What Is JSON? A Comprehensive Guide for Beginners and Developers
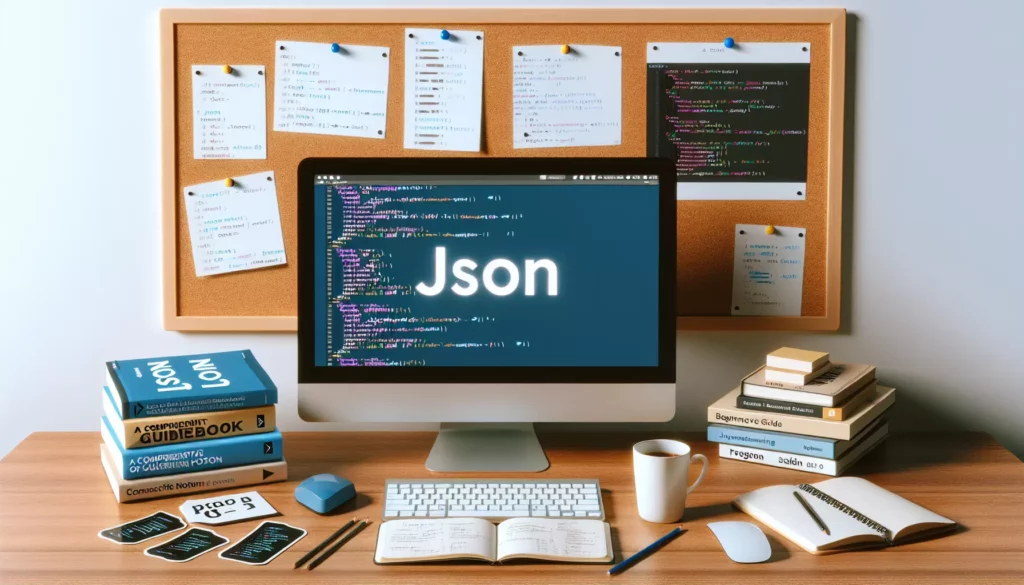
In the world of web development and data exchange, JSON (JavaScript Object Notation) has become an indispensable tool. Whether you’re a beginner just starting your coding journey or an experienced developer looking to brush up on your skills, understanding JSON is crucial. In this comprehensive guide, we’ll explore what JSON is, its syntax, use cases, and how it fits into the broader landscape of coding and data interchange.
Table of Contents
- What is JSON?
- JSON Syntax
- JSON Data Types
- JSON vs. XML
- Using JSON in Programming
- JSON Best Practices
- JSON Tools and Resources
- JSON in APIs
- JSON Security Considerations
- Conclusion
What is JSON?
JSON, which stands for JavaScript Object Notation, is a lightweight data interchange format. It was derived from JavaScript but is language-independent, making it a versatile choice for storing and exchanging data. JSON is human-readable, easy to understand, and can be parsed by machines efficiently.
Created by Douglas Crockford in the early 2000s, JSON quickly gained popularity due to its simplicity and effectiveness in transmitting data between a server and a web application. Today, it’s widely used in various programming languages and has become the de facto standard for API responses and configuration files.
JSON Syntax
JSON syntax is straightforward and based on two structures:
- A collection of name/value pairs (similar to an object in many programming languages)
- An ordered list of values (similar to an array)
Here’s a basic example of JSON syntax:
{
"name": "John Doe",
"age": 30,
"city": "New York",
"hobbies": ["reading", "swimming", "coding"],
"isStudent": false
}
In this example, we have a JSON object enclosed in curly braces {}. The object contains key-value pairs separated by commas. Each key is a string enclosed in double quotes, followed by a colon and its corresponding value.
JSON Data Types
JSON supports several data types, which cover most common programming needs:
- String: A sequence of characters enclosed in double quotes. Example:
"Hello, World!"
- Number: Integers or floating-point numbers. Example:
42
or3.14
- Boolean: Either
true
orfalse
- Array: An ordered list of values enclosed in square brackets []. Example:
[1, 2, 3, 4]
- Object: A collection of key-value pairs enclosed in curly braces {}.
- null: Represents a null value.
It’s important to note that JSON does not support some data types found in programming languages, such as functions, dates, or undefined values. When working with these types, you’ll need to convert them to supported JSON types or use custom serialization techniques.
JSON vs. XML
Before JSON became popular, XML (eXtensible Markup Language) was the primary format for data interchange. While both JSON and XML serve similar purposes, JSON has several advantages:
- Simplicity: JSON has a simpler syntax and is easier to read and write.
- Lightweight: JSON typically requires less markup, resulting in smaller file sizes and faster transmission.
- Parsing Speed: JSON is generally faster to parse than XML, especially in browsers.
- Native JavaScript Support: JSON can be easily parsed into JavaScript objects without additional libraries.
Here’s a comparison of the same data represented in JSON and XML:
JSON:
{
"person": {
"name": "John Doe",
"age": 30,
"city": "New York"
}
}
XML:
<person>
<name>John Doe</name>
<age>30</age>
<city>New York</city>
</person>
As you can see, the JSON representation is more concise and easier to read at a glance.
Using JSON in Programming
JSON is supported by most modern programming languages, either natively or through libraries. Here are some examples of how to work with JSON in popular languages:
JavaScript
In JavaScript, JSON is natively supported. You can parse JSON strings into JavaScript objects and stringify JavaScript objects into JSON:
// Parsing JSON
const jsonString = '{"name": "John", "age": 30}';
const obj = JSON.parse(jsonString);
console.log(obj.name); // Output: John
// Stringifying an object
const person = { name: "Jane", age: 25 };
const jsonStr = JSON.stringify(person);
console.log(jsonStr); // Output: {"name":"Jane","age":25}
Python
Python has a built-in json
module for working with JSON data:
import json
# Parsing JSON
json_string = '{"name": "John", "age": 30}'
data = json.loads(json_string)
print(data['name']) # Output: John
# Converting to JSON
person = {"name": "Jane", "age": 25}
json_str = json.dumps(person)
print(json_str) # Output: {"name": "Jane", "age": 25}
Java
In Java, you can use libraries like Jackson or Gson to work with JSON. Here’s an example using Jackson:
import com.fasterxml.jackson.databind.ObjectMapper;
// Parsing JSON
String jsonString = "{\"name\": \"John\", \"age\": 30}";
ObjectMapper mapper = new ObjectMapper();
JsonNode node = mapper.readTree(jsonString);
System.out.println(node.get("name").asText()); // Output: John
// Converting to JSON
Person person = new Person("Jane", 25);
String json = mapper.writeValueAsString(person);
System.out.println(json); // Output: {"name":"Jane","age":25}
JSON Best Practices
When working with JSON, consider these best practices to ensure your data is efficient, readable, and maintainable:
- Use Consistent Naming Conventions: Choose a naming convention (e.g., camelCase or snake_case) and stick to it throughout your JSON structures.
- Keep It Simple: Avoid overly complex nested structures. If your JSON becomes too deep, consider refactoring or splitting it into smaller, more manageable pieces.
- Validate JSON: Always validate your JSON data to ensure it’s well-formed and follows your expected schema.
- Use Appropriate Data Types: Choose the correct data type for your values (e.g., use numbers for numeric values instead of strings).
- Handle Dates Carefully: Since JSON doesn’t have a native date type, use a consistent format (like ISO 8601) for date strings.
- Minimize Data: Only include necessary data to keep your JSON payload small and efficient.
- Use Compression: For large JSON payloads, consider using compression techniques like GZIP to reduce transmission size.
JSON Tools and Resources
Several tools and resources can help you work with JSON more effectively:
- JSON Validators: Tools like JSONLint help you validate and format your JSON.
- JSON Editors: Online editors like JSON Editor Online provide a visual interface for editing and viewing JSON data.
- JSON Schema: Use JSON Schema to define the structure of your JSON data and validate it against a schema.
- JSON Path: JSONPath is a query language for JSON, similar to XPath for XML.
- JSON-LD: JSON for Linking Data is a method of encoding linked data using JSON.
JSON in APIs
JSON has become the standard format for API responses due to its simplicity and efficiency. When designing APIs that use JSON:
- Use Consistent Response Structures: Maintain a consistent format for your API responses, including error messages.
- Versioning: Include version information in your API responses to help manage changes over time.
- Pagination: For large datasets, implement pagination and include metadata about the current page and total results.
- HATEOAS: Consider implementing HATEOAS (Hypermedia as the Engine of Application State) principles by including relevant links in your JSON responses.
Here’s an example of a well-structured JSON API response:
{
"apiVersion": "1.0",
"data": {
"users": [
{ "id": 1, "name": "John Doe", "email": "john@example.com" },
{ "id": 2, "name": "Jane Smith", "email": "jane@example.com" }
]
},
"pagination": {
"currentPage": 1,
"totalPages": 5,
"itemsPerPage": 2,
"totalItems": 10
},
"links": {
"self": "https://api.example.com/users?page=1",
"next": "https://api.example.com/users?page=2",
"last": "https://api.example.com/users?page=5"
}
}
JSON Security Considerations
While JSON itself is a data format and not inherently insecure, there are some security considerations to keep in mind when working with JSON data:
- JSON Injection: Be cautious when parsing JSON from untrusted sources. Use proper input validation and sanitization to prevent potential injection attacks.
- Sensitive Data: Avoid storing sensitive information (like passwords or API keys) directly in JSON. If necessary, use encryption or secure storage methods.
- JSONP Vulnerabilities: If using JSONP (JSON with Padding), be aware of potential security risks and consider using more secure alternatives like CORS.
- Object Serialization: When deserializing JSON into objects, be cautious about which classes you allow to be instantiated to prevent potential remote code execution vulnerabilities.
Conclusion
JSON has revolutionized the way we exchange data in web applications and APIs. Its simplicity, readability, and wide support across programming languages make it an essential tool for modern developers. By understanding JSON’s syntax, best practices, and use cases, you’ll be well-equipped to work with data in various programming contexts.
As you continue your coding journey, whether you’re a beginner or an experienced developer, mastering JSON will prove invaluable. It’s a fundamental skill that will serve you well in web development, API design, data storage, and many other areas of software engineering.
Remember to practice working with JSON in your preferred programming language, explore JSON-related tools and libraries, and stay updated on best practices and security considerations. With JSON in your toolkit, you’ll be better prepared to tackle complex data interchange challenges and build robust, efficient applications.