The Importance of Time Complexity Analysis in Software Development
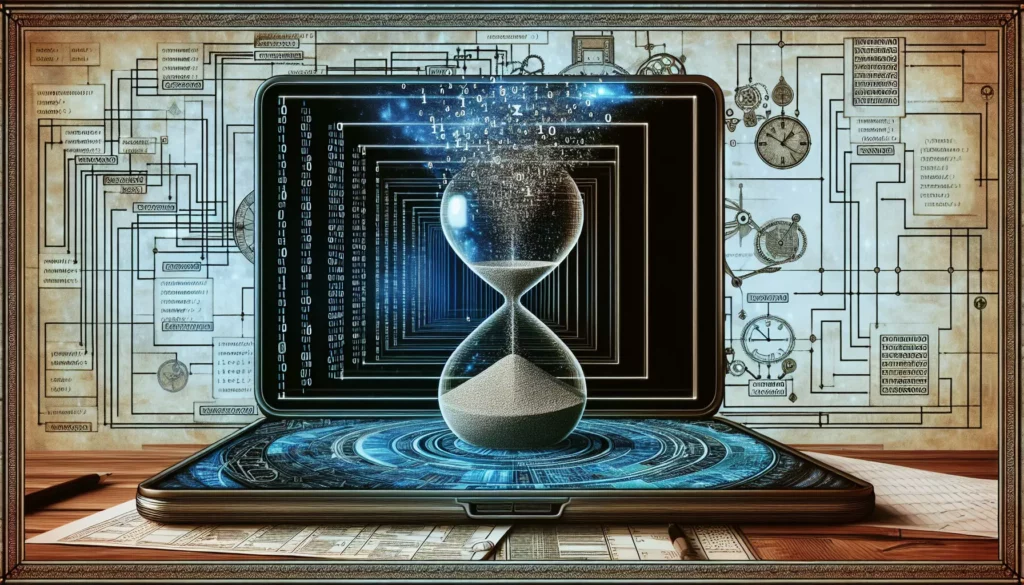
In the fast-paced world of software development, efficiency is key. As developers, we’re constantly striving to create solutions that not only work but work well. One of the most critical aspects of creating efficient software is understanding and optimizing time complexity. But why is time complexity analysis so important, and how can it impact your development process? Let’s dive deep into this crucial concept and explore its significance in real-world scenarios.
What is Time Complexity?
Before we delve into the importance of time complexity analysis, let’s briefly define what time complexity is. Time complexity is a measure of how the runtime of an algorithm increases with respect to the input size. It’s typically expressed using Big O notation, which provides an upper bound on the growth rate of an algorithm’s execution time.
For example:
- O(1) represents constant time complexity
- O(log n) represents logarithmic time complexity
- O(n) represents linear time complexity
- O(n log n) represents linearithmic time complexity
- O(n^2) represents quadratic time complexity
- O(2^n) represents exponential time complexity
The Fallacy of “It Works Fine on My Machine”
One common misconception among developers, especially those new to the field, is the belief that if code runs well and fast on unit tests, time complexity analysis isn’t necessary. This line of thinking often leads to the question: “Why would you care when you can see that the code runs well and fast on the unit tests?”
While it’s true that unit tests are an essential part of the development process, they often fall short in predicting real-world performance. Here’s why:
1. Unit Tests May Not Be Representative
Firstly, are those unit tests truly representative of all the input you’ll be getting in the real world? In many cases, the answer is no. Unit tests are typically designed to cover specific scenarios and edge cases, but they may not account for the full range of inputs your code will encounter in production.
For instance, you might have small tests that run quickly with limited data sets. While most real-world usage might generate similarly small data, there will inevitably be cases where your code encounters much larger inputs. In these situations, inefficient code that performed well on small test cases can suddenly become a significant bottleneck.
2. The Hidden Cost of Inefficiency
When inefficient code makes it to production, the consequences can be severe. You might find yourself spending hours or even days trying to identify and fix issues that only manifest with large-scale data. This not only wastes valuable development time but can also lead to frustrated users and potential revenue loss if the performance issues impact critical systems.
The Predictive Power of Time Complexity Analysis
One of the most compelling reasons to learn and apply time complexity analysis is its predictive power. Understanding the time complexity of an algorithm allows you to make informed decisions about your code’s performance before you even start writing it.
Choosing the Right Algorithm
Consider a scenario where you have an array of 1 million items, and you’re contemplating two different algorithms to process this data. One algorithm has a time complexity of O(n^2), while the other has a time complexity of O(n). Both algorithms might be time-consuming to implement and debug, so which one should you choose?
If you understand time complexity, the choice becomes clear:
- The O(n^2) algorithm would require approximately 1,000,000,000,000 (1 trillion) operations in the worst case.
- The O(n) algorithm would require approximately 1,000,000 (1 million) operations in the worst case.
Even on modern hardware, the O(n^2) algorithm could take tens of minutes to complete, while the O(n) algorithm might finish in under a second or even instantly on faster systems and with optimized code.
Avoiding Costly Mistakes
Without knowledge of time complexity, you might be tempted to implement the first algorithm that comes to mind, only to realize later that it’s not suitable for the task at hand. This can result in wasted development time and the need to rewrite significant portions of your code.
By understanding time complexity, you can estimate how an algorithm will perform with large inputs before investing time in implementation. This foresight can save you hours of work and prevent the frustration of having to scrap and rewrite code due to performance issues.
Real-World Impact of Time Complexity
The importance of time complexity analysis extends far beyond theoretical discussions. It has tangible impacts on real-world applications and user experiences. Let’s explore some scenarios where time complexity can make a significant difference:
1. Web Applications and API Response Times
In web development, response time is crucial for user satisfaction. Consider a social media application that needs to display a user’s feed. An inefficient algorithm for sorting and filtering posts could lead to slow page loads, especially for users with many connections or a long history on the platform.
For example, if the feed generation algorithm has a time complexity of O(n^2) where n is the number of posts to consider, it might work fine for users with a few hundred posts. However, for power users with thousands of posts, the page load time could become unacceptably long.
By optimizing the algorithm to O(n log n) or better, you can ensure that the application remains responsive even for users with large amounts of data.
2. Data Processing in Business Intelligence
Business intelligence (BI) tools often deal with massive datasets. An inefficient algorithm in a BI tool could mean the difference between getting insights in seconds versus waiting hours for a report to generate.
For instance, if a BI tool uses an O(n^2) algorithm to find correlations between different data points, it might work fine for small businesses with limited data. However, for large enterprises with millions of data points, this could result in reports taking days to generate, making the tool practically unusable.
By implementing more efficient algorithms with better time complexity, such as using advanced data structures or parallel processing techniques, the same tool could provide real-time insights even with massive datasets.
3. Mobile Applications and Battery Life
Time complexity doesn’t just affect speed; it can also impact resource usage. In mobile development, an inefficient algorithm can lead to increased CPU usage, which in turn affects battery life.
Consider a music playlist shuffling feature. An O(n^2) shuffling algorithm might not noticeably affect performance for small playlists, but for users with thousands of songs, it could cause the app to consume significant battery power every time they shuffle their library.
By implementing a more efficient O(n) shuffling algorithm, like the Fisher-Yates shuffle, you can ensure that the feature remains fast and energy-efficient regardless of the playlist size.
Common Time Complexities and Their Implications
Understanding different time complexities and their practical implications can help you make better decisions when designing and implementing algorithms. Let’s look at some common time complexities and what they mean in practice:
O(1) – Constant Time
Algorithms with O(1) time complexity perform the same number of operations regardless of the input size. These are typically the most efficient algorithms.
Example: Accessing an array element by its index.
function getElement(array, index) {
return array[index];
}
Implication: These operations are extremely fast and scale well to any input size.
O(log n) – Logarithmic Time
Logarithmic time complexity is often seen in algorithms that divide the problem in half at each step.
Example: Binary search in a sorted array.
function binarySearch(array, target) {
let left = 0;
let right = array.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (array[mid] === target) return mid;
if (array[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
Implication: These algorithms are very efficient and can handle large inputs well. They’re often used in search and divide-and-conquer algorithms.
O(n) – Linear Time
Linear time algorithms perform operations proportional to the input size.
Example: Finding the maximum element in an unsorted array.
function findMax(array) {
let max = array[0];
for (let i = 1; i < array.length; i++) {
if (array[i] > max) max = array[i];
}
return max;
}
Implication: These algorithms scale linearly with input size. They’re generally considered efficient for most purposes.
O(n log n) – Linearithmic Time
This time complexity is often seen in efficient sorting algorithms.
Example: Merge sort algorithm.
function mergeSort(array) {
if (array.length <= 1) return array;
const mid = Math.floor(array.length / 2);
const left = mergeSort(array.slice(0, mid));
const right = mergeSort(array.slice(mid));
return merge(left, right);
}
function merge(left, right) {
let result = [];
let leftIndex = 0;
let rightIndex = 0;
while (leftIndex < left.length && rightIndex < right.length) {
if (left[leftIndex] < right[rightIndex]) {
result.push(left[leftIndex]);
leftIndex++;
} else {
result.push(right[rightIndex]);
rightIndex++;
}
}
return result.concat(left.slice(leftIndex)).concat(right.slice(rightIndex));
}
Implication: These algorithms are efficient for large datasets and are often the best choice for sorting when memory is not a constraint.
O(n^2) – Quadratic Time
Quadratic time algorithms have nested iterations over the input.
Example: Bubble sort algorithm.
function bubbleSort(array) {
for (let i = 0; i < array.length; i++) {
for (let j = 0; j < array.length - i - 1; j++) {
if (array[j] > array[j + 1]) {
// Swap elements
let temp = array[j];
array[j] = array[j + 1];
array[j + 1] = temp;
}
}
}
return array;
}
Implication: These algorithms can become very slow with large inputs. They’re generally avoided for large datasets but can be simple to implement for small inputs.
O(2^n) – Exponential Time
Exponential time algorithms have a runtime that doubles with each addition to the input.
Example: Recursive calculation of Fibonacci numbers (naive approach).
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
Implication: These algorithms are generally impractical for all but very small inputs. They’re often seen in naive solutions to complex problems and usually require optimization or a different approach for practical use.
Strategies for Improving Time Complexity
Understanding time complexity is the first step. The next is knowing how to improve it. Here are some strategies you can use to optimize your algorithms:
1. Use Appropriate Data Structures
Choosing the right data structure can dramatically improve the time complexity of your algorithms. For example:
- Use a hash table (object in JavaScript) for O(1) average case lookup, insertion, and deletion.
- Use a binary search tree for O(log n) search, insertion, and deletion in sorted data.
- Use a heap for O(1) access to the minimum or maximum element and O(log n) insertion and deletion.
2. Avoid Nested Loops When Possible
Nested loops often lead to O(n^2) time complexity. Look for ways to accomplish the same task with a single loop or by using more efficient data structures.
3. Use Divide and Conquer Algorithms
Divide and conquer algorithms, like merge sort or quicksort, can often achieve O(n log n) time complexity, which is much better than O(n^2) for large inputs.
4. Memoization and Dynamic Programming
For problems with overlapping subproblems, use memoization or dynamic programming to avoid redundant calculations. This can often reduce exponential time complexity to polynomial time.
5. Preprocessing and Caching
Sometimes, you can preprocess data or cache results to improve the time complexity of subsequent operations. This is especially useful in scenarios where the same computation is performed repeatedly.
Tools and Techniques for Analyzing Time Complexity
While understanding time complexity conceptually is crucial, there are also practical tools and techniques you can use to analyze and optimize your code:
1. Profiling Tools
Most programming languages have profiling tools that can help you identify performance bottlenecks in your code. For example:
- JavaScript: Chrome DevTools Performance tab
- Python: cProfile module
- Java: JProfiler or VisualVM
2. Big O Analysis
Practice analyzing your algorithms manually to determine their Big O complexity. This involves identifying the dominant terms in your algorithm’s runtime as the input size grows.
3. Benchmarking
Create benchmarks that test your algorithm with various input sizes. This can help you verify your theoretical analysis and catch any unexpected performance issues.
4. Code Review
Regular code reviews with a focus on performance can help catch inefficient algorithms before they make it to production. Encourage team members to discuss and analyze time complexity during these reviews.
Conclusion: The Long-Term Benefits of Time Complexity Analysis
In the world of software development, time complexity analysis is not just an academic exercise—it’s a crucial skill that can significantly impact the quality and efficiency of your code. By understanding and optimizing time complexity, you can:
- Create more scalable and efficient applications
- Predict and prevent performance issues before they occur
- Make informed decisions about algorithm and data structure choices
- Improve user experience by reducing response times and resource usage
- Save development time by avoiding the need to refactor inefficient code
While it may require an initial investment of time to learn and apply time complexity analysis, the long-term benefits far outweigh the costs. As you develop this skill, you’ll find yourself writing better code from the outset, avoiding performance pitfalls, and delivering more robust and scalable solutions.
Remember, in the fast-paced world of technology, efficiency is not just a nice-to-have—it’s often the difference between a successful application and one that fails to meet user expectations. By mastering time complexity analysis, you’re equipping yourself with a powerful tool that will serve you well throughout your career as a developer.
If you’re looking to improve your skills in time complexity analysis and algorithmic thinking, consider exploring the lessons available on AlgoCademy. With interactive tutorials, AI-powered assistance, and a focus on practical coding skills, it’s an excellent resource for developers looking to take their abilities to the next level.
In the end, understanding time complexity is about more than just writing faster code—it’s about becoming a more thoughtful, efficient, and effective developer. So embrace the challenge, start analyzing your algorithms, and watch as your code—and your skills—reach new heights of performance and reliability.