List Comprehension in Python: A Comprehensive Guide
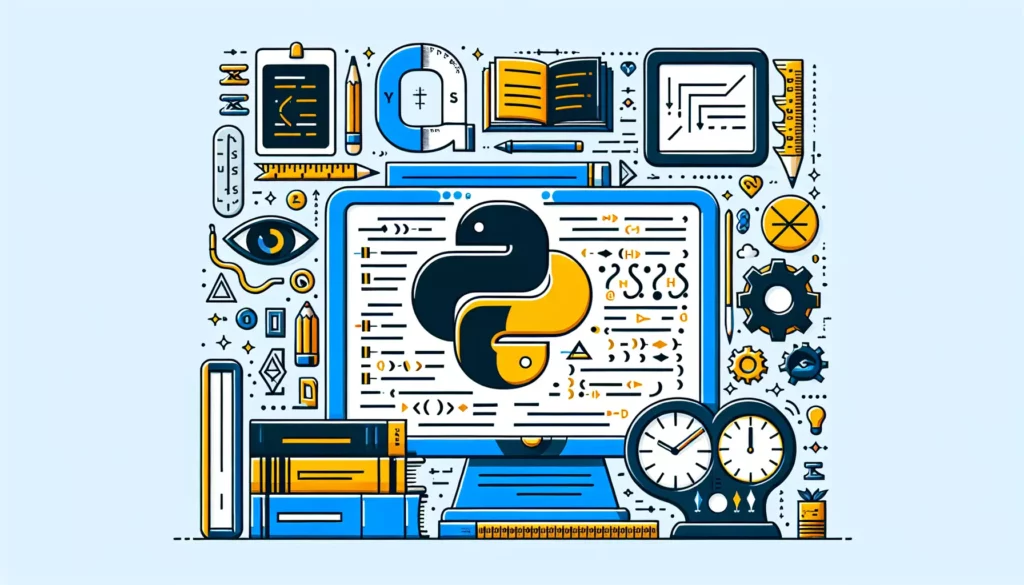
In the world of Python programming, list comprehension stands out as a powerful and elegant feature that can significantly enhance your code’s readability and efficiency. Whether you’re a beginner looking to level up your Python skills or an experienced developer preparing for technical interviews at top tech companies, mastering list comprehension is essential. In this comprehensive guide, we’ll dive deep into the concept of list comprehension, exploring its syntax, use cases, and advanced techniques.
What is List Comprehension?
List comprehension is a concise way to create lists in Python. It provides a more compact and often more readable alternative to traditional for loops and the map()
function. With list comprehension, you can create new lists based on existing lists or other iterable objects in a single line of code.
The basic syntax of list comprehension is as follows:
[expression for item in iterable if condition]
Let’s break down this syntax:
- expression: The operation to be performed on each item
- item: The variable representing each element in the iterable
- iterable: The sequence or collection to iterate over
- condition (optional): A filter to include only certain items
Basic List Comprehension Examples
Let’s start with some simple examples to illustrate how list comprehension works:
1. Creating a list of squares
squares = [x**2 for x in range(10)]
print(squares)
# Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
In this example, we create a list of squares for numbers 0 to 9. The expression x**2
is applied to each item x
in the range 0 to 9.
2. Filtering even numbers
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = [x for x in numbers if x % 2 == 0]
print(even_numbers)
# Output: [2, 4, 6, 8, 10]
Here, we filter the numbers
list to include only even numbers. The condition x % 2 == 0
ensures that only numbers divisible by 2 are included in the new list.
3. Converting strings to uppercase
words = ["hello", "world", "python", "programming"]
uppercase_words = [word.upper() for word in words]
print(uppercase_words)
# Output: ["HELLO", "WORLD", "PYTHON", "PROGRAMMING"]
This example demonstrates how to apply a string method (upper()
) to each item in the list using list comprehension.
Advantages of List Comprehension
List comprehension offers several benefits over traditional loop-based approaches:
- Conciseness: List comprehensions allow you to write more compact code, often reducing multiple lines to a single line.
- Readability: Once you’re familiar with the syntax, list comprehensions can be easier to read and understand at a glance.
- Performance: In many cases, list comprehensions are faster than equivalent for loops, especially for smaller lists.
- Expressiveness: They provide a more declarative way of describing the desired result, focusing on the “what” rather than the “how”.
Nested List Comprehension
List comprehensions can be nested, allowing you to create more complex structures or perform operations on multi-dimensional data. Here’s an example:
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flattened = [num for row in matrix for num in row]
print(flattened)
# Output: [1, 2, 3, 4, 5, 6, 7, 8, 9]
In this example, we flatten a 2D matrix into a 1D list using nested list comprehension. The outer loop iterates over each row, while the inner loop iterates over each number in the row.
List Comprehension with Conditional Statements
You can use conditional statements within list comprehensions to create more complex filtering or transformations. Let’s look at some examples:
1. Using if-else in list comprehension
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
result = ["Even" if x % 2 == 0 else "Odd" for x in numbers]
print(result)
# Output: ["Odd", "Even", "Odd", "Even", "Odd", "Even", "Odd", "Even", "Odd", "Even"]
This example uses an if-else statement within the list comprehension to classify numbers as even or odd.
2. Multiple conditions
numbers = range(1, 51)
result = [x for x in numbers if x % 3 == 0 and x % 5 == 0]
print(result)
# Output: [15, 30, 45]
Here, we use multiple conditions to filter numbers that are divisible by both 3 and 5.
List Comprehension vs. map() and filter()
List comprehensions can often replace the use of map()
and filter()
functions, providing a more Pythonic approach. Let’s compare these methods:
Using map()
numbers = [1, 2, 3, 4, 5]
squared_map = list(map(lambda x: x**2, numbers))
print(squared_map)
# Output: [1, 4, 9, 16, 25]
Equivalent list comprehension
squared_comp = [x**2 for x in numbers]
print(squared_comp)
# Output: [1, 4, 9, 16, 25]
Using filter()
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_filter = list(filter(lambda x: x % 2 == 0, numbers))
print(even_filter)
# Output: [2, 4, 6, 8, 10]
Equivalent list comprehension
even_comp = [x for x in numbers if x % 2 == 0]
print(even_comp)
# Output: [2, 4, 6, 8, 10]
As you can see, list comprehensions often provide a more readable and concise alternative to map()
and filter()
.
Advanced List Comprehension Techniques
Now that we’ve covered the basics, let’s explore some more advanced techniques and use cases for list comprehensions.
1. Working with dictionaries
List comprehensions can be used to create or manipulate dictionaries:
fruits = ["apple", "banana", "cherry"]
fruit_lengths = {fruit: len(fruit) for fruit in fruits}
print(fruit_lengths)
# Output: {"apple": 5, "banana": 6, "cherry": 6}
This example creates a dictionary where the keys are fruit names and the values are the lengths of those names.
2. Set comprehensions
Similar to list comprehensions, you can create set comprehensions:
numbers = [1, 2, 2, 3, 4, 4, 5]
unique_squares = {x**2 for x in numbers}
print(unique_squares)
# Output: {1, 4, 9, 16, 25}
This creates a set of unique squared values from the numbers list.
3. Generator expressions
Generator expressions are similar to list comprehensions but use parentheses instead of square brackets. They are memory-efficient for large datasets:
gen_exp = (x**2 for x in range(10))
print(gen_exp)
# Output: <generator object <genexpr> at 0x...>
for num in gen_exp:
print(num, end=" ")
# Output: 0 1 4 9 16 25 36 49 64 81
Generator expressions are lazy and generate values on-the-fly, making them useful for processing large amounts of data without storing everything in memory.
4. Handling exceptions in list comprehensions
While it’s generally not recommended to handle exceptions in list comprehensions, it can be done for simple cases:
data = ["1", "2", "3", "4", "five", "6"]
numbers = [int(x) if x.isdigit() else 0 for x in data]
print(numbers)
# Output: [1, 2, 3, 4, 0, 6]
This example converts strings to integers, using 0 as a default value for non-digit strings.
Best Practices and Considerations
While list comprehensions are powerful, it’s important to use them judiciously. Here are some best practices to keep in mind:
- Readability is key: If a list comprehension becomes too complex or hard to read, it’s often better to use a traditional for loop instead.
- Avoid side effects: List comprehensions should be used for creating new lists, not for causing side effects.
- Consider performance: For very large lists, a generator expression might be more memory-efficient than a list comprehension.
- Use meaningful variable names: Even in short list comprehensions, use descriptive variable names to enhance readability.
- Limit nesting: While nested list comprehensions are possible, they can quickly become difficult to read. Consider breaking complex operations into multiple steps.
List Comprehension in Technical Interviews
List comprehensions are a favorite topic in technical interviews, especially at top tech companies. They’re often used to assess a candidate’s Python proficiency and ability to write concise, efficient code. Here are some tips for using list comprehensions in interview settings:
- Start simple: If given a problem that involves creating or transforming a list, consider if a list comprehension could provide a clean solution.
- Explain your thought process: When using a list comprehension, briefly explain your approach to the interviewer. This demonstrates your understanding of the concept.
- Show alternatives: If you use a list comprehension, consider mentioning how you would solve the problem using a traditional for loop. This shows flexibility in your thinking.
- Practice common patterns: Familiarize yourself with common list comprehension patterns, such as filtering, mapping, and combining multiple lists.
- Consider readability: In an interview, it’s crucial to write clear, understandable code. If a list comprehension would be too complex, opt for a more straightforward approach.
Common Interview Questions Involving List Comprehensions
Here are some examples of interview questions that can be elegantly solved using list comprehensions:
1. Find all palindromic numbers in a range
palindromes = [num for num in range(1, 1001) if str(num) == str(num)[::-1]]
print(palindromes)
# Output: [1, 2, 3, 4, 5, 6, 7, 8, 9, 11, 22, 33, ..., 999]
2. Create a list of prime numbers
def is_prime(n):
return n > 1 and all(n % i != 0 for i in range(2, int(n**0.5) + 1))
primes = [num for num in range(2, 101) if is_prime(num)]
print(primes)
# Output: [2, 3, 5, 7, 11, 13, 17, 19, 23, 29, ..., 97]
3. Flatten a nested list
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flattened = [item for sublist in nested_list for item in sublist]
print(flattened)
# Output: [1, 2, 3, 4, 5, 6, 7, 8, 9]
4. Remove vowels from a string
text = "Hello, World!"
no_vowels = "".join([char for char in text if char.lower() not in "aeiou"])
print(no_vowels)
# Output: "Hll, Wrld!"
Conclusion
List comprehensions are a powerful feature of Python that can greatly enhance your code’s readability and efficiency. By mastering list comprehensions, you’ll be able to write more elegant and Pythonic code, which is particularly valuable in technical interviews and real-world programming scenarios.
Remember, while list comprehensions are incredibly useful, they shouldn’t be forced into every situation. The key is to use them when they enhance readability and maintainability. As you continue to practice and apply list comprehensions in your Python projects, you’ll develop a better intuition for when and how to use them effectively.
Whether you’re preparing for a technical interview at a top tech company or simply looking to improve your Python skills, a solid understanding of list comprehensions is invaluable. Keep practicing, experimenting with different use cases, and soon you’ll find yourself naturally reaching for this powerful tool in your Python programming toolkit.