Perform
- Post-Interview Follow-up and Learning from Feedback
- Resources for Continuous Learning and Practice
1. Understanding Coding Interviews
Coding interviews are a unique blend of technical assessment and problem-solving evaluation. They’re designed to test not just your coding skills, but also your ability to think critically, communicate effectively, and work under pressure. In 2023, many companies have adapted their interview processes to include remote options, which brings its own set of challenges and opportunities.
Types of Coding Interviews
- Online Assessments: Often the first step, these are automated coding tests that evaluate your basic programming skills.
- Phone Screens: A preliminary interview, usually with an engineer, to assess your background and basic technical knowledge.
- Technical Interviews: In-depth sessions where you’ll be asked to solve coding problems in real-time, often using a shared coding environment.
- System Design Interviews: For more senior positions, you may be asked to design large-scale systems or architectures.
- Behavioral Interviews: These assess your soft skills, past experiences, and cultural fit.
What Interviewers Look For
Understanding what interviewers are evaluating can help you focus your preparation:
- Problem-solving skills: How you approach and break down complex problems.
- Coding proficiency: Your ability to translate ideas into clean, efficient code.
- Algorithmic knowledge: Understanding of fundamental algorithms and data structures.
- Communication: How well you explain your thought process and collaborate with the interviewer.
- Adaptability: Your ability to handle hints, incorporate feedback, and optimize solutions.
2. Essential Skills for Coding Interviews
To excel in coding interviews, you need to develop a strong foundation in several key areas:
Programming Languages
While many companies allow you to code in your preferred language, it’s beneficial to be proficient in at least one of these popular languages:
- Python
- Java
- C++
- JavaScript
Choose a language you’re comfortable with and stick to it for your interview preparation. Consistency will help you focus on problem-solving rather than syntax.
Data Structures
A solid understanding of data structures is crucial. Focus on:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
Know how to implement these structures from scratch and understand their time and space complexities.
Algorithms
Familiarity with common algorithms and their applications is essential:
- Sorting (QuickSort, MergeSort, HeapSort)
- Searching (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Recursion and Backtracking
Time and Space Complexity Analysis
Being able to analyze and optimize the efficiency of your solutions is crucial. Understand:
- Big O notation
- Best, average, and worst-case scenarios
- Space-time tradeoffs
Problem-Solving Strategies
Develop a systematic approach to tackling coding problems:
- Understand the problem thoroughly
- Identify the inputs and outputs
- Consider edge cases and constraints
- Develop a high-level algorithm
- Implement the solution
- Test and optimize
3. Preparation Strategies
Effective preparation is key to success in coding interviews. Here’s a comprehensive strategy to get you ready:
Create a Study Plan
Develop a structured study plan that covers all essential topics. Allocate time for:
- Learning and reviewing concepts
- Practicing coding problems
- Mock interviews
- Reviewing and reflecting on your progress
Consistent Practice
Aim to solve coding problems regularly. Start with easier problems and gradually increase the difficulty. Platforms like LeetCode, HackerRank, and AlgoCademy offer a wide range of problems to practice.
Time Management
Practice solving problems within time constraints. Most coding interviews last 45-60 minutes, so aim to solve problems within this timeframe.
Learn from Solutions
After solving a problem, always review other solutions. This exposes you to different approaches and often more efficient solutions.
Focus on Weak Areas
Identify topics or problem types you struggle with and allocate extra time to improve in these areas.
Stay Updated
Keep abreast of the latest trends in coding interviews. Companies often update their interview processes, and new types of questions may emerge.
4. Common Coding Interview Questions and Topics
While it’s impossible to predict exact questions, certain topics and problem types frequently appear in coding interviews:
Array and String Manipulation
- Two-pointer techniques
- Sliding window problems
- Subarray problems
Example Problem: Two Sum
def two_sum(nums, target):
num_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_map:
return [num_map[complement], i]
num_map[num] = i
return []
Linked Lists
- Reversing a linked list
- Detecting cycles
- Merging sorted lists
Trees and Graphs
- Tree traversals (inorder, preorder, postorder)
- Binary search tree operations
- Graph traversals (DFS, BFS)
- Shortest path algorithms
Example Problem: Inorder Traversal of a Binary Tree
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal(root):
result = []
def inorder(node):
if node:
inorder(node.left)
result.append(node.val)
inorder(node.right)
inorder(root)
return result
Dynamic Programming
- Fibonacci sequence
- Longest common subsequence
- Knapsack problem
Sorting and Searching
- Implementing sorting algorithms
- Binary search and its variations
- Search in rotated sorted array
System Design (for more senior positions)
- Designing a URL shortener
- Creating a social media feed
- Implementing a distributed cache
5. Problem-Solving Techniques
Mastering problem-solving techniques is crucial for tackling unfamiliar problems during interviews:
Break It Down
When faced with a complex problem, break it down into smaller, manageable sub-problems. Solve each sub-problem independently and then combine the solutions.
Start with a Brute Force Approach
Begin with the simplest solution that comes to mind, even if it’s inefficient. This demonstrates your problem-solving ability and provides a starting point for optimization.
Optimize Step by Step
Once you have a working solution, look for ways to optimize it. Consider:
- Can you reduce the time complexity?
- Is there a more efficient data structure you could use?
- Can you reduce the space complexity?
Think Aloud
Verbalize your thought process. This helps the interviewer understand your approach and allows them to provide hints if needed.
Use Examples
Work through small examples to understand the problem better and to test your solution.
Pattern Recognition
Learn to recognize common problem patterns. For example:
- Two-pointer technique for array problems
- Hash map for lookup optimization
- Sliding window for substring problems
- Tree traversal patterns for tree problems
Time Management
Keep track of time during the interview. If you’re stuck, don’t spend too long on one approach. Be ready to pivot or ask for a hint.
6. The Importance of Mock Interviews
Mock interviews are one of the most effective ways to prepare for real coding interviews. They help you:
- Simulate the pressure of a real interview
- Practice explaining your thought process
- Improve your time management
- Get feedback on your performance
How to Conduct Mock Interviews
- Find a Partner: This could be a friend, a colleague, or a professional mock interview service.
- Set the Scene: Use a shared coding environment and a video call to mimic a real interview setting.
- Choose Realistic Problems: Use questions from resources like LeetCode or actual interview experiences shared online.
- Time It: Stick to a realistic timeframe, usually 45-60 minutes.
- Provide and Receive Feedback: After the interview, discuss what went well and areas for improvement.
Self-Mock Interviews
If you can’t find a partner, you can still benefit from self-mock interviews:
- Set a timer and solve problems as if you were in an interview
- Explain your thought process out loud
- Record yourself and review the recording to identify areas for improvement
7. Handling Behavioral Questions
While technical skills are crucial, many companies also place a high value on soft skills and cultural fit. Be prepared to answer behavioral questions such as:
- “Tell me about a time when you faced a significant challenge in a project.”
- “How do you handle disagreements with team members?”
- “Describe a situation where you had to learn a new technology quickly.”
The STAR Method
Use the STAR method to structure your responses to behavioral questions:
- Situation: Describe the context of the event.
- Task: Explain what you were responsible for in that situation.
- Action: Describe the specific actions you took.
- Result: Share the outcomes of your actions.
Prepare Your Stories
Have a few go-to stories that showcase your skills and experiences. These might include:
- A challenging project you completed
- A time you demonstrated leadership
- An instance where you resolved a conflict
- A situation where you learned from failure
8. Interview Day: What to Expect and How to Perform
On the day of your interview, being well-prepared can help you stay calm and perform at your best.
Before the Interview
- Get a good night’s sleep
- Eat a healthy meal
- Review your notes, but don’t try to cram new information
- Test your technical setup if it’s a remote interview
- Have a pen and paper ready for notes
During the Interview
- Listen Carefully: Make sure you understand the problem fully before starting to code.
- Clarify Requirements: Ask questions about inputs, outputs, and any constraints.
- Think Aloud: Share your thought process as you work through the problem.
- Start with a High-Level Approach: Outline your solution before diving into coding.
- Write Clean, Readable Code: Use meaningful variable names and add comments where necessary.
- Test Your Solution: Run through test cases, including edge cases.
- Optimize: If time allows, discuss potential optimizations.
- Stay Calm: If you get stuck, take a deep breath and consider asking for a hint.
Common Pitfalls to Avoid
- Jumping into coding without fully understanding the problem
- Ignoring edge cases
- Writing overly complex solutions
- Failing to communicate your thought process
- Getting defensive about feedback
9. Post-Interview Follow-up and Learning from Feedback
The interview process doesn’t end when you walk out of the room or log off the video call. Proper follow-up can leave a lasting positive impression.
Send a Thank You Note
Within 24 hours of your interview, send a brief email to your interviewer or the HR contact:
- Express your appreciation for their time
- Reiterate your interest in the position
- Briefly mention a specific point from the interview that you found interesting
Reflect on Your Performance
Take some time to review how the interview went:
- What questions were you comfortable with?
- Where did you struggle?
- What could you have done differently?
Request Feedback
If you don’t receive an offer, politely ask for feedback on your performance. This can provide valuable insights for future interviews.
Learn from the Experience
Use the interview as a learning opportunity:
- Research any topics or questions you found challenging
- Practice similar problems to those you encountered
- Adjust your preparation strategy based on your reflections
10. Resources for Continuous Learning and Practice
To stay sharp and continue improving your coding interview skills, leverage these resources:
Online Platforms
- LeetCode: Offers a vast collection of coding problems with solutions and discussions.
- HackerRank: Provides coding challenges and competitions.
- AlgoCademy: Offers interactive coding tutorials and AI-powered assistance for learning algorithms and data structures.
- CodeSignal: Features company-specific assessments and practice problems.
Books
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Elements of Programming Interviews” by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash
- “Introduction to Algorithms” by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein
Online Courses
- Coursera’s “Algorithms Specialization” by Stanford University
- MIT OpenCourseWare’s “Introduction to Algorithms”
- Udacity’s “Data Structures and Algorithms Nanodegree”
YouTube Channels
- Back To Back SWE
- CS Dojo
- Algorithm Training by Errichto
Coding Communities
- Stack Overflow
- Reddit’s r/cscareerquestions and r/leetcode
- GitHub (for open-source contributions)
Conclusion
Cracking coding interviews in 2023 requires a combination of technical skills, problem-solving abilities, and effective communication. By following this guide and consistently practicing, you’ll be well-prepared to tackle even the most challenging interviews at top tech companies.
Remember, the key to success lies in continuous learning and practice. Treat each interview, whether successful or not, as a learning experience. Stay curious, keep coding, and don’t be discouraged by setbacks. With persistence and the right approach, you’ll be well on your way to landing your dream job in the tech industry.
Good luck with your coding interviews!