Top Airbnb Interview Questions: Ace Your Next Technical Interview
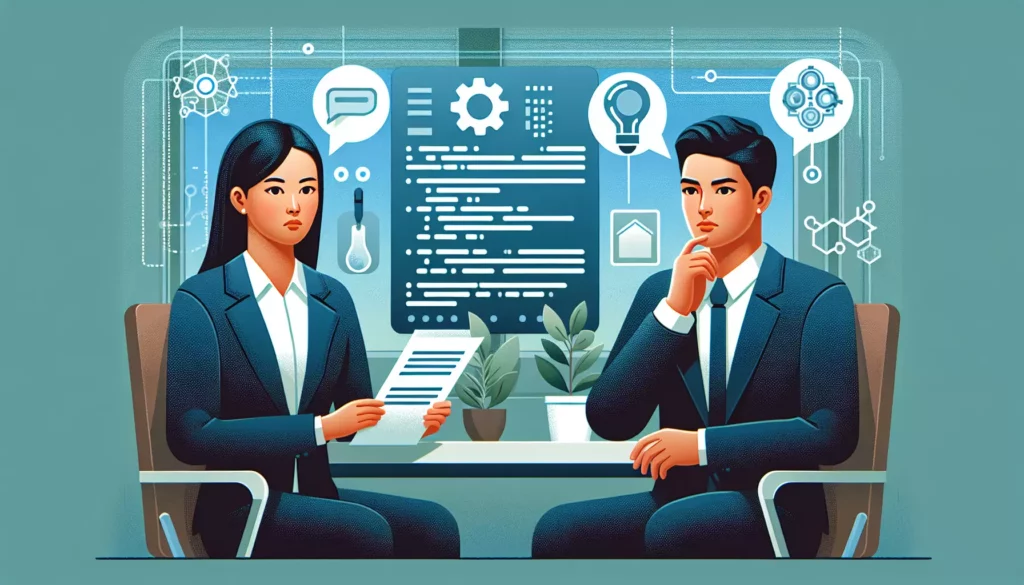
Are you preparing for a technical interview at Airbnb? You’re in the right place! In this comprehensive guide, we’ll explore some of the most common Airbnb interview questions, provide insights into the company’s interview process, and offer tips to help you succeed. Whether you’re a seasoned developer or just starting your journey in tech, this article will equip you with the knowledge and strategies needed to tackle Airbnb’s challenging interview questions.
Table of Contents
- Understanding Airbnb Interviews
- Coding Questions
- System Design Questions
- Behavioral Questions
- Data Science Questions
- Tips for Success
- Preparation Resources
- Conclusion
1. Understanding Airbnb Interviews
Before diving into specific questions, it’s essential to understand the structure of Airbnb’s interview process. Typically, candidates can expect the following stages:
- Phone Screen: An initial conversation with a recruiter to assess your background and interest in the role.
- Technical Phone Interview: A coding interview conducted over the phone or via video call, usually lasting 45-60 minutes.
- On-site Interviews: A series of 4-5 interviews, including coding, system design, and behavioral questions.
- Final Decision: The hiring committee reviews all feedback and makes a decision.
Airbnb places a strong emphasis on both technical skills and cultural fit. They look for candidates who demonstrate problem-solving abilities, creativity, and alignment with their core values.
2. Coding Questions
Coding questions form a significant part of Airbnb’s technical interviews. These questions typically focus on data structures, algorithms, and problem-solving skills. Here are some examples of coding questions you might encounter:
2.1. Implement an Autocomplete System
Design and implement an autocomplete system. Users enter a query string, and the system returns all matching strings from a predefined set of strings.
Example solution:
class TrieNode:
def __init__(self):
self.children = {}
self.is_word = False
class Autocomplete:
def __init__(self, words):
self.root = TrieNode()
for word in words:
self.insert(word)
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_word = True
def search(self, prefix):
node = self.root
for char in prefix:
if char not in node.children:
return []
node = node.children[char]
return self.dfs(node, prefix)
def dfs(self, node, prefix):
results = []
if node.is_word:
results.append(prefix)
for char, child in node.children.items():
results.extend(self.dfs(child, prefix + char))
return results
# Usage
words = ["air", "airbnb", "airplane", "airport", "apple", "apply"]
ac = Autocomplete(words)
print(ac.search("air")) # Output: ['air', 'airbnb', 'airplane', 'airport']
print(ac.search("app")) # Output: ['apple', 'apply']
2.2. Implement a Calendar Class
Design a calendar class that can schedule events, check for conflicts, and manage overlapping time slots.
Example solution:
class Event:
def __init__(self, start_time, end_time):
self.start_time = start_time
self.end_time = end_time
class Calendar:
def __init__(self):
self.events = []
def schedule_event(self, start_time, end_time):
new_event = Event(start_time, end_time)
for event in self.events:
if self.is_conflicting(event, new_event):
return False
self.events.append(new_event)
return True
def is_conflicting(self, event1, event2):
return (event1.start_time < event2.end_time and
event2.start_time < event1.end_time)
def get_available_slots(self, duration):
if not self.events:
return [(0, 24 * 60)] # Return full day if no events
self.events.sort(key=lambda x: x.start_time)
available_slots = []
prev_end = 0
for event in self.events:
if event.start_time - prev_end >= duration:
available_slots.append((prev_end, event.start_time))
prev_end = max(prev_end, event.end_time)
if 24 * 60 - prev_end >= duration:
available_slots.append((prev_end, 24 * 60))
return available_slots
# Usage
calendar = Calendar()
print(calendar.schedule_event(60, 120)) # True
print(calendar.schedule_event(90, 150)) # False (conflict)
print(calendar.schedule_event(150, 210)) # True
print(calendar.get_available_slots(60)) # [(0, 60), (120, 150), (210, 1440)]
2.3. Implement a Rate Limiter
Design a rate limiter that can be used to control the rate of requests a service receives.
Example solution using the token bucket algorithm:
import time
class RateLimiter:
def __init__(self, capacity, refill_rate):
self.capacity = capacity
self.refill_rate = refill_rate
self.tokens = capacity
self.last_refill_time = time.time()
def allow_request(self):
self.refill_tokens()
if self.tokens >= 1:
self.tokens -= 1
return True
return False
def refill_tokens(self):
now = time.time()
time_passed = now - self.last_refill_time
new_tokens = time_passed * self.refill_rate
self.tokens = min(self.capacity, self.tokens + new_tokens)
self.last_refill_time = now
# Usage
limiter = RateLimiter(capacity=10, refill_rate=1) # 10 requests per second
for _ in range(15):
print(limiter.allow_request())
time.sleep(5) # Wait for 5 seconds
for _ in range(15):
print(limiter.allow_request())
3. System Design Questions
System design questions are crucial for senior engineering roles at Airbnb. These questions assess your ability to design scalable, robust, and efficient systems. Here are some examples:
3.1. Design Airbnb’s Search Functionality
Design a system that allows users to search for accommodations based on various criteria such as location, dates, price range, and amenities.
Key considerations:
- Indexing and searching large datasets efficiently
- Handling geospatial queries
- Implementing filters and sorting
- Caching and performance optimization
- Handling concurrent requests and scaling
3.2. Design Airbnb’s Booking System
Create a system that manages the booking process, including availability checks, reservations, and payment processing.
Key considerations:
- Ensuring data consistency and avoiding double bookings
- Handling concurrent booking requests
- Implementing a reliable payment system
- Managing cancellations and refunds
- Scaling to handle peak booking periods
3.3. Design Airbnb’s Review and Rating System
Develop a system that allows users to leave reviews and ratings for their stays, and hosts to respond to these reviews.
Key considerations:
- Storing and retrieving reviews efficiently
- Implementing a fair rating system
- Handling spam and fake reviews
- Aggregating and displaying review data
- Allowing hosts to respond to reviews
4. Behavioral Questions
Airbnb places a strong emphasis on cultural fit and values alignment. Be prepared to answer behavioral questions that assess your problem-solving skills, teamwork, and ability to handle challenging situations. Some examples include:
- Tell me about a time when you had to work with a difficult team member. How did you handle it?
- Describe a situation where you had to make a decision with incomplete information. What was your approach?
- Can you share an example of when you took initiative on a project?
- How do you handle feedback, both positive and constructive?
- Tell me about a time when you had to adapt to a significant change at work.
When answering behavioral questions, use the STAR method (Situation, Task, Action, Result) to structure your responses and provide concrete examples from your past experiences.
5. Data Science Questions
For data science roles at Airbnb, you may encounter questions that test your statistical knowledge, machine learning skills, and ability to derive insights from data. Here are some examples:
5.1. Pricing Algorithm
Design an algorithm to suggest optimal pricing for Airbnb listings based on various factors such as location, seasonality, and demand.
5.2. User Segmentation
How would you segment Airbnb users to create personalized marketing campaigns? What features would you consider, and which clustering techniques might you use?
5.3. Anomaly Detection
Design a system to detect fraudulent activities on the Airbnb platform, such as fake listings or suspicious booking patterns.
5.4. A/B Testing
How would you design and analyze an A/B test to measure the impact of a new feature on the Airbnb platform?
6. Tips for Success
To increase your chances of success in Airbnb interviews, consider the following tips:
- Practice coding problems: Regularly solve coding challenges on platforms like LeetCode, HackerRank, or AlgoCademy to sharpen your problem-solving skills.
- Study system design: Familiarize yourself with common system design patterns and best practices. Read books like “Designing Data-Intensive Applications” by Martin Kleppmann.
- Understand Airbnb’s business: Research Airbnb’s products, features, and challenges to demonstrate your interest in the company.
- Prepare for behavioral questions: Reflect on your past experiences and prepare stories that highlight your skills and alignment with Airbnb’s values.
- Practice explaining your thought process: During interviews, clearly communicate your approach to solving problems and making decisions.
- Ask thoughtful questions: Prepare questions about Airbnb’s technology, culture, and future plans to show your engagement and curiosity.
- Stay up-to-date with industry trends: Keep informed about the latest developments in technology, especially those relevant to Airbnb’s business.
7. Preparation Resources
To help you prepare for your Airbnb interview, consider using the following resources:
- AlgoCademy: An interactive platform that offers coding tutorials, practice problems, and AI-powered assistance to help you prepare for technical interviews.
- LeetCode: A popular platform for practicing coding problems, including a section dedicated to company-specific questions.
- System Design Primer: A GitHub repository that provides a comprehensive overview of system design concepts and examples.
- Glassdoor: Read interview experiences shared by other candidates who have interviewed at Airbnb.
- Airbnb Engineering Blog: Stay updated on Airbnb’s technical challenges and solutions by reading their engineering blog.
- Cracking the Coding Interview: A book by Gayle Laakmann McDowell that covers essential coding interview topics and strategies.
- Designing Data-Intensive Applications: A book by Martin Kleppmann that delves into the principles of designing large-scale distributed systems.
8. Conclusion
Preparing for an Airbnb interview can be challenging, but with the right approach and resources, you can significantly increase your chances of success. Focus on honing your coding skills, understanding system design principles, and aligning your experiences with Airbnb’s values and culture. Remember to practice explaining your thought process clearly and concisely during interviews.
By familiarizing yourself with the types of questions asked in Airbnb interviews and following the tips provided in this guide, you’ll be well-equipped to tackle the challenges ahead. Good luck with your interview preparation, and don’t forget to leverage platforms like AlgoCademy to enhance your coding and problem-solving skills!
Remember, the key to success in any technical interview is not just about knowing the right answers, but also about demonstrating your ability to approach problems methodically, communicate effectively, and showcase your passion for technology and innovation. With thorough preparation and the right mindset, you’ll be well on your way to joining the talented team at Airbnb.