Top Dropbox Interview Questions: Ace Your Technical Interview
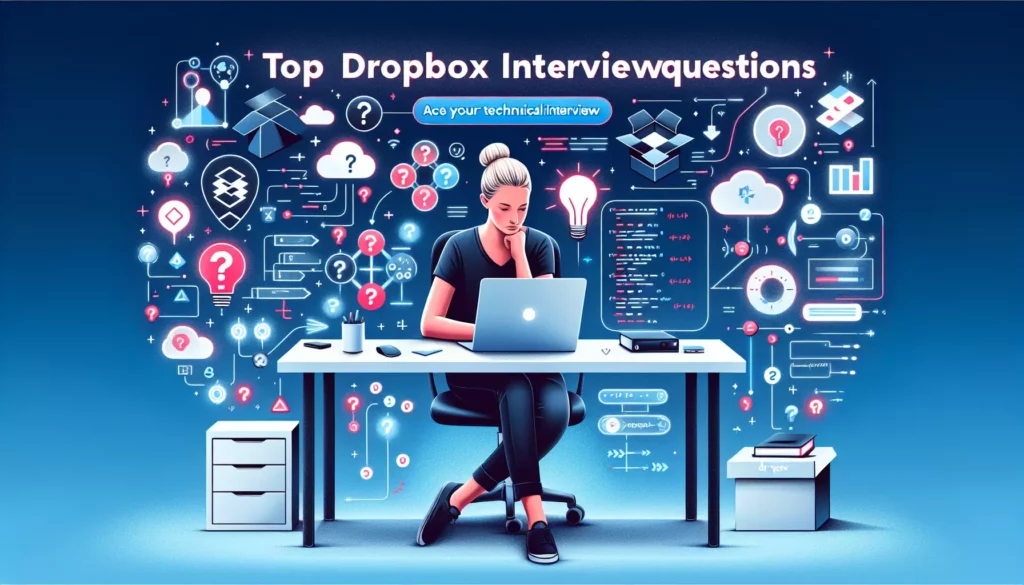
Are you preparing for a technical interview at Dropbox? You’ve come to the right place! In this comprehensive guide, we’ll dive deep into the most common Dropbox interview questions, provide insights into the company’s interview process, and offer valuable tips to help you succeed. Whether you’re a seasoned software engineer or a fresh graduate, this article will equip you with the knowledge and strategies needed to tackle Dropbox’s challenging interview questions.
Table of Contents
- Understanding Dropbox: Company Overview
- The Dropbox Interview Process
- Coding and Algorithm Questions
- System Design Questions
- Behavioral Questions
- Tips for Success
- How to Prepare
- Conclusion
1. Understanding Dropbox: Company Overview
Before diving into the interview questions, it’s crucial to understand Dropbox as a company. Dropbox is a file hosting service that offers cloud storage, file synchronization, and client software. Founded in 2007, it has grown to become one of the leading cloud storage providers, serving both individual users and businesses.
Key aspects of Dropbox:
- Focus on simplicity and ease of use
- Strong emphasis on security and privacy
- Continuous innovation in file sharing and collaboration
- Large-scale distributed systems
Understanding these core aspects will help you align your answers with Dropbox’s values and technical challenges during the interview.
2. The Dropbox Interview Process
The Dropbox interview process typically consists of several stages:
- Initial Phone Screen: A brief conversation with a recruiter to assess your background and interest in the role.
- Technical Phone Interview: A coding interview conducted over the phone or video call, usually lasting 45-60 minutes.
- On-site Interviews: A series of 4-5 interviews, including coding, system design, and behavioral questions.
- Final Decision: The hiring committee reviews all feedback and makes a decision.
Now, let’s explore the types of questions you might encounter in each stage of the interview process.
3. Coding and Algorithm Questions
Dropbox places a strong emphasis on coding skills and algorithmic thinking. Here are some common types of coding questions you might encounter, along with examples:
3.1 Array and String Manipulation
Question: Implement a function to reverse a string in-place.
Solution:
def reverse_string(s):
left, right = 0, len(s) - 1
s = list(s)
while left < right:
s[left], s[right] = s[right], s[left]
left += 1
right -= 1
return ''.join(s)
# Example usage
print(reverse_string("hello")) # Output: "olleh"
3.2 Linked Lists
Question: Detect if a linked list has a cycle.
Solution:
class ListNode:
def __init__(self, x):
self.val = x
self.next = None
def has_cycle(head):
if not head or not head.next:
return False
slow = head
fast = head.next
while slow != fast:
if not fast or not fast.next:
return False
slow = slow.next
fast = fast.next.next
return True
# Example usage
# Create a linked list with a cycle
node1 = ListNode(1)
node2 = ListNode(2)
node3 = ListNode(3)
node4 = ListNode(4)
node1.next = node2
node2.next = node3
node3.next = node4
node4.next = node2 # Create cycle
print(has_cycle(node1)) # Output: True
3.3 Trees and Graphs
Question: Implement a function to find the lowest common ancestor of two nodes in a binary tree.
Solution:
class TreeNode:
def __init__(self, x):
self.val = x
self.left = None
self.right = None
def lowest_common_ancestor(root, p, q):
if not root or root == p or root == q:
return root
left = lowest_common_ancestor(root.left, p, q)
right = lowest_common_ancestor(root.right, p, q)
if left and right:
return root
return left if left else right
# Example usage
# Create a binary tree
root = TreeNode(3)
root.left = TreeNode(5)
root.right = TreeNode(1)
root.left.left = TreeNode(6)
root.left.right = TreeNode(2)
root.right.left = TreeNode(0)
root.right.right = TreeNode(8)
p = root.left # Node with value 5
q = root.right # Node with value 1
lca = lowest_common_ancestor(root, p, q)
print(lca.val) # Output: 3
3.4 Dynamic Programming
Question: Given a set of non-negative integers and a target sum, determine if there is a subset of the given set with a sum equal to the target sum.
Solution:
def subset_sum(nums, target):
n = len(nums)
dp = [[False] * (target + 1) for _ in range(n + 1)]
for i in range(n + 1):
dp[i][0] = True
for i in range(1, n + 1):
for j in range(1, target + 1):
if nums[i - 1] <= j:
dp[i][j] = dp[i - 1][j - nums[i - 1]] or dp[i - 1][j]
else:
dp[i][j] = dp[i - 1][j]
return dp[n][target]
# Example usage
nums = [3, 34, 4, 12, 5, 2]
target = 9
print(subset_sum(nums, target)) # Output: True
4. System Design Questions
For more senior positions, Dropbox often asks system design questions to assess your ability to design large-scale distributed systems. Here are some examples:
4.1 Design a File Sharing System
Question: Design a file sharing system similar to Dropbox.
Key points to consider:
- File storage and retrieval
- Synchronization across devices
- Sharing and collaboration features
- Security and access control
- Scalability and performance
High-level approach:
- Design the client-side application for different platforms (desktop, mobile, web)
- Implement a server-side API for handling file operations and metadata
- Use a distributed file system for storing actual file content
- Implement a notification system for real-time updates
- Design a database schema for storing user information, file metadata, and sharing permissions
- Implement caching mechanisms for frequently accessed files and metadata
- Design a robust authentication and authorization system
4.2 Design a Web Crawler
Question: Design a web crawler that can efficiently crawl and index billions of web pages.
Key points to consider:
- Scalability and parallelization
- Politeness and respect for robots.txt
- Handling duplicate content
- Prioritizing important pages
- Storing and indexing crawled data
High-level approach:
- Design a distributed architecture with multiple crawler nodes
- Implement a URL frontier to manage the list of URLs to be crawled
- Use a distributed queue system for coordinating work between crawler nodes
- Implement a content parser and extractor
- Design a storage system for crawled data (e.g., distributed file system + database)
- Implement a scheduling system to respect crawl delays and politeness policies
- Design an indexing system for efficient search and retrieval of crawled content
5. Behavioral Questions
Dropbox also assesses candidates’ soft skills and cultural fit through behavioral questions. Here are some examples:
- Tell me about a time when you had to work on a challenging project. How did you approach it?
- Describe a situation where you had to resolve a conflict with a coworker.
- How do you stay updated with the latest technologies and industry trends?
- Can you share an example of when you had to explain a complex technical concept to a non-technical person?
- Tell me about a time when you received critical feedback. How did you respond?
When answering behavioral questions, use the STAR method (Situation, Task, Action, Result) to structure your responses and provide concrete examples from your past experiences.
6. Tips for Success
To increase your chances of success in a Dropbox interview, consider the following tips:
- Practice coding problems: Regularly solve coding problems on platforms like LeetCode, HackerRank, or CodeSignal.
- Study system design: Familiarize yourself with system design principles and practice designing large-scale systems.
- Understand Dropbox’s technology stack: Research the technologies and tools used by Dropbox to show your genuine interest in the company.
- Communicate clearly: Explain your thought process and approach to problem-solving throughout the interview.
- Ask clarifying questions: Don’t hesitate to ask for more information or clarification when needed.
- Test your code: Always test your code with different input cases and edge scenarios.
- Be prepared to optimize: After solving a problem, think about ways to optimize your solution for better time or space complexity.
- Show enthusiasm: Demonstrate your passion for technology and your interest in working at Dropbox.
7. How to Prepare
To effectively prepare for your Dropbox interview, follow these steps:
- Review core computer science concepts: Brush up on data structures, algorithms, and system design principles.
- Practice coding problems: Aim to solve at least 2-3 coding problems daily, focusing on different types of questions.
- Mock interviews: Participate in mock interviews with friends or use platforms like Pramp to simulate real interview conditions.
- Study Dropbox’s products and technologies: Familiarize yourself with Dropbox’s offerings and the technical challenges they face.
- Prepare your own questions: Have thoughtful questions ready to ask your interviewers about the role, team, and company.
- Review your past projects: Be prepared to discuss your previous work experiences and technical projects in detail.
- Practice system design: If applying for a senior role, dedicate time to practicing system design questions and studying distributed systems concepts.
- Behavioral preparation: Reflect on your past experiences and prepare stories that showcase your skills and problem-solving abilities.
8. Conclusion
Preparing for a Dropbox interview can be challenging, but with the right approach and dedication, you can significantly increase your chances of success. Focus on strengthening your coding skills, understanding system design principles, and developing your ability to communicate technical concepts clearly.
Remember that the interview process is not just about showcasing your technical skills but also demonstrating your problem-solving approach, ability to work in a team, and passion for technology. By thoroughly preparing and staying calm during the interview, you’ll be well-equipped to tackle Dropbox’s interview questions and impress your interviewers.
Good luck with your Dropbox interview! With proper preparation and the insights provided in this guide, you’re well on your way to joining one of the most innovative companies in the tech industry.