Instacart Technical Interview Prep: A Comprehensive Guide
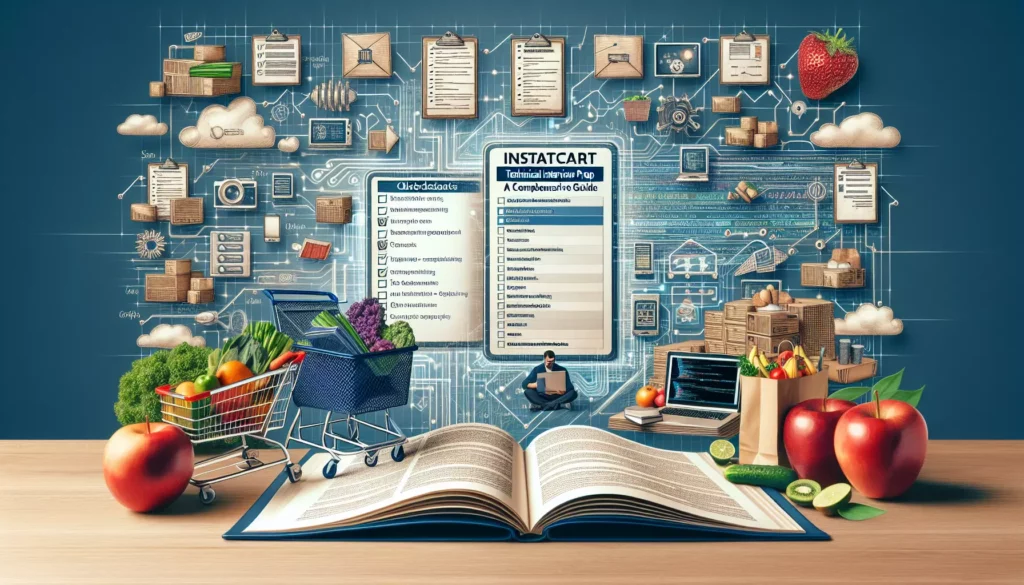
Are you gearing up for a technical interview at Instacart? You’re in the right place! In this comprehensive guide, we’ll walk you through everything you need to know to ace your Instacart technical interview. From understanding the interview process to mastering key algorithms and data structures, we’ve got you covered. Let’s dive in and prepare you for success!
Table of Contents
- Understanding Instacart
- The Instacart Interview Process
- Key Topics to Master
- Preferred Coding Languages
- Practice Problems and Resources
- System Design for Instacart
- Behavioral Questions and Soft Skills
- Tips and Tricks for Interview Success
- Post-Interview Steps
- Conclusion
1. Understanding Instacart
Before diving into the technical aspects of your interview prep, it’s crucial to understand what Instacart does and the technical challenges they face. Instacart is a leading online grocery delivery and pick-up service that connects customers with personal shoppers who hand-pick and deliver items from local stores.
Key aspects of Instacart’s technology include:
- Large-scale distributed systems
- Real-time inventory management
- Efficient routing and logistics algorithms
- Machine learning for demand forecasting and personalization
- Mobile app development for both customers and shoppers
Understanding these aspects will help you align your preparation with Instacart’s technical needs and demonstrate your enthusiasm for the company during the interview.
2. The Instacart Interview Process
The Instacart technical interview process typically consists of several stages:
- Initial Phone Screen: A brief call with a recruiter to discuss your background and interest in Instacart.
- Technical Phone Interview: A 45-60 minute interview with an engineer, focusing on coding and problem-solving skills.
- Take-Home Assignment: Some positions may require a take-home coding project to assess your practical skills.
- On-Site Interviews: A series of interviews (often virtual) including:
- 2-3 coding interviews
- 1 system design interview
- 1-2 behavioral interviews
Each stage is designed to evaluate different aspects of your technical skills, problem-solving abilities, and cultural fit within the company.
3. Key Topics to Master
To excel in your Instacart technical interview, focus on mastering these key topics:
Data Structures
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees (Binary Trees, Binary Search Trees)
- Graphs
- Hash Tables
- Heaps
Algorithms
- Sorting (Quick Sort, Merge Sort, Heap Sort)
- Searching (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Graph Algorithms (Shortest Path, Minimum Spanning Tree)
Specific Areas Relevant to Instacart
- Optimization Algorithms (for routing and logistics)
- Caching Strategies
- Distributed Systems Concepts
- Database Design and Query Optimization
- RESTful API Design
Let’s dive deeper into a few of these topics with code examples:
Binary Search Implementation
Binary search is a fundamental algorithm that Instacart might use for efficient lookups in sorted data structures. Here’s a Python implementation:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15]
target = 7
result = binary_search(sorted_array, target)
print(f"Target {target} found at index: {result}")
Graph Representation for Routing
Instacart’s routing algorithms likely use graph structures. Here’s a simple graph representation using an adjacency list in Python:
from collections import defaultdict
class Graph:
def __init__(self):
self.graph = defaultdict(list)
def add_edge(self, u, v):
self.graph[u].append(v)
def print_graph(self):
for vertex in self.graph:
print(f"{vertex}: {' -> '.join(map(str, self.graph[vertex]))}")
# Example usage
g = Graph()
g.add_edge(0, 1)
g.add_edge(0, 2)
g.add_edge(1, 2)
g.add_edge(2, 0)
g.add_edge(2, 3)
g.add_edge(3, 3)
print("Graph representation:")
g.print_graph()
4. Preferred Coding Languages
While Instacart doesn’t strictly require a specific programming language for their interviews, it’s best to use a language you’re most comfortable with. However, some popular choices among Instacart engineers include:
- Python
- Ruby
- JavaScript
- Java
- Go
Python is often a great choice due to its readability and extensive libraries for data manipulation and algorithms. Here’s a quick example of how you might implement a simple cache in Python, which could be relevant to Instacart’s systems:
from collections import OrderedDict
class LRUCache:
def __init__(self, capacity):
self.cache = OrderedDict()
self.capacity = capacity
def get(self, key):
if key not in self.cache:
return -1
self.cache.move_to_end(key)
return self.cache[key]
def put(self, key, value):
if key in self.cache:
self.cache.move_to_end(key)
self.cache[key] = value
if len(self.cache) > self.capacity:
self.cache.popitem(last=False)
# Example usage
cache = LRUCache(2)
cache.put(1, 1)
cache.put(2, 2)
print(cache.get(1)) # returns 1
cache.put(3, 3) # evicts key 2
print(cache.get(2)) # returns -1 (not found)
cache.put(4, 4) # evicts key 1
print(cache.get(1)) # returns -1 (not found)
print(cache.get(3)) # returns 3
print(cache.get(4)) # returns 4
5. Practice Problems and Resources
To prepare effectively for your Instacart technical interview, make use of these resources:
- LeetCode: Focus on medium to hard problems in areas like arrays, strings, trees, and graphs.
- HackerRank: Offers a good mix of algorithmic challenges and data structure problems.
- AlgoExpert: Provides curated lists of problems often asked in tech interviews.
- Cracking the Coding Interview: A classic book with problems and solutions for tech interviews.
- System Design Primer: An open-source resource for learning about system design concepts.
Here’s a sample problem that might be relevant to Instacart’s domain:
Optimizing Delivery Routes
Problem: Given a list of delivery locations and their coordinates, find the shortest path that visits all locations exactly once and returns to the starting point (also known as the Traveling Salesman Problem).
While this is an NP-hard problem, you can implement a simple approximation algorithm like the nearest neighbor approach:
import math
def distance(point1, point2):
return math.sqrt((point1[0] - point2[0])**2 + (point1[1] - point2[1])**2)
def nearest_neighbor_tsp(points):
unvisited = points[1:]
path = [points[0]]
while unvisited:
last = path[-1]
next_point = min(unvisited, key=lambda x: distance(last, x))
path.append(next_point)
unvisited.remove(next_point)
path.append(points[0]) # Return to start
return path
# Example usage
delivery_points = [(0, 0), (1, 5), (2, 2), (3, 3), (5, 1)]
optimal_route = nearest_neighbor_tsp(delivery_points)
print("Optimal delivery route:", optimal_route)
This solution provides a simple approximation for route optimization, which is a key aspect of Instacart’s logistics operations.
6. System Design for Instacart
System design is a crucial part of the Instacart interview process, especially for more senior positions. You should be prepared to design large-scale distributed systems that can handle Instacart’s specific challenges. Key areas to focus on include:
- Scalability: How to handle millions of users and orders
- Reliability: Ensuring the system remains operational during peak times
- Real-time updates: Managing inventory and order status in real-time
- Data consistency: Maintaining accurate data across distributed systems
- Performance optimization: Reducing latency for a smooth user experience
Here’s a high-level example of how you might approach designing a part of Instacart’s system:
Designing a Real-time Inventory Management System
- Requirements:
- Real-time updates of inventory across multiple stores
- High availability and low latency
- Consistency across distributed systems
- Ability to handle high traffic during peak hours
- High-level Architecture:
- Use a microservices architecture for scalability and maintainability
- Implement a message queue (e.g., Apache Kafka) for real-time updates
- Use a distributed cache (e.g., Redis) for fast read operations
- Implement a database with strong consistency (e.g., PostgreSQL with replication)
- Data Flow:
- Store updates inventory in real-time
- Update is sent to message queue
- Inventory service consumes message and updates database
- Cache is invalidated or updated
- Clients receive real-time updates through WebSocket connections
- Scaling Considerations:
- Horizontal scaling of services behind a load balancer
- Database sharding based on store or product category
- CDN for static assets to reduce load on main servers
Being able to articulate such a design and discuss its trade-offs will greatly impress your interviewers at Instacart.
7. Behavioral Questions and Soft Skills
While technical skills are crucial, Instacart also values soft skills and cultural fit. Be prepared to answer behavioral questions that demonstrate your problem-solving abilities, teamwork, and alignment with Instacart’s values. Some example questions might include:
- Tell me about a time when you had to deal with a particularly challenging technical problem. How did you approach it?
- How do you stay updated with the latest technologies and industry trends?
- Describe a situation where you had to work with a difficult team member. How did you handle it?
- Can you share an experience where you had to make a decision with incomplete information?
- How do you prioritize tasks when working on multiple projects simultaneously?
When answering these questions, use the STAR method (Situation, Task, Action, Result) to structure your responses effectively.
8. Tips and Tricks for Interview Success
- Think out loud: Communicate your thought process clearly during coding and system design questions.
- Ask clarifying questions: Ensure you fully understand the problem before diving into a solution.
- Consider edge cases: Demonstrate thoroughness by addressing potential edge cases in your solutions.
- Optimize your solutions: After implementing a working solution, discuss potential optimizations.
- Practice whiteboarding: Even for virtual interviews, practice explaining your code as if you were writing it on a whiteboard.
- Research Instacart: Show genuine interest by understanding Instacart’s business model and technical challenges.
- Prepare questions: Have thoughtful questions ready for your interviewers about the role and the company.
9. Post-Interview Steps
After your interview:
- Send a thank-you note: Express your appreciation for the interviewer’s time and reiterate your interest in the position.
- Reflect on the experience: Consider what went well and areas for improvement in future interviews.
- Follow up: If you haven’t heard back within the expected timeframe, politely follow up with your recruiter.
- Continue learning: Regardless of the outcome, use the experience to identify areas where you can further improve your skills.
10. Conclusion
Preparing for an Instacart technical interview requires a combination of strong coding skills, system design knowledge, and an understanding of the company’s specific challenges. By focusing on the key topics we’ve covered, practicing regularly, and demonstrating your problem-solving abilities, you’ll be well-prepared to showcase your skills and land that dream job at Instacart.
Remember, the key to success is not just about having the right answers, but also about demonstrating your thought process, ability to learn, and passion for tackling complex problems. With thorough preparation and the right mindset, you’ll be well on your way to joining the innovative team at Instacart.
Good luck with your interview preparation, and may your coding skills be as fresh as Instacart’s groceries!