What Is .NET? A Comprehensive Guide to Microsoft’s Development Framework
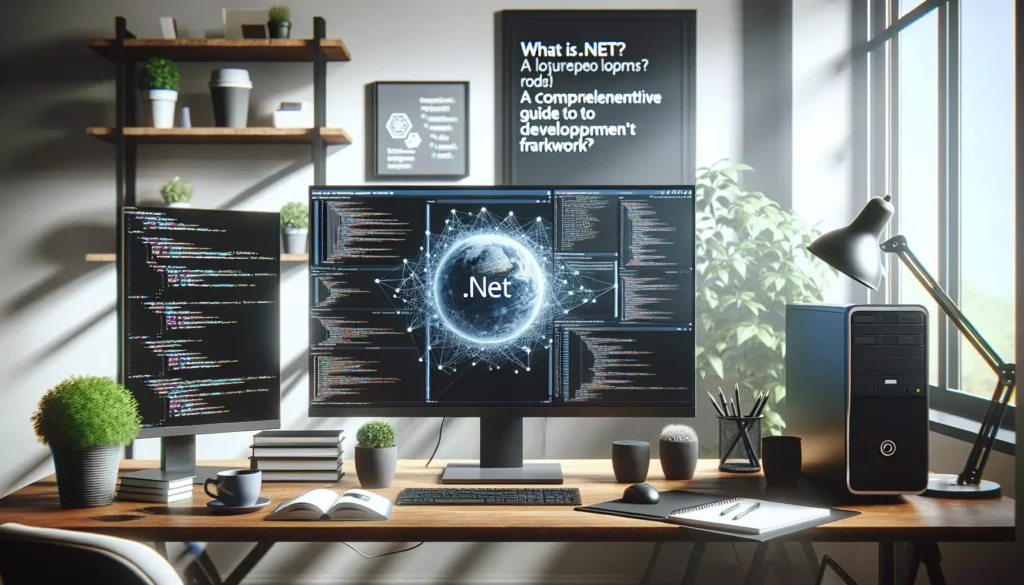
.NET (pronounced “dot net”) is a free, open-source, cross-platform development framework created by Microsoft. It’s designed to build various types of applications, including web, mobile, desktop, cloud, gaming, IoT, and more. In this comprehensive guide, we’ll explore what .NET is, its history, key features, and why it’s an essential tool for modern software development.
Table of Contents
- History of .NET
- Key Components of .NET
- Features of .NET
- Programming Languages in .NET
- Different Versions of .NET
- Use Cases for .NET
- Advantages of Using .NET
- Getting Started with .NET
- The Future of .NET
- Conclusion
History of .NET
The .NET framework was first introduced by Microsoft in 2002 as a proprietary software development platform. It was initially designed to create applications primarily for Windows operating systems. Over the years, .NET has evolved significantly:
- 2002: Initial release of .NET Framework 1.0
- 2006: Introduction of Windows Presentation Foundation (WPF) and Windows Communication Foundation (WCF) in .NET Framework 3.0
- 2010: Release of F# as a supported language
- 2014: Microsoft announces .NET Core, a cross-platform and open-source implementation of .NET
- 2016: First major release of .NET Core 1.0
- 2020: Release of .NET 5, unifying .NET Framework and .NET Core
- 2021: Release of .NET 6, introducing major performance improvements and new features
Today, .NET continues to evolve with regular updates and improvements, maintaining its position as a leading development framework in the software industry.
Key Components of .NET
.NET consists of several key components that work together to provide a comprehensive development environment:
1. Common Language Runtime (CLR)
The CLR is the virtual machine component of .NET that manages the execution of .NET programs. It provides important services such as memory management, security, exception handling, and thread management.
2. Base Class Library (BCL)
The BCL is a comprehensive library of reusable types and methods that developers can use to create applications. It includes classes for common programming tasks such as file I/O, database interaction, XML processing, and more.
3. Application Models
.NET supports various application models, including:
- ASP.NET Core for web applications
- Windows Forms and WPF for desktop applications
- Xamarin for mobile applications
- Unity for game development
4. Development Tools
.NET is supported by a rich ecosystem of development tools, including:
- Visual Studio: Microsoft’s flagship Integrated Development Environment (IDE)
- Visual Studio Code: A lightweight, cross-platform code editor
- JetBrains Rider: A cross-platform .NET IDE
Features of .NET
.NET offers a wide range of features that make it a powerful and versatile framework for software development:
1. Cross-Platform Development
With .NET, developers can build applications that run on Windows, macOS, and Linux, as well as mobile platforms like iOS and Android (using Xamarin).
2. Language Independence
.NET supports multiple programming languages, allowing developers to choose the language they’re most comfortable with or that best suits their project needs.
3. Extensive Class Library
The framework provides a vast collection of pre-built libraries and APIs, saving developers time and effort in implementing common functionalities.
4. Automatic Memory Management
.NET includes a garbage collector that automatically manages memory allocation and deallocation, reducing the risk of memory leaks and other related issues.
5. Security
The framework incorporates various security mechanisms, including code access security and encryption libraries, to help developers build secure applications.
6. Performance
Recent versions of .NET have significantly improved performance, making it competitive with other high-performance languages and frameworks.
Programming Languages in .NET
.NET supports multiple programming languages, each with its own strengths and use cases:
1. C#
C# is the most popular language for .NET development. It’s a modern, object-oriented language with syntax similar to Java and C++. Here’s a simple “Hello, World!” program in C#:
using System;
class Program
{
static void Main()
{
Console.WriteLine("Hello, World!");
}
}
2. F#
F# is a functional-first programming language that also supports object-oriented and imperative programming. It’s particularly well-suited for data science and mathematical computations. Here’s the same “Hello, World!” program in F#:
printfn "Hello, World!"
3. Visual Basic .NET
Visual Basic .NET is an object-oriented language with syntax that’s often considered more accessible for beginners. Here’s the “Hello, World!” program in Visual Basic .NET:
Imports System
Module Module1
Sub Main()
Console.WriteLine("Hello, World!")
End Sub
End Module
While these are the primary languages supported by .NET, the framework’s language-independent nature means that other languages can also be used with .NET, including IronPython and IronRuby.
Different Versions of .NET
Over the years, .NET has evolved into different versions and implementations:
1. .NET Framework
This is the original implementation of .NET, designed primarily for Windows. It’s still maintained but is no longer the focus of new development.
2. .NET Core
Introduced in 2016, .NET Core was a cross-platform, open-source reimplementation of .NET. It was designed to address the limitations of the .NET Framework and enable .NET development on non-Windows platforms.
3. .NET 5 and Beyond
Starting with .NET 5 in 2020, Microsoft unified the various .NET implementations into a single, cross-platform framework simply called “.NET”. This is now the main implementation and the focus of ongoing development.
4. Mono
Mono is an open-source implementation of .NET that predates .NET Core. It’s still used in some contexts, particularly in conjunction with Xamarin for mobile development.
Use Cases for .NET
.NET’s versatility makes it suitable for a wide range of applications:
1. Web Development
ASP.NET Core is a popular choice for building web applications and APIs. It offers high performance and can be used to create everything from simple websites to complex, scalable web applications.
2. Desktop Applications
Windows Forms and Windows Presentation Foundation (WPF) are commonly used for building Windows desktop applications. With .NET 6, developers can also create cross-platform desktop apps using .NET MAUI.
3. Mobile Development
Xamarin, now part of .NET 6 as .NET MAUI, allows developers to build native mobile applications for iOS and Android using C# and .NET.
4. Cloud Computing
.NET is well-integrated with Microsoft Azure, making it an excellent choice for building cloud-based applications and services.
5. Game Development
While not as common as engines like Unity (which itself uses C#), .NET can be used for game development, particularly for Windows games or cross-platform games using MonoGame.
6. Internet of Things (IoT)
.NET can be used to develop applications for IoT devices, especially with .NET nanoFramework for resource-constrained devices.
Advantages of Using .NET
.NET offers several advantages that make it an attractive choice for developers and organizations:
1. Productivity
The extensive class library and high-level abstractions in .NET allow developers to write less code and focus more on solving business problems.
2. Performance
Recent versions of .NET have seen significant performance improvements, making it competitive with traditionally faster languages like C++ for many use cases.
3. Cross-Platform Compatibility
With .NET 5 and later, developers can write code once and run it on multiple platforms, including Windows, macOS, and Linux.
4. Strong Typing
The use of strong typing in .NET languages like C# helps catch errors at compile-time, leading to more robust and less error-prone code.
5. Large Community and Ecosystem
.NET has a large, active community and a vast ecosystem of libraries and tools available through NuGet, Microsoft’s package manager for .NET.
6. Microsoft Support
As a Microsoft product, .NET benefits from strong support, regular updates, and integration with other Microsoft technologies and services.
Getting Started with .NET
If you’re new to .NET, here’s how you can get started:
1. Install the .NET SDK
Download and install the .NET SDK from the official Microsoft website. This will give you the tools you need to develop .NET applications.
2. Choose an IDE
While you can use any text editor to write .NET code, an IDE can greatly enhance your productivity. Visual Studio is the most feature-rich option, but Visual Studio Code with the C# extension is a popular, lightweight alternative.
3. Create Your First Project
You can create a new .NET project using the command line or your IDE. Here’s how to create a new console application using the command line:
dotnet new console -n MyFirstApp
cd MyFirstApp
dotnet run
4. Learn the Basics
Start with the basics of C# (or your chosen .NET language) and gradually move on to more advanced topics. Microsoft’s documentation and tutorials are excellent resources for learning .NET.
5. Explore .NET’s Features
As you become more comfortable with the basics, start exploring .NET’s various features and libraries. Try building different types of applications to get a feel for the framework’s versatility.
The Future of .NET
The future of .NET looks bright, with Microsoft continuing to invest heavily in its development. Some trends and developments to watch for include:
1. Continued Performance Improvements
Microsoft is consistently working on improving .NET’s performance, with each new version bringing significant enhancements.
2. Enhanced Cross-Platform Development
With .NET MAUI (Multi-platform App UI), Microsoft is pushing for even better cross-platform development experiences, allowing developers to create desktop and mobile apps from a single codebase.
3. Integration with Emerging Technologies
Expect to see better integration with emerging technologies like AI, machine learning, and IoT in future .NET releases.
4. Focus on Cloud-Native Development
As cloud computing continues to grow, .NET is likely to offer more features and tools for cloud-native development, particularly in conjunction with Azure.
Conclusion
.NET has come a long way since its introduction in 2002. Today, it stands as a powerful, versatile, and modern development framework capable of building a wide range of applications across multiple platforms. Its cross-platform capabilities, performance improvements, and strong ecosystem make it an excellent choice for developers and organizations alike.
Whether you’re a seasoned developer or just starting your coding journey, .NET offers a rich set of tools and resources to help you build robust, efficient, and scalable applications. As the framework continues to evolve, it’s likely to remain a key player in the software development landscape for years to come.
By understanding what .NET is and what it offers, you’re well-equipped to leverage its power in your own development projects. Whether you’re building web applications, mobile apps, desktop software, or cloud services, .NET provides the tools and capabilities you need to bring your ideas to life.