Essential Tips for Successful Algorithm Practice
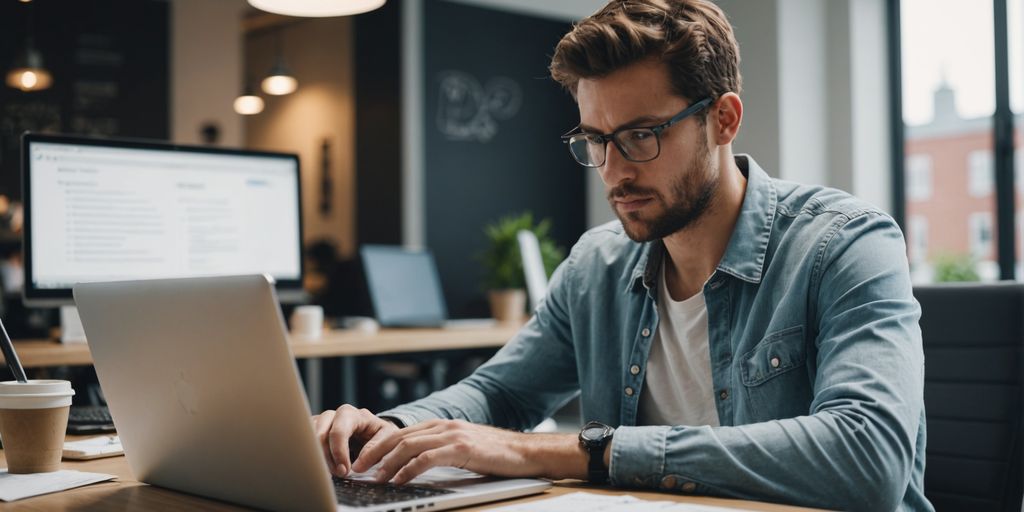
Practicing algorithms is key to becoming a better problem solver in programming. By learning and applying different strategies, you can improve your skills and tackle complex problems more effectively. This article provides essential tips to help you practice algorithms successfully.
Key Takeaways
- Build a strong foundation by understanding basic concepts and common algorithms.
- Regular practice on online coding platforms and participation in competitions can enhance your skills.
- Break down complex problems and learn from your mistakes to improve problem-solving abilities.
- Optimize your algorithms by analyzing their efficiency and choosing the right data structures.
- Stay updated with the latest trends and continuously learn to keep your skills sharp.
Building a Strong Foundation in Algorithm Practice
Understanding Basic Concepts
To start with algorithms, you need to grasp the basic concepts. This includes understanding what an algorithm is, how it works, and why it’s important. Algorithms are step-by-step instructions to solve problems or perform tasks. Knowing the basics helps you tackle more complex problems later.
Importance of Pseudocode
Writing pseudocode is a crucial step in algorithm practice. Pseudocode is a way to plan your algorithm using plain language before coding. It helps you organize your thoughts and ensures you understand the problem. Think of it as a blueprint for your code.
Familiarizing with Common Algorithms
Get to know common algorithms like sorting and searching. These are the building blocks of more complex algorithms. By studying these, you learn how to approach different types of problems. For example, understanding the knapsack problem can help you analyze algorithm efficiency.
Building a strong foundation in algorithms is like laying the groundwork for a house. Without a solid base, everything else will be shaky.
Here’s a quick list to get you started:
- Sorting algorithms (e.g., bubble sort, quicksort)
- Searching algorithms (e.g., binary search)
- Basic data structures (e.g., arrays, linked lists)
Effective Strategies for Regular Practice
Utilizing Online Coding Platforms
Online coding platforms are a great way to practice algorithms. Websites like LeetCode, HackerRank, and CodeSignal offer numerous problems to solve. These platforms provide detailed explanations and user-submitted solutions, which can help you understand different approaches to the same problem. Consistent practice on these platforms can significantly improve your skills.
Participating in Coding Competitions
Coding competitions such as Google Code Jam, Topcoder, and ACM-ICPC are excellent opportunities to test your skills. These contests expose you to a variety of algorithmic challenges and allow you to compete with others. Participating in these competitions regularly can help you stay sharp and learn new techniques.
Implementing Algorithms from Scratch
One of the most effective ways to understand an algorithm is to implement it from scratch. Choose an algorithm that interests you, study it, and then try to code it without looking up any existing solutions. This practice helps solidify your understanding and improves your problem-solving skills.
Regular practice is key to mastering algorithms. Set a regular practice schedule and stick to it. Participate in weekly or bi-weekly coding challenges to keep yourself engaged and motivated.
Enhancing Problem-Solving Skills
Breaking Down Complex Problems
When faced with a tough problem, start by breaking it down into smaller, more manageable parts. This approach makes it easier to understand and solve each part step by step. Identifying the core components of a problem can simplify the process and lead to a more effective solution.
Learning from Mistakes
Mistakes are a natural part of learning. When you make an error, take the time to understand what went wrong and how you can fix it. This reflection helps you avoid similar mistakes in the future and improves your overall problem-solving skills. Remember, every mistake is an opportunity to learn and grow.
Collaborating with Peers
Working with others can provide new perspectives and ideas. Collaborate with your peers to solve problems together. This not only helps you learn from their approaches but also allows you to explain your own thinking, which can reinforce your understanding. Sharing ideas and feedback can lead to better solutions and a deeper understanding of the material.
Optimizing Algorithm Performance
Analyzing Algorithm Efficiency
Understanding how efficient your algorithm is can make a big difference. Time complexity analysis helps you see how your algorithm performs in the worst, average, and best cases. Use Big O notation to estimate this. By knowing the time complexity, you can find ways to make your algorithm faster.
Choosing the Right Data Structures
Picking the right data structure is key. The right choice can make your algorithm run much faster. For example, using a hash table instead of a list can save a lot of time when searching for items. Always think about which data structure fits your problem best.
Iterating and Refining Solutions
Improving your algorithm is an ongoing process. Start by writing a simple version and then make it better. Look for parts of your code that can be optimized. Remove unnecessary steps and use efficient methods. Keep testing and refining until your algorithm is as fast as it can be.
By applying these techniques, you can significantly improve the performance of your algorithms and make them more efficient in solving problems.
Applying Algorithms to Real-World Scenarios
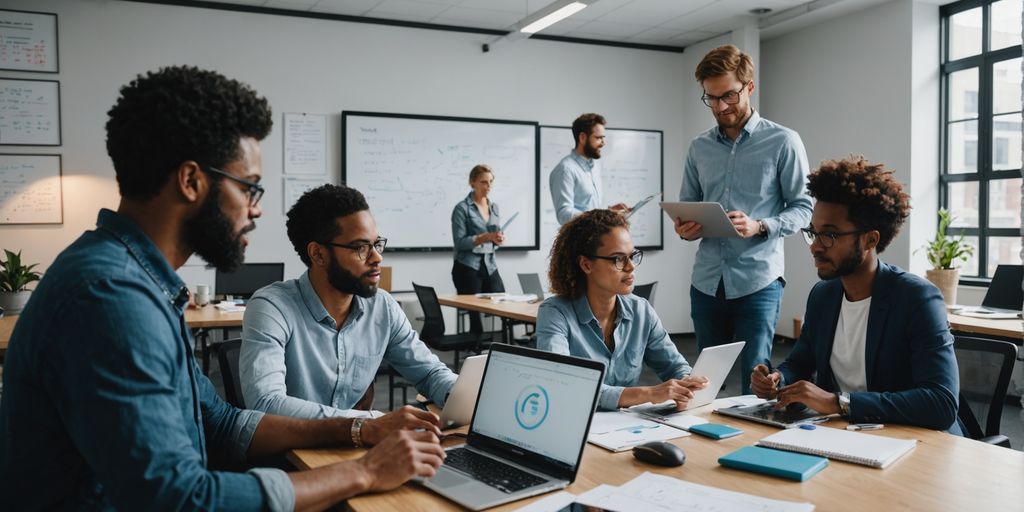
Software Development Applications
In software development, algorithms are crucial for solving problems like sorting and searching. They help make software run faster and use fewer resources, which makes users happier. Efficient algorithms can significantly improve the performance of applications.
Data Analysis and Machine Learning
Algorithm design is super important in data analysis and machine learning. It helps process big sets of data and find useful info. When we make good algorithms for things like grouping data, sorting it, or making predictions, we can find patterns and make smart choices based on them.
Networking and Cryptography
In networking, algorithms optimize data transmission and routing paths. Efficient routing algorithms ensure that data packets are delivered quickly and reliably, minimizing network congestion and latency. Cryptography relies on sophisticated algorithms to secure sensitive information and protect communication channels from unauthorized access. By designing robust encryption and decryption algorithms, cryptographers can safeguard data against cyber threats and ensure confidentiality and integrity.
Understanding the real-life applications of data structures and algorithms can inspire you to learn and apply these concepts in your projects.
Game Development
In video game making, algorithm design helps create lifelike actions, smart enemies, and fun gaming adventures. With algorithms for finding paths, spotting crashes, and deciding how computer characters act, game makers can make exciting games that grab players’ attention.
Staying Updated and Continuously Learning
Following Industry Trends
To stay ahead in the field of algorithms, it’s crucial to keep up with the latest trends. Following industry trends helps you understand the current demands and future directions. Subscribe to newsletters, follow influential tech blogs, and join relevant forums to stay informed.
Reading Research Papers
Reading research papers is an excellent way to dive deep into new algorithmic techniques and theories. Websites like arXiv and Google Scholar offer a plethora of papers on various topics. Make it a habit to read at least one paper a week to stay updated.
Engaging in Continuous Education
Continuous education is key to mastering algorithms. Enroll in online courses, attend workshops, and participate in webinars. Platforms like Coursera, Udemy, and edX offer courses that can help you learn new skills and stay updated with the latest advancements.
Continual learning is a set of approaches to train machine learning models incrementally, using data samples only once as they arrive.
By following these steps, you can ensure that you are always learning and growing in your algorithmic knowledge.
Utilizing Tools and Resources for Practice
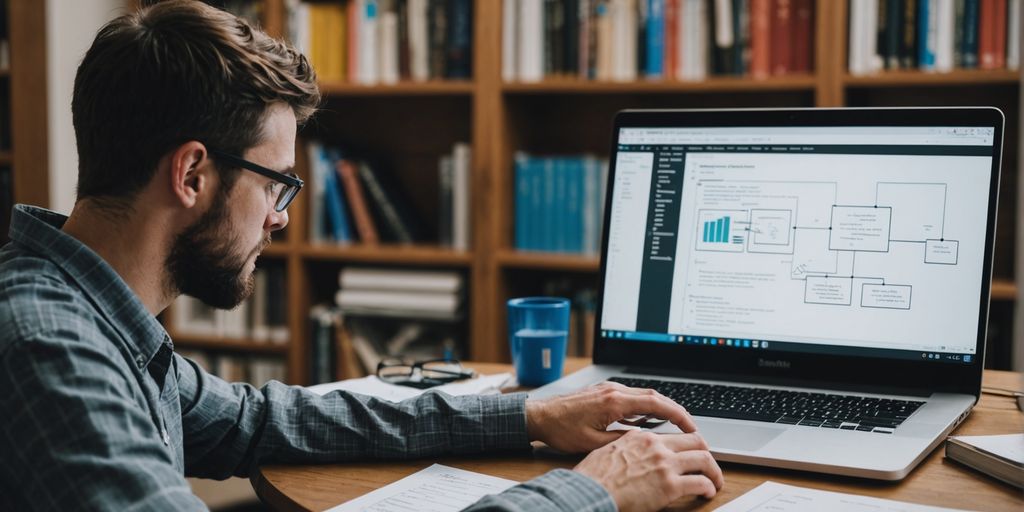
Interactive Learning Platforms
Interactive learning platforms are a great way to practice algorithms. Websites like LeetCode, Codechef, and Codeforces offer a wide range of problems to solve. These platforms provide instant feedback, which helps you learn and improve quickly. They also support many programming languages, so you can practice in the language you are most comfortable with.
Books and Online Resources
Books and online resources are essential for deepening your understanding of algorithms. Websites like GeeksforGeeks and Wikipedia are excellent starting points. For those looking for free resources, I recommend searching for "takeuforward" on YouTube and Google. These resources cover everything from basic to advanced topics, making them invaluable for both beginners and experienced coders.
Visual and Interactive Tools
Visual and interactive tools can make learning algorithms more engaging. Tools like VisuAlgo and Algorithm Visualizer allow you to see how algorithms work in real-time. This can be especially helpful for visual learners who benefit from seeing concepts in action. By using these tools, you can gain a better understanding of complex algorithms and data structures.
Conclusion
In conclusion, mastering algorithms is a journey that requires patience, practice, and a willingness to learn. By regularly solving problems, analyzing your solutions, and staying updated with the latest trends, you can significantly improve your algorithmic skills. Remember, the key is to start with simple problems and gradually tackle more complex ones. Use online platforms, collaborate with peers, and apply what you learn to real-world projects. With dedication and consistent effort, you’ll find yourself becoming more proficient and confident in algorithm design. Keep pushing your limits, and happy coding!
Frequently Asked Questions
Why is learning algorithms important?
Learning algorithms is key because they help you solve problems efficiently in coding. They make your programs run faster and use fewer resources.
How often should I practice algorithms?
You should practice algorithms regularly, ideally every day. Start with simple problems and gradually move to more complex ones to improve your skills.
What are some good online platforms for practicing algorithms?
Websites like LeetCode, HackerRank, and CodeSignal are great for practicing algorithms. They offer a wide range of problems and solutions to help you learn.
How can I improve my problem-solving skills?
You can improve by breaking down complex problems into smaller parts, learning from your mistakes, and working with peers to get different perspectives.
What should I do if I get stuck on a problem?
If you get stuck, take a break and come back to it later with a fresh mind. You can also seek help from online communities or study similar problems to find a solution.
How can algorithms be applied in real life?
Algorithms are used in many areas like software development, data analysis, machine learning, networking, and even game development to solve real-world problems efficiently.