Mastering Pinterest Technical Interviews: A Comprehensive Guide
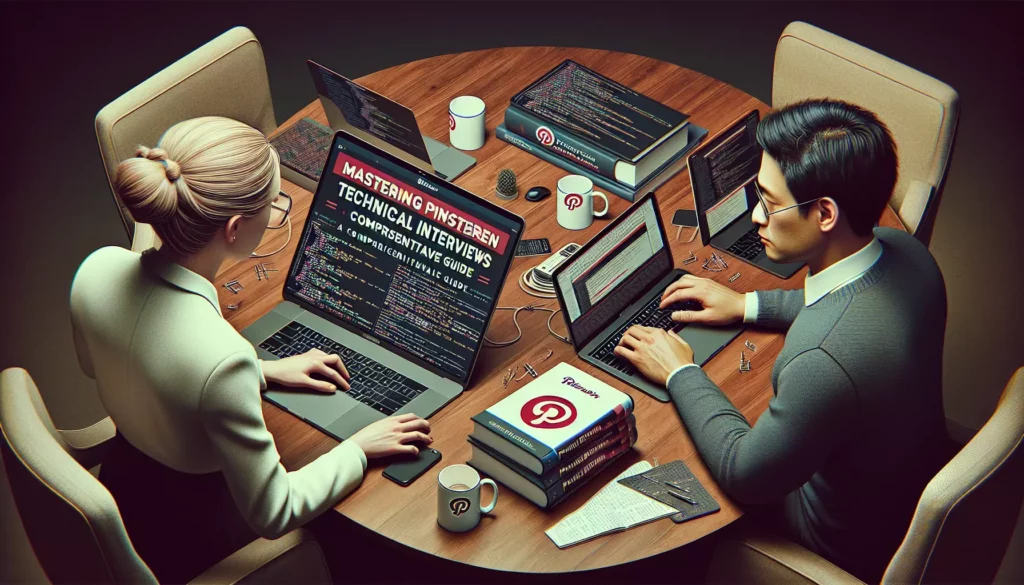
In the competitive landscape of tech careers, securing a position at a company like Pinterest can be a game-changer for your professional journey. Known for its innovative visual discovery engine, Pinterest offers exciting opportunities for software engineers to work on cutting-edge technologies. However, landing a job at Pinterest requires thorough preparation, especially for their technical interviews. This comprehensive guide will walk you through the essential steps to ace your Pinterest technical interview, covering everything from the interview process to specific topics you should focus on.
Understanding the Pinterest Interview Process
Before diving into the preparation strategies, it’s crucial to understand the typical interview process at Pinterest. While the exact steps may vary, the general structure often includes:
- Initial Phone Screen: A brief conversation with a recruiter to discuss your background and interest in the role.
- Technical Phone Interview: A coding interview conducted over the phone or video call, usually lasting 45-60 minutes.
- On-site Interviews: A series of 4-5 interviews covering various technical topics and behavioral questions.
- System Design Interview: For more experienced candidates, this round focuses on designing large-scale systems.
- Culture Fit Interview: A discussion about your work style and how you align with Pinterest’s values.
Key Areas to Focus On
To succeed in Pinterest’s technical interviews, you should be well-versed in the following areas:
1. Data Structures and Algorithms
Pinterest, like many tech companies, places a strong emphasis on algorithmic problem-solving. You should be comfortable with fundamental data structures and algorithms, including:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
- Dynamic Programming
- Sorting and Searching Algorithms
Practice implementing these data structures from scratch and solving problems that utilize them efficiently.
2. System Design
For senior-level positions, system design questions are crucial. You should be able to design scalable systems similar to Pinterest’s features. Key areas to focus on include:
- Distributed Systems
- Database Sharding
- Caching Mechanisms
- Load Balancing
- Microservices Architecture
- Content Delivery Networks (CDNs)
Familiarize yourself with Pinterest’s architecture and how they handle millions of users and images.
3. Web Technologies
Given Pinterest’s nature as a web-based platform, a solid understanding of web technologies is essential:
- HTML, CSS, and JavaScript
- RESTful APIs
- Front-end frameworks (React, Angular, or Vue.js)
- Back-end technologies (Node.js, Python, Java, or Go)
- Database systems (SQL and NoSQL)
4. Machine Learning and AI
Pinterest heavily relies on machine learning for content recommendation and user experience optimization. While not all roles require deep ML knowledge, having a basic understanding can be beneficial:
- Recommendation Systems
- Image Recognition
- Natural Language Processing
- A/B Testing
5. Mobile Development
If you’re interviewing for a mobile-focused role, make sure you’re proficient in:
- iOS Development (Swift, Objective-C)
- Android Development (Java, Kotlin)
- Cross-platform frameworks (React Native, Flutter)
Preparing for Coding Interviews
To excel in the coding portions of Pinterest’s technical interviews, follow these preparation strategies:
1. Practice Coding Problems
Regularly solve coding problems on platforms like LeetCode, HackerRank, or AlgoCademy. Focus on medium to hard difficulty problems, and try to solve at least 2-3 problems daily. Pay special attention to problems related to Pinterest’s domain, such as image processing, data analysis, and recommendation algorithms.
2. Mock Interviews
Conduct mock interviews with friends, mentors, or through online platforms. This will help you get comfortable with explaining your thought process while coding under pressure.
3. Time Management
Practice solving problems within time constraints. In real interviews, you’ll typically have 45-60 minutes to solve 1-2 problems, so train yourself to work efficiently.
4. Whiteboarding Skills
For on-site interviews, practice writing code on a whiteboard. This is a different experience from typing on a computer and requires clear, organized thinking.
5. Code Quality
Focus on writing clean, readable, and efficient code. Pinterest values code quality, so practice writing code that’s not just correct but also well-structured and optimized.
Sample Coding Problem
Here’s an example of a coding problem you might encounter in a Pinterest technical interview:
Problem: Image Clustering
Given a list of image objects, each with attributes like color, size, and category, implement a function to cluster similar images together. The function should return a list of clusters, where each cluster contains images that are considered similar based on their attributes.
Here’s a Python implementation to solve this problem:
class Image:
def __init__(self, color, size, category):
self.color = color
self.size = size
self.category = category
def cluster_images(images, similarity_threshold):
clusters = []
for image in images:
added_to_cluster = False
for cluster in clusters:
if is_similar(image, cluster[0], similarity_threshold):
cluster.append(image)
added_to_cluster = True
break
if not added_to_cluster:
clusters.append([image])
return clusters
def is_similar(img1, img2, threshold):
color_similarity = 1 if img1.color == img2.color else 0
size_similarity = 1 - abs(img1.size - img2.size) / max(img1.size, img2.size)
category_similarity = 1 if img1.category == img2.category else 0
total_similarity = (color_similarity + size_similarity + category_similarity) / 3
return total_similarity >= threshold
# Example usage
images = [
Image("red", 100, "landscape"),
Image("blue", 150, "portrait"),
Image("red", 110, "landscape"),
Image("green", 200, "abstract"),
Image("blue", 160, "portrait")
]
clusters = cluster_images(images, 0.7)
for i, cluster in enumerate(clusters):
print(f"Cluster {i + 1}:")
for img in cluster:
print(f" Color: {img.color}, Size: {img.size}, Category: {img.category}")
This solution demonstrates several key concepts:
- Object-Oriented Programming: Using a class to represent images
- Algorithm Design: Implementing a clustering algorithm
- Similarity Calculation: Defining a metric for image similarity
- Data Structure Usage: Working with lists and custom objects
When discussing this solution in an interview, be prepared to explain:
- The time and space complexity of your algorithm
- How you would scale this solution for a large number of images
- Potential optimizations or alternative approaches
- How this relates to Pinterest’s image recommendation system
System Design for Pinterest
For system design interviews, you might be asked to design a feature similar to Pinterest’s core functionality. Here’s an example of how you might approach designing a simplified version of Pinterest’s image recommendation system:
1. Requirements Gathering
- Functional Requirements:
- Users can upload images
- Users can search for images
- System recommends relevant images to users
- Users can save and organize images into boards
- Non-Functional Requirements:
- High availability and scalability
- Low latency for image retrieval and recommendations
- Ability to handle millions of users and billions of images
2. High-Level Design
[User] <--> [Load Balancer] <--> [Web Servers] <--> [Application Servers]
^
|
v
[CDN] <--> [Image Storage] <--> [Database Cluster]
^
|
v
[Recommendation Engine]
3. Component Details
- Load Balancer: Distributes incoming traffic across multiple web servers.
- Web Servers: Handle HTTP requests and serve static content.
- Application Servers: Process business logic and handle dynamic content generation.
- Database Cluster: Stores user data, image metadata, and relationships. Use a combination of relational (e.g., PostgreSQL) and NoSQL (e.g., Cassandra) databases.
- Image Storage: Use object storage like Amazon S3 for storing actual image files.
- CDN: Caches and serves images from edge locations to reduce latency.
- Recommendation Engine: Utilizes machine learning algorithms to generate personalized image recommendations.
4. Data Model
Consider the following simplified data model:
User:
- id
- username
- email
- password_hash
Image:
- id
- url
- upload_date
- user_id (uploader)
- metadata (tags, description)
Board:
- id
- name
- user_id (owner)
BoardImage:
- board_id
- image_id
UserInteraction:
- user_id
- image_id
- interaction_type (view, save, click)
- timestamp
5. Scaling Considerations
- Database Sharding: Partition data across multiple database servers based on user ID or image ID.
- Caching: Implement multi-level caching (e.g., Redis) to reduce database load and improve response times.
- Asynchronous Processing: Use message queues (e.g., RabbitMQ) for handling time-consuming tasks like image processing and recommendation updates.
- Microservices Architecture: Break down the system into smaller, independently deployable services (e.g., user service, image service, recommendation service).
6. Machine Learning Pipeline
For the recommendation engine:
- Collect user interaction data (views, saves, clicks)
- Process and store this data in a suitable format (e.g., Apache Hadoop)
- Train recommendation models using techniques like collaborative filtering or deep learning
- Deploy models to serve real-time recommendations
- Continuously update models based on new data and A/B test improvements
Behavioral Interview Preparation
While technical skills are crucial, Pinterest also values cultural fit and soft skills. Prepare for behavioral questions by reflecting on your past experiences and how they align with Pinterest’s values. Some key areas to focus on:
1. Collaboration and Teamwork
Prepare examples of how you’ve worked effectively in teams, resolved conflicts, and contributed to a positive work environment.
2. Problem-Solving and Innovation
Highlight instances where you’ve tackled complex problems or introduced innovative solutions in your previous roles.
3. User-Centric Approach
Pinterest is deeply committed to user experience. Discuss how you’ve considered user needs in your past projects or decision-making processes.
4. Adaptability and Learning
The tech industry evolves rapidly. Share examples of how you’ve adapted to new technologies or methodologies in your career.
5. Leadership and Initiative
Even if you’re not applying for a leadership role, Pinterest values individuals who can take initiative. Prepare stories about times you’ve led projects or mentored others.
Final Tips for Success
- Research Pinterest thoroughly: Understand their products, recent developments, and company culture.
- Practice explaining technical concepts: Be prepared to explain complex ideas in simple terms.
- Ask thoughtful questions: This shows your genuine interest in the role and the company.
- Stay calm and composed: If you get stuck during a coding interview, take a deep breath and think out loud.
- Follow-up after the interview: Send a thank-you note expressing your continued interest in the position.
Conclusion
Preparing for a Pinterest technical interview requires a comprehensive approach that covers both technical skills and soft skills. By focusing on the key areas outlined in this guide, practicing regularly, and staying up-to-date with Pinterest’s technologies and values, you’ll be well-equipped to tackle the interview process confidently.
Remember, the goal is not just to pass the interview but to demonstrate how you can contribute to Pinterest’s mission of bringing everyone the inspiration to create a life they love. With thorough preparation and the right mindset, you’ll be well on your way to joining the innovative team at Pinterest. Good luck with your interview preparation!