Top Meta Interview Questions: Ace Your Next Technical Interview
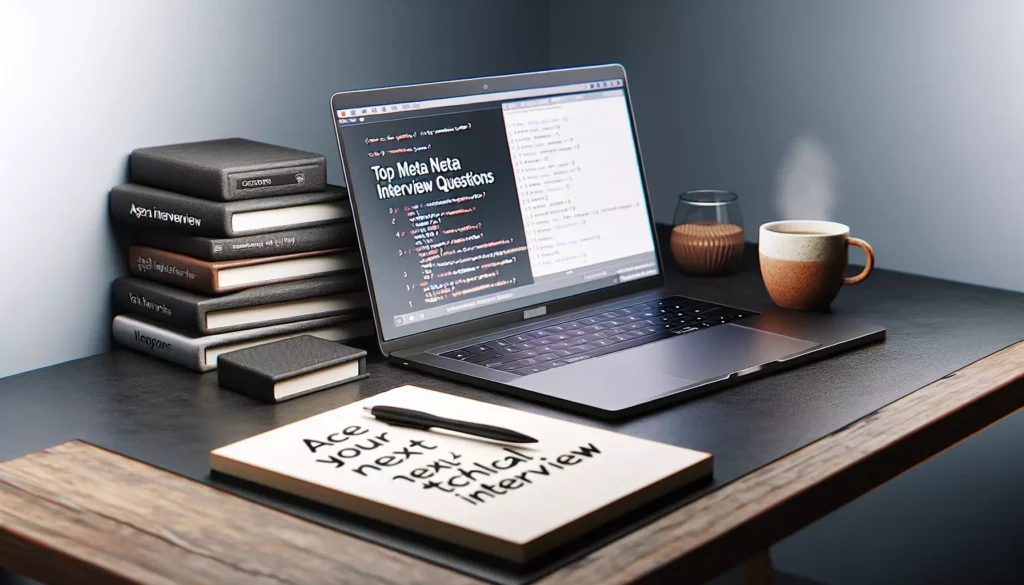
Are you preparing for a technical interview at Meta (formerly Facebook)? You’re in the right place! In this comprehensive guide, we’ll explore some of the most common and challenging Meta interview questions, along with strategies to tackle them effectively. Whether you’re a seasoned developer or just starting your journey in the tech industry, this article will help you sharpen your skills and boost your confidence for that crucial interview.
Table of Contents
- Introduction to Meta’s Interview Process
- Coding Questions
- System Design Questions
- Behavioral Questions
- General Tips for Success
- Additional Resources
- Conclusion
1. Introduction to Meta’s Interview Process
Meta’s interview process is known for its rigor and complexity. It typically consists of several rounds, including:
- Initial phone screen
- Coding interviews (1-2 rounds)
- System design interview (for more experienced candidates)
- Behavioral interview
- On-site interviews (which may include additional coding and system design questions)
The company looks for candidates who not only have strong technical skills but also align with their values and can work well in a collaborative environment. Let’s dive into the types of questions you might encounter in each stage of the interview process.
2. Coding Questions
Coding questions form the core of Meta’s technical interviews. These questions are designed to assess your problem-solving skills, coding ability, and understanding of data structures and algorithms. Here are some common types of coding questions you might encounter:
2.1. Two Pointer Technique
The two-pointer technique is a common approach used in many coding problems, especially those involving arrays or linked lists. Here’s an example question:
Question: Given a sorted array of integers, find two numbers such that they add up to a specific target number.
Solution:
def twoSum(nums, target):
left = 0
right = len(nums) - 1
while left < right:
current_sum = nums[left] + nums[right]
if current_sum == target:
return [nums[left], nums[right]]
elif current_sum < target:
left += 1
else:
right -= 1
return [] # No solution found
This solution uses two pointers, one starting from the beginning of the array and one from the end, moving them inwards based on the sum of the elements they point to.
2.2. Dynamic Programming
Dynamic programming is a powerful technique for solving optimization problems. Here’s a classic example:
Question: Given a list of integers representing the cost of climbing each step, find the minimum cost to reach the top of the staircase. You can either climb 1 or 2 steps at a time.
Solution:
def minCostClimbingStairs(cost):
n = len(cost)
dp = [0] * (n + 1)
for i in range(2, n + 1):
dp[i] = min(dp[i-1] + cost[i-1], dp[i-2] + cost[i-2])
return dp[n]
This solution uses a bottom-up dynamic programming approach to calculate the minimum cost at each step.
2.3. Graph Traversal
Graph problems are common in Meta interviews. Here’s an example of a depth-first search (DFS) problem:
Question: Given a 2D grid map of ‘1’s (land) and ‘0’s (water), count the number of islands.
Solution:
def numIslands(grid):
if not grid:
return 0
count = 0
for i in range(len(grid)):
for j in range(len(grid[0])):
if grid[i][j] == '1':
dfs(grid, i, j)
count += 1
return count
def dfs(grid, i, j):
if i < 0 or j < 0 or i >= len(grid) or j >= len(grid[0]) or grid[i][j] != '1':
return
grid[i][j] = '0'
dfs(grid, i+1, j)
dfs(grid, i-1, j)
dfs(grid, i, j+1)
dfs(grid, i, j-1)
This solution uses DFS to explore each island and mark it as visited by changing ‘1’s to ‘0’s.
2.4. Tree Traversal
Tree problems are also frequently asked in Meta interviews. Here’s an example of a binary tree traversal question:
Question: Given a binary tree, return the level order traversal of its nodes’ values. (i.e., from left to right, level by level)
Solution:
from collections import deque
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def levelOrder(root):
if not root:
return []
result = []
queue = deque([root])
while queue:
level_size = len(queue)
current_level = []
for _ in range(level_size):
node = queue.popleft()
current_level.append(node.val)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
result.append(current_level)
return result
This solution uses a breadth-first search (BFS) approach with a queue to traverse the tree level by level.
3. System Design Questions
For more experienced candidates, Meta often includes system design questions in their interviews. These questions assess your ability to design large-scale distributed systems. Here are some common system design questions you might encounter:
3.1. Design a Social Media Feed
Question: Design a news feed system similar to Facebook’s News Feed.
Key considerations:
- How to efficiently store and retrieve posts
- How to handle the ranking of posts
- How to manage real-time updates
- How to scale the system to millions of users
Possible high-level approach:
- Use a distributed database (e.g., Cassandra) to store user posts
- Implement a publish-subscribe system for real-time updates
- Use a caching layer (e.g., Redis) to store recent feeds
- Implement a ranking algorithm based on factors like recency, user engagement, and relevance
- Use a CDN for serving static content
3.2. Design a Chat Application
Question: Design a real-time chat application like Facebook Messenger.
Key considerations:
- How to handle real-time message delivery
- How to store chat history
- How to ensure message ordering
- How to handle offline users
Possible high-level approach:
- Use WebSockets for real-time communication
- Implement a message queue (e.g., RabbitMQ) for handling offline messages
- Use a distributed database (e.g., MongoDB) for storing chat history
- Implement a notification service for push notifications
- Use a load balancer to distribute traffic across multiple servers
4. Behavioral Questions
Behavioral questions are an essential part of Meta’s interview process. They help assess your cultural fit and how well you align with the company’s values. Here are some common behavioral questions you might encounter:
- Tell me about a time when you had to work on a challenging project.
- How do you handle conflicts with team members?
- Describe a situation where you had to learn a new technology quickly.
- How do you prioritize tasks when working on multiple projects?
- Tell me about a time when you received constructive feedback and how you handled it.
When answering behavioral questions, use the STAR method:
- Situation: Describe the context of the situation.
- Task: Explain what you were responsible for in that situation.
- Action: Describe the specific actions you took to address the situation.
- Result: Share the outcomes of your actions and what you learned from the experience.
5. General Tips for Success
To increase your chances of success in a Meta interview, consider the following tips:
- Practice, practice, practice: Solve coding problems regularly on platforms like LeetCode, HackerRank, or AlgoCademy.
- Study data structures and algorithms: Ensure you have a solid understanding of fundamental data structures (arrays, linked lists, trees, graphs) and algorithms (sorting, searching, dynamic programming).
- Learn system design principles: Familiarize yourself with distributed systems concepts, scalability, and common design patterns.
- Improve your communication skills: Practice explaining your thought process clearly while solving problems.
- Know Meta’s products: Familiarize yourself with Meta’s various products and services.
- Understand Meta’s values: Research Meta’s company culture and core values.
- Prepare questions for your interviewer: This shows your genuine interest in the role and the company.
- Stay calm and composed: Remember to breathe and take your time during the interview.
6. Additional Resources
To further prepare for your Meta interview, consider using the following resources:
- AlgoExpert: A platform with curated coding interview questions and video explanations.
- Grokking the System Design Interview: An online course focused on system design concepts and questions.
- Cracking the Coding Interview by Gayle Laakmann McDowell: A comprehensive book covering various aspects of technical interviews.
- Tech Lead YouTube Channel: Offers insights into tech interviews and software engineering careers.
- Meta Engineering Blog: Provides insights into Meta’s engineering practices and technologies.
7. Conclusion
Preparing for a Meta interview can be challenging, but with the right approach and consistent practice, you can significantly improve your chances of success. Remember to focus on both technical skills and soft skills, as Meta values well-rounded candidates who can contribute to their collaborative and innovative culture.
As you prepare, keep in mind that the interview process is not just about getting the right answers, but also about demonstrating your problem-solving approach, communication skills, and ability to work in a team. Stay confident, be yourself, and showcase your passion for technology and innovation.
Good luck with your Meta interview! With thorough preparation and the right mindset, you’ll be well-equipped to tackle any question that comes your way. Remember, every interview is also a learning experience, so regardless of the outcome, you’ll come out of it with valuable insights and areas for further growth in your career.