Papa Bear’s Coding Adventure: Teaching Mama Bear to Code
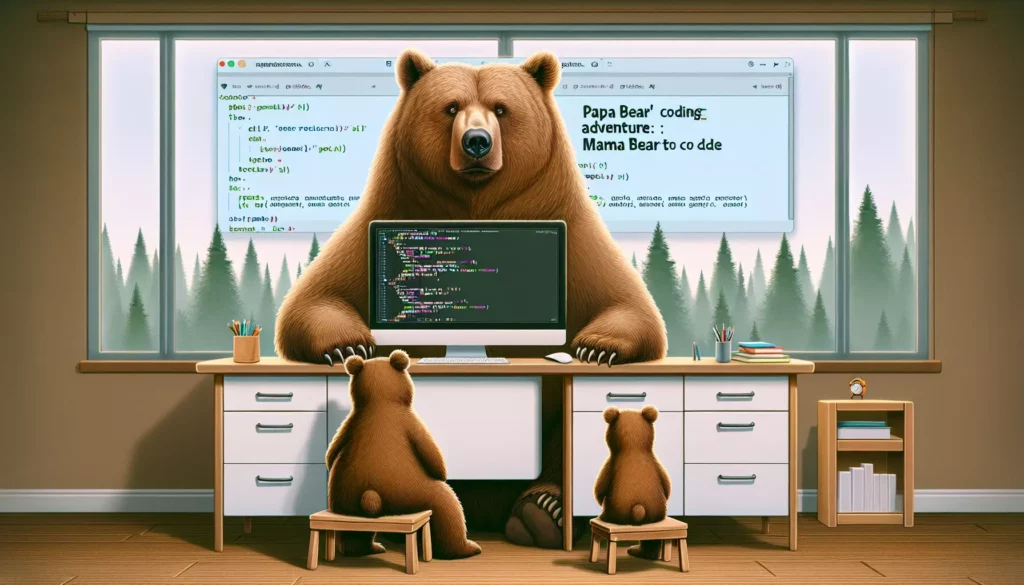
In the cozy den of the Bear family, nestled deep in the heart of the Codewood Forest, an exciting transformation was taking place. Papa Bear, known for his exceptional coding skills, had decided it was time to share his knowledge with Mama Bear. This heartwarming tale of love and learning is not just about a family of bears; it’s a metaphor for the journey many embark upon when entering the world of programming. Let’s dive into this fuzzy, code-filled adventure and see what lessons we can learn along the way.
The Beginning: Why Mama Bear Wanted to Learn
Mama Bear had always been curious about Papa Bear’s work. She’d watch him tap away at his keyboard, creating magical things on the screen. “Honey,” she’d say, “what exactly are you doing?” Papa Bear would smile and reply, “I’m coding, dear. I’m telling the computer what to do, and it listens!”
Intrigued by the power to command computers, Mama Bear decided it was time to learn. She wanted to understand this mysterious language that Papa Bear spoke so fluently. Plus, she had some ideas for apps that could make life in the forest easier for all the animals.
Setting Up the Den: Creating a Learning Environment
Papa Bear knew that a comfortable learning environment was crucial. He cleared a space in their den, set up two computers side by side, and made sure they had plenty of honey-flavored coding snacks nearby. He even hung a “Do Not Disturb” sign on the den entrance to keep Baby Bear from interrupting their lessons.
Creating the right environment is essential for any learner, especially in coding. A quiet space, a reliable computer, and the right software tools are the bare necessities for a budding programmer.
The First Lesson: Hello, Forest!
Papa Bear decided to start with the classic “Hello, World!” program, but with a forest twist. He opened up a text editor and typed:
print("Hello, Forest!")
Mama Bear’s eyes widened as the words appeared on the screen. “That’s it?” she asked, surprised at the simplicity. Papa Bear chuckled, “That’s just the beginning, dear. This is how every great coder starts their journey.”
Starting with simple, achievable tasks is crucial in coding education. It builds confidence and provides immediate feedback, encouraging the learner to continue.
Variables: Storing Honey and Berries
Next, Papa Bear introduced the concept of variables. He explained, “Think of variables like jars where we store different types of food. We can put honey in one jar and berries in another.”
He demonstrated with some Python code:
honey_jar = 100 # Measured in ounces
berry_basket = 50 # Number of berries
print(f"We have {honey_jar} ounces of honey and {berry_basket} berries.")
Mama Bear was fascinated. She realized she could use variables to keep track of their forest pantry inventory!
Conditional Statements: Deciding When to Hibernate
As autumn approached, Papa Bear saw an opportunity to teach conditional statements. He explained, “In coding, we often need to make decisions based on certain conditions, just like we decide when it’s time to hibernate.”
Together, they wrote a simple program:
temperature = 35 # in Fahrenheit
food_supply = "abundant"
if temperature < 40 and food_supply == "abundant":
print("Time to hibernate!")
else:
print("Let's stay awake a bit longer.")
Mama Bear loved how they could use code to make decisions. She started thinking about all the forest situations where this could be useful.
Loops: Counting Fish in the Stream
Papa Bear knew that repetitive tasks were perfect for teaching loops. He suggested they write a program to count fish in the stream. “Loops are like when we count fish jumping over a log. We keep counting until there are no more fish,” he explained.
They wrote this simple loop:
fish_count = 0
while fish_count < 10:
fish_count += 1
print(f"Counted {fish_count} fish!")
print("Finished counting fish.")
Mama Bear was thrilled. She could now automate counting tasks that used to take her hours!
Functions: Organizing Beehive Duties
As Mama Bear’s skills grew, Papa Bear introduced functions. He likened them to assigning tasks to different bears in the forest. “Functions are like specialized workers. We tell them what to do once, and then we can ask them to do it whenever we need,” he said.
They created a function to assign beehive duties:
def assign_beehive_duty(bear_name, duty):
return f"{bear_name} is assigned to {duty} the beehive today."
print(assign_beehive_duty("Mama Bear", "guard"))
print(assign_beehive_duty("Papa Bear", "harvest honey from"))
Mama Bear was excited about the possibilities. She could now create functions for all sorts of forest tasks!
Lists and Dictionaries: Organizing Forest Data
As they progressed, Papa Bear introduced more complex data structures. “Lists and dictionaries are like our forest encyclopedia. They help us organize and access information easily,” he explained.
They created a list of forest animals and a dictionary of their favorite foods:
forest_animals = ["Bear", "Deer", "Rabbit", "Squirrel"]
animal_foods = {
"Bear": "Fish and Berries",
"Deer": "Leaves and Grass",
"Rabbit": "Carrots and Lettuce",
"Squirrel": "Nuts and Seeds"
}
for animal in forest_animals:
print(f"{animal}'s favorite food is {animal_foods[animal]}.")
Mama Bear was amazed at how easily they could store and retrieve information about their forest friends.
Object-Oriented Programming: Creating a Forest Ecosystem
As Mama Bear’s skills advanced, Papa Bear decided it was time to introduce object-oriented programming. He explained, “Think of it like creating different types of bears, each with their own characteristics and behaviors.”
They created a simple Bear class:
class Bear:
def __init__(self, name, color, favorite_food):
self.name = name
self.color = color
self.favorite_food = favorite_food
def introduce(self):
return f"I'm {self.name}, a {self.color} bear, and I love {self.favorite_food}!"
mama_bear = Bear("Mama Bear", "brown", "honey")
papa_bear = Bear("Papa Bear", "black", "salmon")
print(mama_bear.introduce())
print(papa_bear.introduce())
Mama Bear was thrilled. She could now create entire forest ecosystems in code!
The Beary Big Project: Forest Management System
As Mama Bear’s coding skills flourished, Papa Bear suggested they work on a larger project together. They decided to create a Forest Management System to help organize and track various aspects of forest life.
Here’s a simplified version of their project:
class Animal:
def __init__(self, species, name, age):
self.species = species
self.name = name
self.age = age
class Tree:
def __init__(self, species, age, height):
self.species = species
self.age = age
self.height = height
class ForestArea:
def __init__(self, name):
self.name = name
self.animals = []
self.trees = []
def add_animal(self, animal):
self.animals.append(animal)
def add_tree(self, tree):
self.trees.append(tree)
def report(self):
print(f"Report for {self.name}:")
print(f"Number of animals: {len(self.animals)}")
print(f"Number of trees: {len(self.trees)}")
# Creating forest areas
berry_patch = ForestArea("Berry Patch")
fishing_stream = ForestArea("Fishing Stream")
# Adding animals and trees
berry_patch.add_animal(Animal("Bear", "Mama Bear", 10))
berry_patch.add_animal(Animal("Rabbit", "Hoppy", 2))
berry_patch.add_tree(Tree("Berry Bush", 5, 3))
fishing_stream.add_animal(Animal("Bear", "Papa Bear", 12))
fishing_stream.add_animal(Animal("Fish", "Goldie", 1))
fishing_stream.add_tree(Tree("Pine", 50, 30))
# Generating reports
berry_patch.report()
fishing_stream.report()
This project brought together everything Mama Bear had learned. She was now able to model their forest home in code, track the animals and plants, and generate useful reports.
Debugging: When Things Go Grizzly
Of course, not everything was smooth sailing in their coding journey. There were times when their code didn’t work as expected. Papa Bear used these moments to teach Mama Bear about debugging.
“Debugging is like being a detective,” Papa Bear explained. “We look for clues in our code to figure out why it’s not working properly.”
They practiced using print statements to track variable values, learned how to read error messages, and even used the Python debugger for more complex issues.
Mama Bear learned that making mistakes was a natural part of coding, and fixing them was an essential skill to develop.
Version Control: Keeping Track of Changes
As their Forest Management System grew more complex, Papa Bear introduced the concept of version control using Git. He explained it as a way to keep track of all the changes they made to their code, like having a time machine for their project.
They learned basic Git commands:
git init
git add .
git commit -m "Initial commit of Forest Management System"
git branch new-feature
git checkout new-feature
Mama Bear was fascinated by how they could experiment with new features without fear of breaking their main code.
Collaboration: Working with Other Forest Creatures
As word spread about their Forest Management System, other animals became interested in contributing. Papa Bear saw this as an opportunity to teach Mama Bear about collaboration in coding projects.
They set up a GitHub repository for their project and learned about pull requests, code reviews, and merging changes. Mama Bear was excited to see how coding could bring the entire forest community together.
Continuous Learning: The Coding Journey Never Ends
As their coding adventure progressed, Papa Bear reminded Mama Bear that learning to code is a lifelong journey. “Technology is always evolving,” he said, “and there’s always something new to learn.”
They started exploring new programming languages, attended virtual coding meetups with other forest creatures, and even considered contributing to open-source projects.
The Power of Perseverance: Overcoming Coding Challenges
Throughout their journey, there were moments when Mama Bear felt overwhelmed or discouraged. Papa Bear reminded her of the importance of perseverance in coding.
“Remember,” he said, “every expert was once a beginner. The key is to keep trying, keep learning, and never give up.”
Mama Bear took these words to heart, pushing through difficult concepts and celebrating each small victory along the way.
Sharing Knowledge: Mama Bear Becomes a Teacher
As Mama Bear’s skills grew, she found herself able to help other forest animals with their coding questions. She realized that teaching others was not only rewarding but also reinforced her own understanding of coding concepts.
Papa Bear beamed with pride as he watched Mama Bear explain variables to a group of curious squirrels, and debug a rabbit’s carrot-counting program.
The Future: Coding for a Better Forest
With their new skills, Mama and Papa Bear began dreaming up ways to use technology to improve life in the forest. They started working on apps to track berry bush growth, monitor fish populations, and even create a forest-wide communication system.
Their coding journey had not only brought them closer together but had also opened up a world of possibilities for their entire forest community.
Conclusion: The Beary Best Coding Adventure
As we conclude our tale of Papa Bear teaching Mama Bear to code, we’re reminded of the joy, challenges, and growth that come with learning a new skill. Their journey from “Hello, Forest!” to creating a comprehensive Forest Management System mirrors the path many take when learning to code.
Key takeaways from their adventure include:
- Start with the basics and build gradually
- Create a supportive learning environment
- Apply coding concepts to real-world (or forest) problems
- Embrace debugging as part of the learning process
- Collaborate with others and share knowledge
- Persevere through challenges
- Never stop learning and exploring new technologies
Whether you’re a Papa Bear, a Mama Bear, or any other creature in the vast forest of technology, remember that everyone starts somewhere. With patience, perseverance, and a supportive community, you too can embark on your own coding adventure.
So, grab your favorite honey-flavored snack, fire up your computer, and start your coding journey today. Who knows? You might just create something that makes life in your own “forest” a little bit better for everyone.
Happy coding, and may your bugs be few and your compile times be short!