Java Interfaces: A Comprehensive Guide for Aspiring Programmers
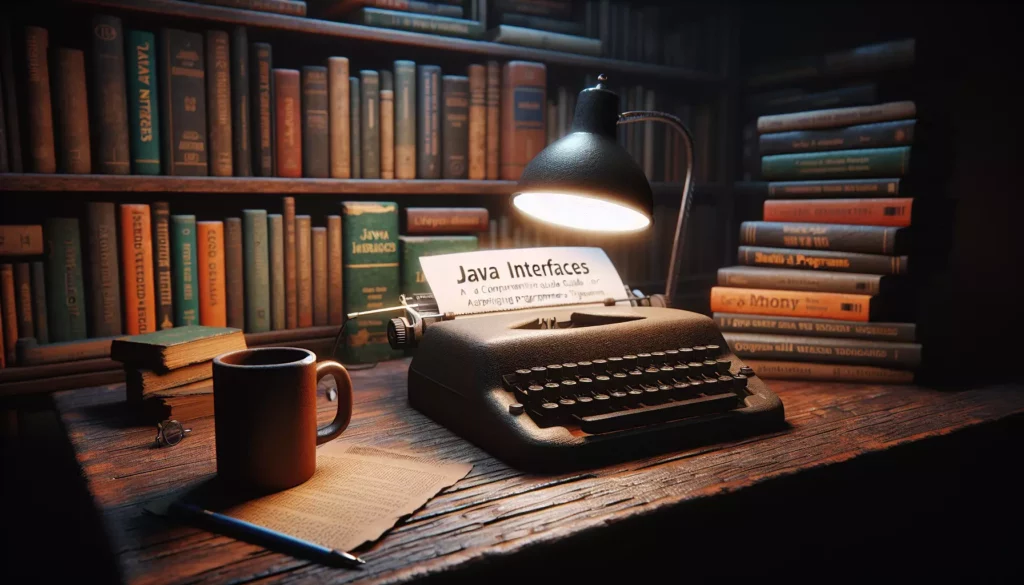
In the world of object-oriented programming, Java interfaces play a crucial role in defining contracts for classes and promoting loose coupling between different parts of a program. As an aspiring programmer or someone preparing for technical interviews at major tech companies, understanding Java interfaces is essential. This comprehensive guide will dive deep into Java interfaces, exploring their purpose, implementation, and best practices.
Table of Contents
- What are Java Interfaces?
- Why Use Interfaces?
- Declaring an Interface
- Implementing Interfaces
- Default Methods in Interfaces
- Static Methods in Interfaces
- Functional Interfaces
- Multiple Inheritance with Interfaces
- Marker Interfaces
- Best Practices for Using Interfaces
- Common Java Interfaces
- Common Interview Questions on Java Interfaces
- Conclusion
1. What are Java Interfaces?
In Java, an interface is a reference type that defines a contract for classes to follow. It’s similar to an abstract class but can only contain abstract methods (methods without a body) and constant fields. Interfaces provide a way to achieve abstraction and define common behavior that can be implemented by multiple classes.
Key characteristics of Java interfaces include:
- They can’t be instantiated directly
- All methods are implicitly public and abstract (prior to Java 8)
- All fields are implicitly public, static, and final
- A class can implement multiple interfaces
2. Why Use Interfaces?
Interfaces serve several important purposes in Java programming:
- Abstraction: Interfaces allow you to define a contract without specifying the implementation details, promoting abstraction in your code.
- Multiple Inheritance: Java doesn’t support multiple inheritance for classes, but a class can implement multiple interfaces, providing a form of multiple inheritance.
- Loose Coupling: Interfaces help create loosely coupled systems by allowing different parts of a program to communicate through well-defined contracts rather than specific implementations.
- Polymorphism: Interfaces enable polymorphic behavior, allowing objects of different classes to be treated uniformly if they implement the same interface.
- API Design: Interfaces are crucial for designing clean and extensible APIs, as they provide a clear separation between what a system does and how it does it.
3. Declaring an Interface
To declare an interface in Java, you use the interface
keyword. Here’s a basic syntax:
public interface InterfaceName {
// Constants (if any)
public static final int CONSTANT_VALUE = 100;
// Abstract methods
public void method1();
public int method2(String param);
}
Let’s break down the components of an interface declaration:
- The
public
keyword is optional. If omitted, the interface has package-level access. - Constants in interfaces are implicitly
public static final
, so you can omit these modifiers. - Methods are implicitly
public
andabstract
, so these modifiers can be omitted as well.
Here’s an example of a simple interface for a shape:
public interface Shape {
double calculateArea();
double calculatePerimeter();
}
4. Implementing Interfaces
To implement an interface, a class uses the implements
keyword followed by the interface name. The class must provide implementations for all the abstract methods defined in the interface.
Here’s an example of a class implementing the Shape
interface:
public class Circle implements Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double calculateArea() {
return Math.PI * radius * radius;
}
@Override
public double calculatePerimeter() {
return 2 * Math.PI * radius;
}
}
In this example, the Circle
class implements the Shape
interface and provides concrete implementations for the calculateArea()
and calculatePerimeter()
methods.
5. Default Methods in Interfaces
Starting from Java 8, interfaces can include default methods. These are methods with a default implementation that can be overridden by implementing classes if needed. Default methods allow you to add new functionality to interfaces without breaking existing implementations.
Here’s an example of an interface with a default method:
public interface Drawable {
void draw();
default void display() {
System.out.println("Displaying the drawable object");
draw();
}
}
In this example, the display()
method has a default implementation that calls the abstract draw()
method. Classes implementing this interface can use the default display()
method as-is or override it if needed.
6. Static Methods in Interfaces
Java 8 also introduced the ability to define static methods in interfaces. These methods belong to the interface itself and cannot be overridden by implementing classes. Static methods in interfaces are often used to provide utility functions related to the interface.
Here’s an example of an interface with a static method:
public interface MathOperations {
static int add(int a, int b) {
return a + b;
}
int subtract(int a, int b);
}
In this example, the add()
method is a static method that can be called directly on the interface, like this: MathOperations.add(5, 3)
.
7. Functional Interfaces
A functional interface is an interface that contains exactly one abstract method. These interfaces are fundamental to Java’s lambda expressions and functional programming features. The @FunctionalInterface
annotation is used to indicate that an interface is intended to be a functional interface.
Here’s an example of a functional interface:
@FunctionalInterface
public interface Comparator<T> {
int compare(T o1, T o2);
}
Functional interfaces can be implemented using lambda expressions, making the code more concise and readable. For example:
Comparator<String> stringLengthComparator = (s1, s2) -> s1.length() - s2.length();
8. Multiple Inheritance with Interfaces
One of the key advantages of interfaces is that they allow a form of multiple inheritance in Java. A class can implement multiple interfaces, inheriting behavior from all of them.
Here’s an example of a class implementing multiple interfaces:
public interface Flyable {
void fly();
}
public interface Swimmable {
void swim();
}
public class Duck implements Flyable, Swimmable {
@Override
public void fly() {
System.out.println("Duck is flying");
}
@Override
public void swim() {
System.out.println("Duck is swimming");
}
}
In this example, the Duck
class implements both the Flyable
and Swimmable
interfaces, inheriting behavior from both.
9. Marker Interfaces
A marker interface is an interface that doesn’t declare any methods or constants. It’s used to “mark” a class as having a certain property or capability. The Java API includes several marker interfaces, such as Serializable
, Cloneable
, and Remote
.
Here’s an example of a custom marker interface:
public interface Persistable {
// This is a marker interface
}
public class User implements Persistable {
private String username;
private String email;
// Constructor, getters, and setters
}
In this example, the Persistable
interface doesn’t declare any methods, but it can be used to indicate that a class (like User
) should be considered persistable, perhaps for use with a database or serialization system.
10. Best Practices for Using Interfaces
When working with interfaces in Java, consider the following best practices:
- Design for extension: Create interfaces that are easy to implement and extend in the future.
- Keep interfaces focused: Follow the Interface Segregation Principle (ISP) and create small, specific interfaces rather than large, general-purpose ones.
- Use interfaces for abstraction: Define interfaces to represent abstract concepts or behaviors, not specific implementations.
- Favor composition over inheritance: Use interfaces to create flexible, composable designs rather than relying on class inheritance.
- Document interface contracts: Clearly document the expected behavior of interface methods, including any exceptions that may be thrown.
- Use functional interfaces judiciously: When appropriate, use functional interfaces to enable lambda expressions and improve code readability.
- Avoid constant interfaces: Don’t use interfaces solely to define constants. Use classes or enums instead.
11. Common Java Interfaces
Java provides many built-in interfaces that are widely used in various applications. Understanding these common interfaces is crucial for effective Java programming. Here are some important interfaces you should be familiar with:
- Comparable<T>: Used for defining a natural ordering of objects.
- Comparator<T>: Used for defining custom ordering of objects.
- Iterable<T>: Allows objects to be used in for-each loops.
- Collection<E>: The root interface in the collection hierarchy.
- List<E>: An ordered collection (sequence) of elements.
- Set<E>: A collection that contains no duplicate elements.
- Map<K,V>: An object that maps keys to values.
- Runnable: Represents a task that can be executed by a thread.
- Callable<V>: Similar to Runnable, but can return a result and throw exceptions.
- Serializable: A marker interface for classes that can be serialized.
Let’s look at an example using the Comparable
interface:
public class Student implements Comparable<Student> {
private String name;
private int grade;
public Student(String name, int grade) {
this.name = name;
this.grade = grade;
}
@Override
public int compareTo(Student other) {
return Integer.compare(this.grade, other.grade);
}
// Getters, setters, and other methods
}
In this example, the Student
class implements the Comparable
interface to define a natural ordering based on the student’s grade.
12. Common Interview Questions on Java Interfaces
When preparing for technical interviews, especially for positions at major tech companies, it’s important to be well-versed in Java interfaces. Here are some common interview questions you might encounter:
- Q: What is the difference between an interface and an abstract class?
A: The main differences are:- An interface can only contain abstract methods (prior to Java 8) and constants, while an abstract class can have both abstract and concrete methods.
- A class can implement multiple interfaces but can extend only one abstract class.
- All methods in an interface are implicitly public and abstract, while an abstract class can have methods with different access modifiers.
- Interfaces are used to define a contract, while abstract classes are used to define a base implementation for subclasses.
- Q: Can an interface extend another interface?
A: Yes, an interface can extend one or more other interfaces using theextends
keyword. - Q: What are the new features introduced for interfaces in Java 8?
A: Java 8 introduced default methods and static methods in interfaces. - Q: How do you resolve the diamond problem in Java when a class implements two interfaces with the same default method?
A: The implementing class must override the conflicting method and explicitly choose which interface’s implementation to use or provide its own implementation. - Q: What is a functional interface, and why is it important in Java 8+?
A: A functional interface is an interface with exactly one abstract method. It’s important because it enables the use of lambda expressions and method references in Java 8 and later versions.
To illustrate the diamond problem and its resolution, consider this example:
public interface A {
default void foo() {
System.out.println("A's foo");
}
}
public interface B {
default void foo() {
System.out.println("B's foo");
}
}
public class C implements A, B {
@Override
public void foo() {
A.super.foo(); // Choose A's implementation
// Or B.super.foo(); // Choose B's implementation
// Or provide a completely new implementation
}
}
In this example, class C
implements both interfaces A
and B
, which have conflicting default methods. Class C
must override the foo()
method and explicitly choose which implementation to use or provide its own.
13. Conclusion
Java interfaces are a powerful feature of the language that enable abstraction, promote loose coupling, and support the creation of flexible and extensible code. As an aspiring programmer or someone preparing for technical interviews, having a solid understanding of interfaces is crucial.
We’ve covered the basics of declaring and implementing interfaces, explored advanced features like default and static methods, discussed functional interfaces, and looked at best practices and common interview questions. By mastering these concepts and practicing their application, you’ll be well-prepared to use interfaces effectively in your Java projects and tackle interface-related questions in technical interviews.
Remember that interfaces are not just a language feature but a design tool. They allow you to think about your code in terms of contracts and behaviors rather than specific implementations. This mindset can lead to more modular, maintainable, and scalable code – skills that are highly valued in the software development industry.
As you continue your journey in Java programming, keep exploring the use of interfaces in real-world applications and open-source projects. This practical exposure will deepen your understanding and help you develop the intuition for when and how to use interfaces effectively in your own code.