DoorDash Technical Interview Prep: A Comprehensive Guide
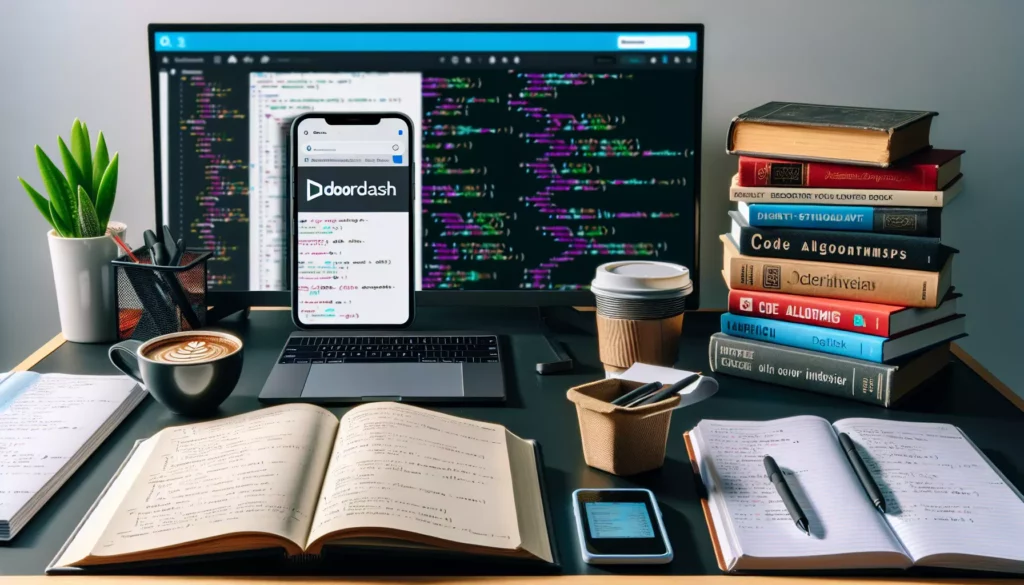
Are you gearing up for a technical interview with DoorDash? You’re in the right place! This comprehensive guide will walk you through everything you need to know to ace your DoorDash technical interview. From understanding the interview process to mastering key algorithms and data structures, we’ve got you covered. Let’s dive in and prepare you for success!
Table of Contents
- Understanding DoorDash’s Interview Process
- Key Technical Skills to Focus On
- Algorithms and Data Structures
- System Design for DoorDash’s Scale
- Coding Best Practices
- Behavioral Questions and Soft Skills
- Mock Interview Questions
- Tips for Interview Day
- Resources for Further Preparation
- Conclusion
1. Understanding DoorDash’s Interview Process
Before diving into the technical aspects, it’s crucial to understand DoorDash’s interview process. Typically, it consists of several stages:
- Initial Phone Screen: A brief call with a recruiter to discuss your background and the role.
- Technical Phone Interview: A coding interview conducted over the phone or video call, usually lasting 45-60 minutes.
- Take-home Assignment: Some positions may require a take-home coding challenge.
- On-site Interviews: A series of interviews, including coding, system design, and behavioral rounds.
Understanding this process helps you prepare effectively for each stage. Now, let’s focus on the technical aspects you need to master.
2. Key Technical Skills to Focus On
DoorDash is a technology-driven company, and they look for candidates with strong technical skills. Here are the key areas to focus on:
- Proficiency in at least one programming language (Python, Java, or JavaScript are commonly used at DoorDash)
- Strong understanding of data structures and algorithms
- Knowledge of object-oriented programming principles
- Familiarity with distributed systems and microservices architecture
- Experience with databases (both SQL and NoSQL)
- Understanding of RESTful APIs
- Version control systems (Git)
- Basic understanding of cloud services (AWS, Google Cloud, or Azure)
Make sure you’re comfortable with these concepts and can apply them in practical coding scenarios.
3. Algorithms and Data Structures
A solid grasp of algorithms and data structures is crucial for succeeding in DoorDash’s technical interviews. Here are some key topics to review:
Data Structures:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees (Binary Trees, Binary Search Trees)
- Graphs
- Hash Tables
- Heaps
Algorithms:
- Sorting (Quick Sort, Merge Sort, Heap Sort)
- Searching (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Graph Algorithms (Shortest Path, Minimum Spanning Tree)
Let’s look at a common algorithm problem you might encounter in a DoorDash interview:
Example: Find the Shortest Delivery Route
Problem: Given a list of delivery locations and their distances from each other, find the shortest route that visits all locations exactly once and returns to the starting point.
This is a variation of the Traveling Salesman Problem. While finding the optimal solution is NP-hard, you can implement a greedy approach:
def find_shortest_route(distances):
n = len(distances)
unvisited = set(range(1, n))
route = [0]
total_distance = 0
while unvisited:
last = route[-1]
next_location = min(unvisited, key=lambda x: distances[last][x])
route.append(next_location)
unvisited.remove(next_location)
total_distance += distances[last][next_location]
# Return to start
route.append(0)
total_distance += distances[route[-2]][0]
return route, total_distance
This algorithm demonstrates your ability to work with graphs and implement a greedy approach to solve a complex problem.
4. System Design for DoorDash’s Scale
DoorDash operates at a massive scale, handling millions of orders across multiple countries. Understanding how to design systems that can handle this scale is crucial. Here are some key aspects to consider:
- Scalability: How to design systems that can handle increasing loads
- Reliability: Ensuring the system remains operational even when components fail
- Availability: Designing for high uptime and minimal service interruptions
- Performance: Optimizing for speed and efficiency
- Consistency: Managing data across distributed systems
A common system design question you might encounter is:
Example: Design DoorDash’s Order Tracking System
When approaching this problem, consider the following components:
- User Interface: Mobile app and web interface for customers and dashers
- Order Service: Handles order creation, updates, and status changes
- Restaurant Service: Manages restaurant information and menu items
- Delivery Service: Assigns orders to dashers and optimizes routes
- Notification Service: Sends real-time updates to customers and dashers
- Database: Stores order information, user data, and restaurant details
- Caching Layer: Improves performance for frequently accessed data
- Load Balancer: Distributes incoming traffic across multiple servers
Here’s a high-level diagram of how these components might interact:
[User] <--> [Load Balancer] <--> [API Gateway]
|
----------------+----------------
| | |
[Order Service] [Restaurant Service] [Delivery Service]
| | |
----------------+----------------
|
[Notification Service]
|
----------------+----------------
| | |
[Database] [Cache] [Message Queue]
Be prepared to discuss trade-offs, potential bottlenecks, and how you would ensure the system’s scalability and reliability.
5. Coding Best Practices
During your DoorDash interview, it’s not just about solving the problem—it’s also about how you solve it. Here are some best practices to keep in mind:
- Write Clean Code: Use meaningful variable names, proper indentation, and follow language-specific conventions.
- Modularity: Break your code into logical functions or methods.
- Efficiency: Consider time and space complexity. Optimize where possible, but don’t sacrifice readability for minor optimizations.
- Error Handling: Consider edge cases and handle potential errors gracefully.
- Testing: Discuss how you would test your code. Consider writing unit tests if time allows.
- Documentation: Add comments to explain complex logic or assumptions.
Here’s an example of how you might apply these practices to a simple problem:
Example: Implement a Function to Find the Most Frequent Item in an Array
from typing import List
from collections import Counter
def find_most_frequent(arr: List[int]) -> int:
"""
Finds the most frequent item in the given array.
Args:
arr (List[int]): The input array of integers
Returns:
int: The most frequent item in the array
Raises:
ValueError: If the input array is empty
"""
if not arr:
raise ValueError("Input array cannot be empty")
# Use Counter to count occurrences of each item
count = Counter(arr)
# Find the item with the maximum count
most_frequent = max(count, key=count.get)
return most_frequent
# Example usage and testing
if __name__ == "__main__":
test_cases = [
[1, 2, 3, 2, 1, 2, 3, 1, 1], # Expected: 1
[1], # Expected: 1
[1, 2, 3, 4, 5], # Expected: 1 (or any, all have same frequency)
]
for i, case in enumerate(test_cases):
result = find_most_frequent(case)
print(f"Test case {i + 1}: {result}")
# Test error case
try:
find_most_frequent([])
except ValueError as e:
print(f"Error case: {str(e)}")
This example demonstrates clean code, proper documentation, error handling, and even includes a basic test suite.
6. Behavioral Questions and Soft Skills
While technical skills are crucial, DoorDash also values soft skills and cultural fit. Be prepared to answer behavioral questions that demonstrate your problem-solving abilities, teamwork, and alignment with DoorDash’s values. Some common questions include:
- Tell me about a time when you faced a significant technical challenge. How did you overcome it?
- Describe a situation where you had to work with a difficult team member. How did you handle it?
- How do you stay updated with the latest technologies and industry trends?
- Can you give an example of how you’ve contributed to improving a team’s processes or productivity?
When answering these questions, use the STAR method (Situation, Task, Action, Result) to structure your responses effectively.
7. Mock Interview Questions
To help you prepare, here are some sample technical questions you might encounter in a DoorDash interview:
- Design a system to handle real-time order tracking for millions of concurrent users.
- Implement an algorithm to optimize delivery routes for multiple orders.
- How would you design a recommendation system for suggesting restaurants to users?
- Implement a rate limiter for DoorDash’s API.
- Design a database schema for DoorDash’s core functionality (users, restaurants, orders, etc.).
- How would you handle peak load times (e.g., lunch rush) in DoorDash’s system?
- Implement a function to calculate the estimated delivery time based on various factors (distance, traffic, etc.).
Practice solving these problems and explaining your thought process clearly.
8. Tips for Interview Day
On the day of your DoorDash interview, keep these tips in mind:
- Be on Time: Set up your environment well before the interview starts.
- Test Your Tech: Ensure your internet connection, microphone, and camera are working properly.
- Have a Backup: Keep a phone nearby in case of technical issues with your primary device.
- Use a Clean Coding Environment: Have a blank IDE or coding platform ready.
- Think Aloud: Communicate your thought process as you work through problems.
- Ask Clarifying Questions: Don’t hesitate to seek clarification on requirements or constraints.
- Stay Calm: If you get stuck, take a deep breath and approach the problem step by step.
- Be Yourself: Show your personality and enthusiasm for the role and company.
9. Resources for Further Preparation
To further enhance your preparation, consider using these resources:
- LeetCode: Practice coding problems, especially those tagged as frequently asked in DoorDash interviews.
- System Design Primer: A comprehensive GitHub repository covering system design concepts.
- DoorDash Engineering Blog: Read about the technical challenges and solutions at DoorDash.
- Cracking the Coding Interview: A classic book for technical interview preparation.
- Mock Interview Platforms: Websites like Pramp or InterviewBit for realistic interview practice.
10. Conclusion
Preparing for a DoorDash technical interview requires a combination of strong coding skills, system design knowledge, and the ability to communicate your ideas effectively. By focusing on the areas we’ve covered in this guide—from algorithms and data structures to system design and soft skills—you’ll be well-equipped to tackle the challenges of the interview process.
Remember, the key to success is consistent practice and a genuine interest in solving complex problems. DoorDash is looking for candidates who are not only technically proficient but also passionate about using technology to solve real-world logistics challenges.
As you prepare, keep in mind that the interview is also an opportunity for you to learn about DoorDash and determine if it’s the right fit for your career goals. Don’t hesitate to ask questions about the team, the projects you might work on, and the company culture.
Good luck with your DoorDash technical interview! With thorough preparation and the right mindset, you’ll be well on your way to joining one of the most innovative companies in the food delivery and logistics space.