Shopify Technical Interview Prep: A Comprehensive Guide
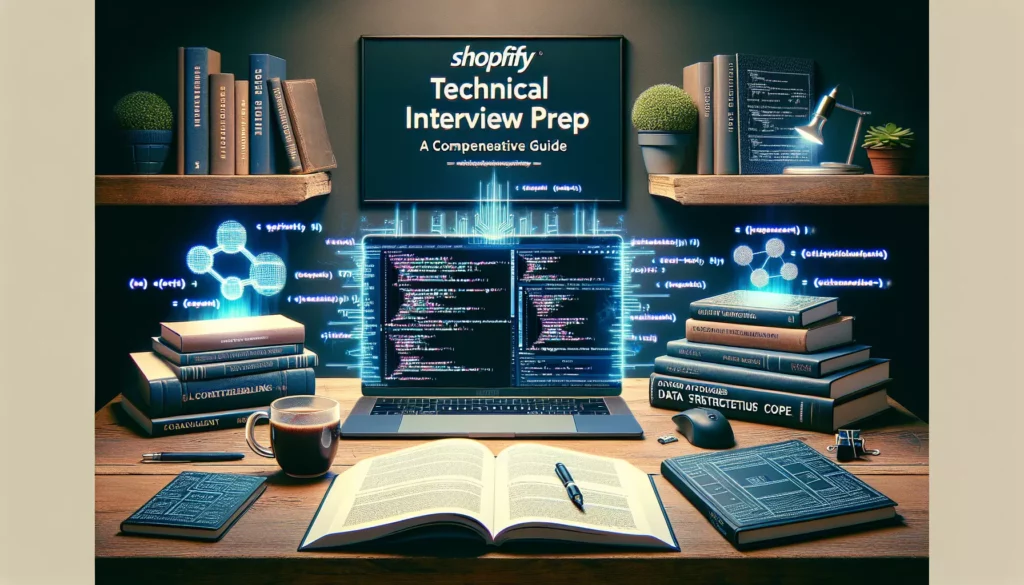
Are you gearing up for a technical interview at Shopify? You’re in the right place! This comprehensive guide will walk you through everything you need to know to ace your Shopify technical interview. From understanding the interview process to mastering key coding concepts, we’ve got you covered. Let’s dive in and set you up for success!
Table of Contents
- Understanding Shopify
- The Shopify Interview Process
- Key Technical Skills to Master
- Coding Languages to Focus On
- Essential Data Structures
- Important Algorithms to Know
- System Design Concepts
- Practice Questions and Resources
- Interview Tips and Strategies
- Post-Interview Steps
1. Understanding Shopify
Before diving into the technical aspects, it’s crucial to understand what Shopify is and what they do. Shopify is a leading e-commerce platform that allows businesses to create and manage online stores. They provide a wide range of services, from website building to payment processing and inventory management.
Key points to remember about Shopify:
- Founded in 2006
- Headquartered in Ottawa, Canada
- Serves millions of businesses across 175 countries
- Offers a variety of APIs and developer tools
- Emphasizes scalability, performance, and user experience
Understanding Shopify’s business model and technical challenges will give you an edge in your interview. It shows that you’re not just prepared for the coding questions, but you’re also interested in the company’s mission and the problems they solve.
2. The Shopify Interview Process
The Shopify technical interview process typically consists of several stages:
- Initial Application: Submit your resume and cover letter through Shopify’s careers page.
- Screening Call: A brief call with a recruiter to discuss your background and the role.
- Technical Screen: A coding challenge or a brief technical interview to assess your basic skills.
- Technical Interviews: Usually 2-3 rounds of in-depth technical interviews.
- System Design Interview: For more senior positions, you may have a system design round.
- Cultural Fit Interview: A discussion about your work style and how you’d fit into Shopify’s culture.
- Final Decision: The hiring team reviews all feedback and makes a decision.
Each stage is designed to evaluate different aspects of your skills and experience. Be prepared for a mix of algorithmic problems, practical coding tasks, and discussions about your past projects and experiences.
3. Key Technical Skills to Master
To excel in your Shopify technical interview, you should be proficient in the following areas:
- Strong understanding of object-oriented programming (OOP) principles
- Proficiency in at least one programming language (Ruby, Python, JavaScript, etc.)
- Knowledge of web technologies (HTTP, REST APIs, JSON)
- Understanding of database concepts (SQL and NoSQL)
- Familiarity with version control systems (Git)
- Experience with cloud platforms (AWS, Google Cloud, or Azure)
- Understanding of scalability and performance optimization
- Knowledge of testing methodologies and practices
While you don’t need to be an expert in all these areas, having a solid foundation in each will significantly boost your chances of success.
4. Coding Languages to Focus On
While Shopify uses a variety of programming languages, some are more prevalent than others. Here are the top languages to focus on:
Ruby
Ruby is the primary language used at Shopify, particularly Ruby on Rails for web development. Make sure you’re comfortable with Ruby syntax, object-oriented concepts in Ruby, and common Ruby on Rails patterns.
Example of a Ruby class:
class Product
attr_reader :name, :price
def initialize(name, price)
@name = name
@price = price
end
def discount(percentage)
@price *= (1 - percentage / 100.0)
end
end
product = Product.new("T-shirt", 20)
product.discount(10)
puts product.price # Output: 18.0
JavaScript
JavaScript is crucial for front-end development and is also used in Node.js applications. Focus on modern JavaScript (ES6+) features, asynchronous programming, and popular frameworks like React.
Example of asynchronous JavaScript:
async function fetchProduct(id) {
try {
const response = await fetch(`https://api.example.com/products/${id}`);
const data = await response.json();
return data;
} catch (error) {
console.error("Error fetching product:", error);
}
}
fetchProduct(123).then(product => console.log(product));
Python
While not as prevalent as Ruby or JavaScript, Python is used in various data analysis and machine learning tasks at Shopify. Familiarity with Python can be beneficial, especially for data-related positions.
Example of a Python function:
def calculate_total_revenue(orders):
return sum(order.total for order in orders)
class Order:
def __init__(self, id, total):
self.id = id
self.total = total
orders = [Order(1, 100), Order(2, 150), Order(3, 200)]
total_revenue = calculate_total_revenue(orders)
print(f"Total revenue: ${total_revenue}") # Output: Total revenue: $450
5. Essential Data Structures
A solid understanding of data structures is crucial for any technical interview. Here are some key data structures you should be familiar with:
Arrays and Strings
Arrays and strings are fundamental data structures. Be comfortable with operations like searching, sorting, and manipulating arrays and strings.
Example of array manipulation in Ruby:
def remove_duplicates(arr)
arr.uniq
end
original = [1, 2, 2, 3, 4, 4, 5]
unique = remove_duplicates(original)
puts unique.inspect # Output: [1, 2, 3, 4, 5]
Hash Tables (Dictionaries)
Hash tables are crucial for solving many algorithmic problems efficiently. Understand how they work and when to use them.
Example of using a hash table in Python:
def find_pair_with_sum(arr, target_sum):
complement_map = {}
for num in arr:
if num in complement_map:
return (complement_map[num], num)
complement_map[target_sum - num] = num
return None
result = find_pair_with_sum([10, 5, 2, 3, 7, 5], 10)
print(result) # Output: (3, 7)
Linked Lists
Linked lists are often used in interview questions. Be familiar with singly and doubly linked lists, and common operations like insertion, deletion, and reversal.
Example of a linked list in JavaScript:
class ListNode {
constructor(val = 0, next = null) {
this.val = val;
this.next = next;
}
}
function reverseLinkedList(head) {
let prev = null;
let current = head;
while (current !== null) {
let nextTemp = current.next;
current.next = prev;
prev = current;
current = nextTemp;
}
return prev;
}
// Create a linked list: 1 -> 2 -> 3
let head = new ListNode(1);
head.next = new ListNode(2);
head.next.next = new ListNode(3);
// Reverse the linked list
let reversed = reverseLinkedList(head);
// Print the reversed list
while (reversed !== null) {
console.log(reversed.val);
reversed = reversed.next;
}
// Output: 3, 2, 1
Trees and Graphs
Tree and graph problems are common in technical interviews. Focus on binary trees, binary search trees, and basic graph traversal algorithms.
Example of a binary tree traversal in Ruby:
class TreeNode
attr_accessor :val, :left, :right
def initialize(val = 0, left = nil, right = nil)
@val = val
@left = left
@right = right
end
end
def inorder_traversal(root)
result = []
inorder_helper(root, result)
result
end
def inorder_helper(node, result)
return if node.nil?
inorder_helper(node.left, result)
result << node.val
inorder_helper(node.right, result)
end
# Create a binary tree
root = TreeNode.new(1)
root.left = TreeNode.new(2)
root.right = TreeNode.new(3)
root.left.left = TreeNode.new(4)
root.left.right = TreeNode.new(5)
puts inorder_traversal(root).inspect # Output: [4, 2, 5, 1, 3]
6. Important Algorithms to Know
Mastering key algorithms is essential for succeeding in technical interviews. Here are some important algorithms you should be familiar with:
Sorting Algorithms
Understand the implementation and time complexity of common sorting algorithms like QuickSort, MergeSort, and BubbleSort.
Example of QuickSort in Python:
def quicksort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
print(quicksort([3, 6, 8, 10, 1, 2, 1])) # Output: [1, 1, 2, 3, 6, 8, 10]
Search Algorithms
Be comfortable with linear search, binary search, and their applications.
Example of binary search in JavaScript:
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
const sortedArray = [1, 3, 5, 7, 9, 11, 13, 15];
console.log(binarySearch(sortedArray, 7)); // Output: 3
console.log(binarySearch(sortedArray, 6)); // Output: -1
Graph Algorithms
Understand basic graph traversal algorithms like Breadth-First Search (BFS) and Depth-First Search (DFS).
Example of BFS in Ruby:
def bfs(graph, start)
visited = {}
queue = [start]
visited[start] = true
while !queue.empty?
vertex = queue.shift
puts vertex
graph[vertex].each do |neighbor|
if !visited[neighbor]
visited[neighbor] = true
queue.push(neighbor)
end
end
end
end
graph = {
0 => [1, 2],
1 => [2],
2 => [0, 3],
3 => [3]
}
bfs(graph, 2) # Output: 2, 0, 3, 1
Dynamic Programming
Dynamic programming is a powerful technique for solving optimization problems. Understand the basics and practice solving DP problems.
Example of a dynamic programming solution in Python:
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
print(fibonacci(10)) # Output: 55
7. System Design Concepts
For more senior positions, you may be asked system design questions. Here are some key concepts to understand:
- Scalability: Understand how to design systems that can handle increasing loads.
- Load Balancing: Know how to distribute traffic across multiple servers.
- Caching: Understand caching strategies and when to use them.
- Database Sharding: Know how to partition data across multiple databases.
- Microservices Architecture: Understand the principles and trade-offs of microservices.
- API Design: Know how to design clean, efficient APIs.
Example of a simple API design in Ruby on Rails:
# app/controllers/api/v1/products_controller.rb
module Api
module V1
class ProductsController < ApplicationController
def index
products = Product.all
render json: products, status: :ok
end
def show
product = Product.find(params[:id])
render json: product, status: :ok
rescue ActiveRecord::RecordNotFound
render json: { error: "Product not found" }, status: :not_found
end
def create
product = Product.new(product_params)
if product.save
render json: product, status: :created
else
render json: { errors: product.errors.full_messages }, status: :unprocessable_entity
end
end
private
def product_params
params.require(:product).permit(:name, :price, :description)
end
end
end
end
8. Practice Questions and Resources
To prepare for your Shopify technical interview, it’s crucial to practice coding questions regularly. Here are some resources to help you:
- LeetCode: A platform with a vast collection of coding problems, many of which are commonly asked in technical interviews.
- HackerRank: Offers coding challenges and competitions to improve your skills.
- Cracking the Coding Interview: A book by Gayle Laakmann McDowell that provides a comprehensive guide to technical interviews.
- Shopify Engineering Blog: Read about the technical challenges and solutions at Shopify.
- System Design Primer: A GitHub repository with resources for learning system design.
Here’s a sample coding question you might encounter:
Problem: Implement a function to find the k most frequent elements in an array.
Solution in Ruby:
def top_k_frequent(nums, k)
frequency = Hash.new(0)
nums.each { |num| frequency[num] += 1 }
frequency.sort_by { |_, count| -count }.first(k).map(&:first)
end
puts top_k_frequent([1,1,1,2,2,3], 2).inspect # Output: [1, 2]
puts top_k_frequent([1], 1).inspect # Output: [1]
9. Interview Tips and Strategies
Here are some tips to help you succeed in your Shopify technical interview:
- Think out loud: Explain your thought process as you solve problems. This gives the interviewer insight into how you approach challenges.
- Ask clarifying questions: Make sure you understand the problem fully before starting to code.
- Consider edge cases: Think about potential edge cases and how your solution handles them.
- Optimize your solution: If you come up with a working solution, think about ways to optimize it for better time or space complexity.
- Test your code: After implementing a solution, walk through it with a test case to catch any bugs.
- Be open to feedback: If the interviewer provides hints or suggestions, be receptive and incorporate them into your approach.
- Show enthusiasm: Demonstrate your passion for coding and your interest in Shopify’s mission.
10. Post-Interview Steps
After your interview, consider the following steps:
- Send a thank-you note: Express your appreciation for the interviewer’s time and reiterate your interest in the position.
- Reflect on the experience: Think about what went well and areas where you could improve for future interviews.
- Follow up: If you haven’t heard back within the expected timeframe, don’t hesitate to follow up politely with your recruiter.
- Keep practicing: Regardless of the outcome, continue improving your coding skills and preparing for future opportunities.
Remember, interviewing is a skill that improves with practice. Each interview, regardless of the outcome, is an opportunity to learn and grow as a developer.
Conclusion
Preparing for a Shopify technical interview requires dedication and practice, but with the right approach, you can significantly increase your chances of success. Focus on strengthening your foundational knowledge in data structures and algorithms, practice coding regularly, and familiarize yourself with Shopify’s technology stack and business model. Stay curious, keep learning, and approach each interview as an opportunity to showcase your skills and passion for technology.
Good luck with your Shopify technical interview! Remember, the key to success is thorough preparation and a positive attitude. You’ve got this!