JavaScript Versions: A Comprehensive Guide to the Evolution of ECMAScript
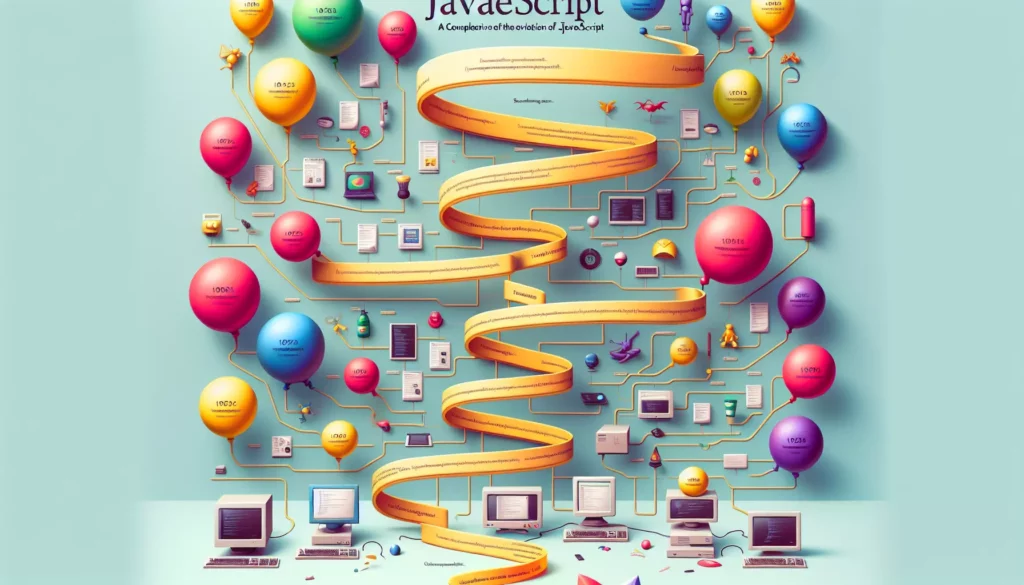
JavaScript, the ubiquitous language of the web, has come a long way since its inception in 1995. As the backbone of modern web development, JavaScript has undergone significant changes and improvements over the years. These changes are reflected in the various versions of ECMAScript, the standardized specification for JavaScript. In this comprehensive guide, we’ll explore the evolution of JavaScript through its different versions, highlighting key features and improvements that have shaped the language into what it is today.
Table of Contents
- Introduction to JavaScript and ECMAScript
- Early Versions: ES1 to ES3
- ES5: The Game Changer
- ES6/ES2015: The Revolution
- ES7/ES2016: Small but Significant
- ES8/ES2017: Async Awaits You
- ES9/ES2018: Spreading the Love
- ES10/ES2019: Flattening Arrays and Objects
- ES11/ES2020: The Nullish Coalescing Operator
- ES12/ES2021: Logical Assignment Operators
- ES13/ES2022: Top-level Await
- The Future of JavaScript
- Conclusion
1. Introduction to JavaScript and ECMAScript
Before diving into the various versions of JavaScript, it’s essential to understand the relationship between JavaScript and ECMAScript. JavaScript is the programming language, while ECMAScript is the specification that defines the standardized version of JavaScript. The European Computer Manufacturers Association (ECMA) is responsible for standardizing JavaScript, and each version of ECMAScript introduces new features and improvements to the language.
As we explore the different versions, keep in mind that browser support for newer features may vary. It’s always a good practice to check browser compatibility before using cutting-edge features in production code.
2. Early Versions: ES1 to ES3
The early versions of ECMAScript laid the foundation for the JavaScript we know today.
ES1 (1997)
This was the first standardized version of JavaScript. It included basic language syntax, data types, and core objects like Array, Date, and Math.
ES2 (1998)
This version made minor editorial changes to keep the specification fully aligned with ISO/IEC 16262 international standard.
ES3 (1999)
ES3 introduced several features that are still widely used today:
- Regular expressions
- Better string handling
- New control statements (do-while, switch)
- Try/catch exception handling
Example of a switch statement introduced in ES3:
let day = 3;
let dayName;
switch (day) {
case 1:
dayName = "Monday";
break;
case 2:
dayName = "Tuesday";
break;
case 3:
dayName = "Wednesday";
break;
default:
dayName = "Unknown";
}
console.log(dayName); // Output: Wednesday
3. ES5: The Game Changer
After a long gap, ES5 was released in 2009, bringing significant improvements to the language.
Key Features of ES5:
- Strict mode
- JSON support
- Array methods (forEach, map, filter, reduce, some, every)
- Object methods (Object.create, Object.keys, Object.freeze)
- Function.prototype.bind()
Example of using array methods introduced in ES5:
let numbers = [1, 2, 3, 4, 5];
// Using map to double each number
let doubled = numbers.map(num => num * 2);
console.log(doubled); // Output: [2, 4, 6, 8, 10]
// Using filter to get even numbers
let evens = numbers.filter(num => num % 2 === 0);
console.log(evens); // Output: [2, 4]
// Using reduce to sum all numbers
let sum = numbers.reduce((acc, curr) => acc + curr, 0);
console.log(sum); // Output: 15
4. ES6/ES2015: The Revolution
ES6, also known as ES2015, was a major update to the language, introducing a plethora of new features that significantly improved JavaScript’s capabilities and syntax.
Key Features of ES6:
- Let and const keywords
- Arrow functions
- Classes
- Template literals
- Destructuring assignment
- Spread operator
- Rest parameters
- Default parameters
- Promises for asynchronous programming
- Modules (import/export)
- Symbol data type
- Iterators and generators
- Enhanced object literals
- Map and Set data structures
Example showcasing some ES6 features:
// Arrow function
const greet = name => `Hello, ${name}!`;
// Destructuring and default parameters
const printPersonInfo = ({ name, age = 25 }) => {
console.log(`${name} is ${age} years old.`);
};
// Class definition
class Person {
constructor(name) {
this.name = name;
}
sayHello() {
console.log(`${this.name} says hello!`);
}
}
// Usage
console.log(greet("Alice")); // Output: Hello, Alice!
printPersonInfo({ name: "Bob" }); // Output: Bob is 25 years old.
const person = new Person("Charlie");
person.sayHello(); // Output: Charlie says hello!
5. ES7/ES2016: Small but Significant
ES7 was a smaller update compared to ES6, but it introduced two useful features:
- Exponentiation operator (**)
- Array.prototype.includes()
Example of ES7 features:
// Exponentiation operator
console.log(2 ** 3); // Output: 8
// Array.includes()
const fruits = ["apple", "banana", "orange"];
console.log(fruits.includes("banana")); // Output: true
console.log(fruits.includes("grape")); // Output: false
6. ES8/ES2017: Async Awaits You
ES8 brought significant improvements to asynchronous programming and object manipulation.
Key Features of ES8:
- Async/await
- Object.values() and Object.entries()
- String padding (padStart() and padEnd())
- Object.getOwnPropertyDescriptors()
- Trailing commas in function parameter lists
Example of async/await:
async function fetchUserData(userId) {
try {
const response = await fetch(`https://api.example.com/users/${userId}`);
const data = await response.json();
console.log(data);
} catch (error) {
console.error("Error fetching user data:", error);
}
}
fetchUserData(123);
7. ES9/ES2018: Spreading the Love
ES9 further enhanced JavaScript’s capabilities, especially in handling asynchronous operations and working with objects.
Key Features of ES9:
- Asynchronous iteration
- Rest/Spread properties for objects
- Promise.prototype.finally()
- Regular expression improvements
Example of rest/spread properties for objects:
const person = { name: "Alice", age: 30, city: "New York" };
const { name, ...rest } = person;
console.log(name); // Output: Alice
console.log(rest); // Output: { age: 30, city: "New York" }
const updatedPerson = { ...person, job: "Developer" };
console.log(updatedPerson);
// Output: { name: "Alice", age: 30, city: "New York", job: "Developer" }
8. ES10/ES2019: Flattening Arrays and Objects
ES10 introduced several useful methods for working with arrays and objects.
Key Features of ES10:
- Array.prototype.flat() and Array.prototype.flatMap()
- Object.fromEntries()
- String.prototype.trimStart() and String.prototype.trimEnd()
- Optional catch binding
- Function.prototype.toString() revision
Example of Array.prototype.flat() and Object.fromEntries():
// Array.prototype.flat()
const nestedArray = [1, [2, 3], [4, [5, 6]]];
console.log(nestedArray.flat(2)); // Output: [1, 2, 3, 4, 5, 6]
// Object.fromEntries()
const entries = [["name", "Alice"], ["age", 30]];
const obj = Object.fromEntries(entries);
console.log(obj); // Output: { name: "Alice", age: 30 }
9. ES11/ES2020: The Nullish Coalescing Operator
ES11 introduced several features that improved code readability and provided better ways to handle null and undefined values.
Key Features of ES11:
- Nullish coalescing operator (??)
- Optional chaining (?.)
- BigInt
- Promise.allSettled()
- globalThis
Example of nullish coalescing operator and optional chaining:
// Nullish coalescing operator
const foo = null ?? "default value";
console.log(foo); // Output: "default value"
const bar = 0 ?? 42;
console.log(bar); // Output: 0
// Optional chaining
const user = {
name: "Alice",
address: {
street: "123 Main St"
}
};
console.log(user?.address?.zipCode); // Output: undefined
console.log(user?.contact?.email); // Output: undefined
10. ES12/ES2021: Logical Assignment Operators
ES12 introduced new operators and methods to simplify common programming patterns.
Key Features of ES12:
- Logical assignment operators (??=, &&=, ||=)
- Numeric separators
- String.prototype.replaceAll()
- Promise.any()
- WeakRef and FinalizationRegistry objects
Example of logical assignment operators and numeric separators:
// Logical assignment operators
let a = null;
a ??= 10;
console.log(a); // Output: 10
let b = 5;
b &&= 20;
console.log(b); // Output: 20
let c = null;
c ||= 30;
console.log(c); // Output: 30
// Numeric separators
const largeNumber = 1_000_000_000;
console.log(largeNumber); // Output: 1000000000
11. ES13/ES2022: Top-level Await
ES13 brought several exciting features, including the ability to use await outside of async functions.
Key Features of ES13:
- Top-level await
- Class fields (public and private)
- Static class fields and methods
- Ergonomic brand checks for private fields
- Array.prototype.at()
- Object.hasOwn()
Example of top-level await and class fields:
// Top-level await (in modules)
const response = await fetch("https://api.example.com/data");
const data = await response.json();
console.log(data);
// Class fields and methods
class Counter {
#count = 0; // Private field
increment() {
this.#count++;
}
get value() {
return this.#count;
}
static description = "A simple counter class"; // Static public field
}
const counter = new Counter();
counter.increment();
console.log(counter.value); // Output: 1
console.log(Counter.description); // Output: "A simple counter class"
12. The Future of JavaScript
JavaScript continues to evolve, with new proposals being discussed and implemented regularly. Some exciting proposals for future versions include:
- Pattern matching
- Record and Tuple types
- Decorators
- Pipeline operator
- Temporal (a new date/time API)
These proposals aim to make JavaScript even more powerful and expressive, addressing common pain points and introducing new paradigms for developers.
13. Conclusion
The evolution of JavaScript through its various ECMAScript versions has transformed it from a simple scripting language into a powerful, versatile programming language capable of handling complex applications both in the browser and on the server.
As developers, it’s crucial to stay updated with the latest JavaScript features and best practices. Each new version brings improvements that can make our code more efficient, readable, and maintainable. However, it’s equally important to consider browser compatibility and use appropriate tools like transpilers (e.g., Babel) when working with cutting-edge features.
By understanding the history and evolution of JavaScript, we can better appreciate its capabilities and make informed decisions about which features to use in our projects. As JavaScript continues to grow and improve, it remains an essential skill for any web developer and a cornerstone of modern web development.
Remember, the journey of learning JavaScript is ongoing. As new versions and features are released, continue to explore, experiment, and integrate them into your projects. Happy coding!