What Is CRUD? Understanding the Backbone of Web Development
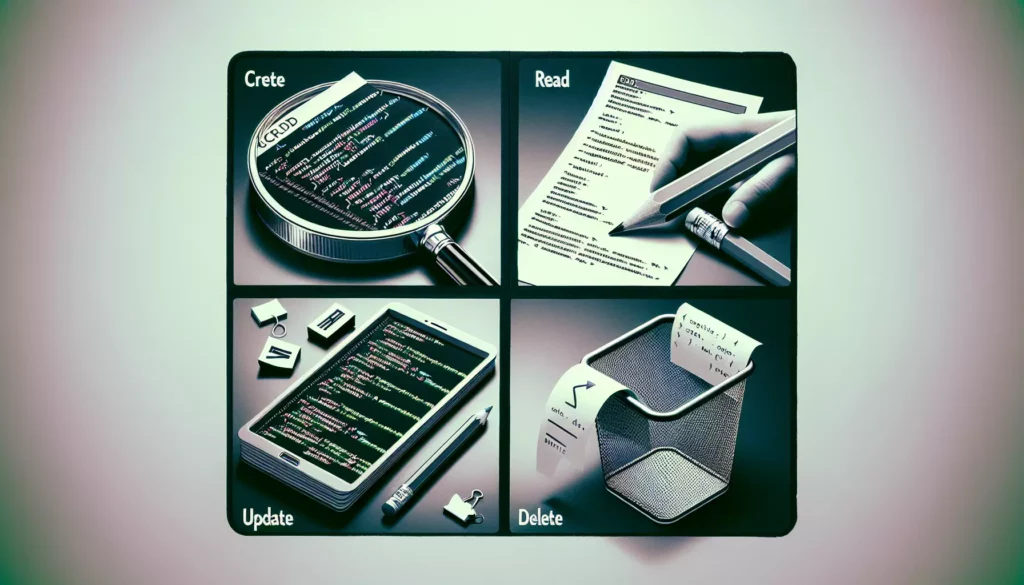
In the world of web development and database management, CRUD is a fundamental concept that every aspiring programmer should understand. Whether you’re just starting your coding journey or preparing for technical interviews at major tech companies, grasping CRUD operations is essential. In this comprehensive guide, we’ll dive deep into what CRUD is, why it’s important, and how it’s implemented in various programming contexts.
Table of Contents
- What Is CRUD?
- The Importance of CRUD in Web Development
- Breaking Down CRUD Operations
- CRUD in Database Management
- CRUD and RESTful APIs
- Implementing CRUD in Different Programming Languages
- CRUD Best Practices and Design Patterns
- Security Considerations in CRUD Operations
- CRUD vs. Other Data Manipulation Patterns
- CRUD in Modern Web Frameworks
- Testing CRUD Functionality
- Conclusion
What Is CRUD?
CRUD is an acronym that stands for Create, Read, Update, and Delete. These four operations represent the basic functions that models should be able to perform and are considered necessary to implement a persistent storage application. Let’s break down each component:
- Create: The operation that adds new data to a database or system.
- Read: The operation that retrieves existing data from a database or system.
- Update: The operation that modifies existing data in a database or system.
- Delete: The operation that removes existing data from a database or system.
CRUD operations are the foundation of data manipulation in most software applications. They provide a memorable framework for developers to design the user interfaces, database structures, and APIs that power modern web and mobile applications.
The Importance of CRUD in Web Development
Understanding CRUD is crucial for several reasons:
- Universal Application: CRUD operations are used in virtually every application that involves storing and retrieving data, from simple to-do lists to complex enterprise systems.
- Database Interaction: CRUD provides a standardized way to interact with databases, making it easier to design and implement data storage solutions.
- API Design: RESTful APIs, which are the backbone of many web services, are often structured around CRUD principles.
- User Interface Design: CRUD operations often map directly to user interface elements, helping developers create intuitive user experiences.
- Simplicity and Clarity: The CRUD model simplifies complex data manipulations into four basic operations, making it easier to understand and implement data management systems.
For those preparing for technical interviews, especially at FAANG companies (Facebook, Amazon, Apple, Netflix, Google), having a solid grasp of CRUD concepts is essential. These operations form the basis of many interview questions and real-world programming scenarios.
Breaking Down CRUD Operations
Let’s explore each CRUD operation in more detail:
Create
The Create operation is used to add new records to a database or system. In a web application, this might involve:
- Inserting a new user into a database after they sign up
- Adding a new product to an e-commerce inventory
- Creating a new blog post in a content management system
In SQL, the CREATE operation typically uses the INSERT statement:
INSERT INTO users (username, email, password) VALUES ('johndoe', 'john@example.com', 'hashedpassword');
Read
The Read operation retrieves existing data from the system. This could involve:
- Fetching user details for a profile page
- Retrieving a list of products for a category page
- Loading the content of a blog post
In SQL, the READ operation uses the SELECT statement:
SELECT * FROM users WHERE username = 'johndoe';
Update
The Update operation modifies existing records. Examples include:
- Changing a user’s password or email address
- Updating the price or quantity of a product
- Editing the content of a blog post
In SQL, the UPDATE operation uses the UPDATE statement:
UPDATE users SET email = 'newemail@example.com' WHERE username = 'johndoe';
Delete
The Delete operation removes records from the system. This might involve:
- Deleting a user account
- Removing a product from inventory
- Deleting a blog post
In SQL, the DELETE operation uses the DELETE statement:
DELETE FROM users WHERE username = 'johndoe';
CRUD in Database Management
In database management systems, CRUD operations are fundamental. They map directly to SQL commands and are essential for maintaining data integrity and consistency. Here’s how CRUD operations typically correspond to SQL commands:
- Create → INSERT
- Read → SELECT
- Update → UPDATE
- Delete → DELETE
However, it’s important to note that CRUD is not limited to SQL databases. NoSQL databases, such as MongoDB, also implement CRUD operations, albeit with different syntax and methodologies.
For instance, in MongoDB, CRUD operations might look like this:
// Create
db.users.insertOne({ username: "johndoe", email: "john@example.com" })
// Read
db.users.find({ username: "johndoe" })
// Update
db.users.updateOne({ username: "johndoe" }, { $set: { email: "newemail@example.com" } })
// Delete
db.users.deleteOne({ username: "johndoe" })
CRUD and RESTful APIs
RESTful APIs (Representational State Transfer) are designed around resources and use HTTP methods to manipulate these resources. CRUD operations map neatly to HTTP methods in RESTful APIs:
- Create → POST
- Read → GET
- Update → PUT/PATCH
- Delete → DELETE
For example, a RESTful API for a user management system might have these endpoints:
POST /users // Create a new user
GET /users/{id} // Read user details
PUT /users/{id} // Update user details
DELETE /users/{id} // Delete a user
Understanding this mapping is crucial for developers working on web services and APIs, as it forms the basis of many modern web architectures.
Implementing CRUD in Different Programming Languages
CRUD operations can be implemented in various programming languages. Let’s look at some examples:
Python with SQLite
import sqlite3
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
# Create
cursor.execute("INSERT INTO users (username, email) VALUES (?, ?)", ('johndoe', 'john@example.com'))
# Read
cursor.execute("SELECT * FROM users WHERE username = ?", ('johndoe',))
user = cursor.fetchone()
# Update
cursor.execute("UPDATE users SET email = ? WHERE username = ?", ('newemail@example.com', 'johndoe'))
# Delete
cursor.execute("DELETE FROM users WHERE username = ?", ('johndoe',))
conn.commit()
conn.close()
JavaScript with Node.js and MongoDB
const MongoClient = require('mongodb').MongoClient;
const url = "mongodb://localhost:27017/";
MongoClient.connect(url, function(err, db) {
if (err) throw err;
const dbo = db.db("mydb");
// Create
dbo.collection("users").insertOne({username: "johndoe", email: "john@example.com"}, function(err, res) {
if (err) throw err;
console.log("1 document inserted");
});
// Read
dbo.collection("users").findOne({username: "johndoe"}, function(err, result) {
if (err) throw err;
console.log(result);
});
// Update
dbo.collection("users").updateOne({username: "johndoe"}, {$set: {email: "newemail@example.com"}}, function(err, res) {
if (err) throw err;
console.log("1 document updated");
});
// Delete
dbo.collection("users").deleteOne({username: "johndoe"}, function(err, obj) {
if (err) throw err;
console.log("1 document deleted");
});
db.close();
});
Java with JDBC
import java.sql.*;
public class CRUDExample {
public static void main(String[] args) {
try {
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password");
// Create
PreparedStatement createStmt = conn.prepareStatement("INSERT INTO users (username, email) VALUES (?, ?)");
createStmt.setString(1, "johndoe");
createStmt.setString(2, "john@example.com");
createStmt.executeUpdate();
// Read
PreparedStatement readStmt = conn.prepareStatement("SELECT * FROM users WHERE username = ?");
readStmt.setString(1, "johndoe");
ResultSet rs = readStmt.executeQuery();
while (rs.next()) {
System.out.println(rs.getString("email"));
}
// Update
PreparedStatement updateStmt = conn.prepareStatement("UPDATE users SET email = ? WHERE username = ?");
updateStmt.setString(1, "newemail@example.com");
updateStmt.setString(2, "johndoe");
updateStmt.executeUpdate();
// Delete
PreparedStatement deleteStmt = conn.prepareStatement("DELETE FROM users WHERE username = ?");
deleteStmt.setString(1, "johndoe");
deleteStmt.executeUpdate();
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
CRUD Best Practices and Design Patterns
When implementing CRUD operations, it’s important to follow best practices to ensure your code is efficient, maintainable, and secure:
- Use Prepared Statements: To prevent SQL injection attacks, always use prepared statements or parameterized queries when interacting with databases.
- Implement Data Validation: Validate and sanitize input data before performing CRUD operations to maintain data integrity and prevent errors.
- Handle Errors Gracefully: Implement proper error handling and logging to make debugging easier and improve user experience.
- Use Transactions: When performing multiple related CRUD operations, use database transactions to ensure data consistency.
- Implement Access Control: Ensure that users have the appropriate permissions before allowing them to perform CRUD operations.
- Follow the Single Responsibility Principle: Separate your CRUD logic from other parts of your application to improve maintainability.
- Use ORM Tools: Consider using Object-Relational Mapping (ORM) tools to abstract away the complexity of database operations and improve code readability.
Security Considerations in CRUD Operations
Security is paramount when implementing CRUD operations. Here are some key security considerations:
- Input Validation: Always validate and sanitize user input to prevent injection attacks and ensure data integrity.
- Authentication and Authorization: Implement robust user authentication and role-based access control to ensure users can only perform CRUD operations they’re authorized for.
- Use HTTPS: When performing CRUD operations over a network, always use HTTPS to encrypt data in transit.
- Implement Rate Limiting: To prevent abuse, implement rate limiting on your API endpoints that perform CRUD operations.
- Audit Logging: Keep detailed logs of all CRUD operations for security auditing and troubleshooting purposes.
- Data Encryption: Consider encrypting sensitive data at rest in your database.
CRUD vs. Other Data Manipulation Patterns
While CRUD is widely used, it’s not the only pattern for data manipulation. Here are some alternatives and how they compare to CRUD:
CQRS (Command Query Responsibility Segregation)
CQRS separates read and write operations into different models. This can be beneficial for complex systems with different read and write workloads.
Event Sourcing
Instead of storing just the current state, event sourcing stores a sequence of events. This provides a complete audit trail and allows for complex event replay and system reconstruction.
BREAD (Browse, Read, Edit, Add, Delete)
BREAD is an extension of CRUD that adds a “Browse” operation, which is useful for displaying lists or collections of data.
While these patterns have their use cases, CRUD remains the most straightforward and widely applicable pattern for most applications.
CRUD in Modern Web Frameworks
Modern web frameworks often provide built-in support or utilities for implementing CRUD operations. Here are a few examples:
Ruby on Rails
Rails provides scaffolding that can automatically generate CRUD operations for a resource:
rails generate scaffold User name:string email:string
Django (Python)
Django’s class-based views provide generic views for CRUD operations:
from django.views.generic import ListView, DetailView, CreateView, UpdateView, DeleteView
class UserListView(ListView):
model = User
class UserDetailView(DetailView):
model = User
class UserCreateView(CreateView):
model = User
fields = ['name', 'email']
class UserUpdateView(UpdateView):
model = User
fields = ['name', 'email']
class UserDeleteView(DeleteView):
model = User
success_url = reverse_lazy('user-list')
Express.js (Node.js)
While Express doesn’t provide built-in CRUD operations, it’s common to implement them using middleware and routing:
const express = require('express');
const router = express.Router();
// Create
router.post('/users', (req, res) => {
// Create user logic
});
// Read
router.get('/users/:id', (req, res) => {
// Read user logic
});
// Update
router.put('/users/:id', (req, res) => {
// Update user logic
});
// Delete
router.delete('/users/:id', (req, res) => {
// Delete user logic
});
Testing CRUD Functionality
Testing CRUD operations is crucial to ensure your application works correctly. Here are some approaches to testing CRUD functionality:
Unit Testing
Write unit tests for each CRUD operation to ensure they work correctly in isolation. For example, using Jest for a Node.js application:
const User = require('./models/user');
test('Create user', async () => {
const userData = { name: 'John Doe', email: 'john@example.com' };
const user = await User.create(userData);
expect(user.name).toBe(userData.name);
expect(user.email).toBe(userData.email);
});
test('Read user', async () => {
const user = await User.findById(userId);
expect(user).not.toBeNull();
expect(user.name).toBe('John Doe');
});
test('Update user', async () => {
const updatedData = { name: 'Jane Doe' };
const user = await User.findByIdAndUpdate(userId, updatedData, { new: true });
expect(user.name).toBe(updatedData.name);
});
test('Delete user', async () => {
await User.findByIdAndDelete(userId);
const user = await User.findById(userId);
expect(user).toBeNull();
});
Integration Testing
Integration tests ensure that your CRUD operations work correctly with your database and other system components. These tests often involve setting up a test database and running operations against it.
API Testing
If you’re building a RESTful API, you should test your CRUD endpoints to ensure they respond correctly to different types of requests. Tools like Postman or Supertest can be useful for this.
Conclusion
CRUD operations form the backbone of data manipulation in software development. Whether you’re building a simple web application or preparing for technical interviews at major tech companies, a solid understanding of CRUD is essential.
By mastering CRUD operations, you’ll be well-equipped to design and implement efficient, secure, and scalable data management systems. Remember to always consider best practices, security implications, and the specific needs of your project when implementing CRUD functionality.
As you continue your journey in software development, you’ll find that CRUD concepts appear in various forms across different technologies and frameworks. The fundamental principles remain the same, making CRUD a valuable skill that will serve you well throughout your career.
Keep practicing, experimenting with different databases and programming languages, and don’t hesitate to explore more advanced patterns as you grow in your development skills. With a strong foundation in CRUD, you’ll be well-prepared to tackle complex data management challenges and excel in your coding interviews and projects.