What Is Deep Learning? A Comprehensive Guide for Beginners
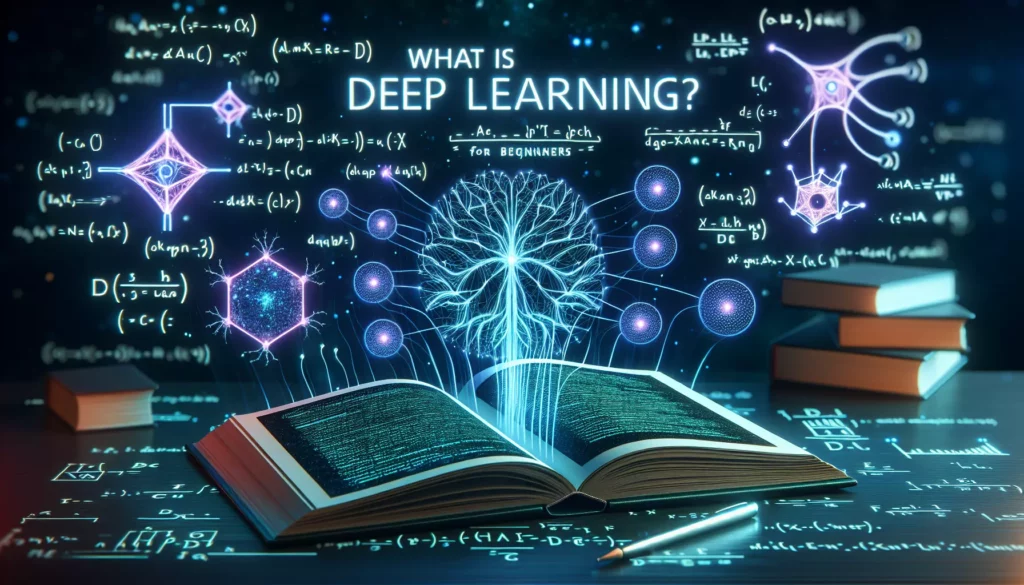
In the rapidly evolving world of technology, deep learning has emerged as a powerful subset of machine learning that’s revolutionizing various industries. From self-driving cars to voice assistants, deep learning is behind many of the AI-powered innovations we encounter daily. But what exactly is deep learning, and how does it work? In this comprehensive guide, we’ll explore the fundamentals of deep learning, its applications, and its significance in the field of artificial intelligence.
Understanding Deep Learning
Deep learning is a subset of machine learning, which itself is a branch of artificial intelligence (AI). To understand deep learning, it’s essential to first grasp the basics of machine learning.
Machine Learning vs. Deep Learning
Machine learning is a method of data analysis that automates analytical model building. It’s based on the idea that systems can learn from data, identify patterns, and make decisions with minimal human intervention. Deep learning, on the other hand, is a more advanced technique within machine learning that uses neural networks with multiple layers (hence the term “deep”) to analyze various factors of data.
The key difference between traditional machine learning and deep learning lies in how features are extracted:
- In traditional machine learning, features are manually engineered by domain experts.
- In deep learning, the system automatically learns to identify the best features without human intervention.
Neural Networks: The Foundation of Deep Learning
At the core of deep learning are artificial neural networks, which are inspired by the structure and function of the human brain. These networks consist of interconnected nodes (neurons) organized in layers:
- Input Layer: Receives the initial data
- Hidden Layers: Process the data through a series of transformations
- Output Layer: Produces the final result or prediction
The “deep” in deep learning refers to the number of hidden layers in the neural network. While a traditional neural network might have only one or two hidden layers, deep learning models can have hundreds.
How Deep Learning Works
Deep learning works by processing data through multiple layers of artificial neural networks. Each layer learns to identify different features of the data, with higher layers building upon the features recognized by lower layers to form a hierarchical representation.
The Learning Process
The learning process in deep learning involves the following steps:
- Forward Propagation: Input data is fed into the network and processed through each layer.
- Loss Calculation: The network’s output is compared to the desired output, and the difference (loss) is calculated.
- Backpropagation: The error is propagated back through the network, and the weights of the connections between neurons are adjusted to minimize the loss.
- Iteration: This process is repeated many times with large amounts of data until the network achieves satisfactory performance.
Types of Deep Learning Networks
There are several types of deep learning networks, each designed for specific tasks:
- Convolutional Neural Networks (CNNs): Primarily used for image and video processing
- Recurrent Neural Networks (RNNs): Ideal for sequential data like time series or natural language
- Long Short-Term Memory Networks (LSTMs): A type of RNN that can learn long-term dependencies
- Generative Adversarial Networks (GANs): Used for generating new, synthetic instances of data
Applications of Deep Learning
Deep learning has found applications across various industries and domains:
1. Computer Vision
Deep learning has revolutionized computer vision tasks such as:
- Image classification
- Object detection
- Facial recognition
- Autonomous vehicles
2. Natural Language Processing (NLP)
Deep learning models have significantly improved NLP tasks, including:
- Machine translation
- Sentiment analysis
- Chatbots and virtual assistants
- Text summarization
3. Speech Recognition
Deep learning has enhanced speech recognition systems, enabling:
- Voice assistants (e.g., Siri, Alexa)
- Transcription services
- Voice-controlled devices
4. Healthcare
In the medical field, deep learning is being used for:
- Disease diagnosis from medical images
- Drug discovery
- Personalized medicine
5. Finance
Deep learning applications in finance include:
- Fraud detection
- Algorithmic trading
- Risk assessment
Advantages of Deep Learning
Deep learning offers several advantages over traditional machine learning approaches:
1. Automatic Feature Extraction
Deep learning models can automatically learn the most important features from raw data, eliminating the need for manual feature engineering.
2. Scalability
Deep learning models often improve in performance as they are exposed to more data, making them highly scalable for big data applications.
3. Versatility
The same deep learning architecture can be applied to various types of data and problems with minimal modifications.
4. Continuous Improvement
Deep learning models can continue to learn and improve their performance as they are exposed to new data over time.
Challenges in Deep Learning
Despite its power and versatility, deep learning also faces several challenges:
1. Data Requirements
Deep learning models typically require large amounts of labeled data to achieve high performance, which can be expensive and time-consuming to obtain.
2. Computational Resources
Training deep learning models often requires significant computational power, including specialized hardware like GPUs.
3. Interpretability
Deep learning models are often considered “black boxes,” making it difficult to understand how they arrive at their decisions. This lack of interpretability can be problematic in sensitive applications like healthcare or finance.
4. Overfitting
Deep learning models with many parameters are prone to overfitting, where they perform well on training data but poorly on new, unseen data.
Getting Started with Deep Learning
If you’re interested in exploring deep learning, here are some steps to get started:
1. Learn the Fundamentals
Start by understanding the basics of machine learning, linear algebra, and calculus. These foundational concepts are crucial for grasping deep learning principles.
2. Choose a Programming Language
Python is the most popular language for deep learning due to its extensive libraries and frameworks. Some key libraries include:
- TensorFlow
- PyTorch
- Keras
3. Study Neural Network Architectures
Familiarize yourself with different types of neural networks and their applications.
4. Practice with Datasets
Start with simple projects using well-known datasets like MNIST for image classification or IMDb for sentiment analysis.
5. Stay Updated
The field of deep learning is rapidly evolving. Stay current by following research papers, attending conferences, and participating in online communities.
Deep Learning in Coding Education
As we explore deep learning, it’s important to consider its role in coding education and how it relates to platforms like AlgoCademy. While deep learning itself may not be a primary focus for beginners learning to code, understanding its principles can be valuable for several reasons:
1. Algorithmic Thinking
Deep learning models rely on complex algorithms and data structures. Learning about these can enhance a programmer’s ability to think algorithmically and solve complex problems efficiently.
2. Data Manipulation
Working with deep learning often involves handling large datasets. This experience can improve a programmer’s skills in data manipulation and preprocessing, which are valuable in many areas of software development.
3. Performance Optimization
Training deep learning models requires optimizing code for performance. This focus on efficiency can help programmers write better, more optimized code in general.
4. Interdisciplinary Applications
Understanding deep learning can open up opportunities to work on cutting-edge projects that combine programming with fields like computer vision, natural language processing, or robotics.
5. AI-Powered Tools
Many coding education platforms, including AlgoCademy, use AI-powered tools to assist learners. Understanding the basics of deep learning can help students better appreciate and utilize these tools.
Implementing a Simple Neural Network
To give you a practical understanding of deep learning, let’s implement a simple neural network using Python and the NumPy library. This example will create a basic feedforward neural network with one hidden layer to solve a binary classification problem.
import numpy as np
# Activation function (Sigmoid)
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# Derivative of Sigmoid function
def sigmoid_derivative(x):
return x * (1 - x)
# Neural Network class
class NeuralNetwork:
def __init__(self, x, y):
self.input = x
self.weights1 = np.random.rand(self.input.shape[1], 4)
self.weights2 = np.random.rand(4, 1)
self.y = y
self.output = np.zeros(y.shape)
def feedforward(self):
self.layer1 = sigmoid(np.dot(self.input, self.weights1))
self.output = sigmoid(np.dot(self.layer1, self.weights2))
def backprop(self):
d_weights2 = np.dot(self.layer1.T, 2 * (self.y - self.output) * sigmoid_derivative(self.output))
d_weights1 = np.dot(self.input.T, np.dot(2 * (self.y - self.output) * sigmoid_derivative(self.output), self.weights2.T) * sigmoid_derivative(self.layer1))
self.weights1 += d_weights1
self.weights2 += d_weights2
def train(self, iterations):
for _ in range(iterations):
self.feedforward()
self.backprop()
# Example usage
if __name__ == "__main__":
X = np.array([[0,0,1], [0,1,1], [1,0,1], [1,1,1]])
y = np.array([[0], [1], [1], [0]])
nn = NeuralNetwork(X, y)
nn.train(1500)
print(nn.output)
This simple implementation demonstrates the basic concepts of feedforward propagation and backpropagation in a neural network. While it’s not a deep learning model (as it only has one hidden layer), it serves as a starting point for understanding how neural networks learn from data.
Conclusion
Deep learning is a powerful and rapidly evolving field that has the potential to revolutionize many aspects of our lives. By mimicking the human brain’s neural networks, deep learning algorithms can process vast amounts of data and learn to perform complex tasks with remarkable accuracy.
As we’ve explored in this guide, deep learning has applications across various industries, from computer vision and natural language processing to healthcare and finance. Its ability to automatically extract features from raw data and scale with increasing amounts of information makes it a valuable tool for solving complex problems.
However, it’s important to remember that deep learning is not a magic solution for all problems. It comes with challenges such as the need for large amounts of data, significant computational resources, and issues with interpretability. As the field continues to advance, researchers and practitioners are working to address these challenges and expand the capabilities of deep learning systems.
For those interested in coding and software development, understanding deep learning can provide valuable insights into advanced algorithmic thinking, data manipulation, and the cutting-edge applications of computer science. While platforms like AlgoCademy focus on building foundational coding skills and preparing for technical interviews, the principles learned in deep learning can enhance a programmer’s problem-solving abilities and open up exciting career opportunities in AI and machine learning.
As you continue your journey in programming and computer science, keep an eye on the developments in deep learning. Whether you choose to specialize in this field or simply want to understand its impact on technology, the knowledge you gain will be invaluable in our increasingly AI-driven world.