Asana Technical Interview Prep: A Comprehensive Guide
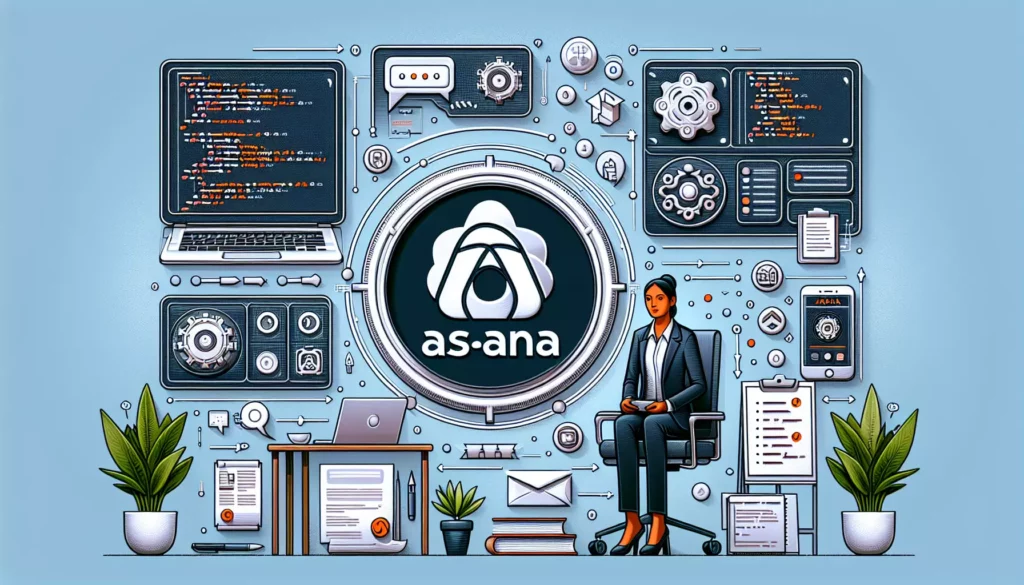
Are you gearing up for a technical interview at Asana? You’re in the right place! This comprehensive guide will walk you through everything you need to know to ace your Asana technical interview. From understanding the interview process to mastering key coding concepts and practicing with sample questions, we’ve got you covered.
Table of Contents
- Understanding Asana
- The Asana Interview Process
- Key Topics to Master
- Coding Languages to Focus On
- Essential Data Structures
- Important Algorithms
- System Design Concepts
- Sample Interview Questions
- Tips for Success
- Additional Resources
1. Understanding Asana
Before diving into interview preparation, it’s crucial to understand what Asana does and its core values. Asana is a leading work management platform that helps teams organize, track, and manage their work. Founded by Facebook co-founder Dustin Moskovitz and ex-Google and Facebook engineer Justin Rosenstein, Asana aims to help humanity thrive by enabling all teams to work together effortlessly.
Key points about Asana:
- Focus on productivity and collaboration tools
- Emphasis on user experience and intuitive design
- Scalable infrastructure to support millions of users
- Strong engineering culture with a focus on innovation
Understanding these aspects will help you align your responses and demonstrate your interest in the company during the interview process.
2. The Asana Interview Process
The Asana technical interview process typically consists of several stages:
- Initial Phone Screen: A brief call with a recruiter to discuss your background and interest in Asana.
- Technical Phone Interview: A 45-60 minute interview focusing on coding and problem-solving skills.
- Take-Home Project: Some candidates may be asked to complete a small project to showcase their coding abilities.
- On-site Interviews: A full day of interviews, including:
- Coding interviews
- System design discussion
- Behavioral interviews
- Culture fit assessment
Each stage is designed to evaluate different aspects of your skills and how well you’d fit into the Asana team.
3. Key Topics to Master
To excel in your Asana technical interview, focus on mastering these key areas:
- Data Structures and Algorithms
- Object-Oriented Programming (OOP)
- System Design and Scalability
- Web Technologies (HTTP, REST APIs, etc.)
- Database concepts (SQL and NoSQL)
- Front-end Technologies (JavaScript, React, etc.)
- Back-end Technologies (Node.js, Python, etc.)
- Version Control (Git)
- Testing and Debugging
- Agile Methodologies
Ensure you have a solid understanding of these topics and can apply them to real-world scenarios.
4. Coding Languages to Focus On
While Asana uses various programming languages, it’s essential to be proficient in at least one of the following:
- JavaScript (and TypeScript)
- Python
- Java
- C++
JavaScript is particularly important given Asana’s web-based platform. Here’s a simple example of a JavaScript function you might be asked to write:
function findMissingNumber(arr) {
const n = arr.length + 1;
const expectedSum = (n * (n + 1)) / 2;
const actualSum = arr.reduce((sum, num) => sum + num, 0);
return expectedSum - actualSum;
}
// Example usage:
const numbers = [1, 2, 4, 6, 3, 7, 8];
console.log(findMissingNumber(numbers)); // Output: 5
This function finds the missing number in an array of consecutive integers (with one number missing). Make sure you can explain the logic behind such functions and discuss their time and space complexity.
5. Essential Data Structures
Familiarize yourself with these fundamental data structures:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Hash Tables
- Trees (Binary Trees, Binary Search Trees, Balanced Trees)
- Graphs
- Heaps
Here’s an example of implementing a simple binary tree node in Python:
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
# Example usage:
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
Be prepared to implement these data structures from scratch and explain their properties, use cases, and trade-offs.
6. Important Algorithms
Master these essential algorithms and their implementations:
- Sorting algorithms (Quick Sort, Merge Sort, Heap Sort)
- Searching algorithms (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Graph algorithms (Dijkstra’s, Bellman-Ford, Floyd-Warshall)
- String manipulation algorithms
Here’s an example of implementing the Merge Sort algorithm in Java:
public class MergeSort {
public static void mergeSort(int[] arr, int left, int right) {
if (left < right) {
int mid = (left + right) / 2;
mergeSort(arr, left, mid);
mergeSort(arr, mid + 1, right);
merge(arr, left, mid, right);
}
}
private static void merge(int[] arr, int left, int mid, int right) {
int[] temp = new int[right - left + 1];
int i = left, j = mid + 1, k = 0;
while (i <= mid && j <= right) {
if (arr[i] <= arr[j]) {
temp[k++] = arr[i++];
} else {
temp[k++] = arr[j++];
}
}
while (i <= mid) {
temp[k++] = arr[i++];
}
while (j <= right) {
temp[k++] = arr[j++];
}
System.arraycopy(temp, 0, arr, left, temp.length);
}
public static void main(String[] args) {
int[] arr = {64, 34, 25, 12, 22, 11, 90};
mergeSort(arr, 0, arr.length - 1);
System.out.println(Arrays.toString(arr));
}
}
Be prepared to implement these algorithms, analyze their time and space complexity, and discuss when to use one algorithm over another.
7. System Design Concepts
For more senior roles, you’ll likely face system design questions. Be familiar with:
- Scalability and Load Balancing
- Caching strategies
- Database sharding and replication
- Microservices architecture
- API design
- Real-time systems
- Distributed systems concepts
A sample system design question might be: “Design a task management system like Asana.” Here’s a high-level approach:
- Clarify requirements (e.g., user authentication, task creation, assignment, notifications)
- Estimate scale (number of users, tasks, etc.)
- Design the data model (Users, Tasks, Projects, etc.)
- Outline the API endpoints
- Discuss the architecture (microservices vs monolith)
- Address scalability concerns (caching, load balancing, database sharding)
- Consider additional features (real-time updates, search functionality)
Be prepared to draw diagrams and explain your design choices during the interview.
8. Sample Interview Questions
Here are some sample questions you might encounter in an Asana technical interview:
Coding Questions:
- Implement a function to detect a cycle in a linked list.
- Design a data structure for a Least Recently Used (LRU) cache.
- Write a function to find the kth smallest element in a binary search tree.
- Implement a function to serialize and deserialize a binary tree.
- Design a class to efficiently find the median from a stream of numbers.
System Design Questions:
- Design a real-time collaboration feature for a document editing system.
- How would you design a notification system for Asana?
- Design a system to handle millions of concurrent websocket connections.
- How would you implement a search feature for Asana that can handle large amounts of data?
- Design a system to track and display task dependencies in a project management tool.
Behavioral Questions:
- Describe a challenging project you worked on and how you overcame obstacles.
- How do you stay updated with the latest technologies and industry trends?
- Tell me about a time when you had to work with a difficult team member.
- How do you approach learning a new programming language or framework?
- Describe a situation where you had to make a trade-off between perfect code and meeting a deadline.
Practice answering these questions out loud, explaining your thought process clearly and concisely.
9. Tips for Success
To maximize your chances of success in the Asana technical interview, keep these tips in mind:
- Practice, practice, practice: Solve coding problems regularly on platforms like LeetCode, HackerRank, or AlgoCademy.
- Think out loud: Communicate your thought process clearly during the interview.
- Ask clarifying questions: Ensure you understand the problem before diving into a solution.
- Consider edge cases: Always think about potential edge cases and how to handle them.
- Optimize your solutions: Start with a working solution, then look for ways to optimize it.
- Be familiar with Asana’s products: Use Asana before the interview to understand its features and functionality.
- Prepare questions for the interviewer: This shows your genuine interest in the role and company.
- Stay calm and positive: Even if you struggle with a question, maintain a positive attitude and keep trying.
- Review your past projects: Be prepared to discuss your previous work in detail.
- Practice good coding habits: Write clean, well-commented code during the interview.
10. Additional Resources
To further prepare for your Asana technical interview, consider these resources:
- Books:
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “System Design Interview” by Alex Xu
- “Clean Code” by Robert C. Martin
- Online Platforms:
- AlgoCademy for interactive coding tutorials and interview prep
- LeetCode for coding practice
- HackerRank for coding challenges
- Websites:
- Asana Engineering Blog
- GeeksforGeeks for algorithm explanations
- HighScalability for system design articles
- YouTube Channels:
- CS Dojo for algorithm explanations
- System Design Interview for system design concepts
- TechLead for interview tips and industry insights
Remember, consistent practice and a deep understanding of fundamental concepts are key to succeeding in your Asana technical interview. Good luck with your preparation!
Conclusion
Preparing for an Asana technical interview requires dedication, practice, and a solid understanding of computer science fundamentals. By focusing on the key areas outlined in this guide, practicing with sample questions, and leveraging additional resources, you’ll be well-equipped to showcase your skills and land that dream job at Asana.
Remember that the interview process is not just about demonstrating your technical prowess, but also about showing your problem-solving approach, communication skills, and cultural fit. Stay confident, be yourself, and let your passion for technology and collaboration shine through.
As you continue your preparation, consider using platforms like AlgoCademy to further hone your skills with interactive coding tutorials and personalized interview prep. With the right preparation and mindset, you’ll be well on your way to joining the innovative team at Asana. Best of luck in your upcoming interview!