The Matrix of Coding: Your Path to Success
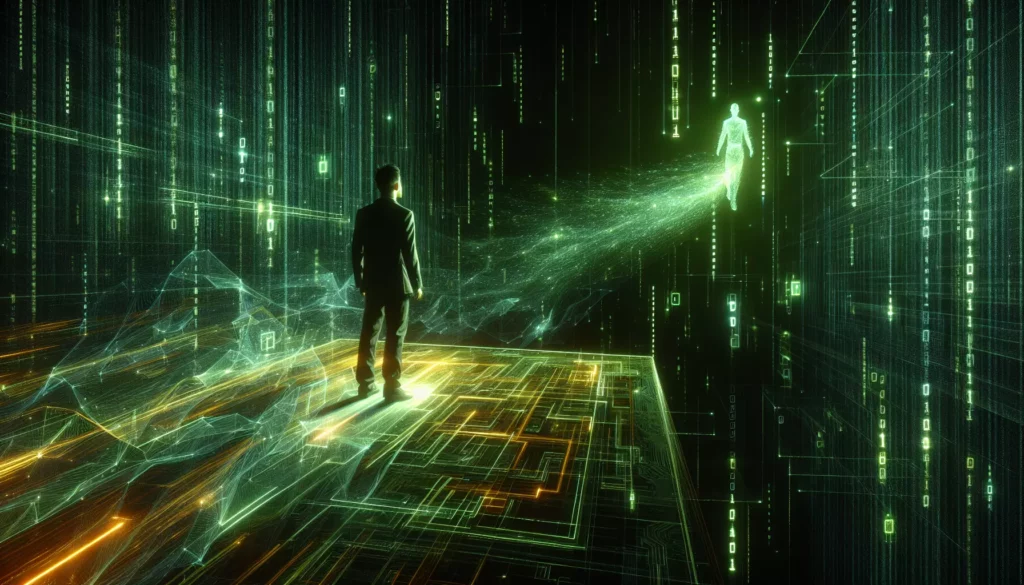
Have you ever dreamed of downloading knowledge directly into your brain, like in the Matrix? The idea of “learning by digital osmosis” is undeniably intriguing. However, as captivating as this concept may be, we all know that’s not how it works in the real world, especially not in the realm of coding. Yet, the journey of learning to code can be just as transformative as Neo’s adventure in the Matrix.
As your guide on this journey – let’s call me Morpheus – I present to you a choice that reflects the essence of your path into programming. This isn’t about hiding textbooks under your pillow and hoping for knowledge to seep in overnight. It’s about the path you choose to walk – a decision that could fundamentally shape your understanding of the digital realm.
The Two Paths: Red Pill vs. Blue Pill
The Red Pill: Traditional Coding Education
The Red Pill represents the traditional route of coding education. If you choose this path, you’ll find yourself immersed in syntax and frameworks, memorizing concepts, and hoping for understanding to seep in through repetition and tutorials. This approach is akin to learning a language by memorizing a dictionary – it’s detailed and thorough, but it might miss the essence of communication.
In the Red Pill world, you might find yourself:
- Memorizing syntax for multiple programming languages
- Following step-by-step tutorials to build specific projects
- Focusing on mastering individual technologies and frameworks
- Spending hours debugging code without fully understanding the underlying principles
While this approach can lead to proficiency in specific languages or frameworks, it may leave you struggling when faced with novel problems or when trying to adapt to new technologies.
The Blue Pill: Algorithmic Thinking and Problem Solving
The Blue Pill path is less about memorization and more about developing your thought process. It’s similar to how a writer learns to weave stories rather than just memorizing vocabulary. On this path, you’ll focus on problem-solving skills and algorithmic thinking – the very backbone of programming logic.
Choosing the Blue Pill means:
- Understanding the structure of programming, not just the syntax
- Focusing on data structures and algorithms – the foundation of problem-solving abilities
- Developing a mindset that can tackle any coding challenge, regardless of the language
- Learning to think like a programmer, not just code like one
This approach prepares you not just for the coding challenges of today, but for the unknown problems of tomorrow.
Programming: An Art and a Science
In the world of code, success isn’t just about knowing the most languages or memorizing the most functions. It’s about understanding the art and science of programming. Your creativity, paired with logic, will define your success in this field.
Companies aren’t just looking for coders who can recite syntax. They’re seeking individuals who can think critically, solve complex problems, and bring innovative solutions to the table. This is where the Blue Pill approach shines – it equips you with the mental tools to tackle any coding challenge, regardless of the specific language or framework.
The Choice: Shaping Your Digital Future
So, which pill will you choose? Will you walk the path of the Red Pill, mastering the languages and tools of the digital world? Or will you opt for the Blue Pill, focusing on the structure and logic that underpin all coding languages?
Remember, the decision you make today is not just about learning to code. It’s about how you wish to understand and interact with the ever-evolving digital world. This choice shapes not just how you learn, but how you think and solve the puzzles of the future.
The AlgoCademy Approach: Blending the Best of Both Worlds
While the choice between Red and Blue pills presents a stark contrast, the reality of effective coding education often lies in a balanced approach. This is where platforms like AlgoCademy come into play, offering a unique blend of practical coding skills and fundamental problem-solving abilities.
Interactive Learning: Beyond Passive Absorption
AlgoCademy recognizes that true learning comes from active engagement, not passive absorption. Their interactive coding tutorials go beyond simple “copy and paste” exercises, challenging you to think critically about each line of code you write. This approach helps bridge the gap between theoretical knowledge and practical application.
Focus on Algorithmic Thinking
At the core of AlgoCademy’s philosophy is the emphasis on algorithmic thinking. This aligns closely with the Blue Pill approach, focusing on the underlying principles that make great programmers. By understanding algorithms and data structures, you’re equipped to solve a wide array of problems, regardless of the specific programming language.
Here’s a simple example of how algorithmic thinking can be applied to a common programming problem:
def find_maximum(numbers):
if not numbers: # Check if the list is empty
return None
max_number = numbers[0] # Assume the first number is the maximum
for number in numbers[1:]: # Iterate through the rest of the list
if number > max_number:
max_number = number # Update max_number if we find a larger number
return max_number
# Example usage
numbers_list = [3, 7, 2, 9, 1, 5]
result = find_maximum(numbers_list)
print(f"The maximum number is: {result}")
This simple algorithm demonstrates the process of finding the maximum number in a list. The key here isn’t the specific Python syntax, but the logical process of comparing each number and keeping track of the largest one found so far.
AI-Powered Assistance: Personalized Learning
One of AlgoCademy’s standout features is its AI-powered assistance. This technology adapts to your learning style and pace, providing personalized guidance as you progress through coding challenges. It’s like having a mentor who understands both the Red and Blue pill approaches, guiding you towards a comprehensive understanding of programming.
Preparing for Real-World Challenges
AlgoCademy doesn’t just teach you to code; it prepares you for the challenges you’ll face in the tech industry. With a focus on interview preparation, especially for major tech companies (often referred to as FAANG – Facebook, Amazon, Apple, Netflix, Google), the platform ensures you’re ready to demonstrate both your coding skills and your problem-solving abilities.
The Journey from Beginner to Problem Solver
Learning to code is a journey, and like Neo in the Matrix, you’ll go through various stages of awakening and realization. Here’s how your journey might unfold:
1. The Awakening: Understanding the Basics
In this initial stage, you’ll learn the fundamental concepts of programming. This includes basic syntax, data types, and simple control structures. It’s like Neo first becoming aware that there’s more to the world than he initially thought.
# Basic Python example
print("Hello, World!")
name = input("What's your name? ")
print(f"Welcome to the world of coding, {name}!")
2. The Training: Building Your Skills
As you progress, you’ll start tackling more complex problems and learning about functions, objects, and basic data structures. This is akin to Neo’s training sessions in the Matrix, where he begins to understand the rules of his new reality.
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n-1)
result = factorial(5)
print(f"The factorial of 5 is: {result}")
3. The Realization: Grasping Algorithmic Thinking
This is where the Blue Pill approach really starts to shine. You’ll begin to see patterns in problems and understand how to approach them systematically. It’s like Neo starting to see the code that makes up the Matrix.
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
unsorted_list = [64, 34, 25, 12, 22, 11, 90]
sorted_list = bubble_sort(unsorted_list)
print(f"Sorted list: {sorted_list}")
4. The Mastery: Solving Complex Problems
At this stage, you’re not just writing code; you’re crafting solutions. You can break down complex problems into manageable parts and implement efficient solutions. This is Neo at his most powerful, bending the rules of the Matrix to his will.
class Graph:
def __init__(self, vertices):
self.V = vertices
self.graph = []
def add_edge(self, u, v, w):
self.graph.append([u, v, w])
def find(self, parent, i):
if parent[i] == i:
return i
return self.find(parent, parent[i])
def union(self, parent, rank, x, y):
xroot = self.find(parent, x)
yroot = self.find(parent, y)
if rank[xroot] < rank[yroot]:
parent[xroot] = yroot
elif rank[xroot] > rank[yroot]:
parent[yroot] = xroot
else:
parent[yroot] = xroot
rank[xroot] += 1
def kruskal_mst(self):
result = []
i, e = 0, 0
self.graph = sorted(self.graph, key=lambda item: item[2])
parent = []
rank = []
for node in range(self.V):
parent.append(node)
rank.append(0)
while e < self.V - 1:
u, v, w = self.graph[i]
i = i + 1
x = self.find(parent, u)
y = self.find(parent, v)
if x != y:
e = e + 1
result.append([u, v, w])
self.union(parent, rank, x, y)
return result
# Example usage
g = Graph(4)
g.add_edge(0, 1, 10)
g.add_edge(0, 2, 6)
g.add_edge(0, 3, 5)
g.add_edge(1, 3, 15)
g.add_edge(2, 3, 4)
mst = g.kruskal_mst()
print("Edges in the constructed MST:")
for u, v, weight in mst:
print(f"{u} -- {v} == {weight}")
This implementation of Kruskal’s algorithm for finding a Minimum Spanning Tree in a graph demonstrates advanced problem-solving skills and understanding of complex data structures.
Embracing Your Neo Journey
As you embark on your coding journey, remember that it’s not just about accumulating knowledge, but about transforming how you think and solve problems. Whether you lean towards the Red Pill’s focus on specific technologies or the Blue Pill’s emphasis on underlying principles, platforms like AlgoCademy offer a balanced approach to help you become a true problem solver in the world of programming.
Are you ready to take the leap and start your journey to becoming a coding maestro? Remember, all I’m offering is the truth about learning to code – nothing more. The choice, as always, is yours.
Embrace your inner Neo, challenge yourself, and step into the world of algorithmic thinking and problem-solving. Your journey to becoming a skilled programmer starts now!
Embrace Your Neo Journey and Become a Problem Solver with AlgoCademy Today!