Understanding HTTP Requests: A Comprehensive Guide for Developers
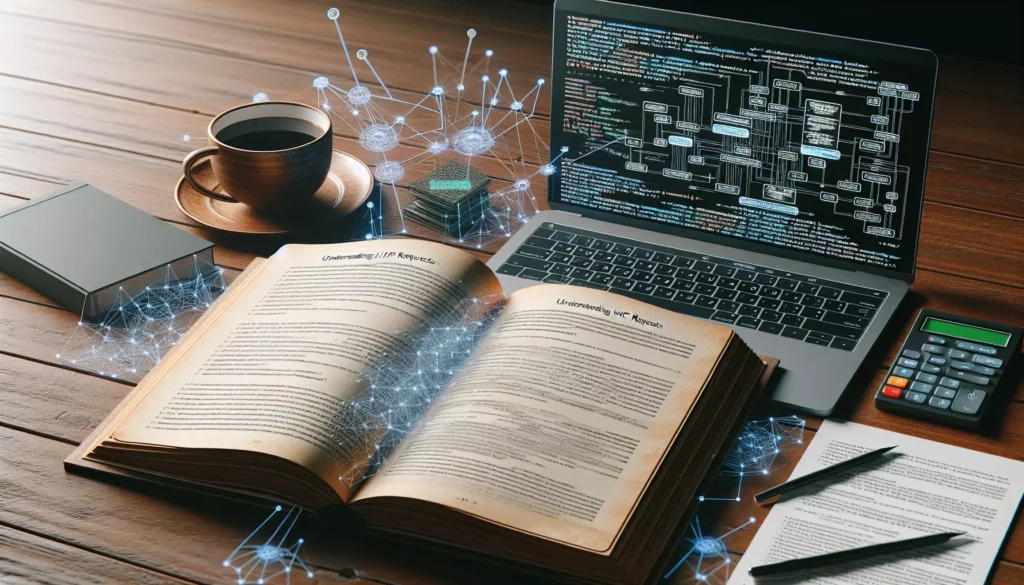
In the world of web development and programming, HTTP requests form the backbone of communication between clients and servers. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at major tech companies, a solid understanding of HTTP requests is crucial. This comprehensive guide will dive deep into the intricacies of HTTP requests, exploring their types, structure, and how they facilitate the exchange of information across the internet.
What are HTTP Requests?
HTTP (Hypertext Transfer Protocol) requests are messages sent by a client (usually a web browser) to a server, asking for specific actions to be performed. These requests are the foundation of the client-server model that powers the World Wide Web. When you type a URL into your browser or click a link, you’re essentially sending an HTTP request to a server.
The Anatomy of an HTTP Request
An HTTP request consists of several key components:
- Request Line: This includes the HTTP method, the URL, and the HTTP version.
- Headers: Additional information about the request or the client.
- Body: Optional data sent with the request (common in POST requests).
Let’s break down each of these components in more detail.
1. Request Line
The request line is the first line of an HTTP request and contains three crucial pieces of information:
- HTTP Method: Specifies the desired action to be performed on the resource (e.g., GET, POST, PUT, DELETE).
- URL: The address of the resource being requested.
- HTTP Version: The version of the HTTP protocol being used (e.g., HTTP/1.1 or HTTP/2).
Example of a request line:
GET /index.html HTTP/1.1
2. Headers
Headers provide additional information about the request or the client making the request. They are key-value pairs, with each pair on a new line. Some common headers include:
- Host: Specifies the domain name of the server.
- User-Agent: Identifies the client software making the request.
- Accept: Indicates what types of content the client can process.
- Content-Type: Specifies the media type of the request body (for POST or PUT requests).
- Authorization: Contains credentials for authenticating the client with the server.
Example of headers:
Host: www.example.com
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36
Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8
Accept-Language: en-US,en;q=0.5
Accept-Encoding: gzip, deflate, br
Connection: keep-alive
3. Body
The request body is optional and typically used with POST, PUT, or PATCH requests to send data to the server. This could be form data, JSON payloads, or other types of content. The body is separated from the headers by a blank line.
Example of a request body (for a POST request):
{
"username": "johndoe",
"email": "johndoe@example.com",
"password": "securepassword123"
}
Types of HTTP Methods
HTTP defines several methods (also called verbs) that indicate the desired action to be performed on the identified resource. The most commonly used methods are:
1. GET
The GET method requests a representation of the specified resource. GET requests should only retrieve data and should have no other effect on the data.
Example:
GET /api/users HTTP/1.1
Host: api.example.com
2. POST
The POST method submits data to be processed to the specified resource. It’s often used to create new resources or submit form data.
Example:
POST /api/users HTTP/1.1
Host: api.example.com
Content-Type: application/json
{
"name": "John Doe",
"email": "john@example.com"
}
3. PUT
The PUT method updates an existing resource or creates a new resource if it doesn’t exist at the specified URL.
Example:
PUT /api/users/123 HTTP/1.1
Host: api.example.com
Content-Type: application/json
{
"name": "John Smith",
"email": "john.smith@example.com"
}
4. DELETE
The DELETE method deletes the specified resource.
Example:
DELETE /api/users/123 HTTP/1.1
Host: api.example.com
5. PATCH
The PATCH method applies partial modifications to a resource.
Example:
PATCH /api/users/123 HTTP/1.1
Host: api.example.com
Content-Type: application/json
{
"email": "newemail@example.com"
}
HTTP Status Codes
When a server receives an HTTP request, it responds with an HTTP status code, indicating the outcome of the request. These status codes are grouped into five classes:
- 1xx (Informational): The request was received, continuing process.
- 2xx (Successful): The request was successfully received, understood, and accepted.
- 3xx (Redirection): Further action needs to be taken to complete the request.
- 4xx (Client Error): The request contains bad syntax or cannot be fulfilled.
- 5xx (Server Error): The server failed to fulfill a valid request.
Some common status codes include:
- 200 OK: The request was successful.
- 201 Created: The request was successful and a new resource was created.
- 204 No Content: The request was successful, but there’s no content to send back.
- 400 Bad Request: The server cannot process the request due to a client error.
- 401 Unauthorized: The request requires authentication.
- 403 Forbidden: The server understood the request but refuses to authorize it.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: A generic error message when the server encounters an unexpected condition.
Making HTTP Requests in Different Programming Languages
Now that we understand the structure and types of HTTP requests, let’s look at how to make these requests in different programming languages. This knowledge is crucial for developers working on web applications or preparing for technical interviews at major tech companies.
Python
In Python, the `requests` library is commonly used for making HTTP requests. Here’s an example of a GET request:
import requests
response = requests.get('https://api.example.com/users')
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f"Error: {response.status_code}")
And here’s an example of a POST request:
import requests
data = {
'name': 'John Doe',
'email': 'john@example.com'
}
response = requests.post('https://api.example.com/users', json=data)
if response.status_code == 201:
print("User created successfully")
else:
print(f"Error: {response.status_code}")
JavaScript
In JavaScript, you can use the Fetch API for making HTTP requests. Here’s an example of a GET request:
fetch('https://api.example.com/users')
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
return response.json();
})
.then(data => console.log(data))
.catch(error => console.log('There was a problem with the fetch operation: ' + error.message));
And here’s an example of a POST request:
const data = {
name: 'John Doe',
email: 'john@example.com'
};
fetch('https://api.example.com/users', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(data),
})
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
return response.json();
})
.then(data => console.log('User created successfully:', data))
.catch(error => console.log('There was a problem with the fetch operation: ' + error.message));
Java
In Java, you can use the `HttpClient` class (introduced in Java 11) for making HTTP requests. Here’s an example of a GET request:
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://api.example.com/users"))
.build();
client.sendAsync(request, HttpResponse.BodyHandlers.ofString())
.thenApply(HttpResponse::body)
.thenAccept(System.out::println)
.join();
And here’s an example of a POST request:
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
String json = "{\"name\":\"John Doe\",\"email\":\"john@example.com\"}";
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://api.example.com/users"))
.header("Content-Type", "application/json")
.POST(HttpRequest.BodyPublishers.ofString(json))
.build();
client.sendAsync(request, HttpResponse.BodyHandlers.ofString())
.thenApply(HttpResponse::body)
.thenAccept(System.out::println)
.join();
HTTP Request Headers in Detail
HTTP headers play a crucial role in providing additional information about the request or response. Let’s dive deeper into some important headers:
1. Content-Type
This header specifies the media type of the resource or the data sent in the request body. Common values include:
- application/json
- application/x-www-form-urlencoded
- multipart/form-data
- text/html
2. Authorization
This header is used to send credentials for authenticating the client with the server. It’s commonly used with Bearer tokens:
Authorization: Bearer eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9...
3. User-Agent
This header identifies the client software making the request. It often includes information about the browser and operating system:
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36
4. Accept
This header specifies which content types are acceptable for the response. It allows the client to negotiate the content type with the server:
Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8
5. Cookie
This header is used to send cookies from the client to the server. Cookies are often used for session management and user tracking:
Cookie: session_id=abc123; user_preference=dark_mode
Security Considerations in HTTP Requests
When working with HTTP requests, it’s crucial to consider security to protect sensitive data and prevent unauthorized access. Here are some key security considerations:
1. Use HTTPS
Always use HTTPS instead of HTTP to encrypt data in transit. This prevents eavesdropping and man-in-the-middle attacks.
2. Validate Input
Always validate and sanitize user input on both the client and server side to prevent injection attacks.
3. Use Authentication and Authorization
Implement proper authentication and authorization mechanisms to ensure that only authorized users can access sensitive resources.
4. Protect Against CSRF
Implement Cross-Site Request Forgery (CSRF) protection to prevent unauthorized commands from being transmitted from a user that the web application trusts.
5. Set Proper CORS Headers
Configure Cross-Origin Resource Sharing (CORS) headers correctly to control which domains can make requests to your server.
Debugging HTTP Requests
Debugging HTTP requests is an essential skill for any developer. Here are some tools and techniques to help you debug your requests:
1. Browser Developer Tools
Most modern browsers come with built-in developer tools that allow you to inspect network requests. You can see details about each request, including headers, body, and response.
2. Postman
Postman is a popular tool for testing API endpoints. It allows you to send requests with custom headers and bodies, and provides a user-friendly interface for viewing responses.
3. cURL
cURL is a command-line tool for transferring data using various protocols. It’s particularly useful for quickly testing HTTP requests from the terminal.
Example of a GET request using cURL:
curl https://api.example.com/users
Example of a POST request using cURL:
curl -X POST -H "Content-Type: application/json" -d '{"name":"John Doe","email":"john@example.com"}' https://api.example.com/users
4. Logging
Implement logging in your application to track the details of incoming requests and outgoing responses. This can be invaluable when debugging issues in production environments.
Conclusion
Understanding HTTP requests is fundamental for any developer working with web technologies. From the basic structure of requests to the intricacies of different HTTP methods and status codes, this knowledge forms the backbone of client-server communication on the web.
As you continue your journey in coding education and prepare for technical interviews at major tech companies, remember that proficiency in working with HTTP requests is not just about making calls to APIs. It’s about understanding the underlying principles, security considerations, and best practices that enable robust and secure web applications.
Whether you’re building a simple website or a complex distributed system, the concepts we’ve covered in this guide will serve as a solid foundation. Keep practicing, experimenting with different languages and tools, and always stay curious about the technologies that power the web.
Remember, mastering HTTP requests is just one piece of the puzzle in becoming a proficient developer. Continue to explore other areas of web development, algorithms, and software design to round out your skills and prepare for the challenges that lie ahead in your coding career.