What Is A Spring Bean? A Comprehensive Guide for Java Developers
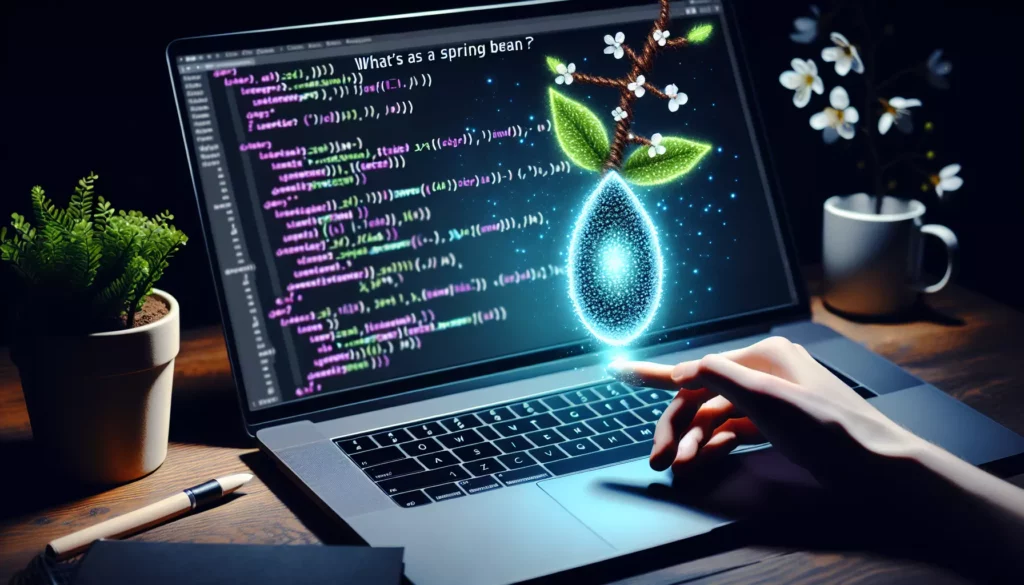
As you delve deeper into the world of Java development, particularly when working with the Spring Framework, you’ll inevitably encounter the term “Spring Bean.” Understanding Spring Beans is crucial for mastering Spring-based applications and leveraging the full power of this popular framework. In this comprehensive guide, we’ll explore what Spring Beans are, how they work, and why they’re essential in modern Java development.
Table of Contents
- Definition of a Spring Bean
- IoC and Dependency Injection
- Spring Bean Lifecycle
- Bean Scopes in Spring
- Creating and Configuring Spring Beans
- Bean Wiring and Dependency Injection
- Best Practices for Using Spring Beans
- Common Issues and Troubleshooting
- Advanced Concepts in Spring Beans
- Conclusion
1. Definition of a Spring Bean
At its core, a Spring Bean is simply a Java object that is managed by the Spring IoC (Inversion of Control) container. These objects form the backbone of your application and are created, configured, and managed by the Spring Framework.
Key characteristics of Spring Beans include:
- They are instantiated, assembled, and managed by the Spring IoC container.
- They can be configured through metadata (XML, Java annotations, or Java code).
- They often represent the services, repositories, or other components of your application.
- They can have dependencies on other beans, which are automatically injected by the container.
Here’s a simple example of a Spring Bean:
@Component
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User getUserById(Long id) {
return userRepository.findById(id);
}
}
In this example, UserService
is a Spring Bean. It’s annotated with @Component
, which tells Spring to manage this class as a bean. The @Autowired
annotation indicates that Spring should inject a UserRepository
bean into this service.
2. IoC and Dependency Injection
To truly understand Spring Beans, it’s essential to grasp the concepts of Inversion of Control (IoC) and Dependency Injection (DI), which are fundamental to the Spring Framework.
Inversion of Control (IoC)
IoC is a design principle where the control over the flow of a program is inverted: instead of the programmer controlling the flow, the framework takes control. In the context of Spring, this means that the framework manages the lifecycle and configuration of application objects.
The IoC container in Spring is responsible for:
- Instantiating the application objects
- Configuring the objects
- Assembling the dependencies between objects
- Managing the entire lifecycle of the objects
Dependency Injection (DI)
DI is a specific form of IoC where the dependencies of a class are “injected” into it from the outside rather than created by the class itself. This promotes loose coupling and makes the code more testable and modular.
Spring supports several types of dependency injection:
- Constructor Injection
- Setter Injection
- Field Injection
Here’s an example of constructor injection:
@Service
public class OrderService {
private final PaymentService paymentService;
private final InventoryService inventoryService;
@Autowired
public OrderService(PaymentService paymentService, InventoryService inventoryService) {
this.paymentService = paymentService;
this.inventoryService = inventoryService;
}
// Service methods...
}
In this example, OrderService
depends on PaymentService
and InventoryService
. These dependencies are injected through the constructor, which is annotated with @Autowired
.
3. Spring Bean Lifecycle
Understanding the lifecycle of a Spring Bean is crucial for effective development and troubleshooting. The lifecycle of a bean can be broken down into several stages:
- Instantiation: The bean is created.
- Populating Properties: Dependencies are injected.
- BeanNameAware: If the bean implements BeanNameAware, its setBeanName method is called.
- BeanFactoryAware: If the bean implements BeanFactoryAware, its setBeanFactory method is called.
- Pre-Initialization: Custom init methods are called.
- InitializingBean: If the bean implements InitializingBean, its afterPropertiesSet method is called.
- Post-Initialization: Post-initialization callbacks are called.
- Bean is ready for use
- Destruction: When the container is shut down, the bean’s destroy method is called.
You can hook into various stages of the bean lifecycle using annotations or by implementing specific interfaces. Here’s an example:
@Component
public class MyBean implements InitializingBean, DisposableBean {
@PostConstruct
public void postConstruct() {
System.out.println("Bean is going through post-construction.");
}
@Override
public void afterPropertiesSet() throws Exception {
System.out.println("InitializingBean's afterPropertiesSet() method called.");
}
@PreDestroy
public void preDestroy() {
System.out.println("Bean is being destroyed.");
}
@Override
public void destroy() throws Exception {
System.out.println("DisposableBean's destroy() method called.");
}
}
4. Bean Scopes in Spring
Spring provides several bean scopes that define the lifecycle and visibility of a bean. Understanding these scopes is crucial for managing application state and resource usage effectively.
Singleton Scope
This is the default scope. Only one instance of the bean is created for the entire application context.
@Component
@Scope("singleton")
public class SingletonBean {
// Bean implementation
}
Prototype Scope
A new instance is created each time the bean is requested.
@Component
@Scope("prototype")
public class PrototypeBean {
// Bean implementation
}
Request Scope
A new instance is created for each HTTP request (only valid in web-aware Spring ApplicationContext).
@Component
@Scope(value = WebApplicationContext.SCOPE_REQUEST, proxyMode = ScopedProxyMode.TARGET_CLASS)
public class RequestScopedBean {
// Bean implementation
}
Session Scope
A new instance is created for each HTTP session (only valid in web-aware Spring ApplicationContext).
@Component
@Scope(value = WebApplicationContext.SCOPE_SESSION, proxyMode = ScopedProxyMode.TARGET_CLASS)
public class SessionScopedBean {
// Bean implementation
}
Application Scope
One instance per ServletContext (only valid in web-aware Spring ApplicationContext).
@Component
@Scope(value = WebApplicationContext.SCOPE_APPLICATION, proxyMode = ScopedProxyMode.TARGET_CLASS)
public class ApplicationScopedBean {
// Bean implementation
}
5. Creating and Configuring Spring Beans
There are several ways to create and configure Spring Beans. Let’s explore the most common methods:
XML Configuration
This is the traditional way of configuring beans in Spring. You define beans in an XML file:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="userService" class="com.example.UserService">
<constructor-arg ref="userRepository"/>
</bean>
<bean id="userRepository" class="com.example.UserRepository"/>
</beans>
Java-based Configuration
This approach uses Java classes to define beans:
@Configuration
public class AppConfig {
@Bean
public UserService userService(UserRepository userRepository) {
return new UserService(userRepository);
}
@Bean
public UserRepository userRepository() {
return new UserRepository();
}
}
Annotation-based Configuration
This is the most common approach in modern Spring applications. You use annotations like @Component
, @Service
, @Repository
, etc., to define beans:
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
// Service methods...
}
@Repository
public class UserRepository {
// Repository methods...
}
6. Bean Wiring and Dependency Injection
Bean wiring refers to the process of connecting beans together within the Spring container. This is typically done through dependency injection. Let’s look at different ways to wire beans:
Constructor Injection
This is the recommended approach for required dependencies:
@Service
public class OrderService {
private final PaymentService paymentService;
@Autowired
public OrderService(PaymentService paymentService) {
this.paymentService = paymentService;
}
// Service methods...
}
Setter Injection
This can be used for optional dependencies:
@Service
public class UserService {
private EmailService emailService;
@Autowired
public void setEmailService(EmailService emailService) {
this.emailService = emailService;
}
// Service methods...
}
Field Injection
While convenient, this method is generally discouraged as it makes the code harder to test:
@Service
public class ProductService {
@Autowired
private ProductRepository productRepository;
// Service methods...
}
7. Best Practices for Using Spring Beans
To make the most of Spring Beans and avoid common pitfalls, consider these best practices:
- Favor constructor injection: It ensures that required dependencies are available when the bean is created.
- Keep beans stateless when possible: This improves thread safety and scalability.
- Use appropriate scopes: Choose the right scope based on your bean’s lifecycle requirements.
- Avoid circular dependencies: They can lead to complex initialization issues.
- Use interfaces for dependencies: This promotes loose coupling and easier testing.
- Leverage Spring’s stereotype annotations: Use
@Service
,@Repository
, etc., to clarify the role of your beans. - Keep configuration simple: Use Java-based or annotation-based configuration for better type safety and refactoring support.
- Properly manage bean lifecycle: Implement lifecycle methods when necessary, but don’t overuse them.
8. Common Issues and Troubleshooting
Even experienced developers can run into issues with Spring Beans. Here are some common problems and their solutions:
No qualifying bean of type found
This often occurs when Spring can’t find a bean of the required type. Ensure that:
- The bean is properly annotated (e.g.,
@Component
,@Service
). - Component scanning is configured correctly.
- The package containing the bean is included in the component scan.
Circular dependency detected
This happens when beans depend on each other in a circular manner. To resolve:
- Redesign your beans to break the circular dependency.
- Use setter injection instead of constructor injection for one of the dependencies.
- Use
@Lazy
annotation to defer one of the bean creations.
Multiple beans of the same type
When multiple beans of the same type exist, Spring doesn’t know which one to inject. Solutions include:
- Use
@Qualifier
annotation to specify which bean to use. - Use
@Primary
annotation to designate a primary bean when multiple options exist.
Bean not in the expected scope
This can lead to unexpected behavior. Ensure that:
- You’re using the correct scope annotation (
@Scope
). - You understand the implications of each scope, especially in web applications.
9. Advanced Concepts in Spring Beans
As you become more proficient with Spring Beans, you’ll encounter more advanced concepts:
Method Injection
Useful when a singleton bean needs to use a non-singleton bean:
@Component
public abstract class CommandManager {
public Object process(Object commandState) {
Command command = createCommand();
command.setState(commandState);
return command.execute();
}
@Lookup
protected abstract Command createCommand();
}
Factory Beans
When you need complex logic to instantiate a bean:
@Component
public class ComplexObjectFactory implements FactoryBean<ComplexObject> {
@Override
public ComplexObject getObject() throws Exception {
// Complex instantiation logic
return new ComplexObject();
}
@Override
public Class<?> getObjectType() {
return ComplexObject.class;
}
@Override
public boolean isSingleton() {
return true;
}
}
Bean Post-Processors
For customizing bean instantiation and configuration:
@Component
public class CustomBeanPostProcessor implements BeanPostProcessor {
@Override
public Object postProcessBeforeInitialization(Object bean, String beanName) throws BeansException {
if (bean instanceof AwareBean) {
((AwareBean) bean).setBeanName(beanName);
}
return bean;
}
@Override
public Object postProcessAfterInitialization(Object bean, String beanName) throws BeansException {
// Post-initialization logic
return bean;
}
}
10. Conclusion
Spring Beans are a fundamental concept in the Spring Framework, providing a powerful way to manage and configure application components. By understanding how to create, configure, and use Spring Beans effectively, you can build more maintainable, testable, and scalable Java applications.
Remember that mastering Spring Beans is an ongoing process. As you work on more complex projects, you’ll encounter new challenges and opportunities to apply these concepts in innovative ways. Keep experimenting, stay curious, and don’t hesitate to dive into the Spring documentation for more advanced topics.
Whether you’re building a simple web application or a complex enterprise system, a solid understanding of Spring Beans will serve you well in your journey as a Java developer. Happy coding!