Top Pinterest Interview Questions: Ace Your Coding Interview
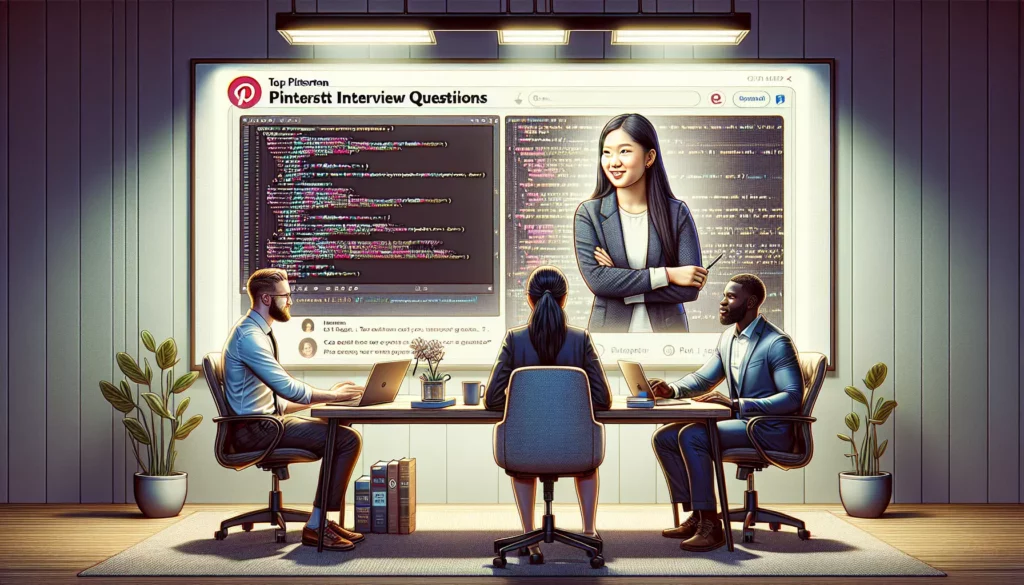
Are you preparing for a technical interview at Pinterest? You’re in the right place! In this comprehensive guide, we’ll explore some of the most common Pinterest interview questions, provide insights into the company’s interview process, and offer tips to help you succeed. Whether you’re a seasoned developer or just starting your journey in tech, this article will equip you with the knowledge and strategies needed to excel in your Pinterest interview.
Table of Contents
- Introduction to Pinterest Interviews
- The Pinterest Interview Process
- Coding Questions
- System Design Questions
- Behavioral Questions
- Tips for Success
- Conclusion
1. Introduction to Pinterest Interviews
Pinterest, the popular visual discovery platform, is known for its innovative technology and user-friendly interface. As a company that values creativity and technical excellence, Pinterest’s interview process is designed to identify candidates who can contribute to its mission of bringing everyone the inspiration to create a life they love.
The interview process at Pinterest is rigorous and comprehensive, covering various aspects of a candidate’s skills, including coding proficiency, system design knowledge, and cultural fit. By understanding the types of questions asked and the company’s expectations, you can better prepare yourself for success.
2. The Pinterest Interview Process
The Pinterest interview process typically consists of several stages:
- Initial Phone Screen: This is usually a brief conversation with a recruiter to discuss your background and interest in the role.
- Technical Phone Interview: You’ll be asked to solve coding problems in real-time, often using a shared coding environment.
- On-site Interviews: This stage includes multiple rounds of interviews, covering coding, system design, and behavioral questions.
- Final Decision: After the on-site interviews, the hiring team will make a decision based on your performance across all stages.
Now, let’s dive into the types of questions you might encounter during your Pinterest interview.
3. Coding Questions
Coding questions are a crucial part of the Pinterest interview process. These questions assess your problem-solving skills, coding ability, and knowledge of data structures and algorithms. Here are some examples of coding questions you might encounter:
3.1. Two Sum
Question: Given an array of integers and a target sum, return the indices of two numbers in the array that add up to the target sum.
Example solution:
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
# Test the function
print(two_sum([2, 7, 11, 15], 9)) # Output: [0, 1]
3.2. Implement a Queue using Stacks
Question: Implement a first-in-first-out (FIFO) queue using only two stacks. The implemented queue should support all the functions of a normal queue (enqueue, dequeue, peek, empty).
Example solution:
class MyQueue:
def __init__(self):
self.stack1 = []
self.stack2 = []
def enqueue(self, x: int) -> None:
self.stack1.append(x)
def dequeue(self) -> int:
self._transfer()
return self.stack2.pop()
def peek(self) -> int:
self._transfer()
return self.stack2[-1]
def empty(self) -> bool:
return len(self.stack1) == 0 and len(self.stack2) == 0
def _transfer(self):
if not self.stack2:
while self.stack1:
self.stack2.append(self.stack1.pop())
# Test the queue
q = MyQueue()
q.enqueue(1)
q.enqueue(2)
print(q.peek()) # Output: 1
print(q.dequeue()) # Output: 1
print(q.empty()) # Output: False
3.3. Longest Palindromic Substring
Question: Given a string s, return the longest palindromic substring in s.
Example solution:
def longest_palindrome(s: str) -> str:
if not s:
return ""
start, max_len = 0, 1
def expand_around_center(left: int, right: int) -> int:
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return right - left - 1
for i in range(len(s)):
len1 = expand_around_center(i, i)
len2 = expand_around_center(i, i + 1)
length = max(len1, len2)
if length > max_len:
start = i - (length - 1) // 2
max_len = length
return s[start:start + max_len]
# Test the function
print(longest_palindrome("babad")) # Output: "bab" or "aba"
print(longest_palindrome("cbbd")) # Output: "bb"
3.4. Merge K Sorted Lists
Question: You are given an array of k linked-lists lists, each linked-list is sorted in ascending order. Merge all the linked-lists into one sorted linked-list and return it.
Example solution:
import heapq
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def mergeKLists(lists):
dummy = ListNode(0)
current = dummy
heap = []
# Add the head of each list to the heap
for i, lst in enumerate(lists):
if lst:
heapq.heappush(heap, (lst.val, i, lst))
while heap:
val, i, node = heapq.heappop(heap)
current.next = node
current = current.next
if node.next:
heapq.heappush(heap, (node.next.val, i, node.next))
return dummy.next
# Helper function to create a linked list from a list
def create_linked_list(lst):
dummy = ListNode(0)
current = dummy
for val in lst:
current.next = ListNode(val)
current = current.next
return dummy.next
# Helper function to convert a linked list to a list
def linked_list_to_list(head):
result = []
while head:
result.append(head.val)
head = head.next
return result
# Test the function
lists = [
create_linked_list([1,4,5]),
create_linked_list([1,3,4]),
create_linked_list([2,6])
]
merged = mergeKLists(lists)
print(linked_list_to_list(merged)) # Output: [1,1,2,3,4,4,5,6]
4. System Design Questions
For more senior positions, Pinterest often includes system design questions in their interviews. These questions assess your ability to design scalable, efficient, and robust systems. Here are some examples of system design questions you might encounter:
4.1. Design Pinterest’s Feed System
Question: Design a system that generates and serves personalized content feeds for Pinterest users.
Key considerations:
- Content ingestion and storage
- User profile and interest management
- Content ranking and personalization algorithms
- Caching and content delivery
- Scalability and performance optimizations
4.2. Design a URL Shortener
Question: Design a URL shortening service similar to bit.ly.
Key considerations:
- URL encoding and decoding algorithms
- Data storage for mappings between short and long URLs
- Handling redirects
- Analytics and tracking
- Scalability and load balancing
4.3. Design a Distributed Key-Value Store
Question: Design a distributed key-value store that can handle high read and write throughput.
Key considerations:
- Data partitioning and replication
- Consistency models (e.g., eventual consistency, strong consistency)
- Handling node failures and network partitions
- Load balancing and request routing
- Scalability and performance optimizations
5. Behavioral Questions
Pinterest also values cultural fit and soft skills. You may be asked behavioral questions to assess your teamwork, problem-solving, and communication skills. Here are some examples:
- Tell me about a time when you had to work on a challenging project with a tight deadline.
- Describe a situation where you had to resolve a conflict with a coworker.
- How do you stay updated with the latest technologies and industry trends?
- Can you share an example of a time when you received constructive feedback and how you handled it?
- Describe a project you’re particularly proud of and your role in its success.
6. Tips for Success
To increase your chances of success in a Pinterest interview, consider the following tips:
- Practice coding problems: Use platforms like LeetCode, HackerRank, or AlgoCademy to practice coding questions regularly.
- Study system design: Familiarize yourself with common system design patterns and best practices.
- Understand Pinterest’s product: Use the Pinterest app and familiarize yourself with its features and functionality.
- Communicate clearly: During the interview, explain your thought process and approach to solving problems.
- Ask questions: Don’t hesitate to ask for clarification or additional information when needed.
- Review Pinterest’s values: Familiarize yourself with Pinterest’s company culture and values to align your responses in behavioral questions.
- Practice mock interviews: Conduct mock interviews with friends or use online platforms to simulate the interview experience.
- Be prepared to discuss your past projects: Have detailed examples of your previous work ready to share.
7. Conclusion
Preparing for a Pinterest interview can be challenging, but with the right approach and dedication, you can increase your chances of success. By focusing on coding proficiency, system design knowledge, and cultural fit, you’ll be well-equipped to tackle the various aspects of the interview process.
Remember that the key to success lies in consistent practice and a thorough understanding of fundamental concepts. Use the resources available to you, such as coding platforms, system design books, and mock interview tools, to refine your skills and boost your confidence.
As you prepare, keep in mind that Pinterest values innovation, creativity, and a user-centric approach. Demonstrating these qualities, along with your technical skills, will help you stand out as a strong candidate.
Good luck with your Pinterest interview! With thorough preparation and the right mindset, you’ll be well on your way to joining the team at one of the most innovative companies in the tech industry.