Node Package Manager (NPM): The Essential Tool for JavaScript Developers
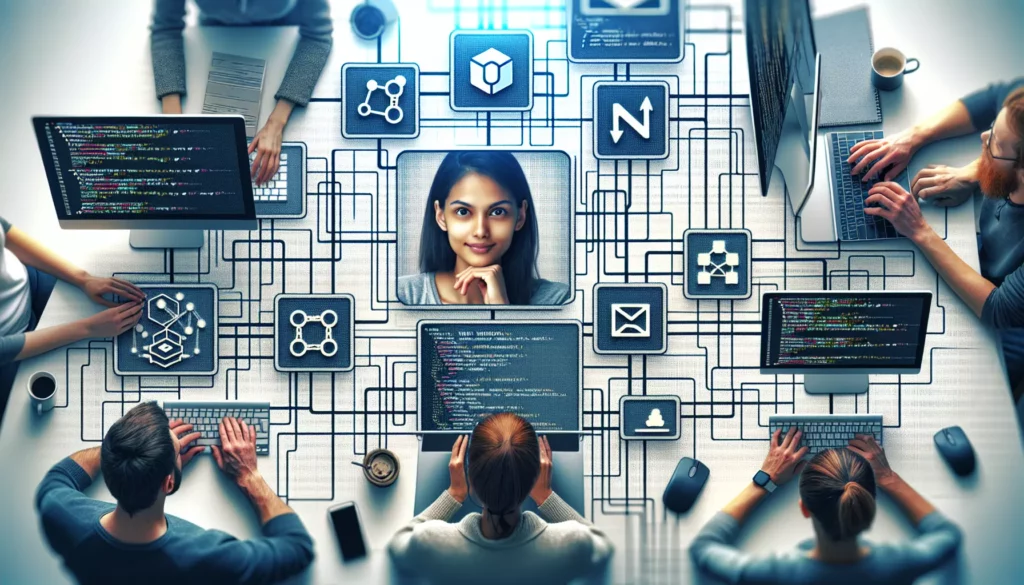
In the ever-evolving world of web development, JavaScript has emerged as one of the most popular and versatile programming languages. As projects grow in complexity, managing dependencies and packages becomes crucial for efficient development. This is where the Node Package Manager, commonly known as NPM, comes into play. In this comprehensive guide, we’ll explore NPM, its features, and how it can revolutionize your JavaScript development workflow.
What is Node Package Manager (NPM)?
Node Package Manager (NPM) is the default package manager for Node.js, a JavaScript runtime environment that executes JavaScript code outside of a web browser. NPM is an essential tool for JavaScript developers, providing a vast ecosystem of reusable code packages and a powerful command-line interface for managing project dependencies.
NPM was created in 2010 and has since become an integral part of the JavaScript development ecosystem. It allows developers to easily share, discover, and use code packages created by the global developer community, significantly speeding up the development process and promoting code reuse.
Key Features of NPM
NPM offers a wide range of features that make it an indispensable tool for JavaScript developers. Let’s explore some of its key functionalities:
1. Package Management
At its core, NPM is a package manager. It allows you to install, update, and remove packages (also called modules) in your project. These packages can be libraries, frameworks, or tools that extend the functionality of your application.
To install a package, you simply need to run the following command in your terminal:
npm install <package-name>
This command downloads the specified package and its dependencies, storing them in a node_modules
folder in your project directory.
2. Dependency Management
NPM excels at managing dependencies for your project. When you install a package, NPM automatically handles its dependencies, ensuring that all required packages are installed and compatible with each other.
The dependencies for your project are listed in a package.json
file, which serves as a manifest for your project. This file includes information about your project, such as its name, version, and dependencies.
3. Version Control
NPM uses semantic versioning (SemVer) to manage package versions. This system allows developers to specify which versions of a package their project is compatible with, helping to prevent breaking changes when updating dependencies.
In your package.json
file, you can specify version ranges for your dependencies using special syntax. For example:
"dependencies": {
"express": "^4.17.1",
"lodash": "~4.17.21"
}
In this example, the ^
symbol allows updates to any future minor or patch version, while ~
allows only patch updates.
4. Scripts
NPM provides a powerful scripting feature that allows you to define and run custom scripts for your project. These scripts can automate common tasks such as starting your application, running tests, or building your project for production.
Scripts are defined in the package.json
file under the scripts
section:
"scripts": {
"start": "node server.js",
"test": "mocha tests/*.js",
"build": "webpack --mode production"
}
You can run these scripts using the npm run
command, like this:
npm run start
5. NPM Registry
The NPM Registry is a vast, public database of JavaScript packages. It serves as a central repository where developers can publish their packages and discover packages created by others. As of 2023, the NPM Registry contains over 1.5 million packages, making it the largest software registry in the world.
Getting Started with NPM
Now that we understand the basics of NPM, let’s walk through the process of setting up and using NPM in a project.
Step 1: Installing Node.js and NPM
NPM comes bundled with Node.js, so you’ll need to install Node.js to use NPM. You can download and install Node.js from the official website: https://nodejs.org
After installation, you can verify that both Node.js and NPM are installed correctly by running these commands in your terminal:
node --version
npm --version
Step 2: Initializing a New Project
To start a new project with NPM, navigate to your project directory in the terminal and run:
npm init
This command will prompt you to answer a series of questions about your project. After answering these questions (or pressing Enter to accept the defaults), NPM will create a package.json
file in your project directory.
Step 3: Installing Packages
Now that your project is initialized, you can start installing packages. For example, to install the popular Express.js framework, you would run:
npm install express
This command will download Express and its dependencies, add them to your node_modules
folder, and update your package.json
file to include Express as a dependency.
Step 4: Using Installed Packages
After installing a package, you can use it in your JavaScript code by requiring it. For example, to use Express in your application:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Step 5: Running Scripts
To run a script defined in your package.json
file, use the npm run
command followed by the script name. For example, if you have a start script defined, you can run it with:
npm run start
Advanced NPM Features
As you become more comfortable with NPM, you’ll discover many advanced features that can further enhance your development workflow. Let’s explore some of these features:
1. Global Installation
Some packages, particularly command-line tools, are designed to be installed globally on your system. You can install a package globally using the -g
flag:
npm install -g <package-name>
Global packages are accessible from any directory on your system, making them ideal for tools you use across multiple projects.
2. Development Dependencies
NPM allows you to distinguish between dependencies required for production and those only needed during development. You can install a package as a development dependency using the --save-dev
flag:
npm install --save-dev <package-name>
These dependencies are listed under devDependencies
in your package.json
file and are not installed when someone installs your package as a dependency in their project.
3. NPM Scripts with Arguments
You can pass arguments to your NPM scripts, allowing for more flexible and powerful script definitions. For example:
"scripts": {
"start": "node server.js",
"start:dev": "NODE_ENV=development node server.js",
"test": "mocha tests/*.js",
"test:watch": "mocha tests/*.js --watch"
}
You can then run these scripts with different configurations:
npm run start:dev
npm run test:watch
4. NPM Audit
NPM includes a built-in security feature called npm audit. This command checks your project’s dependencies for known security vulnerabilities:
npm audit
If vulnerabilities are found, npm audit provides information about the affected packages and suggests ways to resolve the issues.
5. Package Versioning
NPM uses semantic versioning to manage package versions. Understanding this system can help you manage your dependencies more effectively:
- Major version: Incremented for incompatible API changes
- Minor version: Incremented for backwards-compatible feature additions
- Patch version: Incremented for backwards-compatible bug fixes
For example, version 2.1.3 indicates major version 2, minor version 1, and patch version 3.
6. NPM Cache
NPM maintains a local cache of downloaded packages to speed up future installations. You can manage this cache using various commands:
npm cache verify # Verify the contents of the cache
npm cache clean # Clear the entire cache
Best Practices for Using NPM
To make the most of NPM and ensure smooth development, consider following these best practices:
1. Use a .gitignore File
Always include a .gitignore
file in your project to prevent the node_modules
directory from being committed to your version control system. This directory can be quite large and should be regenerated on each machine using the package.json
file.
2. Lock Dependencies
Use a package-lock.json
file to lock your dependencies to specific versions. This file is automatically generated when you install packages and ensures that all team members are using the exact same package versions.
3. Regularly Update Dependencies
Keep your dependencies up to date to benefit from bug fixes and new features. You can check for outdated packages using:
npm outdated
And update them with:
npm update
4. Use Semantic Versioning Wisely
When specifying dependencies in your package.json
file, use semantic versioning ranges thoughtfully. For example:
^1.2.3
: Allow updates to any 1.x.x version~1.2.3
: Allow updates to any 1.2.x version1.2.3
: Use this exact version
5. Document Scripts
Include comments in your package.json
file to explain what each script does. This helps team members understand how to use the project:
"scripts": {
// Start the development server
"start": "node server.js",
// Run tests
"test": "mocha tests/*.js",
// Build for production
"build": "webpack --mode production"
}
NPM in the Context of Coding Education
As we consider NPM in the broader context of coding education and skill development, it’s clear that understanding and effectively using NPM is crucial for aspiring JavaScript developers. Here’s how NPM fits into the landscape of coding education:
1. Foundation for Modern Web Development
NPM is a fundamental tool in modern web development workflows. Learning to use NPM effectively is often one of the first steps in transitioning from writing simple scripts to developing full-fledged web applications. It’s an essential skill for anyone looking to build a career in web development, especially when preparing for technical interviews at major tech companies.
2. Introduction to Dependency Management
NPM introduces developers to the concept of dependency management, which is crucial in large-scale software development. Understanding how to manage project dependencies efficiently is a valuable skill that extends beyond JavaScript to other programming languages and ecosystems.
3. Exposure to Open Source Ecosystem
Through NPM, developers are exposed to the vast world of open-source software. This exposure can inspire learners to contribute to open-source projects, collaborate with other developers, and even publish their own packages. These activities are excellent for building a strong portfolio and gaining real-world development experience.
4. Automation and Productivity
NPM’s scripting capabilities introduce developers to task automation, an important concept in professional development environments. Learning to automate common tasks with NPM scripts can significantly boost productivity and is a valuable skill in any development role.
5. Best Practices and Standards
Working with NPM encourages developers to follow industry best practices, such as semantic versioning, proper project structuring, and security consciousness (through features like npm audit). These practices are essential for writing maintainable, scalable, and secure code – skills that are highly valued in technical interviews and professional settings.
6. Preparation for Advanced Concepts
Mastering NPM paves the way for understanding more advanced concepts in the JavaScript ecosystem, such as module bundlers (like Webpack), task runners (like Gulp), and modern frontend frameworks (like React, Vue, or Angular). These tools often rely on NPM for package management and build processes.
Conclusion
Node Package Manager (NPM) is more than just a tool – it’s a gateway to the vast and vibrant JavaScript ecosystem. By providing an efficient way to manage dependencies, automate tasks, and share code, NPM has become an indispensable part of modern JavaScript development.
For those embarking on a journey of coding education, particularly in web development, mastering NPM is a crucial step. It not only simplifies the development process but also introduces important concepts and best practices that are valuable across the software development industry.
As you continue to grow as a developer, remember that NPM is not just about installing packages. It’s about understanding the ecosystem, contributing to the community, and leveraging the collective knowledge of developers worldwide to create better, more efficient software.
Whether you’re preparing for technical interviews, building your next big project, or simply exploring the world of JavaScript, NPM will be there as a faithful companion on your coding journey. Embrace it, learn from it, and let it propel you towards becoming a more skilled and efficient developer.