What Is Apache Spark? A Comprehensive Guide to Big Data Processing
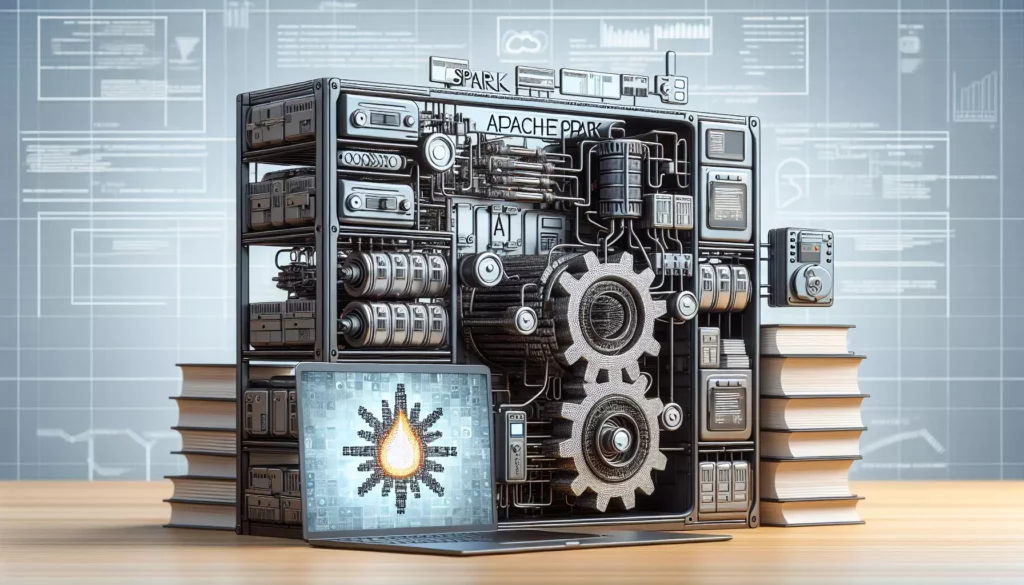
In the ever-evolving landscape of big data and distributed computing, Apache Spark has emerged as a powerful and versatile framework that has revolutionized the way we process and analyze large-scale datasets. Whether you’re a beginner programmer looking to expand your skillset or an experienced data engineer preparing for technical interviews at major tech companies, understanding Spark is crucial in today’s data-driven world.
In this comprehensive guide, we’ll dive deep into Apache Spark, exploring its core concepts, architecture, and key features. We’ll also discuss how Spark fits into the broader ecosystem of big data technologies and why it has become an essential tool for data scientists and engineers alike.
Table of Contents
- Introduction to Apache Spark
- A Brief History of Spark
- Spark Architecture
- Key Components of Spark
- Spark Programming Model
- Common Use Cases for Spark
- Spark vs. Hadoop MapReduce
- Getting Started with Spark
- Best Practices and Optimization Tips
- The Future of Spark
- Conclusion
1. Introduction to Apache Spark
Apache Spark is an open-source, distributed computing system designed for fast and general-purpose data processing. It provides a unified analytics engine for large-scale data processing, capable of handling batch processing, real-time streaming, machine learning, and graph computation.
At its core, Spark aims to address the limitations of traditional MapReduce-based systems by offering:
- In-memory computing for improved performance
- A more flexible and expressive programming model
- Support for a wide range of workloads beyond batch processing
- Easy-to-use APIs in multiple programming languages
Spark’s ability to process data in-memory allows it to perform computations up to 100 times faster than Hadoop MapReduce for certain types of applications, making it an attractive option for organizations dealing with large-scale data processing and analysis.
2. A Brief History of Spark
To truly appreciate Spark’s significance, it’s essential to understand its origins and evolution:
- 2009: Spark was originally developed at the University of California, Berkeley’s AMPLab by Matei Zaharia.
- 2010: The project was open-sourced under a BSD license.
- 2013: Spark was donated to the Apache Software Foundation and became an Apache Top-Level Project.
- 2014: Spark 1.0 was released, marking a significant milestone in its development.
- 2016: Spark 2.0 introduced major performance improvements and new features like Structured Streaming.
- 2020: Spark 3.0 was released, bringing further optimizations and new functionalities.
Throughout its history, Spark has continuously evolved to meet the growing demands of big data processing, incorporating new features and optimizations with each release.
3. Spark Architecture
Understanding Spark’s architecture is crucial for effectively leveraging its capabilities. The Spark architecture consists of several key components:
3.1 Driver Program
The driver program is the entry point of a Spark application. It contains the main() function and defines the distributed datasets on the cluster, as well as applying operations to those datasets.
3.2 Cluster Manager
Spark can run on various cluster managers, including:
- Standalone (Spark’s built-in cluster manager)
- Apache Mesos
- Hadoop YARN
- Kubernetes
The cluster manager is responsible for allocating resources across applications.
3.3 Worker Nodes
Worker nodes are responsible for executing the actual computations and data processing. Each worker node hosts one or more executor processes.
3.4 Executors
Executors are processes launched on worker nodes that run tasks and keep data in memory or disk storage across them. Each application has its own executors.
3.5 Tasks
Tasks are the smallest unit of work in Spark. They are executed by executors and operate on partitions of data.
This distributed architecture allows Spark to efficiently process large datasets across a cluster of machines, providing fault tolerance and scalability.
4. Key Components of Spark
Spark’s ecosystem consists of several integrated components that work together to provide a comprehensive data processing platform:
4.1 Spark Core
Spark Core is the foundation of the entire Spark project. It provides distributed task dispatching, scheduling, and basic I/O functionalities. The main programming abstraction in Spark Core is the Resilient Distributed Dataset (RDD), which represents a collection of elements partitioned across the nodes of the cluster.
4.2 Spark SQL
Spark SQL is a module for structured data processing. It provides a programming abstraction called DataFrames and can also act as a distributed SQL query engine. Spark SQL allows you to query structured data inside Spark programs using either SQL or a familiar DataFrame API.
4.3 Spark Streaming
Spark Streaming enables scalable, high-throughput, fault-tolerant stream processing of live data streams. Data can be ingested from many sources like Kafka, Flume, or TCP sockets and can be processed using complex algorithms expressed with high-level functions like map, reduce, join, and window.
4.4 MLlib (Machine Learning)
MLlib is Spark’s machine learning library. It provides a wide range of machine learning algorithms, including classification, regression, clustering, and collaborative filtering. MLlib also includes utilities for feature extraction, transformation, dimensionality reduction, and model evaluation.
4.5 GraphX
GraphX is a distributed graph processing framework built on top of Spark. It provides an API for expressing graph computation that can model user-defined graphs by using Pregel abstraction.
5. Spark Programming Model
Spark’s programming model is centered around the concept of Resilient Distributed Datasets (RDDs) and more recently, DataFrames and Datasets. Let’s explore these abstractions:
5.1 Resilient Distributed Datasets (RDDs)
RDDs are the fundamental programming abstraction in Spark. An RDD is an immutable, partitioned collection of elements that can be operated on in parallel. RDDs can be created through deterministic operations on either data on stable storage or other RDDs.
Here’s a simple example of creating and operating on an RDD in Python:
from pyspark import SparkContext
sc = SparkContext("local", "Word Count")
text = sc.textFile("path/to/file.txt")
word_counts = text.flatMap(lambda line: line.split(" ")) \
.map(lambda word: (word, 1)) \
.reduceByKey(lambda a, b: a + b)
print(word_counts.collect())
5.2 DataFrames
DataFrames are a distributed collection of data organized into named columns. They are conceptually equivalent to a table in a relational database or a data frame in R/Python, but with richer optimizations under the hood.
Here’s an example of creating and querying a DataFrame in Python:
from pyspark.sql import SparkSession
spark = SparkSession.builder.appName("DataFrame Example").getOrCreate()
df = spark.read.json("path/to/people.json")
df.show()
df.select("name", "age").filter(df["age"] > 21).show()
5.3 Datasets
Datasets are a distributed collection of data that provides the benefits of RDDs (strong typing, ability to use powerful lambda functions) with the benefits of Spark SQL’s optimized execution engine. Datasets are available in Scala and Java APIs.
Here’s a Scala example of working with Datasets:
case class Person(name: String, age: Long)
val peopleDS = spark.read.json("path/to/people.json").as[Person]
peopleDS.show()
peopleDS.filter(person => person.age > 21).show()
6. Common Use Cases for Spark
Apache Spark’s versatility makes it suitable for a wide range of data processing and analysis tasks. Here are some common use cases:
6.1 Batch Processing
Spark excels at processing large volumes of historical data in batch mode. This is useful for tasks like:
- ETL (Extract, Transform, Load) operations
- Data warehousing
- Generating reports and analytics
6.2 Real-time Stream Processing
With Spark Streaming, you can process real-time data streams for:
- Real-time fraud detection
- Log processing and analysis
- Social media sentiment analysis
6.3 Machine Learning
Spark’s MLlib library enables large-scale machine learning for:
- Predictive analytics
- Customer segmentation
- Recommendation systems
6.4 Graph Processing
Using GraphX, Spark can efficiently process and analyze graph-structured data for:
- Social network analysis
- Fraud detection in financial networks
- Route optimization in transportation networks
6.5 Interactive Analytics
Spark’s in-memory processing capabilities make it suitable for interactive data exploration and ad-hoc querying, often used in data science workflows.
7. Spark vs. Hadoop MapReduce
While both Spark and Hadoop MapReduce are designed for distributed data processing, there are several key differences:
7.1 Processing Speed
Spark can be up to 100 times faster than Hadoop MapReduce for certain types of applications, primarily due to its in-memory processing capabilities.
7.2 Ease of Use
Spark provides more user-friendly APIs in multiple languages (Scala, Java, Python, R), making it easier to write complex data processing logic compared to MapReduce.
7.3 Flexibility
Spark supports a wider range of computational models beyond MapReduce, including interactive queries, streaming data processing, machine learning, and graph processing.
7.4 Memory Management
Spark can cache intermediate data in memory, reducing the need for disk I/O and significantly speeding up iterative algorithms.
7.5 Fault Tolerance
Both Spark and Hadoop provide fault tolerance, but Spark achieves this through its RDD lineage graph, which can recreate lost data without the need for replication.
8. Getting Started with Spark
If you’re new to Spark, here’s a quick guide to help you get started:
8.1 Installation
You can download Spark from the official Apache Spark website. Choose the version that matches your Hadoop distribution (if you’re using one) and extract the files to a directory of your choice.
8.2 Setting Up the Environment
Ensure you have Java installed on your system. Set the SPARK_HOME environment variable to point to your Spark installation directory. Add $SPARK_HOME/bin to your PATH.
8.3 Running Spark
You can start the Spark shell for interactive exploration:
- For Scala:
spark-shell
- For Python:
pyspark
8.4 Writing Your First Spark Application
Here’s a simple word count application in PySpark:
from pyspark.sql import SparkSession
spark = SparkSession.builder.appName("WordCount").getOrCreate()
# Read input file
lines = spark.read.text("path/to/input.txt").rdd.map(lambda r: r[0])
# Split lines into words and count
word_counts = lines.flatMap(lambda line: line.split(" ")) \
.map(lambda word: (word, 1)) \
.reduceByKey(lambda a, b: a + b)
# Save the results
word_counts.saveAsTextFile("path/to/output")
spark.stop()
8.5 Submitting a Spark Application
To submit a Spark application, use the spark-submit script:
spark-submit --class org.example.MyApp --master local[*] path/to/my-app.jar
9. Best Practices and Optimization Tips
To get the most out of Spark, consider these best practices and optimization techniques:
9.1 Use the Right Level of Parallelism
Adjust the number of partitions to match the available resources in your cluster. Too few partitions may not fully utilize your cluster, while too many can lead to excessive overhead.
9.2 Minimize Shuffling
Shuffling data across the network is expensive. Use operations like reduceByKey
instead of groupByKey
when possible, as they combine data locally before shuffling.
9.3 Cache Wisely
Use caching (cache()
or persist()
) for RDDs that are reused multiple times, but be mindful of memory usage.
9.4 Use Broadcast Variables
For large shared data that doesn’t change, use broadcast variables to efficiently distribute it to all nodes.
9.5 Optimize Data Serialization
Use Kryo serialization instead of Java serialization for better performance when serializing data.
9.6 Monitor and Tune
Use Spark’s web UI to monitor job execution and identify bottlenecks. Adjust configurations like executor memory and core count based on your workload.
10. The Future of Spark
As big data continues to grow in importance, Spark is evolving to meet new challenges and opportunities:
10.1 Improved Performance
Each new version of Spark brings performance improvements, with ongoing work on query optimization, memory management, and I/O efficiency.
10.2 Better Integration with Cloud Services
Spark is becoming more tightly integrated with cloud platforms, making it easier to run Spark jobs on cloud infrastructure and interact with cloud-native services.
10.3 Enhanced Support for AI and Deep Learning
While Spark already has MLlib for machine learning, there’s ongoing work to improve integration with deep learning frameworks like TensorFlow and PyTorch.
10.4 Simplified APIs and Abstractions
Future versions of Spark may introduce new APIs or abstractions to make it even easier for developers to express complex data processing logic.
10.5 Improved Support for Real-time Processing
As the demand for real-time data processing grows, Spark is likely to enhance its streaming capabilities to handle even lower latency requirements.
11. Conclusion
Apache Spark has revolutionized the big data landscape, providing a powerful, flexible, and user-friendly platform for large-scale data processing and analysis. Its ability to handle diverse workloads—from batch processing to real-time streaming and machine learning—makes it an essential tool for data engineers and scientists alike.
As you continue your journey in coding education and prepare for technical interviews, especially for positions at major tech companies, having a solid understanding of Spark will be a valuable asset. The concepts and skills you learn with Spark—distributed computing, data processing at scale, and working with big data technologies—are highly relevant in today’s data-driven world.
Remember that mastering Spark, like any technology, requires practice and hands-on experience. Start with small projects, experiment with different Spark components, and gradually tackle more complex use cases. As you gain proficiency, you’ll be well-equipped to handle the big data challenges that many organizations face today.
Whether you’re aiming to become a data engineer, a machine learning specialist, or a general software developer working with large-scale systems, your knowledge of Apache Spark will serve you well in your career journey. Keep learning, keep practicing, and embrace the power of big data processing with Spark!