Top Uber Interview Questions: Ace Your Next Technical Interview
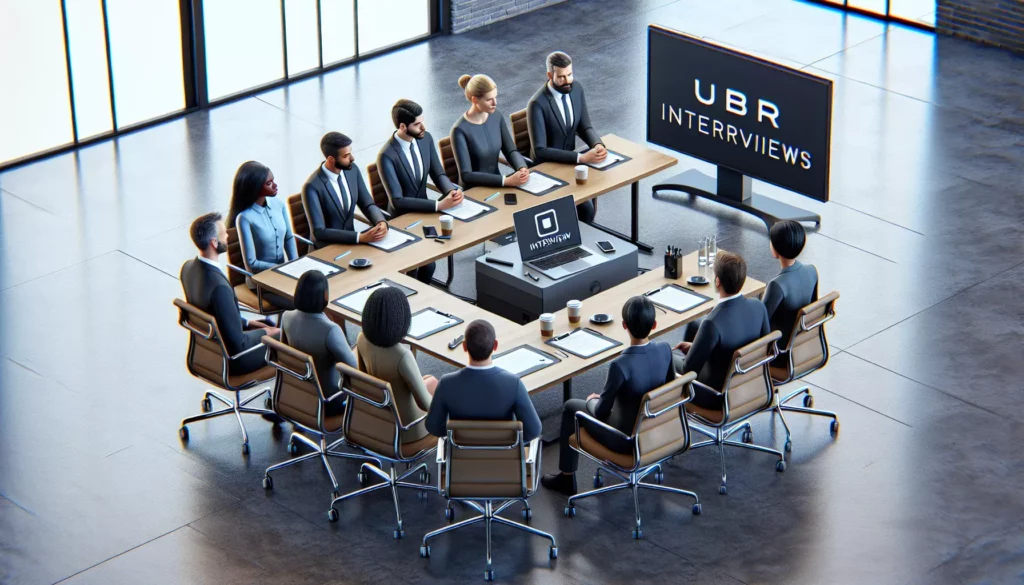
Are you gearing up for a technical interview at Uber? As one of the leading tech companies in the ride-sharing and food delivery industry, Uber is known for its rigorous interview process. To help you prepare, we’ve compiled a comprehensive list of common Uber interview questions, along with tips and strategies to tackle them effectively. Whether you’re a seasoned developer or a fresh graduate, this guide will give you the confidence to showcase your skills and land that dream job at Uber.
Table of Contents
- Introduction to Uber’s Interview Process
- Coding and Algorithm Questions
- System Design Questions
- Behavioral Questions
- Data Structures and Algorithms
- Problem-Solving Approach
- Uber-Specific Technical Questions
- Tips for Success
- How to Prepare
- Conclusion
1. Introduction to Uber’s Interview Process
Before diving into the specific questions, it’s essential to understand Uber’s interview process. Typically, it consists of several rounds:
- Initial Phone Screen: A brief conversation with a recruiter to assess your background and interest in the role.
- Technical Phone Interview: A coding interview conducted over the phone or video call, usually lasting 45-60 minutes.
- On-site Interviews: A series of face-to-face interviews (or virtual equivalents) that include coding, system design, and behavioral questions.
- Final Interview: Sometimes, there’s a final round with a senior engineer or hiring manager.
Throughout these stages, Uber evaluates candidates on their technical skills, problem-solving abilities, and cultural fit. Now, let’s explore the types of questions you might encounter in each category.
2. Coding and Algorithm Questions
Coding questions form the core of Uber’s technical interviews. These questions assess your ability to write clean, efficient code and solve algorithmic problems. Here are some examples of coding questions you might face:
2.1. Two Sum Problem
Question: Given an array of integers and a target sum, return indices of two numbers such that they add up to the target.
Example solution:
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
2.2. Reverse a Linked List
Question: Given the head of a singly linked list, reverse the list and return the new head.
Example solution:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverse_linked_list(head):
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
2.3. Implement a Queue using Stacks
Question: Implement a first-in-first-out (FIFO) queue using only two stacks.
Example solution:
class Queue:
def __init__(self):
self.stack1 = []
self.stack2 = []
def push(self, x):
self.stack1.append(x)
def pop(self):
if not self.stack2:
while self.stack1:
self.stack2.append(self.stack1.pop())
return self.stack2.pop() if self.stack2 else None
def peek(self):
if not self.stack2:
while self.stack1:
self.stack2.append(self.stack1.pop())
return self.stack2[-1] if self.stack2 else None
def empty(self):
return len(self.stack1) == 0 and len(self.stack2) == 0
These are just a few examples of the coding questions you might encounter. Uber’s interviewers often focus on data structures, algorithms, and problem-solving skills. Be prepared to explain your thought process and discuss the time and space complexity of your solutions.
3. System Design Questions
For more senior positions or backend roles, system design questions are crucial. These questions assess your ability to design scalable, reliable, and efficient systems. Here are some common system design questions you might face at Uber:
3.1. Design Uber’s Ride-Sharing System
Question: Design a simplified version of Uber’s ride-sharing system. Consider aspects like matching riders with drivers, handling real-time location updates, and managing surge pricing.
Key points to consider:
- Geolocation services for tracking drivers and riders
- Matching algorithm for pairing drivers with riders
- Real-time updates and notifications
- Database design for storing user information, ride history, and payments
- Scalability to handle millions of concurrent users
- Load balancing and caching strategies
3.2. Design a Distributed Rate Limiter
Question: Design a distributed rate limiter that can handle high-volume API requests across multiple servers.
Key points to consider:
- Choosing an appropriate rate-limiting algorithm (e.g., Token Bucket, Leaky Bucket)
- Distributed storage for maintaining rate limit data
- Consistency and synchronization across multiple servers
- Handling edge cases and failure scenarios
- Scalability and performance considerations
3.3. Design a Real-Time Analytics Dashboard
Question: Design a real-time analytics dashboard for Uber that displays metrics like active rides, revenue, and user growth.
Key points to consider:
- Data ingestion and processing pipeline
- Storage solutions for historical and real-time data
- Aggregation and computation of metrics
- Frontend architecture for real-time updates
- Scalability to handle large volumes of data
- Data visualization techniques
When tackling system design questions, focus on breaking down the problem, discussing trade-offs, and explaining your reasoning for architectural decisions. Be prepared to go into detail on specific components if the interviewer asks for more information.
4. Behavioral Questions
Behavioral questions are an essential part of Uber’s interview process. They help assess your soft skills, cultural fit, and past experiences. Here are some common behavioral questions you might encounter:
4.1. Teamwork and Collaboration
- Describe a time when you had to work closely with someone whose personality was very different from yours.
- Tell me about a successful project you worked on as part of a team. What was your role, and how did you contribute to the team’s success?
- Have you ever had a conflict with a team member? How did you resolve it?
4.2. Problem-Solving and Decision Making
- Describe a situation where you had to solve a difficult problem. What did you do, and what was the outcome?
- Tell me about a time when you had to make a decision without all the necessary information. How did you proceed?
- Have you ever made a mistake that impacted your team or project? How did you handle it?
4.3. Leadership and Initiative
- Describe a situation where you took the lead on a project or initiative.
- Tell me about a time when you had to motivate others to achieve a common goal.
- Have you ever implemented a process improvement or suggested an innovative solution? What was the result?
4.4. Adaptability and Learning
- Describe a time when you had to learn a new technology or skill quickly. How did you approach it?
- Tell me about a situation where you had to adapt to a significant change at work.
- How do you stay updated with the latest trends and technologies in your field?
When answering behavioral questions, use the STAR method (Situation, Task, Action, Result) to structure your responses. Provide specific examples from your past experiences, and focus on demonstrating your skills and problem-solving abilities.
5. Data Structures and Algorithms
A solid understanding of data structures and algorithms is crucial for succeeding in Uber’s technical interviews. Here are some key areas to focus on:
5.1. Arrays and Strings
- Two-pointer techniques
- Sliding window algorithms
- String manipulation and parsing
5.2. Linked Lists
- Traversal and reversal
- Detecting cycles
- Merging and sorting linked lists
5.3. Trees and Graphs
- Binary tree traversals (in-order, pre-order, post-order)
- Binary search trees
- Graph representations (adjacency list, adjacency matrix)
- Graph traversals (BFS, DFS)
- Shortest path algorithms (Dijkstra’s, Bellman-Ford)
5.4. Stacks and Queues
- Implementation and applications
- Monotonic stack problems
- Priority queues and heaps
5.5. Hash Tables
- Hash functions and collision resolution
- Applications in solving array and string problems
5.6. Dynamic Programming
- Memoization and tabulation techniques
- Common DP patterns (e.g., 0/1 Knapsack, Longest Common Subsequence)
5.7. Sorting and Searching
- Comparison-based sorting algorithms (Quicksort, Mergesort)
- Binary search and its variations
Make sure you can implement these data structures and algorithms from scratch and understand their time and space complexities. Practice solving problems that utilize these concepts, as they form the foundation of many interview questions.
6. Problem-Solving Approach
Uber’s interviewers are not just looking for correct solutions; they want to see your problem-solving process. Here’s a step-by-step approach to tackle coding problems effectively:
- Understand the problem: Carefully read the question and ask clarifying questions if needed. Make sure you understand the input, output, and any constraints.
- Analyze the problem: Consider edge cases and potential challenges. Think about similar problems you’ve encountered before.
- Brainstorm solutions: Come up with multiple approaches to solve the problem. Consider their pros and cons.
- Choose an approach: Select the most appropriate solution based on time and space complexity, as well as implementation simplicity.
- Plan your code: Outline the main steps of your algorithm before starting to code.
- Implement the solution: Write clean, readable code. Use meaningful variable names and add comments if necessary.
- Test your code: Go through your code with a simple example. Check for edge cases and potential bugs.
- Optimize: If time allows, think about ways to improve your solution’s efficiency or readability.
Remember to communicate your thoughts throughout the process. Uber’s interviewers want to understand your reasoning and problem-solving skills, not just see the final code.
7. Uber-Specific Technical Questions
While Uber’s interviews cover general programming concepts, you might also encounter questions specific to Uber’s technology stack and business domain. Here are some areas to be familiar with:
7.1. Geospatial Algorithms
- Calculating distances between coordinates
- Finding nearby drivers or restaurants
- Optimizing routes for multiple stops
7.2. Real-Time Systems
- Handling concurrent requests
- Implementing pub-sub systems
- Designing real-time notification systems
7.3. Distributed Systems
- Consistency models in distributed databases
- Handling network partitions
- Designing fault-tolerant systems
7.4. Machine Learning and Data Science
- Basics of recommendation systems
- Fraud detection algorithms
- Demand forecasting techniques
7.5. Mobile Development
- Android and iOS platform specifics
- Optimizing mobile app performance
- Handling offline scenarios in mobile apps
While you may not be asked directly about these topics, having a general understanding can help you provide more insightful answers to system design or problem-solving questions.
8. Tips for Success
To increase your chances of success in Uber’s interview process, keep these tips in mind:
- Practice, practice, practice: Solve coding problems regularly on platforms like LeetCode, HackerRank, or AlgoCademy.
- Mock interviews: Conduct mock interviews with friends or use online platforms to simulate the interview experience.
- Time management: During the interview, manage your time wisely. If you’re stuck, communicate your thoughts and ask for hints if needed.
- Communicate clearly: Explain your thought process clearly. Think out loud and discuss trade-offs in your solutions.
- Ask questions: Don’t hesitate to ask clarifying questions about the problem or requirements.
- Know your resume: Be prepared to discuss any project or technology mentioned in your resume in detail.
- Stay calm: If you make a mistake or get stuck, stay calm and composed. Show that you can handle pressure professionally.
- Follow-up: At the end of the interview, ask thoughtful questions about the role, team, or company to show your interest and engagement.
9. How to Prepare
To effectively prepare for your Uber interview, consider the following steps:
- Review fundamentals: Brush up on core computer science concepts, data structures, and algorithms.
- Practice coding: Solve problems on coding platforms regularly. Focus on medium to hard difficulty questions.
- Study system design: Read books or online resources on system design principles and practice designing large-scale systems.
- Mock interviews: Conduct mock interviews to simulate the real experience and get feedback on your performance.
- Learn about Uber: Research Uber’s products, technologies, and recent developments to show your interest in the company.
- Prepare your stories: Have specific examples ready for behavioral questions, following the STAR method.
- Review your projects: Be prepared to discuss your past projects, challenges faced, and lessons learned.
- Stay updated: Keep up with the latest trends in technology, especially in areas relevant to Uber’s business.
10. Conclusion
Preparing for an Uber interview can be challenging, but with the right approach and dedication, you can significantly increase your chances of success. Focus on strengthening your coding skills, understanding system design principles, and developing your problem-solving abilities. Remember that the interview process is not just about finding the correct answer, but also about demonstrating your thought process, communication skills, and ability to work in a team.
By thoroughly preparing for the types of questions discussed in this guide and following the tips provided, you’ll be well-equipped to tackle Uber’s interview process. Stay confident, communicate clearly, and showcase your passion for technology and innovation. Good luck with your Uber interview!