Top NVIDIA Interview Questions: Mastering the Technical Challenge
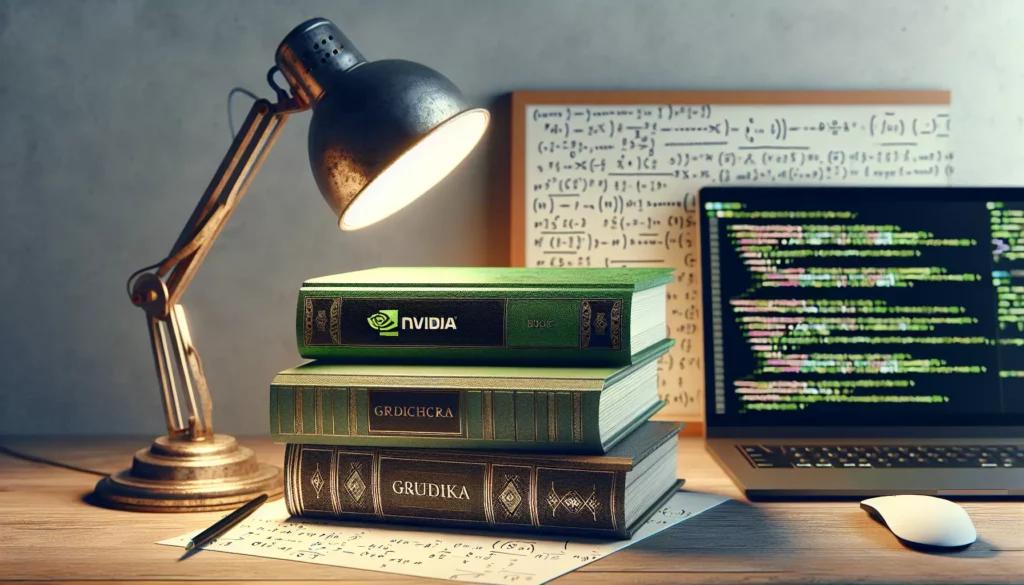
Are you gearing up for an interview with NVIDIA, one of the world’s leading technology companies? Known for their groundbreaking work in graphics processing units (GPUs) and artificial intelligence, NVIDIA is always on the lookout for top-tier talent. To help you prepare, we’ve compiled a comprehensive list of NVIDIA interview questions that will give you a solid foundation for your upcoming technical interview.
Table of Contents
- Introduction to NVIDIA Interviews
- Coding and Algorithm Questions
- System Design Questions
- GPU-Specific Questions
- Machine Learning and AI Questions
- Behavioral Questions
- Tips for Success
- Conclusion
1. Introduction to NVIDIA Interviews
NVIDIA’s interview process is known for its rigor and depth, reflecting the company’s position at the forefront of GPU technology and AI research. Candidates can expect a multi-stage process that typically includes:
- Initial phone screen
- Technical phone interview
- On-site interviews (or virtual equivalent)
- Coding challenges
- System design discussions
- Behavioral interviews
The questions you’ll face will vary depending on the specific role you’re applying for, but they generally fall into several categories. Let’s dive into each of these categories and explore some common questions you might encounter.
2. Coding and Algorithm Questions
NVIDIA places a strong emphasis on coding skills and algorithmic thinking. Here are some examples of coding questions you might face:
2.1. Reverse a Linked List
This is a classic problem that tests your understanding of data structures and pointers.
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverseList(head):
prev = None
current = head
while current:
next_temp = current.next
current.next = prev
prev = current
current = next_temp
return prev
2.2. Implement a Queue using Two Stacks
This question tests your understanding of basic data structures and your ability to think creatively about their implementation.
class Queue:
def __init__(self):
self.stack1 = []
self.stack2 = []
def enqueue(self, x):
self.stack1.append(x)
def dequeue(self):
if not self.stack2:
while self.stack1:
self.stack2.append(self.stack1.pop())
if not self.stack2:
return None
return self.stack2.pop()
def peek(self):
if not self.stack2:
while self.stack1:
self.stack2.append(self.stack1.pop())
if not self.stack2:
return None
return self.stack2[-1]
2.3. Find the Kth Largest Element in an Array
This problem tests your knowledge of sorting algorithms and your ability to optimize for time and space complexity.
import heapq
def findKthLargest(nums, k):
return heapq.nlargest(k, nums)[-1]
2.4. Implement a Trie (Prefix Tree)
This advanced data structure question tests your ability to work with tree-like structures and optimize for prefix searches.
class TrieNode:
def __init__(self):
self.children = {}
self.is_end = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end = True
def search(self, word):
node = self.root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_end
def startsWith(self, prefix):
node = self.root
for char in prefix:
if char not in node.children:
return False
node = node.children[char]
return True
3. System Design Questions
System design questions are crucial for senior roles at NVIDIA. They test your ability to architect large-scale systems and make important design decisions. Here are some examples:
3.1. Design a Distributed Cache
This question tests your understanding of distributed systems and caching mechanisms. Key points to discuss include:
- Cache eviction policies (LRU, LFU, etc.)
- Consistency models (strong, eventual)
- Partitioning and replication strategies
- Handling cache misses and write-through vs write-back policies
3.2. Design a Real-time Analytics Dashboard
This question assesses your ability to handle large-scale data processing and visualization. Consider discussing:
- Data ingestion and processing pipelines
- Storage solutions for different types of data
- Aggregation and summarization techniques
- Real-time update mechanisms
- Scalability and performance optimizations
3.3. Design a Distributed File System
This complex system design question tests your knowledge of storage systems and distributed computing. Key areas to cover include:
- File system structure and metadata management
- Data replication and consistency
- Chunking and distribution strategies
- Fault tolerance and recovery mechanisms
- Access control and security
4. GPU-Specific Questions
Given NVIDIA’s focus on GPU technology, you can expect questions that delve into GPU architecture and parallel computing concepts.
4.1. Explain the difference between CUDA cores and Tensor cores.
CUDA (Compute Unified Device Architecture) cores are designed for general-purpose parallel computing tasks. They can handle a wide range of computations and are versatile in their application. Tensor cores, on the other hand, are specialized cores designed specifically for matrix multiplication and convolution operations, which are crucial for deep learning and AI workloads. Tensor cores can perform these operations much faster than CUDA cores, but they are less flexible in terms of the types of computations they can handle.
4.2. How does memory coalescing work in CUDA, and why is it important?
Memory coalescing in CUDA refers to the process of combining multiple memory accesses into a single transaction. This is important because it significantly improves memory bandwidth utilization and overall performance. When threads in a warp (a group of 32 threads that execute together) access contiguous memory locations, these accesses can be coalesced into a single memory transaction, reducing the number of memory requests and improving efficiency.
4.3. Describe the concept of warp divergence and its impact on GPU performance.
Warp divergence occurs when threads within the same warp take different execution paths due to conditional statements. This can significantly impact performance because GPUs are designed to execute instructions in a SIMT (Single Instruction, Multiple Thread) fashion. When divergence occurs, the different execution paths are serialized, effectively reducing parallelism and overall performance. To optimize GPU code, it’s important to minimize warp divergence by structuring code to avoid complex branching within warps when possible.
4.4. Explain the purpose and functioning of shared memory in CUDA programming.
Shared memory in CUDA is a fast, on-chip memory that can be accessed by all threads within a thread block. Its primary purpose is to reduce global memory accesses, which are much slower. Shared memory can be used as a software-managed cache, allowing threads to collaborate and share data efficiently. This is particularly useful for algorithms that require frequent data reuse or inter-thread communication within a block. Proper use of shared memory can significantly improve the performance of CUDA kernels.
5. Machine Learning and AI Questions
With NVIDIA’s strong presence in the AI and machine learning space, you may encounter questions related to these topics, especially if you’re interviewing for an AI-focused role.
5.1. Explain the concept of backpropagation in neural networks.
Backpropagation is a fundamental algorithm used in training neural networks. It works by calculating the gradient of the loss function with respect to each weight in the network, moving backwards from the output layer to the input layer. This gradient is then used to update the weights in a direction that minimizes the loss function. The process involves two main steps:
- Forward pass: Input data is passed through the network to generate predictions.
- Backward pass: The error is calculated and propagated backwards through the network, updating weights along the way.
5.2. What is the difference between CNN and RNN architectures?
Convolutional Neural Networks (CNNs) and Recurrent Neural Networks (RNNs) are two different types of neural network architectures designed for different types of data and tasks:
- CNNs are primarily used for processing grid-like data, such as images. They use convolutional layers to detect spatial hierarchies in the input data.
- RNNs are designed for sequential data, such as time series or natural language. They have a ‘memory’ component that allows them to process sequences of inputs.
5.3. Describe the concept of transfer learning and its applications.
Transfer learning is a machine learning technique where a model developed for one task is reused as the starting point for a model on a second task. This approach is particularly useful when you have limited labeled data for the task you’re interested in, but there’s a related task with abundant data. The process typically involves:
- Training a base network on a base dataset and task
- Reusing the learned features, or transfer them, to a second target network to be trained on a target dataset and task
Transfer learning is widely used in computer vision and natural language processing tasks, where pre-trained models on large datasets (like ImageNet for vision tasks or BERT for NLP tasks) are fine-tuned for specific applications.
5.4. Explain the concept of batch normalization and its benefits.
Batch normalization is a technique used to improve the stability and performance of neural networks. It normalizes the inputs to each layer for each mini-batch, which helps to address the internal covariate shift problem. The benefits of batch normalization include:
- Faster training: It allows higher learning rates and reduces the dependence on careful initialization.
- Regularization: It has a slight regularization effect, potentially reducing the need for dropout.
- Reduces sensitivity to network initialization
- Simplifies the creation of deeper networks
6. Behavioral Questions
While technical skills are crucial, NVIDIA also values soft skills and cultural fit. Here are some behavioral questions you might encounter:
6.1. Describe a time when you had to work on a challenging project. How did you approach it?
This question assesses your problem-solving skills and ability to handle complex tasks. Focus on describing the challenge, your approach, the actions you took, and the results you achieved.
6.2. How do you stay updated with the latest developments in technology?
NVIDIA values continuous learning. Discuss your methods for staying informed, such as following tech blogs, attending conferences, or participating in online courses.
6.3. Describe a situation where you had to work with a difficult team member. How did you handle it?
This question evaluates your interpersonal skills and ability to work in a team. Emphasize your communication skills, empathy, and problem-solving approach in difficult situations.
6.4. Tell me about a time when you had to learn a new technology quickly. How did you approach it?
This assesses your ability to adapt and learn quickly, which is crucial in the fast-paced tech industry. Describe your learning strategy, the resources you used, and how you applied your new knowledge.
7. Tips for Success
To increase your chances of success in an NVIDIA interview, consider the following tips:
- Practice coding regularly: Use platforms like LeetCode or HackerRank to sharpen your coding skills.
- Study GPU architecture: Familiarize yourself with NVIDIA’s GPU architecture and CUDA programming concepts.
- Brush up on system design: For senior roles, make sure you can discuss large-scale system design concepts.
- Stay updated on AI and ML: Keep abreast of the latest developments in artificial intelligence and machine learning.
- Prepare your “stories”: Have concrete examples ready for behavioral questions.
- Ask thoughtful questions: Prepare questions about NVIDIA’s technology, culture, and future plans to show your genuine interest.
- Be ready to explain your thought process: NVIDIA interviewers are often as interested in how you approach problems as they are in your final solution.
8. Conclusion
Preparing for an NVIDIA interview can be challenging, but with the right approach and preparation, you can significantly increase your chances of success. Remember that the interview process is not just about testing your technical skills, but also about assessing your problem-solving approach, your ability to learn and adapt, and your fit with NVIDIA’s culture.
Focus on strengthening your understanding of algorithms, data structures, and system design. If you’re interviewing for a GPU-specific role, make sure you have a solid grasp of parallel computing concepts and CUDA programming. For AI-focused positions, brush up on your machine learning and deep learning knowledge.
Don’t forget to prepare for behavioral questions as well. NVIDIA values teamwork, innovation, and continuous learning, so be ready to demonstrate these qualities through your experiences and responses.
Lastly, stay calm and confident during the interview. Remember that the interview is also an opportunity for you to learn more about NVIDIA and determine if it’s the right fit for your career goals. Good luck with your preparation, and may you ace your NVIDIA interview!