Mastering HTML Inline Styles: A Comprehensive Guide
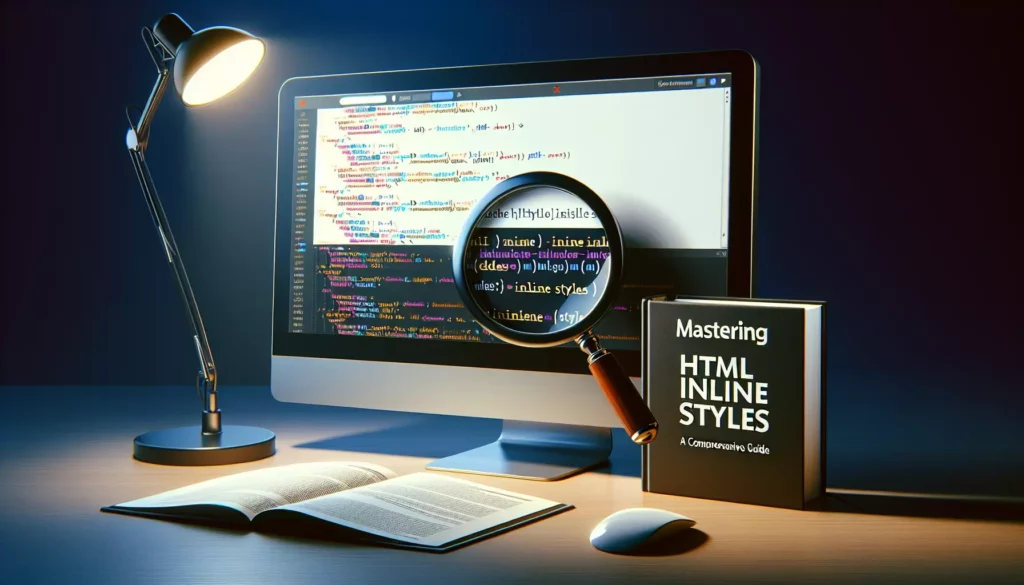
In the world of web development, HTML (Hypertext Markup Language) serves as the backbone of every webpage. While HTML provides the structure, CSS (Cascading Style Sheets) brings life to web pages through styling. One of the ways to apply styles directly to HTML elements is through inline styles. In this comprehensive guide, we’ll dive deep into HTML inline styles, exploring their uses, advantages, disadvantages, and best practices.
What Are HTML Inline Styles?
HTML inline styles are a way to apply CSS styling directly to individual HTML elements using the style
attribute. Instead of defining styles in a separate CSS file or within a <style>
tag in the HTML document’s head, inline styles are written directly within the HTML tags.
Here’s a basic example of an inline style:
<p style="color: blue; font-size: 16px;">This is a blue paragraph with 16px font size.</p>
In this example, the style
attribute is used to set the text color to blue and the font size to 16 pixels for this specific paragraph.
The Syntax of Inline Styles
The syntax for inline styles is straightforward:
- Use the
style
attribute within the opening tag of an HTML element. - Set the value of the
style
attribute to one or more CSS property-value pairs. - Separate multiple property-value pairs with semicolons.
Here’s an example with multiple style properties:
<div style="background-color: #f0f0f0; padding: 20px; border: 1px solid #ccc; border-radius: 5px;">
This is a styled div element.
</div>
When to Use Inline Styles
While it’s generally recommended to separate content (HTML) from presentation (CSS), there are situations where inline styles can be useful:
- Quick prototyping: When you need to quickly test or demonstrate a style change without modifying external stylesheets.
- Email templates: Many email clients have limited CSS support, making inline styles necessary for consistent rendering across different platforms.
- Dynamic styling: When styles need to be applied dynamically based on user interactions or data from a server.
- Overriding external styles: To apply specific styles to an element that should take precedence over styles defined in external stylesheets.
- Single-use styles: For unique styles that are only used once in a document and don’t warrant a separate CSS rule.
Advantages of Inline Styles
Inline styles offer several benefits in certain scenarios:
- Specificity: Inline styles have the highest specificity in the CSS cascade, meaning they will override other styles applied to the element.
- Immediacy: You can see the effect of the style immediately without switching between HTML and CSS files.
- Portability: The styling is contained within the HTML element, making it easy to copy and paste styled content between documents.
- Performance: In some cases, inline styles can offer a slight performance advantage as the browser doesn’t need to match selectors.
- Isolation: Inline styles don’t affect other elements, reducing the risk of unintended style conflicts.
Disadvantages of Inline Styles
Despite their advantages, inline styles have several drawbacks that make them less ideal for large-scale web development:
- Maintainability: As a project grows, managing styles scattered throughout the HTML becomes increasingly difficult.
- Code repetition: Inline styles can lead to duplicate code if the same styles need to be applied to multiple elements.
- Lack of separation of concerns: Mixing content and presentation goes against the principle of separating structure (HTML) from style (CSS).
- Reduced reusability: Styles applied inline cannot be easily reused across different elements or pages.
- Increased file size: For larger projects, inline styles can significantly increase the size of HTML documents, potentially impacting load times.
- Difficulty in making global changes: Updating styles across an entire site becomes a tedious task when using inline styles extensively.
Best Practices for Using Inline Styles
If you decide to use inline styles, follow these best practices to minimize potential issues:
- Use sparingly: Reserve inline styles for exceptional cases rather than as a primary styling method.
- Prioritize external stylesheets: Use external CSS files for the majority of your styling needs to maintain separation of concerns.
- Avoid redundancy: Don’t repeat the same inline styles across multiple elements. Instead, consider creating a CSS class.
- Keep it simple: Use inline styles for simple, one-off style adjustments rather than complex, multi-property declarations.
- Document your choices: If you use inline styles, comment on why you chose this method for future reference.
- Consider specificity: Be aware that inline styles will override other CSS rules, which may lead to unexpected results.
Inline Styles vs. Internal and External CSS
To better understand the role of inline styles, let’s compare them with other CSS implementation methods:
1. Inline Styles
<p style="color: red; font-weight: bold;">This is an inline styled paragraph.</p>
2. Internal CSS (within the <style> tag in the HTML document)
<style>
p {
color: red;
font-weight: bold;
}
</style>
<p>This paragraph is styled using internal CSS.</p>
3. External CSS (in a separate .css file)
In your HTML file:
<link rel="stylesheet" href="styles.css">
<p>This paragraph is styled using external CSS.</p>
In your styles.css file:
p {
color: red;
font-weight: bold;
}
While inline styles offer immediacy and high specificity, internal and external CSS provide better organization, reusability, and maintainability for larger projects.
Advanced Techniques with Inline Styles
Despite the general recommendation to avoid overusing inline styles, there are some advanced techniques where they can be particularly useful:
1. Dynamic Styling with JavaScript
Inline styles are often used when dynamically changing element styles with JavaScript:
<button id="colorButton">Change Color</button>
<div id="colorBox" style="width: 100px; height: 100px; background-color: red;"></div>
<script>
document.getElementById('colorButton').addEventListener('click', function() {
var box = document.getElementById('colorBox');
box.style.backgroundColor = 'blue';
});
</script>
In this example, clicking the button changes the background color of the div from red to blue using inline styles.
2. CSS-in-JS Libraries
Some modern JavaScript libraries and frameworks, like styled-components for React, use a technique that essentially generates inline styles. While not traditional inline styles, they share some similarities:
import styled from 'styled-components';
const Button = styled.button`
background-color: blue;
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
`;
function App() {
return <Button>Click me</Button>;
}
This approach combines the power of CSS with the flexibility of JavaScript, often resulting in styles that are applied inline.
3. Critical CSS
For performance optimization, some developers use a technique called “Critical CSS” where the most important styles are inlined in the <head>
of the document to improve initial render times:
<head>
<style>
/* Critical styles here */
body { font-family: Arial, sans-serif; }
.header { background-color: #f0f0f0; padding: 20px; }
</style>
<link rel="stylesheet" href="non-critical-styles.css" media="print" onload="this.media='all'">
</head>
While not exactly inline styles on individual elements, this technique uses a similar principle of embedding styles directly in the HTML for performance benefits.
Inline Styles and Responsive Design
One area where inline styles fall short is in responsive design. Media queries, which are crucial for creating responsive layouts, cannot be used with inline styles. For example:
/* This can't be done with inline styles */
@media (max-width: 600px) {
.container {
width: 100%;
}
}
If you need to create responsive designs, it’s best to use external stylesheets or internal styles within <style>
tags.
Tools for Managing Inline Styles
While it’s generally better to avoid extensive use of inline styles, there are tools available to help manage them if necessary:
- Purify CSS: This tool can help remove unused CSS, including inline styles.
- CSS Inliner tools: These are particularly useful for email template development, converting external CSS to inline styles for better email client compatibility.
- Style lint: This linter can be configured to warn about or enforce rules regarding inline styles.
Browser Developer Tools and Inline Styles
Browser developer tools are invaluable for working with and debugging inline styles:
- You can view and edit inline styles in real-time using the “Styles” pane.
- The “Computed” tab shows you the final applied styles, including those from inline declarations.
- You can temporarily add or modify inline styles for testing purposes.
// Example of viewing inline styles in developer tools
<div style="color: red; font-size: 20px;" id="example">Hello, World!</div>
// In the console:
var element = document.getElementById('example');
console.log(element.style.color); // Outputs: "red"
console.log(element.style.fontSize); // Outputs: "20px"
Security Considerations with Inline Styles
When using inline styles, especially with dynamic content, be aware of potential security risks:
- Cross-Site Scripting (XSS): If user input is directly inserted into inline styles without proper sanitization, it could lead to XSS vulnerabilities.
- Content Security Policy (CSP): Strict CSP settings may block inline styles. You might need to use the
style-src 'unsafe-inline'
directive if inline styles are necessary.
Always sanitize and validate any dynamic content used in inline styles to prevent security issues.
Conclusion
HTML inline styles offer a quick and powerful way to apply CSS directly to HTML elements. While they have their uses in specific scenarios like email templates, quick prototyping, or dynamic styling with JavaScript, they should be used judiciously in larger web projects.
For most web development work, it’s recommended to use external stylesheets or internal styles to maintain a clear separation between content and presentation, improve code maintainability, and leverage the full power of CSS, including media queries for responsive design.
Understanding inline styles and their place in the CSS ecosystem is crucial for any web developer. By knowing when and how to use them effectively, you can make informed decisions about styling strategies in your projects, balancing immediacy and specificity with maintainability and scalability.