Comprehensive Guide to Testing Types: Ensuring Software Quality and Reliability
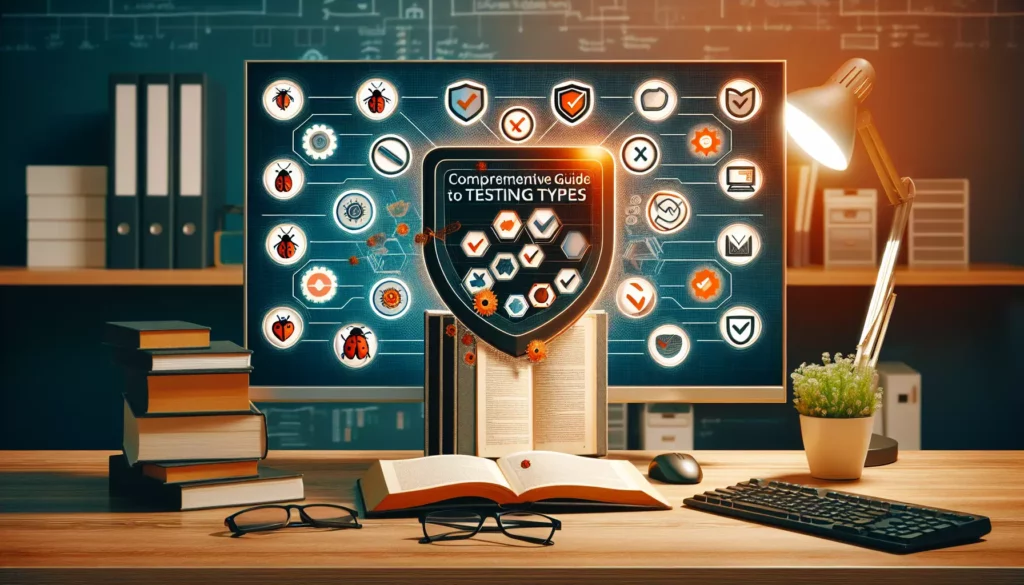
In the world of software development, testing plays a crucial role in ensuring the quality, reliability, and functionality of applications. As coding education platforms like AlgoCademy emphasize the importance of practical skills and problem-solving, understanding various testing types becomes essential for aspiring developers. This comprehensive guide will explore different testing types, their significance, and how they contribute to creating robust software solutions.
1. Unit Testing
Unit testing is the foundation of software testing and a critical skill for developers to master. It involves testing individual components or functions of a program in isolation to ensure they work correctly.
Key Features of Unit Testing:
- Tests small, isolated pieces of code
- Typically written and run by developers
- Helps identify bugs early in the development process
- Improves code quality and maintainability
Example of a Unit Test in Python:
import unittest
def add_numbers(a, b):
return a + b
class TestAddNumbers(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add_numbers(2, 3), 5)
def test_add_negative_numbers(self):
self.assertEqual(add_numbers(-1, -1), -2)
def test_add_mixed_numbers(self):
self.assertEqual(add_numbers(-1, 1), 0)
if __name__ == '__main__':
unittest.main()
In this example, we’re testing a simple add_numbers
function with different scenarios to ensure it works correctly for various input types.
2. Integration Testing
Integration testing focuses on verifying that different components or modules of an application work together correctly. This type of testing is crucial for ensuring that the various parts of a system interact seamlessly.
Key Aspects of Integration Testing:
- Tests the interaction between different modules or components
- Identifies issues in component interfaces
- Verifies that integrated components meet specified requirements
- Often performed after unit testing and before system testing
Example of Integration Testing Scenario:
Consider a simple e-commerce application with separate modules for user authentication, product catalog, and shopping cart. An integration test might involve:
- Logging in a user (testing the authentication module)
- Browsing the product catalog (testing the catalog module)
- Adding items to the cart (testing the shopping cart module)
- Verifying that the cart correctly reflects the added items and user information
3. Functional Testing
Functional testing verifies that the software meets the specified functional requirements. It focuses on testing the application’s features and functionality from the user’s perspective.
Characteristics of Functional Testing:
- Tests the software against functional specifications
- Verifies that the application does what it’s supposed to do
- Typically includes both positive and negative test cases
- Can be manual or automated
Example of a Functional Test Case:
For a login feature:
- Test Case: Valid Login
- Input: Correct username and password
- Expected Output: User successfully logs in and is redirected to the dashboard
- Test Case: Invalid Login
- Input: Incorrect username or password
- Expected Output: Error message displayed, user remains on login page
4. Performance Testing
Performance testing evaluates how a system performs under various conditions, focusing on responsiveness, stability, and scalability. This type of testing is crucial for applications that need to handle high loads or process large amounts of data.
Types of Performance Testing:
- Load Testing: Evaluates system behavior under expected load
- Stress Testing: Assesses system performance under extreme conditions
- Scalability Testing: Determines the system’s ability to scale with increasing load
- Endurance Testing: Tests system performance over an extended period
Example of Performance Testing Scenario:
For an e-commerce website:
- Simulate 10,000 concurrent users browsing products
- Measure response times for product page loads
- Monitor server resource utilization (CPU, memory, network)
- Identify performance bottlenecks and optimize accordingly
5. Security Testing
Security testing aims to identify vulnerabilities in the software that could be exploited by malicious users. It’s a critical aspect of testing, especially for applications handling sensitive data or financial transactions.
Key Areas of Security Testing:
- Authentication and Authorization
- Data Encryption
- SQL Injection
- Cross-Site Scripting (XSS)
- Cross-Site Request Forgery (CSRF)
- Session Management
Example of Security Testing:
Testing for SQL Injection vulnerability:
// Vulnerable code
$username = $_POST['username'];
$query = "SELECT * FROM users WHERE username = '$username'";
// Secure code
$username = mysqli_real_escape_string($connection, $_POST['username']);
$query = "SELECT * FROM users WHERE username = ?";
$stmt = $connection->prepare($query);
$stmt->bind_param("s", $username);
$stmt->execute();
In this example, the secure code uses prepared statements to prevent SQL injection attacks.
6. Usability Testing
Usability testing focuses on evaluating how user-friendly and intuitive an application is. It involves observing real users as they interact with the software to identify any usability issues or areas for improvement.
Key Aspects of Usability Testing:
- User Interface (UI) Design
- Navigation and Information Architecture
- Content Clarity and Readability
- Accessibility for Users with Disabilities
- Overall User Experience (UX)
Example of Usability Testing Process:
- Define test objectives and user personas
- Create realistic tasks for users to complete
- Recruit representative test participants
- Conduct testing sessions, observing and recording user interactions
- Analyze results and identify usability issues
- Provide recommendations for improvements
7. Regression Testing
Regression testing ensures that new changes or updates to the software don’t negatively impact existing functionality. It involves re-running previously executed tests to verify that the system still works as expected after modifications.
Importance of Regression Testing:
- Identifies unintended side effects of code changes
- Ensures that bug fixes don’t introduce new issues
- Maintains the overall stability of the application
- Provides confidence in the software’s reliability
Example of Regression Testing Strategy:
- Identify critical functionality and high-risk areas
- Create a suite of regression tests covering these areas
- Automate regression tests where possible
- Run regression tests after each significant code change or update
- Analyze test results and address any failures promptly
8. Acceptance Testing
Acceptance testing is the final phase of testing before the software is released to end-users. It verifies that the system meets the business requirements and is ready for deployment.
Types of Acceptance Testing:
- User Acceptance Testing (UAT): Performed by end-users to validate the system
- Alpha Testing: Internal testing performed by the development team
- Beta Testing: External testing performed by a limited group of real users
- Contract Acceptance Testing: Verifies that the system meets contractual requirements
Example of User Acceptance Testing Scenario:
For a new feature in a project management tool:
- Provide a group of actual users access to the new feature
- Ask users to perform their regular tasks using the new feature
- Collect feedback on usability, functionality, and overall satisfaction
- Identify any issues or areas for improvement
- Make necessary adjustments based on user feedback
- Obtain final approval from stakeholders
9. Exploratory Testing
Exploratory testing is a more informal approach where testers actively explore the application, learning about its features and potential issues as they go. This method can uncover unexpected bugs or usability issues that might be missed by more structured testing approaches.
Key Characteristics of Exploratory Testing:
- Simultaneous learning, test design, and test execution
- Emphasizes tester’s creativity and intuition
- Adaptable to changing requirements or newly discovered information
- Useful for identifying edge cases and unexpected scenarios
Example of Exploratory Testing Session:
- Define a time-boxed session (e.g., 2 hours)
- Choose a specific area or feature to explore
- Start using the application, noting observations and potential issues
- Try different input combinations and user flows
- Document findings, including bugs, usability issues, and suggestions
- Summarize and report results to the development team
10. Compatibility Testing
Compatibility testing ensures that the software works correctly across different environments, including various operating systems, devices, browsers, and network conditions.
Areas of Compatibility Testing:
- Cross-browser compatibility
- Cross-platform compatibility (Windows, macOS, Linux, etc.)
- Mobile device compatibility (iOS, Android)
- Network compatibility (3G, 4G, Wi-Fi)
- Database compatibility
Example of Cross-Browser Compatibility Testing:
- Identify target browsers and versions (e.g., Chrome, Firefox, Safari, Edge)
- Create a test matrix covering key functionality
- Test the application on each browser, noting any differences in appearance or behavior
- Pay special attention to CSS rendering, JavaScript functionality, and responsive design
- Document and report any compatibility issues
- Work with developers to resolve cross-browser inconsistencies
Conclusion
Understanding and implementing various testing types is crucial for developing high-quality, reliable software. As emphasized in coding education platforms like AlgoCademy, a strong foundation in testing practices is essential for aspiring developers preparing for technical interviews and real-world programming challenges.
By incorporating a comprehensive testing strategy that includes unit testing, integration testing, functional testing, and other types discussed in this guide, developers can:
- Identify and fix bugs early in the development process
- Ensure software meets functional and non-functional requirements
- Improve overall code quality and maintainability
- Enhance user satisfaction and trust in the application
- Reduce the cost and effort of fixing issues in later stages of development or after release
As you continue to develop your coding skills and prepare for technical interviews, remember that proficiency in various testing types will not only make you a better developer but also a valuable asset to any software development team. Practice implementing these testing strategies in your projects, and you’ll be well-equipped to tackle the challenges of modern software development and excel in technical interviews with major tech companies.