Essential SQL Commands for Database Management: A Comprehensive Guide
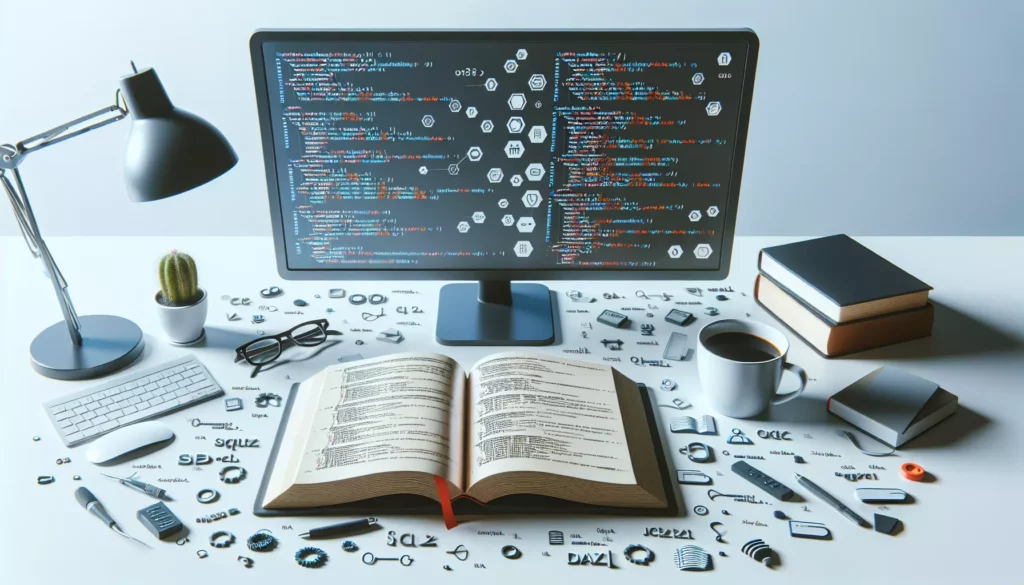
In today’s data-driven world, understanding SQL (Structured Query Language) is crucial for anyone working with databases or aspiring to enter the field of data management. Whether you’re a beginner just starting your journey in coding education or an experienced programmer looking to brush up on your database skills, mastering SQL commands is essential. This comprehensive guide will walk you through the most important SQL commands, their syntax, and practical examples to help you become proficient in managing and manipulating data.
Table of Contents
- Introduction to SQL
- SELECT: Retrieving Data
- INSERT: Adding New Records
- UPDATE: Modifying Existing Data
- DELETE: Removing Records
- CREATE: Building Database Structures
- ALTER: Modifying Database Structures
- DROP: Deleting Database Objects
- JOIN: Combining Data from Multiple Tables
- Aggregate Functions: Summarizing Data
- Subqueries: Nested SQL Statements
- Indexing: Optimizing Query Performance
- Transactions: Ensuring Data Integrity
- Views: Creating Virtual Tables
- Stored Procedures: Reusable SQL Code
- Conclusion
1. Introduction to SQL
SQL is a standardized language used for managing and manipulating relational databases. It allows you to create, retrieve, update, and delete data, as well as define and modify database structures. SQL is essential for various roles in the tech industry, including database administrators, data analysts, and backend developers.
Before we dive into specific SQL commands, it’s important to note that while SQL is standardized, different database management systems (DBMS) like MySQL, PostgreSQL, and Oracle may have slight variations in syntax or additional features. This guide will focus on standard SQL commands that work across most systems.
2. SELECT: Retrieving Data
The SELECT statement is one of the most fundamental and frequently used SQL commands. It allows you to retrieve data from one or more tables in a database.
Basic Syntax:
SELECT column1, column2, ...
FROM table_name
WHERE condition;
Examples:
1. Retrieve all columns from a table:
SELECT *
FROM employees;
2. Retrieve specific columns with a condition:
SELECT first_name, last_name, salary
FROM employees
WHERE department = 'Sales';
3. Use ORDER BY to sort results:
SELECT product_name, price
FROM products
ORDER BY price DESC;
4. Use LIMIT to restrict the number of results:
SELECT customer_id, total_purchase
FROM orders
ORDER BY total_purchase DESC
LIMIT 10;
3. INSERT: Adding New Records
The INSERT statement is used to add new records to a table in the database.
Basic Syntax:
INSERT INTO table_name (column1, column2, ...)
VALUES (value1, value2, ...);
Examples:
1. Insert a single record:
INSERT INTO employees (first_name, last_name, email, hire_date)
VALUES ('John', 'Doe', 'john.doe@example.com', '2023-05-01');
2. Insert multiple records:
INSERT INTO products (product_name, price, category)
VALUES
('Laptop', 999.99, 'Electronics'),
('Desk Chair', 199.99, 'Furniture'),
('Coffee Maker', 49.99, 'Appliances');
4. UPDATE: Modifying Existing Data
The UPDATE statement is used to modify existing records in a table.
Basic Syntax:
UPDATE table_name
SET column1 = value1, column2 = value2, ...
WHERE condition;
Examples:
1. Update a single column for all records:
UPDATE employees
SET salary = salary * 1.05;
2. Update multiple columns with a condition:
UPDATE customers
SET email = 'new.email@example.com', status = 'Active'
WHERE customer_id = 1001;
5. DELETE: Removing Records
The DELETE statement is used to remove records from a table.
Basic Syntax:
DELETE FROM table_name
WHERE condition;
Examples:
1. Delete a single record:
DELETE FROM orders
WHERE order_id = 10001;
2. Delete multiple records based on a condition:
DELETE FROM products
WHERE category = 'Discontinued' AND last_sale_date < '2022-01-01';
6. CREATE: Building Database Structures
The CREATE statement is used to create new database objects, such as tables, indexes, or views.
Basic Syntax for Creating a Table:
CREATE TABLE table_name (
column1 datatype constraints,
column2 datatype constraints,
...
);
Example:
CREATE TABLE employees (
employee_id INT PRIMARY KEY AUTO_INCREMENT,
first_name VARCHAR(50) NOT NULL,
last_name VARCHAR(50) NOT NULL,
email VARCHAR(100) UNIQUE,
hire_date DATE,
salary DECIMAL(10, 2)
);
7. ALTER: Modifying Database Structures
The ALTER statement is used to modify existing database objects, such as adding or removing columns from a table.
Basic Syntax:
ALTER TABLE table_name
ADD column_name datatype;
Examples:
1. Add a new column to an existing table:
ALTER TABLE employees
ADD phone_number VARCHAR(20);
2. Modify the data type of an existing column:
ALTER TABLE products
MODIFY COLUMN price DECIMAL(12, 2);
8. DROP: Deleting Database Objects
The DROP statement is used to remove database objects such as tables, indexes, or views.
Basic Syntax:
DROP TABLE table_name;
Example:
DROP TABLE old_employees;
Be cautious when using the DROP command, as it permanently deletes the specified object and all its data.
9. JOIN: Combining Data from Multiple Tables
JOIN clauses are used to combine rows from two or more tables based on a related column between them.
Types of JOINs:
- INNER JOIN
- LEFT JOIN (or LEFT OUTER JOIN)
- RIGHT JOIN (or RIGHT OUTER JOIN)
- FULL JOIN (or FULL OUTER JOIN)
Example of INNER JOIN:
SELECT orders.order_id, customers.customer_name, orders.order_date
FROM orders
INNER JOIN customers ON orders.customer_id = customers.customer_id;
Example of LEFT JOIN:
SELECT employees.employee_id, employees.first_name, departments.department_name
FROM employees
LEFT JOIN departments ON employees.department_id = departments.department_id;
10. Aggregate Functions: Summarizing Data
Aggregate functions perform calculations on a set of values and return a single result.
Common Aggregate Functions:
- COUNT()
- SUM()
- AVG()
- MAX()
- MIN()
Examples:
1. Count the number of employees:
SELECT COUNT(*) AS total_employees
FROM employees;
2. Calculate the average salary by department:
SELECT department, AVG(salary) AS avg_salary
FROM employees
GROUP BY department;
11. Subqueries: Nested SQL Statements
Subqueries are queries nested within another query, allowing you to perform complex operations and comparisons.
Example:
SELECT employee_id, first_name, last_name
FROM employees
WHERE salary > (SELECT AVG(salary) FROM employees);
This query selects employees whose salary is above the average salary of all employees.
12. Indexing: Optimizing Query Performance
Indexes are used to speed up data retrieval operations on database tables.
Creating an Index:
CREATE INDEX idx_last_name
ON employees (last_name);
This creates an index on the last_name column of the employees table, which can improve the performance of queries that search or sort by last name.
13. Transactions: Ensuring Data Integrity
Transactions are used to ensure that a series of SQL statements are executed as a single unit of work, maintaining data integrity.
Basic Transaction Syntax:
BEGIN TRANSACTION;
-- SQL statements
COMMIT;
-- or
ROLLBACK;
Example:
BEGIN TRANSACTION;
UPDATE accounts SET balance = balance - 100 WHERE account_id = 1001;
UPDATE accounts SET balance = balance + 100 WHERE account_id = 1002;
COMMIT;
14. Views: Creating Virtual Tables
Views are virtual tables based on the result of a SELECT statement. They can simplify complex queries and provide an additional layer of security.
Creating a View:
CREATE VIEW employee_details AS
SELECT e.employee_id, e.first_name, e.last_name, d.department_name
FROM employees e
JOIN departments d ON e.department_id = d.department_id;
This creates a view that combines employee and department information, which can be queried like a regular table.
15. Stored Procedures: Reusable SQL Code
Stored procedures are precompiled SQL statements that can be saved and reused, improving performance and code organization.
Creating a Stored Procedure:
DELIMITER //
CREATE PROCEDURE get_employee_by_id(IN emp_id INT)
BEGIN
SELECT * FROM employees WHERE employee_id = emp_id;
END //
DELIMITER ;
This creates a stored procedure that retrieves employee information based on the provided employee ID.
Calling a Stored Procedure:
CALL get_employee_by_id(1001);
Conclusion
Mastering SQL commands is essential for anyone working with databases or pursuing a career in data management. This comprehensive guide has covered the most important SQL commands, from basic data retrieval and manipulation to more advanced concepts like transactions and stored procedures.
As you continue your journey in coding education and programming skills development, remember that practice is key to becoming proficient in SQL. Try experimenting with these commands on sample databases, and don’t hesitate to explore more advanced features specific to your chosen database management system.
By understanding and applying these SQL commands, you’ll be well-equipped to handle various database tasks, from simple queries to complex data analysis. This knowledge will prove invaluable as you progress in your career, whether you’re aiming for positions at major tech companies or working on personal projects.
Keep learning, practicing, and exploring the vast world of database management, and you’ll find yourself well-prepared for technical interviews and real-world database challenges. Happy coding!