Mastering Palantir Interview Questions: A Comprehensive Guide
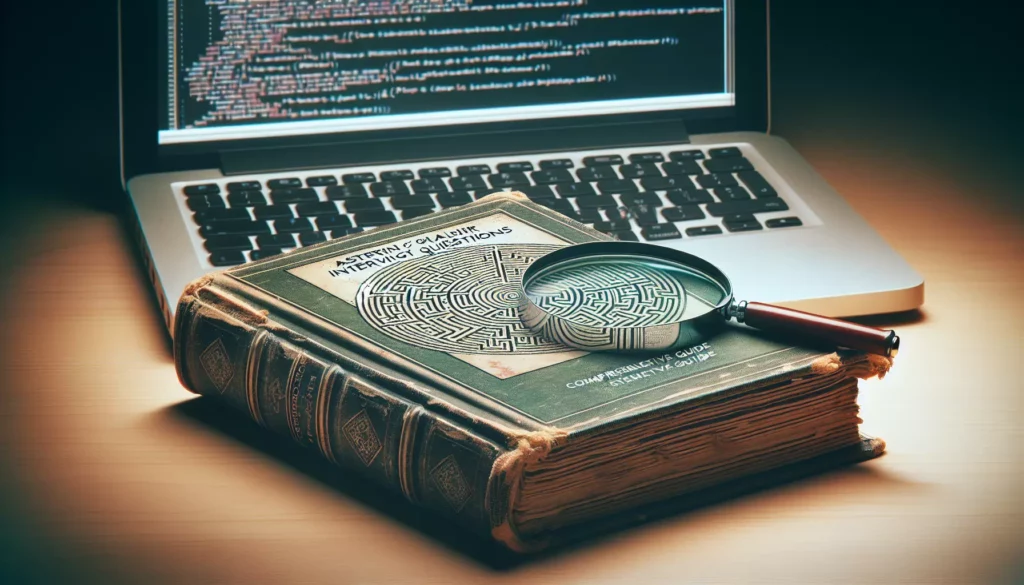
Are you preparing for a technical interview at Palantir? You’re in the right place! This comprehensive guide will walk you through the types of questions you might encounter, provide strategies for success, and offer insights into what Palantir is looking for in candidates. Whether you’re a seasoned programmer or just starting your journey in tech, this article will help you navigate the challenging waters of Palantir’s interview process.
Table of Contents
- Understanding Palantir: Company Overview
- The Palantir Interview Process
- Common Technical Questions
- Coding Challenges and Problem-Solving
- System Design Questions
- Behavioral and Cultural Fit Questions
- Preparation Tips and Strategies
- Additional Resources
- Conclusion
1. Understanding Palantir: Company Overview
Before diving into the interview questions, it’s crucial to understand what Palantir does and what they value. Palantir Technologies is a software company that specializes in big data analytics. They work with government agencies, financial institutions, and other organizations to help them make sense of large, complex datasets.
Key points about Palantir:
- Founded in 2003 by Peter Thiel, Nathan Gettings, Joe Lonsdale, Stephen Cohen, and Alex Karp
- Focuses on data integration, information management, and analytics
- Known for their two main software platforms: Palantir Gotham and Palantir Foundry
- Emphasizes privacy and civil liberties in their approach to data analysis
Understanding these aspects of Palantir will help you align your responses and demonstrate your interest in the company during the interview process.
2. The Palantir Interview Process
The interview process at Palantir is known to be rigorous and comprehensive. It typically consists of several stages:
- Initial Phone Screen: A brief conversation with a recruiter to discuss your background and interest in Palantir.
- Technical Phone Interview: A more in-depth discussion of your technical skills, often including coding questions.
- Take-Home Assignment: Some candidates may be asked to complete a coding project or problem-solving task.
- On-Site Interviews: A series of face-to-face interviews (or virtual equivalents) that include:
- Technical interviews with coding challenges
- System design discussions
- Behavioral and cultural fit interviews
- Final Decision: The hiring committee reviews all feedback to make a decision.
Each stage is designed to assess different aspects of your skills, knowledge, and fit for the role and company culture.
3. Common Technical Questions
Palantir’s technical questions often focus on fundamental computer science concepts, data structures, and algorithms. Here are some areas you should be well-versed in:
Data Structures
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
Algorithms
- Sorting and Searching
- Depth-First Search (DFS) and Breadth-First Search (BFS)
- Dynamic Programming
- Greedy Algorithms
- Recursion and Backtracking
Example Question: Implement a Trie (Prefix Tree)
Here’s an example of a technical question you might encounter:
Question: Implement a trie data structure with insert, search, and startsWith methods.
Solution:
class TrieNode:
def __init__(self):
self.children = {}
self.is_end_of_word = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word: str) -> None:
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end_of_word = True
def search(self, word: str) -> bool:
node = self.root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_end_of_word
def startsWith(self, prefix: str) -> bool:
node = self.root
for char in prefix:
if char not in node.children:
return False
node = node.children[char]
return True
This implementation demonstrates knowledge of tree-like data structures and efficient string operations, both of which are important in Palantir’s work with large datasets.
4. Coding Challenges and Problem-Solving
Palantir places a strong emphasis on problem-solving skills. During the interview, you may be presented with complex scenarios that require you to think critically and write efficient code. Here are some tips for approaching coding challenges:
- Clarify the problem: Ask questions to ensure you fully understand the requirements.
- Think out loud: Share your thought process as you work through the problem.
- Consider edge cases: Discuss potential edge cases and how your solution handles them.
- Optimize: After implementing a working solution, discuss potential optimizations.
- Test your code: Walk through your code with sample inputs to demonstrate its correctness.
Example Problem: Find the Kth Largest Element
Question: Given an unsorted array of integers, find the kth largest element in the array.
Solution:
import heapq
def findKthLargest(nums: List[int], k: int) -> int:
heap = []
for num in nums:
heapq.heappush(heap, num)
if len(heap) > k:
heapq.heappop(heap)
return heap[0]
This solution uses a min-heap to efficiently find the kth largest element. It demonstrates knowledge of heap data structures and their application in solving real-world problems.
5. System Design Questions
For more senior positions or roles that involve architecture, you may encounter system design questions. These assess your ability to design scalable, efficient, and robust systems. Some topics to prepare for include:
- Distributed systems
- Database design and scaling
- Caching strategies
- Load balancing
- Microservices architecture
Example System Design Question
Question: Design a system to handle real-time analytics for a social media platform with millions of active users.
When approaching this question, consider the following aspects:
- Data ingestion: How will you collect and process incoming data?
- Storage: What type of database(s) will you use to store the data?
- Processing: How will you handle real-time computations?
- Scalability: How will your system scale to handle increasing load?
- Fault tolerance: How will you ensure the system remains available in case of failures?
A high-level solution might involve using Apache Kafka for data ingestion, Apache Spark for real-time processing, and a combination of relational and NoSQL databases for storage. Discuss the trade-offs of different architectural choices and be prepared to dive into specific components if asked.
6. Behavioral and Cultural Fit Questions
Palantir places a strong emphasis on cultural fit. They look for candidates who align with their values and can work effectively in their environment. Some common behavioral questions include:
- Tell me about a time when you had to work on a challenging project. How did you approach it?
- How do you handle disagreements with team members?
- Describe a situation where you had to learn a new technology quickly. How did you go about it?
- What interests you about working at Palantir?
- How do you stay updated with the latest developments in technology?
When answering these questions, use the STAR method (Situation, Task, Action, Result) to structure your responses. Be specific and provide concrete examples from your past experiences.
7. Preparation Tips and Strategies
To maximize your chances of success in a Palantir interview, consider the following preparation strategies:
- Review Computer Science Fundamentals: Brush up on data structures, algorithms, and system design concepts.
- Practice Coding: Solve problems on platforms like LeetCode, HackerRank, or CodeSignal regularly.
- Mock Interviews: Conduct mock interviews with friends or use services like Pramp to get comfortable with the interview format.
- Study Palantir: Research the company, its products, and recent news to demonstrate genuine interest during the interview.
- Improve Communication Skills: Practice explaining your thought process clearly and concisely.
- Prepare Questions: Have thoughtful questions ready to ask your interviewers about the company and the role.
Sample Study Plan
Here’s a sample 4-week study plan to help you prepare:
- Week 1: Review data structures and basic algorithms. Solve 2-3 easy problems daily.
- Week 2: Focus on more advanced algorithms (e.g., dynamic programming, graph algorithms). Solve 2-3 medium problems daily.
- Week 3: Study system design concepts and practice designing scalable systems. Solve 1-2 hard problems daily.
- Week 4: Conduct mock interviews, review behavioral questions, and research Palantir extensively.
8. Additional Resources
To further aid your preparation, consider the following resources:
- Books:
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “System Design Interview” by Alex Xu
- “Designing Data-Intensive Applications” by Martin Kleppmann
- Online Platforms:
- LeetCode
- HackerRank
- AlgoExpert
- Courses:
- MIT OpenCourseWare – Introduction to Algorithms
- Coursera – Algorithms Specialization by Stanford University
- Palantir-Specific Resources:
- Palantir’s official website and blog
- Glassdoor interview experiences for Palantir
9. Conclusion
Preparing for a Palantir interview can be challenging, but with the right approach and dedication, you can significantly improve your chances of success. Remember that Palantir is not just looking for technical skills, but also for problem-solvers who can think critically and communicate effectively.
Key takeaways:
- Master fundamental data structures and algorithms
- Practice solving complex problems and explaining your thought process
- Understand system design principles for scalable applications
- Prepare for behavioral questions and demonstrate cultural fit
- Stay updated on Palantir’s work and the broader tech industry
By following the strategies and tips outlined in this guide, you’ll be well-equipped to tackle Palantir’s interview process. Remember to stay calm, think methodically, and showcase your passion for technology and problem-solving. Good luck with your interview!