Cloudflare Technical Interview Prep: A Comprehensive Guide
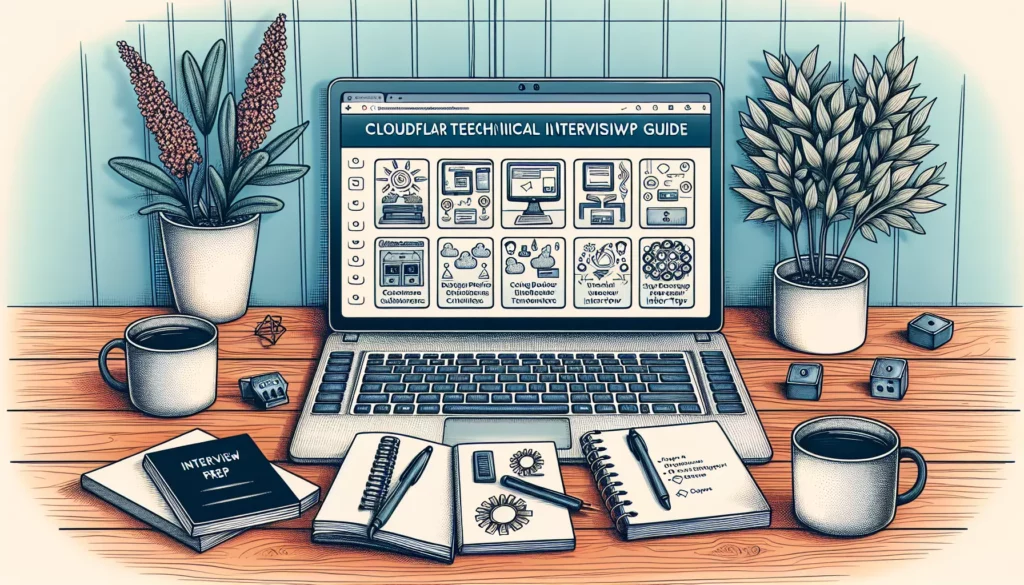
Are you gearing up for a technical interview at Cloudflare? You’re in the right place! This comprehensive guide will walk you through everything you need to know to ace your Cloudflare technical interview. From understanding the company’s culture to mastering the technical skills they’re looking for, we’ve got you covered.
Table of Contents
- Understanding Cloudflare
- The Cloudflare Interview Process
- Key Technical Skills to Master
- Common Coding Challenges and Solutions
- System Design Questions
- Essential Networking Concepts
- Security Knowledge
- Behavioral Questions and Company Culture
- Preparation Tips and Resources
- What to Expect on Interview Day
- Post-Interview Follow-up
1. Understanding Cloudflare
Before diving into the technical aspects, it’s crucial to understand what Cloudflare does and its mission. Cloudflare is a web infrastructure and website security company that provides content delivery network (CDN) services, DDoS mitigation, Internet security, and distributed domain name server services.
Key points to remember about Cloudflare:
- Founded in 2009 by Matthew Prince, Lee Holloway, and Michelle Zatlyn
- Mission: To help build a better Internet
- Offers a range of services including CDN, DDoS protection, DNS, and more
- Known for its innovative approach to Internet security and performance
Understanding Cloudflare’s products and services will give you an edge in your interview, as it demonstrates your genuine interest in the company and its technology.
2. The Cloudflare Interview Process
The Cloudflare interview process typically consists of several stages:
- Initial Screening: Usually a phone call with a recruiter to discuss your background and the role.
- Technical Phone Screen: A 45-60 minute call with an engineer, involving coding and technical questions.
- Take-Home Assignment: Some positions may require a take-home coding project.
- On-site Interviews: A series of interviews (currently conducted virtually) including:
- Coding interviews
- System design discussions
- Behavioral interviews
- Domain-specific technical questions
- Final Decision: The hiring team meets to make a decision based on your performance across all interviews.
Each stage is designed to assess different aspects of your skills and fit for the role. Let’s dive deeper into what you need to prepare for each of these areas.
3. Key Technical Skills to Master
Cloudflare looks for engineers with a strong foundation in computer science and practical coding skills. Here are the key areas to focus on:
Programming Languages
While Cloudflare uses various languages, proficiency in at least one of these is crucial:
- Go (Golang)
- Rust
- C/C++
- Python
- JavaScript
Data Structures and Algorithms
Be prepared to demonstrate your understanding of:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Sorting and Searching algorithms
- Dynamic Programming
- Big O notation and time/space complexity analysis
Web Technologies
Given Cloudflare’s focus on web infrastructure, knowledge of these is beneficial:
- HTTP/HTTPS protocols
- RESTful APIs
- WebSockets
- Content Delivery Networks (CDNs)
Distributed Systems
Understanding distributed systems concepts is crucial:
- Consistency models
- Replication and partitioning
- Consensus algorithms (e.g., Raft, Paxos)
- Load balancing
- Caching strategies
4. Common Coding Challenges and Solutions
During your Cloudflare interview, you’re likely to encounter coding challenges. Here are some common types of problems and how to approach them:
String Manipulation
Example: Implement a function to reverse words in a string.
function reverseWords(str) {
return str.split(' ').reverse().join(' ');
}
// Test
console.log(reverseWords("Hello World")); // Output: "World Hello"
Array Processing
Example: Find the missing number in an array containing 1 to n integers.
function findMissingNumber(arr, n) {
const expectedSum = (n * (n + 1)) / 2;
const actualSum = arr.reduce((sum, num) => sum + num, 0);
return expectedSum - actualSum;
}
// Test
console.log(findMissingNumber([1, 2, 4, 6, 3, 7, 8], 8)); // Output: 5
Tree Traversal
Example: Implement in-order traversal of a binary tree.
class TreeNode {
constructor(val) {
this.val = val;
this.left = null;
this.right = null;
}
}
function inorderTraversal(root) {
const result = [];
function traverse(node) {
if (node === null) return;
traverse(node.left);
result.push(node.val);
traverse(node.right);
}
traverse(root);
return result;
}
// Test
const root = new TreeNode(1);
root.left = new TreeNode(2);
root.right = new TreeNode(3);
root.left.left = new TreeNode(4);
root.left.right = new TreeNode(5);
console.log(inorderTraversal(root)); // Output: [4, 2, 5, 1, 3]
Remember, it’s not just about getting the correct answer, but also about demonstrating your problem-solving process, coding style, and ability to optimize solutions.
5. System Design Questions
System design questions are a crucial part of the Cloudflare interview process, especially for more senior positions. Here are some tips and a sample question:
Tips for System Design Questions
- Clarify requirements and constraints
- Start with a high-level design
- Deep dive into specific components
- Discuss trade-offs in your design choices
- Consider scalability, reliability, and security
Sample System Design Question: Design a URL Shortener
Here’s a basic approach to designing a URL shortener:
- Requirements Clarification:
- Functional: Shorten URL, redirect to original URL
- Non-functional: High availability, low latency, scalability
- High-level Design:
- API Gateway
- Application servers
- Database (for URL mappings)
- Cache (for frequently accessed URLs)
- Detailed Design:
- URL encoding algorithm (e.g., base62 encoding)
- Database schema
- Caching strategy
- Scaling Considerations:
- Database sharding
- Load balancing
- CDN for global distribution
Be prepared to discuss each component in detail and explain your design choices.
6. Essential Networking Concepts
Given Cloudflare’s focus on Internet infrastructure, a solid understanding of networking concepts is crucial. Here are key areas to review:
TCP/IP Protocol Suite
- Understanding of the OSI model
- TCP vs UDP
- IP addressing and subnetting
- DNS (Domain Name System)
HTTP and HTTPS
- HTTP methods (GET, POST, PUT, DELETE, etc.)
- Status codes
- Headers
- HTTPS and SSL/TLS
Content Delivery Networks (CDNs)
- How CDNs work
- Caching strategies
- Edge computing
Load Balancing
- Types of load balancers
- Load balancing algorithms
- Health checks
Be prepared to discuss these concepts in the context of Cloudflare’s services and how they contribute to improving web performance and security.
7. Security Knowledge
Security is a core focus for Cloudflare, so demonstrating your knowledge in this area can set you apart. Key areas to study include:
Common Web Vulnerabilities
- Cross-Site Scripting (XSS)
- SQL Injection
- Cross-Site Request Forgery (CSRF)
- Man-in-the-Middle (MITM) attacks
DDoS Attacks and Mitigation
- Types of DDoS attacks
- DDoS mitigation techniques
- Cloudflare’s approach to DDoS protection
Encryption and Authentication
- Symmetric vs Asymmetric encryption
- SSL/TLS protocols
- Two-factor authentication
Web Application Firewalls (WAF)
- How WAFs work
- Rule-based vs. AI-based WAFs
- Cloudflare’s WAF offerings
Being able to discuss these security concepts and how they relate to Cloudflare’s products will demonstrate your alignment with the company’s mission and technical focus.
8. Behavioral Questions and Company Culture
Cloudflare places a high value on cultural fit. Be prepared to answer behavioral questions that assess your alignment with the company’s values and work style. Some common themes include:
- Teamwork and collaboration
- Problem-solving and innovation
- Adaptability and learning
- Handling challenges and conflicts
Example questions you might encounter:
- “Tell me about a time when you had to learn a new technology quickly.”
- “Describe a situation where you had to work with a difficult team member.”
- “How do you stay updated with the latest trends in technology?”
- “Can you share an example of how you’ve contributed to improving a process or system?”
When answering these questions, use the STAR method (Situation, Task, Action, Result) to structure your responses and provide concrete examples from your experience.
9. Preparation Tips and Resources
To maximize your chances of success in the Cloudflare interview, consider the following preparation strategies:
Coding Practice
- Solve problems on platforms like LeetCode, HackerRank, or CodeSignal
- Focus on medium to hard difficulty problems
- Practice explaining your thought process while coding
System Design Study
- Read books like “Designing Data-Intensive Applications” by Martin Kleppmann
- Study system design interview guides online
- Practice designing real-world systems
Networking and Security Resources
- Review Cloudflare’s learning center and blog for in-depth articles
- Study networking fundamentals through online courses or textbooks
- Keep up with the latest security trends and vulnerabilities
Company Research
- Read Cloudflare’s annual reports and recent news
- Understand their products and how they work
- Follow Cloudflare on social media for recent updates
Mock Interviews
- Practice with friends or use platforms like Pramp for mock technical interviews
- Record yourself answering behavioral questions to improve your delivery
10. What to Expect on Interview Day
On the day of your Cloudflare interview (whether in-person or virtual), keep these points in mind:
For Virtual Interviews
- Test your internet connection, camera, and microphone beforehand
- Choose a quiet, well-lit location
- Have a backup device ready in case of technical issues
- Keep a notepad and pen handy for taking notes
For In-Person Interviews
- Arrive early to account for any unexpected delays
- Bring multiple copies of your resume
- Dress professionally, even if the company culture is casual
General Tips
- Be prepared to code on a whiteboard or in a shared coding environment
- Ask clarifying questions before diving into problems
- Think out loud to show your problem-solving process
- Be ready to discuss your past projects and experiences in detail
- Prepare thoughtful questions to ask your interviewers about Cloudflare and the role
11. Post-Interview Follow-up
After your interview, take these steps to leave a positive final impression:
- Send a thank-you email to your interviewers within 24 hours
- Briefly reiterate your interest in the position and Cloudflare
- If you discussed any specific topics or projects, you could mention them to show you were engaged
- Be patient while waiting for a response, but don’t hesitate to follow up if you haven’t heard back after a week
Conclusion
Preparing for a Cloudflare technical interview requires a comprehensive approach, covering not just coding skills but also system design, networking concepts, and security knowledge. By thoroughly preparing in these areas and understanding Cloudflare’s mission and culture, you’ll be well-equipped to showcase your skills and stand out as a strong candidate.
Remember, the interview is not just about demonstrating your technical prowess but also about showing your passion for technology, your problem-solving approach, and your fit with Cloudflare’s innovative culture. Stay calm, be yourself, and let your skills and enthusiasm shine through. Good luck with your Cloudflare interview!