Zoom Technical Interview Prep: A Comprehensive Guide
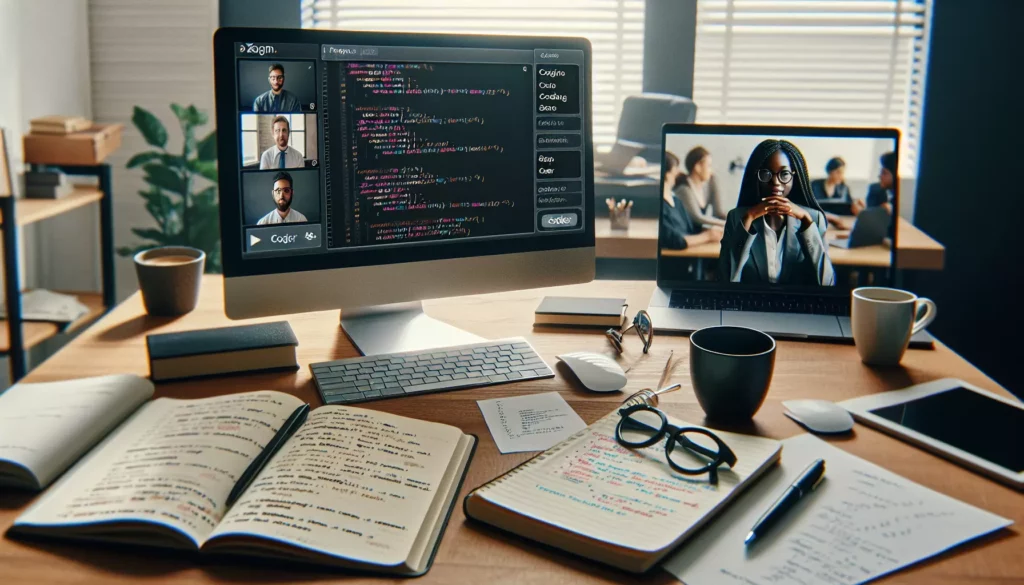
As the tech industry continues to evolve, companies like Zoom have become integral parts of our daily lives, especially in the era of remote work and digital communication. If you’re a software engineer or developer looking to join the Zoom team, you’ll need to be well-prepared for their technical interview process. In this comprehensive guide, we’ll walk you through everything you need to know to ace your Zoom technical interview, from understanding the company’s culture to mastering the most common coding challenges.
Table of Contents
- Understanding Zoom as a Company
- The Zoom Interview Process
- Key Technical Skills to Master
- Common Coding Challenges and How to Approach Them
- System Design Questions
- Behavioral Questions and Company Culture Fit
- Preparation Tips and Resources
- What to Expect on Interview Day
- Post-Interview Follow-up and Next Steps
- Conclusion
1. Understanding Zoom as a Company
Before diving into the technical aspects of your interview preparation, it’s crucial to understand Zoom as a company. Zoom Video Communications, Inc. was founded in 2011 by Eric Yuan, with a mission to make video communications frictionless and secure. The company has grown exponentially, especially during the COVID-19 pandemic, becoming a household name for video conferencing.
Key points to remember about Zoom:
- Focus on user experience and reliability
- Emphasis on scalability and performance
- Commitment to security and privacy
- Continuous innovation in video communication technology
Understanding these core values and focus areas will help you align your responses and demonstrate your fit with the company culture during the interview process.
2. The Zoom Interview Process
The Zoom technical interview process typically consists of several stages:
- Initial Screening: A phone or video call with a recruiter to discuss your background and the role.
- Technical Phone Screen: A 45-60 minute interview with an engineer, focusing on coding and problem-solving skills.
- On-site Interviews (or Virtual On-site): A series of interviews, usually 4-5 rounds, including:
- Coding interviews
- System design interview
- Behavioral interviews
- Final Decision: The hiring team reviews all feedback and makes a decision.
Each stage is designed to assess different aspects of your skills and fit for the role. Let’s dive deeper into what you need to prepare for each of these stages.
3. Key Technical Skills to Master
To succeed in Zoom’s technical interviews, you should be proficient in the following areas:
Programming Languages
While Zoom uses various languages, proficiency in at least one of these is crucial:
- C++
- Java
- Python
- JavaScript (for frontend roles)
Data Structures
Ensure you have a solid understanding of:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
Algorithms
Be prepared to implement and discuss:
- Sorting algorithms (QuickSort, MergeSort)
- Searching algorithms (Binary Search)
- Graph algorithms (BFS, DFS, Dijkstra’s)
- Dynamic Programming
- Recursion
System Design Concepts
Familiarize yourself with:
- Distributed Systems
- Scalability
- Load Balancing
- Caching
- Database Design
- API Design
Networking
Given Zoom’s focus on video communication, understanding these concepts is crucial:
- TCP/IP protocol
- WebRTC
- Video Codecs
- Network Security
4. Common Coding Challenges and How to Approach Them
Zoom’s coding interviews typically focus on algorithmic problem-solving. Here are some common types of questions you might encounter, along with approaches to solve them:
String Manipulation
Example: Implement a function to reverse words in a string.
def reverse_words(s):
# Split the string into words
words = s.split()
# Reverse the list of words
words.reverse()
# Join the words back into a string
return " ".join(words)
# Test the function
print(reverse_words("Hello World from Zoom")) # Output: "Zoom from World Hello"
Array Processing
Example: Find the maximum subarray sum in an array of integers.
def max_subarray_sum(arr):
max_sum = current_sum = arr[0]
for num in arr[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
# Test the function
print(max_subarray_sum([-2, 1, -3, 4, -1, 2, 1, -5, 4])) # Output: 6
Tree Traversal
Example: Implement an in-order traversal of a binary tree.
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal(root):
result = []
if root:
result += inorder_traversal(root.left)
result.append(root.val)
result += inorder_traversal(root.right)
return result
# Test the function
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
print(inorder_traversal(root)) # Output: [4, 2, 5, 1, 3]
Graph Algorithms
Example: Implement Breadth-First Search (BFS) on a graph.
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
vertex = queue.popleft()
print(vertex, end=" ")
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
# Test the function
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
bfs(graph, 'A') # Output: A B C D E F
Remember, when solving these problems:
- Clarify the problem and any assumptions
- Think out loud and explain your thought process
- Start with a brute force approach, then optimize
- Consider edge cases and test your solution
5. System Design Questions
For more senior roles, you’ll likely encounter system design questions. These assess your ability to design large-scale distributed systems. Some potential Zoom-related system design questions might include:
- Design a video conferencing system like Zoom
- Design a chat application that can handle millions of users
- Design a system for real-time collaboration on documents
When approaching system design questions:
- Clarify Requirements: Understand the scale, features, and constraints of the system.
- High-Level Design: Start with a basic architecture and main components.
- Deep Dive: Elaborate on key components, discussing tradeoffs and potential bottlenecks.
- Scalability: Explain how your design would scale to handle increased load.
- Data Management: Discuss data storage, caching, and consistency considerations.
For example, when designing a video conferencing system like Zoom, you might consider:
- WebRTC for peer-to-peer connections
- Media servers for multi-party conferences
- Load balancers to distribute traffic
- Caching mechanisms for user data and preferences
- Database design for user accounts and meeting data
- Security measures like end-to-end encryption
6. Behavioral Questions and Company Culture Fit
Zoom places a high value on cultural fit. Be prepared to answer behavioral questions that demonstrate your alignment with Zoom’s values and work culture. Some common questions might include:
- Tell me about a time when you had to overcome a significant technical challenge.
- How do you stay updated with the latest technologies in your field?
- Describe a situation where you had to work with a difficult team member.
- How do you handle tight deadlines and pressure?
- Can you give an example of how you’ve contributed to improving a product or process?
When answering these questions:
- Use the STAR method (Situation, Task, Action, Result)
- Be specific and provide concrete examples
- Highlight your problem-solving skills and ability to work in a team
- Show your passion for technology and continuous learning
- Demonstrate your alignment with Zoom’s mission and values
7. Preparation Tips and Resources
To effectively prepare for your Zoom technical interview:
- Practice Coding: Use platforms like LeetCode, HackerRank, or AlgoCademy to solve coding problems regularly.
- Study System Design: Read books like “Designing Data-Intensive Applications” by Martin Kleppmann or “System Design Interview” by Alex Xu.
- Mock Interviews: Practice with friends or use services like Pramp for mock technical interviews.
- Review Computer Science Fundamentals: Brush up on data structures, algorithms, and networking concepts.
- Learn About Zoom: Study Zoom’s products, recent news, and technological challenges they might be facing.
- Prepare Questions: Have thoughtful questions ready to ask your interviewers about the role and company.
Useful resources:
- Zoom’s Engineering Blog
- Glassdoor interview experiences for Zoom
- YouTube videos on system design and coding interviews
- Online courses on advanced programming and system design
8. What to Expect on Interview Day
On the day of your Zoom interview (which will likely be conducted over Zoom!):
- Test Your Setup: Ensure your internet connection, camera, and microphone are working properly.
- Dress Professionally: Even for a virtual interview, dress as you would for an in-person interview.
- Have Your Environment Ready: Choose a quiet, well-lit space for your interview.
- Be Prepared to Share Your Screen: For coding interviews, you might be asked to write code in a shared editor.
- Have Water and a Notepad Handy: You might want to jot down notes or questions during the interview.
- Stay Calm and Focused: Remember to breathe and take your time when answering questions.
9. Post-Interview Follow-up and Next Steps
After your interview:
- Send Thank You Emails: Within 24 hours, send personalized thank you emails to your interviewers.
- Reflect on the Experience: Make notes about the questions asked and areas where you felt strong or weak.
- Follow Up: If you haven’t heard back within the timeframe provided, it’s appropriate to follow up with your recruiter.
- Prepare for Next Steps: If you’re moved forward, you might have additional interviews or receive an offer.
- Handle Rejection Gracefully: If you don’t get the position, ask for feedback and consider it a learning experience.
10. Conclusion
Preparing for a Zoom technical interview requires a combination of strong technical skills, problem-solving abilities, and cultural fit. By focusing on mastering core computer science concepts, practicing coding challenges, understanding system design principles, and aligning yourself with Zoom’s values, you’ll be well-prepared to showcase your skills and land that dream job at Zoom.
Remember, the key to success in any technical interview is not just about having the right answers, but also about demonstrating your thought process, your ability to learn and adapt, and your passion for technology. With thorough preparation and the right mindset, you’ll be well on your way to joining the team that’s revolutionizing how the world communicates.
Good luck with your Zoom technical interview!