Rivian Technical Interview Prep: A Comprehensive Guide for Success
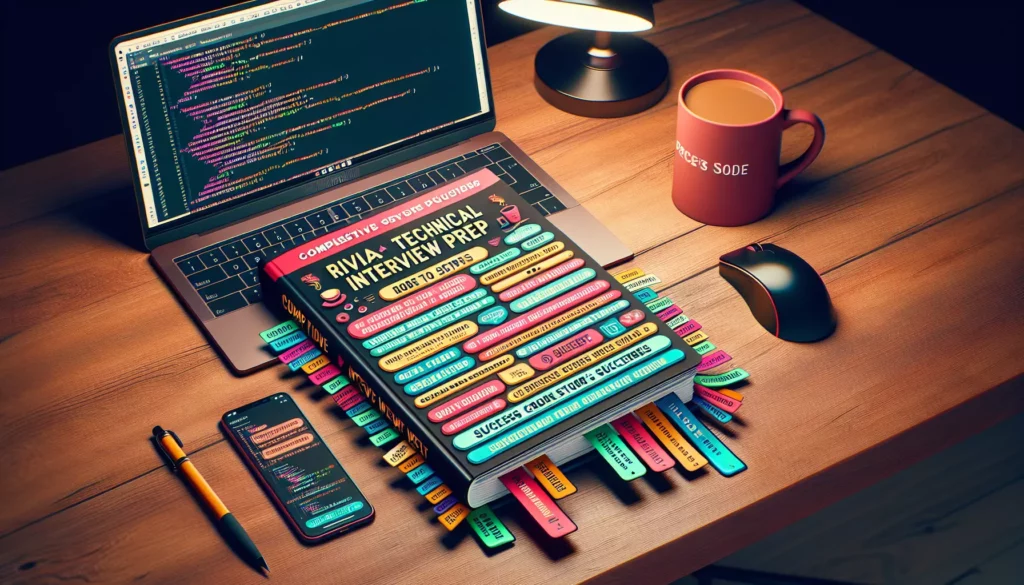
Are you gearing up for a technical interview at Rivian, the innovative electric vehicle manufacturer? You’re in the right place! This comprehensive guide will walk you through everything you need to know to ace your Rivian technical interview. From understanding the company’s unique focus to mastering the essential coding skills, we’ve got you covered.
Table of Contents
- Understanding Rivian: More Than Just Cars
- The Rivian Technical Interview Process
- Core Skills to Master
- Coding Languages to Focus On
- Essential Data Structures
- Key Algorithms to Know
- System Design Concepts
- EV-Specific Knowledge
- Practice Resources and Mock Interviews
- Interview Day Tips and Strategies
- Conclusion: Driving Towards Success
1. Understanding Rivian: More Than Just Cars
Before diving into the technical aspects, it’s crucial to understand what makes Rivian unique in the automotive and tech landscape. Rivian is not just another car company; it’s a technology-driven enterprise focused on sustainable transportation solutions.
Key points to remember about Rivian:
- Founded in 2009, Rivian has quickly become a leader in electric vehicle (EV) technology.
- The company focuses on adventure vehicles, including electric trucks and SUVs.
- Rivian places a strong emphasis on software and technology integration in their vehicles.
- They’re committed to sustainability, both in their products and manufacturing processes.
Understanding these aspects will help you align your responses and showcase your enthusiasm for Rivian’s mission during the interview.
2. The Rivian Technical Interview Process
The Rivian technical interview process typically consists of several stages:
- Initial Screening: Usually a phone or video call with a recruiter to discuss your background and interest in Rivian.
- Technical Phone Screen: A 45-60 minute interview with a Rivian engineer, focusing on your technical skills and problem-solving abilities.
- Coding Assignment: Some positions may require a take-home coding assignment to assess your practical skills.
- On-site Interviews: A series of interviews (currently often conducted virtually) including:
- Coding interviews
- System design discussions
- Behavioral interviews
- Final Review: The interview team evaluates your performance and makes a hiring decision.
Each stage is designed to assess different aspects of your skills and fit for the role at Rivian.
3. Core Skills to Master
To excel in a Rivian technical interview, you should be proficient in the following core skills:
- Problem-solving: The ability to break down complex problems into manageable parts.
- Data Structures and Algorithms: A solid understanding of fundamental CS concepts.
- Coding Proficiency: The ability to write clean, efficient, and readable code.
- System Design: Understanding how to design scalable and efficient systems.
- Version Control: Familiarity with Git and collaborative development practices.
- Testing and Debugging: Knowledge of testing methodologies and debugging techniques.
- Automotive Software: Familiarity with automotive software systems is a plus.
Focus on honing these skills through practice and study as you prepare for your interview.
4. Coding Languages to Focus On
While Rivian uses a variety of programming languages, some are more prevalent than others. Focus on mastering these languages:
- C++: Widely used in automotive software for its performance and low-level control.
- Python: Used for data analysis, scripting, and rapid prototyping.
- Java: Common in Android development for in-vehicle infotainment systems.
- JavaScript: Used for web-based interfaces and some in-vehicle applications.
Here’s a simple example of a C++ function that might be relevant in an automotive context:
#include <iostream>
class Battery {
private:
double charge;
const double maxCharge = 100.0;
public:
Battery() : charge(0.0) {}
void chargeBattery(double amount) {
if (charge + amount > maxCharge) {
charge = maxCharge;
} else {
charge += amount;
}
}
double getChargeLevel() const {
return charge;
}
};
int main() {
Battery evBattery;
evBattery.chargeB attery(75.5);
std::cout << "Battery charge level: " << evBattery.getChargeLevel() << "%\n";
return 0;
}
This code demonstrates a simple Battery class with methods to charge the battery and get the current charge level, which could be part of a larger electric vehicle system.
5. Essential Data Structures
A strong grasp of data structures is crucial for any technical interview. Make sure you’re comfortable with the following:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees (Binary Trees, Binary Search Trees)
- Graphs
- Hash Tables
- Heaps
Here’s an example of implementing a simple binary tree node in Python:
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def insert(root, value):
if root is None:
return TreeNode(value)
if value < root.value:
root.left = insert(root.left, value)
else:
root.right = insert(root.right, value)
return root
# Example usage
root = None
values = [5, 3, 7, 1, 9]
for value in values:
root = insert(root, value)
This code creates a binary search tree and provides a method to insert new values, which could be useful in various applications, including data management in vehicle systems.
6. Key Algorithms to Know
Familiarize yourself with these fundamental algorithms:
- Sorting: Quick Sort, Merge Sort, Heap Sort
- Searching: Binary Search, Depth-First Search (DFS), Breadth-First Search (BFS)
- Dynamic Programming
- Graph Algorithms: Dijkstra’s, A*
- String Manipulation
Here’s an example of implementing a binary search algorithm in Java:
public class BinarySearch {
public static int binarySearch(int[] arr, int target) {
int left = 0;
int right = arr.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Target not found
}
public static void main(String[] args) {
int[] sortedArray = {1, 3, 5, 7, 9, 11, 13, 15};
int target = 7;
int result = binarySearch(sortedArray, target);
System.out.println(result != -1 ? "Element found at index " + result : "Element not found");
}
}
This binary search implementation could be useful in various scenarios, such as quickly locating specific data in a sorted dataset within a vehicle’s system.
7. System Design Concepts
For more senior positions, you may be asked about system design. Key areas to focus on include:
- Scalability: How to design systems that can handle increasing loads.
- Reliability: Ensuring systems are fault-tolerant and can recover from failures.
- Performance: Optimizing systems for speed and efficiency.
- Security: Protecting systems and data from unauthorized access and attacks.
- Data Management: Efficient storage, retrieval, and processing of large datasets.
Consider how these concepts might apply to Rivian’s products. For example, how would you design a scalable system for managing over-the-air updates for a fleet of electric vehicles?
8. EV-Specific Knowledge
While not always required, having some knowledge of EV-specific concepts can give you an edge:
- Battery Management Systems (BMS)
- Electric Motor Control
- Charging Systems and Protocols
- Autonomous Driving Technologies
- Vehicle-to-Grid (V2G) Systems
Here’s a simple example of a function that might be used in a battery management system:
def estimate_range(battery_charge, energy_consumption_rate):
"""
Estimates the remaining range of an electric vehicle.
:param battery_charge: Current battery charge in kWh
:param energy_consumption_rate: Energy consumption rate in kWh/mile
:return: Estimated range in miles
"""
if energy_consumption_rate == 0:
return float('inf') # Avoid division by zero
estimated_range = battery_charge / energy_consumption_rate
return round(estimated_range, 2)
# Example usage
current_charge = 75 # kWh
consumption_rate = 0.3 # kWh/mile
range_estimate = estimate_range(current_charge, consumption_rate)
print(f"Estimated range: {range_estimate} miles")
This function demonstrates a basic calculation that might be part of a larger battery management system in an electric vehicle.
9. Practice Resources and Mock Interviews
To prepare effectively, make use of these resources:
- LeetCode: Practice coding problems similar to those you might encounter in the interview.
- HackerRank: Another platform with a wide range of coding challenges.
- System Design Primer: A GitHub repository with comprehensive system design resources.
- Mock Interviews: Practice with peers or use platforms like Pramp for realistic interview simulations.
- Rivian’s Tech Blog: Stay updated on the company’s latest technological developments.
Regular practice with these resources will help you build confidence and improve your problem-solving skills.
10. Interview Day Tips and Strategies
On the day of your interview, keep these tips in mind:
- Think Aloud: Verbalize your thought process as you work through problems.
- Ask Clarifying Questions: Ensure you fully understand the problem before diving into a solution.
- Consider Edge Cases: Demonstrate thoroughness by considering various scenarios and input types.
- Optimize Your Solution: If time allows, discuss potential optimizations for your initial solution.
- Connect to Rivian’s Mission: When appropriate, relate your answers to Rivian’s goals and challenges.
- Be Honest: If you don’t know something, admit it and discuss how you’d approach learning it.
- Show Enthusiasm: Demonstrate your passion for technology and Rivian’s mission.
Remember, the interview is not just about getting the right answer, but about demonstrating your problem-solving approach and how you think through challenges.
11. Conclusion: Driving Towards Success
Preparing for a technical interview at Rivian is an exciting journey that combines traditional software engineering skills with the innovative world of electric vehicles. By focusing on core computer science concepts, practicing coding problems, understanding system design, and familiarizing yourself with EV-specific knowledge, you’ll be well-equipped to showcase your skills and passion.
Remember that Rivian is not just looking for technical prowess, but also for individuals who align with their mission of creating sustainable transportation solutions. As you prepare, keep in mind how your skills and interests can contribute to Rivian’s goals of revolutionizing the automotive industry.
Approach your interview with confidence, curiosity, and a genuine enthusiasm for the challenges that lie ahead. With thorough preparation and the right mindset, you’ll be well on your way to joining the innovative team at Rivian and contributing to the future of electric vehicles.
Good luck with your interview, and may your journey with Rivian be as exciting and groundbreaking as the vehicles they create!