Top Databricks Interview Questions: Mastering the Art of Data Engineering
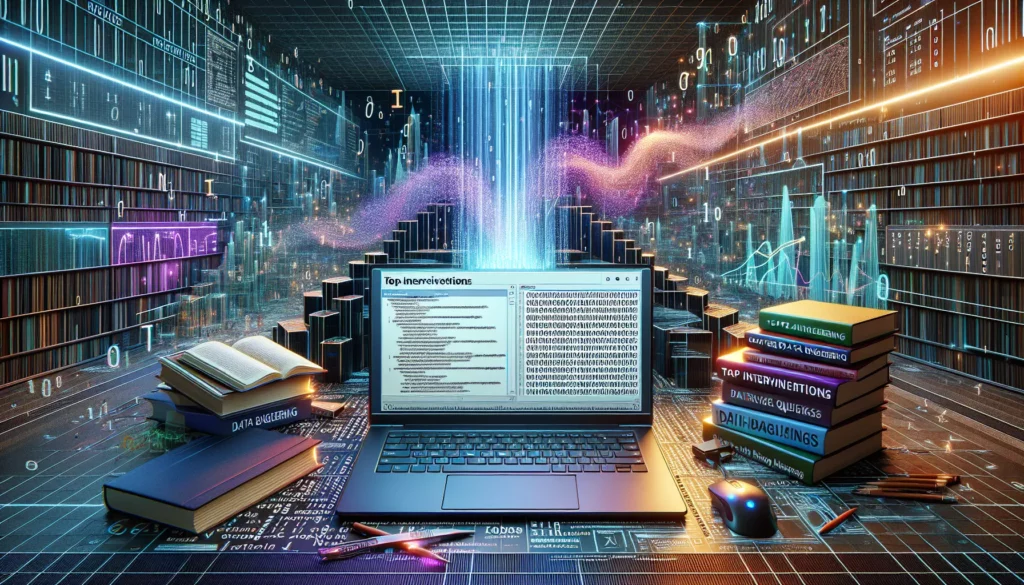
As the world of big data continues to evolve, Databricks has emerged as a leading platform for data engineering, analytics, and machine learning. With its growing popularity, many tech professionals are setting their sights on career opportunities within Databricks or companies that utilize its technology. If you’re preparing for a Databricks interview, you’ve come to the right place. In this comprehensive guide, we’ll explore the most common Databricks interview questions, providing you with the knowledge and confidence you need to ace your interview.
Table of Contents
- Introduction to Databricks
- Databricks Basics
- Apache Spark and Databricks
- Delta Lake and Data Management
- Machine Learning and AI on Databricks
- Performance Optimization
- Security and Compliance
- Integration and Ecosystem
- Real-world Use Cases
- Coding Challenges
- Conclusion
1. Introduction to Databricks
Before diving into specific questions, it’s crucial to understand what Databricks is and why it’s important in the data engineering landscape.
Q: What is Databricks, and how does it differ from traditional data processing platforms?
A: Databricks is a unified analytics platform that combines the best of data warehouses and data lakes to offer an integrated solution for big data processing and machine learning. It was founded by the creators of Apache Spark and provides a collaborative environment for data scientists, data engineers, and business analysts to work together on data projects.
Key differences from traditional platforms include:
- Seamless integration of data processing and machine learning workflows
- Built-in version control and collaboration features
- Automated cluster management and optimization
- Support for multiple programming languages (Python, R, SQL, Scala)
- Easy scalability and cloud-native architecture
Q: Can you explain the Databricks Lakehouse architecture?
A: The Databricks Lakehouse architecture is a modern data management paradigm that combines the best features of data warehouses and data lakes. It provides the following benefits:
- ACID transactions on data lakes, ensuring data consistency and reliability
- Schema enforcement and evolution, allowing for structured and semi-structured data
- Support for diverse data types and workloads (SQL analytics, streaming, machine learning)
- Direct access to source data without the need for ETL processes
- Open formats and APIs, preventing vendor lock-in
The Lakehouse architecture is built on top of open-source technologies like Apache Spark and Delta Lake, providing a unified platform for data management and analytics.
2. Databricks Basics
Understanding the fundamental concepts and components of Databricks is crucial for any interview. Let’s explore some common questions in this area.
Q: What are the main components of the Databricks platform?
A: The main components of the Databricks platform include:
- Databricks Workspace: A collaborative environment for data teams to create, share, and manage notebooks, dashboards, and ML models.
- Databricks Runtime: An optimized version of Apache Spark with additional libraries and performance improvements.
- Delta Lake: An open-source storage layer that brings reliability to data lakes.
- MLflow: An open-source platform for managing the machine learning lifecycle.
- Databricks SQL: A SQL-native tool for running queries, creating visualizations, and building dashboards.
- Databricks Clusters: Managed Spark clusters that can be easily scaled up or down based on workload requirements.
- Databricks Jobs: A scheduling and orchestration service for running production workflows.
Q: How does Databricks handle data storage?
A: Databricks uses a multi-tiered approach to data storage:
- DBFS (Databricks File System): A distributed file system that’s mounted on Databricks clusters and provides a unified view of data stored in various locations.
- Cloud Object Storage: Databricks can directly access and process data stored in cloud object storage services like Amazon S3, Azure Blob Storage, or Google Cloud Storage.
- Delta Lake: An open-source storage layer that provides ACID transactions, scalable metadata handling, and unifies streaming and batch data processing.
- Managed Tables: Databricks can create and manage tables that are stored in a highly optimized format, providing better performance and ease of use.
This flexible approach allows Databricks to work with data in various formats and locations, providing a unified analytics experience.
3. Apache Spark and Databricks
Since Databricks is built on top of Apache Spark, a solid understanding of Spark concepts is essential for any Databricks interview.
Q: What are the main differences between Apache Spark on Databricks and standalone Apache Spark?
A: While Databricks is built on Apache Spark, it offers several enhancements and optimizations:
- Optimized Runtime: Databricks Runtime includes performance improvements and additional libraries that can make Spark jobs run up to 50x faster.
- Automatic Cluster Management: Databricks handles cluster provisioning, scaling, and termination automatically, simplifying infrastructure management.
- Collaborative Environment: Databricks provides a web-based notebook interface for interactive development and collaboration.
- Delta Lake Integration: Built-in support for Delta Lake, providing ACID transactions and improved data reliability.
- MLflow Integration: Native support for MLflow, simplifying the machine learning lifecycle.
- Security Features: Enhanced security controls, including fine-grained access control and encryption.
- Managed Services: Databricks offers managed services like Delta Engine and Photon, which further optimize query performance.
Q: Can you explain the concept of Spark DataFrames and how they are used in Databricks?
A: Spark DataFrames are distributed collections of data organized into named columns, similar to tables in a relational database. In Databricks, DataFrames are a fundamental data structure for processing structured and semi-structured data. Key points about DataFrames in Databricks include:
- They provide a domain-specific language for structured data manipulation.
- DataFrames are immutable and lazily evaluated, allowing Spark to optimize query execution.
- They support various data sources, including CSV, JSON, Parquet, and Delta Lake tables.
- DataFrames can be manipulated using SQL queries or DataFrame API operations.
- They are optimized for performance through features like the Catalyst optimizer and whole-stage code generation.
Here’s a simple example of creating and manipulating a DataFrame in Databricks using PySpark:
# Create a DataFrame from a list of tuples
data = [("Alice", 25), ("Bob", 30), ("Charlie", 35)]
df = spark.createDataFrame(data, ["name", "age"])
# Show the DataFrame
df.show()
# Filter and select operations
result = df.filter(df.age > 28).select("name")
result.show()
4. Delta Lake and Data Management
Delta Lake is a key component of the Databricks ecosystem, providing reliable data management capabilities for data lakes.
Q: What is Delta Lake, and what problems does it solve?
A: Delta Lake is an open-source storage layer that brings ACID transactions to Apache Spark and big data workloads. It addresses several challenges associated with traditional data lakes:
- Data Reliability: Delta Lake ensures data consistency through ACID transactions, preventing data corruption during concurrent writes.
- Schema Evolution: It allows for easy schema changes without the need to rewrite entire tables.
- Time Travel: Delta Lake maintains a history of data changes, enabling data versioning and the ability to query data as it existed at a specific point in time.
- Unified Batch and Streaming: It provides a single API for both batch and streaming data processing, simplifying data pipelines.
- Data Quality: Delta Lake enforces schema validation, ensuring that only data matching the defined schema is written to tables.
- Performance Optimization: It includes features like data skipping and Z-Order clustering to improve query performance.
Q: How would you implement a slowly changing dimension (SCD) Type 2 in Databricks using Delta Lake?
A: Implementing a slowly changing dimension (SCD) Type 2 in Databricks using Delta Lake involves tracking historical changes to dimension data. Here’s a high-level approach:
- Create a Delta table with additional columns for tracking changes (e.g., effective_date, end_date, is_current).
- When updating the dimension, identify changed records.
- For changed records, update the existing row by setting end_date and is_current.
- Insert new rows for the changed records with updated information and new effective dates.
- Use merge operation to perform both updates and inserts in a single transaction.
Here’s a simplified example in PySpark:
# Assume we have a customer dimension table
from pyspark.sql.functions import *
# New data to be merged
new_data = spark.createDataFrame([
(1, "John Doe", "New York"),
(2, "Jane Smith", "Los Angeles")
], ["customer_id", "name", "city"])
# Merge statement
merge_statement = (
delta_table.alias("target")
.merge(
new_data.alias("source"),
"target.customer_id = source.customer_id"
)
.whenMatchedUpdate(
condition = "target.name != source.name OR target.city != source.city",
set = {
"end_date": "current_date()",
"is_current": "false"
}
)
.whenNotMatchedInsert(
values = {
"customer_id": "source.customer_id",
"name": "source.name",
"city": "source.city",
"effective_date": "current_date()",
"end_date": "null",
"is_current": "true"
}
)
.execute()
)
# Insert new rows for changed records
changed_records = delta_table.toDF().join(
new_data,
"customer_id"
).filter(
"target.name != source.name OR target.city != source.city"
)
changed_records.select(
"source.customer_id",
"source.name",
"source.city",
current_date().alias("effective_date"),
lit(None).alias("end_date"),
lit(True).alias("is_current")
).write.mode("append").saveAsTable("customer_dimension")
This example demonstrates how to use Delta Lake’s merge operation to implement SCD Type 2, ensuring that historical changes are tracked while maintaining data consistency.
5. Machine Learning and AI on Databricks
Databricks provides robust support for machine learning and AI workflows. Understanding these capabilities is crucial for data scientists and ML engineers working with the platform.
Q: How does Databricks support the machine learning lifecycle?
A: Databricks supports the entire machine learning lifecycle through several integrated components and features:
- Data Preparation:
- Use Spark DataFrames and SQL for data cleaning and feature engineering
- Leverage Delta Lake for reliable and versioned datasets
- Model Development:
- Support for popular ML libraries like scikit-learn, TensorFlow, and PyTorch
- Integration with Spark MLlib for distributed machine learning
- Notebook environment for interactive development and experimentation
- Model Tracking and Management:
- MLflow integration for experiment tracking, model versioning, and model registry
- Reproducibility through versioned notebooks and MLflow runs
- Model Deployment:
- Model serving capabilities for real-time and batch inference
- Integration with cloud-specific deployment options (e.g., Azure ML, AWS SageMaker)
- Model Monitoring and Maintenance:
- Databricks Jobs for scheduling model retraining and evaluation
- Integration with monitoring tools for tracking model performance in production
Q: Can you explain how to use MLflow in Databricks for experiment tracking and model management?
A: MLflow is deeply integrated into Databricks, providing powerful capabilities for managing the machine learning lifecycle. Here’s how to use MLflow in Databricks:
- Experiment Tracking:
- Use
mlflow.start_run()
to begin tracking an experiment - Log parameters, metrics, and artifacts using MLflow’s logging functions
- Experiments are automatically organized by notebook
- Use
- Model Packaging:
- Use MLflow’s model flavors to package models in a standardized format
- Log models using
mlflow.<framework>.log_model()
functions
- Model Registry:
- Register models in the MLflow Model Registry for versioning and stage transitions
- Use the Databricks UI or MLflow API to manage model versions and stages
- Model Serving:
- Deploy models directly from the Model Registry for real-time serving
- Use MLflow’s deployment tools for batch inference
Here’s a simple example of using MLflow in a Databricks notebook:
import mlflow
import mlflow.sklearn
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Load and prepare data
data = spark.table("my_dataset").toPandas()
X = data.drop("target", axis=1)
y = data["target"]
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
# Start an MLflow run
with mlflow.start_run():
# Set parameters
n_estimators = 100
max_depth = 5
mlflow.log_param("n_estimators", n_estimators)
mlflow.log_param("max_depth", max_depth)
# Train model
rf = RandomForestClassifier(n_estimators=n_estimators, max_depth=max_depth)
rf.fit(X_train, y_train)
# Log metrics
predictions = rf.predict(X_test)
accuracy = accuracy_score(y_test, predictions)
mlflow.log_metric("accuracy", accuracy)
# Log model
mlflow.sklearn.log_model(rf, "random_forest_model")
# Register the model
model_uri = f"runs:/{mlflow.active_run().info.run_id}/random_forest_model"
registered_model = mlflow.register_model(model_uri, "MyRandomForestModel")
This example demonstrates how to use MLflow to track experiments, log parameters and metrics, save models, and register them in the Model Registry, all within the Databricks environment.
6. Performance Optimization
Optimizing performance is crucial when working with big data. Databricks offers various tools and techniques to enhance query and job performance.
Q: What are some strategies for optimizing Spark job performance in Databricks?
A: There are several strategies to optimize Spark job performance in Databricks:
- Data Partitioning:
- Partition data based on frequently filtered columns
- Use appropriate partition sizes to avoid small file problems
- Caching:
- Cache frequently used DataFrames or tables using
cache()
orpersist()
- Use appropriate storage levels based on memory availability and access patterns
- Cache frequently used DataFrames or tables using
- Broadcast Joins:
- Use broadcast joins for small tables to reduce shuffle operations
- Explicitly hint broadcast joins using
broadcast()
function
- Avoid Skew:
- Identify and handle skewed data to prevent bottlenecks
- Use salting techniques or adaptive query execution to mitigate skew
- Optimize File Formats:
- Use columnar formats like Parquet or ORC for better compression and query performance
- Leverage Delta Lake for improved read and write performance
- Tune Spark Configurations:
- Adjust executor and driver memory settings
- Configure appropriate parallelism using
spark.default.parallelism
andspark.sql.shuffle.partitions
- Use Databricks Runtime Features:
- Enable Photon engine for query acceleration
- Utilize Adaptive Query Execution for dynamic query optimization
Q: How can you diagnose and resolve performance issues in a Databricks notebook?
A: To diagnose and resolve performance issues in a Databricks notebook, you can follow these steps:
- Use the Spark UI:
- Access the Spark UI through the cluster’s “Spark UI” tab
- Analyze job stages, tasks, and executor statistics
- Identify bottlenecks, data skew, and resource utilization issues
- Leverage Ganglia Metrics:
- Monitor cluster-wide resource utilization
- Identify memory pressure or CPU bottlenecks
- Use Explain Plans:
- Generate query explain plans using
df.explain()
orEXPLAIN
SQL command - Analyze logical and physical plans to identify optimization opportunities
- Generate query explain plans using
- Profile Code Execution:
- Use
%%timeit
magic command to measure cell execution time - Employ Python profiling tools like
cProfile
for detailed analysis
- Use
- Check Query History:
- Review the Query History in the Databricks SQL dashboard
- Analyze query execution times and resource consumption
- Optimize Data Access Patterns:
- Use appropriate file formats and compression
- Implement partitioning and bucketing strategies
- Tune Spark Configurations:
- Adjust memory settings, parallelism, and shuffle partitions
- Use cluster-specific configurations for optimal performance
Here’s an example of how to generate an explain plan and profile code execution in a Databricks notebook:
# Generate explain plan
df = spark.table("my_large_table")
filtered_df = df.filter(df.column > 100).groupBy("category").agg({"value": "sum"})
filtered_df.explain(mode="extended")
# Profile code execution
%%timeit
result = filtered_df.collect()
# Use Delta Lake optimization features
from delta.tables import *
delta_table = DeltaTable.forPath(spark, "/path/to/delta/table")
delta_table.optimize().executeCompaction()
By following these steps and using the provided tools, you can effectively diagnose and resolve performance issues in Databricks notebooks, ensuring optimal execution of your data processing and analytics tasks.
7. Security and Compliance
Security is a critical aspect of any data platform, especially when dealing with sensitive information. Databricks provides robust security features to protect data and ensure compliance with various regulations.
Q: What security features does Databricks offer, and how would you implement them?
A: Databricks offers a comprehensive set of security features to protect data and ensure compliance. Here are some key security features and how to implement them:
- Access Control:
- Use Role-Based Access Control (RBAC) to manage user permissions
- Implement Table Access Control (TAC) for fine-grained access to tables and views
- Utilize Cluster Access Control to restrict user access to specific clusters
- Data Encryption:
- Enable encryption at rest for DBFS and cloud storage
- Use encryption in transit with TLS/SSL
- Implement customer-managed keys for additional control
- Network Security:
- Configure IP access lists to restrict access to Databricks workspaces
- Use Private Link (AWS) or Private Endpoint (Azure) for secure connectivity
- Implement VPC peering or VNet peering for secure communication with other resources
- Audit Logging:
- Enable workspace audit logs to track user activities
- Use cluster log delivery to capture cluster-specific logs
- Secrets Management:
- Utilize Databricks Secrets to securely store and access sensitive information
- Integrate with Azure Key Vault or AWS Secrets Manager for centralized secrets management
- Compliance:
- Leverage Databricks’ compliance certifications (e.g., SOC 2, HIPAA, GDPR)
- Implement data retention policies and access controls to meet regulatory requirements
Here’s an example of how to implement some of these security features:
# Enable Table Access Control
%sql
ALTER TABLE my_sensitive_table
SET TBLPROPERTIES ('delta.columnMapping.mode' = 'name', 'delta.minReaderVersion' = '2', 'delta.minWriterVersion' = '5')
# Grant access to specific users or groups
%sql
GRANT SELECT ON TABLE my_sensitive_table TO `user@example.com`
GRANT MODIFY ON TABLE my_sensitive_table TO `data_engineers`
# Use Databricks Secrets to securely access sensitive information
from pyspark.dbutils import DBUtils
dbutils = DBUtils(spark)
secret_value = dbutils.secrets.get(scope="my-secret-scope", key="my-secret-key")
# Configure IP access list (Admin Console)
# Navigate to Admin Console > Network > IP Access Lists
# Add allowed IP ranges or CIDR blocks
# Enable audit logging (Admin Console)
# Navigate to Admin Console > Workspace Settings
# Enable "Audit Logs" and configure delivery settings
Q: How does Databricks ensure compliance with data protection regulations like GDPR or CCPA?
A: Databricks provides several features and practices to help organizations comply with data protection regulations like GDPR (General Data Protection Regulation) or CCPA (California Consumer Privacy Act):
- Data Governance:
- Use Delta Lake to implement time travel and data versioning
- Leverage Table Access Control for fine-grained access management
- Implement data cataloging and lineage tracking
- Data Encryption:
- Ensure data is encrypted at rest and in transit
- Use customer-managed keys for additional control over encryption
- Access Controls:
- Implement Role-Based Access Control (RBAC) to restrict data access
- Use Column-Level Security to mask or restrict access to sensitive columns
- Audit Logging:
- Enable comprehensive audit logging to track data access and modifications
- Use log analytics tools to monitor compliance-related events
- Data Retention and Deletion:
- Implement data retention policies using Delta Lake’s time travel feature
- Use VACUUM command to permanently delete data when required
- Data Subject Rights:
- Develop processes to handle data subject access requests (DSARs)
- Implement data portability features using Databricks export capabilities
- Data Processing Agreements:
- Databricks provides Data Processing Addendums (DPAs) for GDPR compliance
- Ensure all third-party integrations have appropriate data processing agreements
Here’s an example of implementing some compliance-related features in Databricks:
# Implement data retention policy using Delta Lake
from delta.tables import *
# Set retention period for a Delta table
delta_table = DeltaTable.forPath(spark, "/path/to/delta/table")
delta_table.vacuum(retention_hours=168) # 7 days retention
# Implement column-level security
from pyspark.sql.functions import when, col
def mask_sensitive_data(df, sensitive_columns):
for column in sensitive_columns:
df = df.withColumn(column, when(col("user_role") == "admin", col(column)).otherwise("*****"))
return df
sensitive_df = mask_sensitive_data(original_df, ["email", "phone_number"])
# Handle data subject access request
def handle_dsar(user_id):
user_data = spark.sql(f"SELECT * FROM user_data WHERE user_id = '{user_id}'")
user_data.write.format("json").save(f"/path/to/dsar_exports/{user_id}")
# Delete user data (implement right to be forgotten)
def delete_user_data(user_id):
spark.sql(f"DELETE FROM user_data WHERE user_id = '{user_id}'")
spark.sql(f"DELETE FROM user_activity WHERE user_id = '{user_id}'")
# Use VACUUM to permanently remove deleted data
delta_table = DeltaTable.forPath(spark, "/path/to/user_data")
delta_table.vacuum(0) # Immediately remove deleted files
By implementing these features and following best practices, organizations can use Databricks to process and manage data in compliance with regulations like GDPR and CCPA. It’s important to note that compliance is an ongoing process that requires regular audits, updates to policies and procedures, and staying informed about changes in regulations.
8. Integration and Ecosystem
Databricks integrates with a wide range of data sources, tools, and services. Understanding these integrations is crucial for building comprehensive data solutions.
Q: How does Databricks integrate with other data tools and services in the cloud ecosystem?
A: Databricks offers extensive integration capabilities with various data tools and services across major cloud platforms. Here are some key integrations:
- Data Storage:
- Native integration with cloud object storage (S3, Azure Blob Storage, Google Cloud Storage)
- Support for HDFS-compatible file systems
- Data Warehouses:
- Integration with Snowflake, Redshift, BigQuery, and Azure Synapse Analytics
- Support for federated queries across data sources