Git Setup: A Comprehensive Guide for Beginners
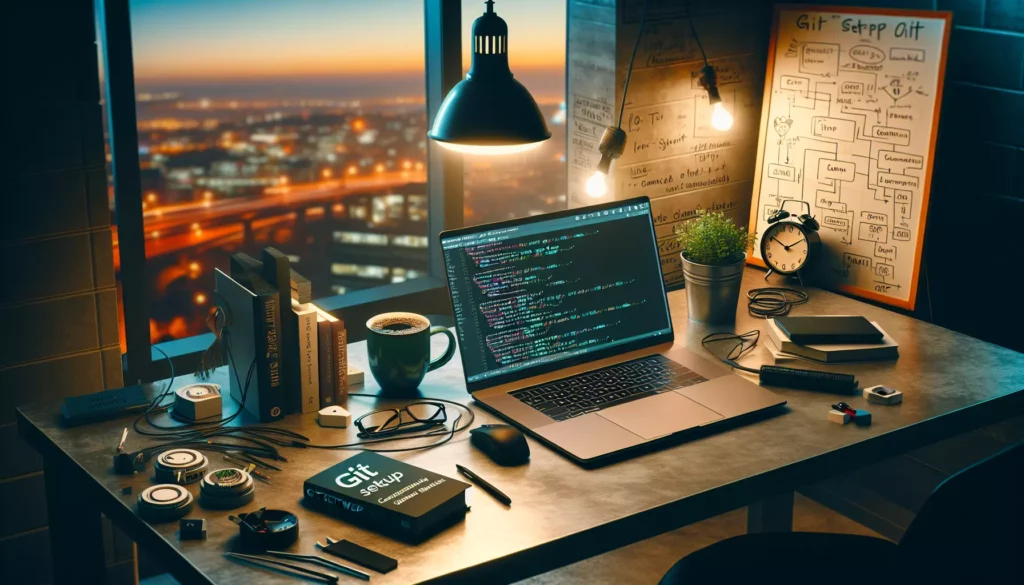
Welcome to AlgoCademy’s comprehensive guide on Git setup! Whether you’re a coding novice or looking to refresh your version control knowledge, this tutorial will walk you through the process of setting up Git on your system. Git is an essential tool for programmers, enabling efficient collaboration and version management of your code. Let’s dive in and get you ready to start using Git in your development workflow.
Table of Contents
- What is Git?
- Why Use Git?
- Installing Git
- Configuring Git
- Creating Your First Repository
- Basic Git Commands
- Git Workflow
- Git Best Practices
- Troubleshooting Common Issues
- Advanced Git Features
- Conclusion
What is Git?
Git is a distributed version control system that helps developers track changes in their code over time. It was created by Linus Torvalds in 2005 and has since become the industry standard for version control. Git allows multiple developers to work on the same project simultaneously, merge their changes, and maintain a complete history of all modifications.
Some key features of Git include:
- Distributed development
- Strong support for non-linear development (branching and merging)
- Efficient handling of large projects
- Cryptographic authentication of history
- Toolkit-based design
Why Use Git?
Git has become an indispensable tool in modern software development for several reasons:
- Version Control: Git allows you to track changes in your code over time, making it easy to revert to previous versions if needed.
- Collaboration: Multiple developers can work on the same project simultaneously without interfering with each other’s work.
- Branching and Merging: Git’s branching model allows for easy experimentation and feature development without affecting the main codebase.
- Backup: By pushing your code to remote repositories, you create backups of your work, ensuring you never lose progress.
- Open Source Contribution: Git is the backbone of many open-source projects, making it easier for developers to contribute to and improve software.
Now that we understand the importance of Git, let’s move on to setting it up on your system.
Installing Git
The installation process for Git varies depending on your operating system. Here’s how to install Git on the three major operating systems:
Windows
- Visit the official Git website: https://git-scm.com/download/win
- Download the latest version of Git for Windows.
- Run the installer and follow the installation wizard.
- During installation, you can leave the default options selected unless you have specific preferences.
- Once installed, you can access Git through the Git Bash terminal or through the regular Command Prompt.
macOS
- The easiest way to install Git on macOS is through the Xcode Command Line Tools. Open Terminal and run:
xcode-select --install
- Follow the prompts to install the Xcode Command Line Tools, which includes Git.
- Alternatively, you can download Git from the official website: https://git-scm.com/download/mac
- You can also use package managers like Homebrew to install Git:
brew install git
Linux
For most Linux distributions, Git can be installed using the default package manager:
For Ubuntu or Debian:
sudo apt-get update
sudo apt-get install git
For Fedora:
sudo dnf install git
For CentOS or RHEL:
sudo yum install git
After installation, verify that Git is installed correctly by opening a terminal or command prompt and typing:
git --version
This should display the installed version of Git.
Configuring Git
After installing Git, it’s important to configure it with your personal information. This helps identify your commits in the project history. Open a terminal or command prompt and run the following commands:
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
Replace “Your Name” with your actual name and “youremail@example.com” with your email address.
You can also set your preferred text editor for Git to use when creating commit messages:
git config --global core.editor "your-preferred-editor"
For example, to use Visual Studio Code as your Git editor:
git config --global core.editor "code --wait"
To view your Git configuration, use:
git config --list
Creating Your First Repository
Now that Git is installed and configured, let’s create your first Git repository:
- Create a new directory for your project:
mkdir my-first-repo cd my-first-repo
- Initialize the directory as a Git repository:
git init
- Create a new file:
echo "Hello, Git!" > README.md
- Stage the file for commit:
git add README.md
- Commit the changes:
git commit -m "Initial commit"
Congratulations! You’ve just created your first Git repository and made your first commit.
Basic Git Commands
Here are some essential Git commands you’ll use frequently:
git init
: Initialize a new Git repositorygit clone [url]
: Clone a repository from a remote sourcegit add [file]
: Add a file to the staging areagit commit -m "[message]"
: Commit staged changes with a messagegit status
: Check the status of your working directorygit log
: View commit historygit branch
: List, create, or delete branchesgit checkout [branch-name]
: Switch to a different branchgit merge [branch]
: Merge changes from one branch into the current branchgit pull
: Fetch and merge changes from a remote repositorygit push
: Push local changes to a remote repository
Git Workflow
A typical Git workflow involves the following steps:
- Create a new branch for a feature or bug fix:
git checkout -b feature-branch
- Make changes to your code
- Stage your changes:
git add .
- Commit your changes:
git commit -m "Implement new feature"
- Push your changes to the remote repository:
git push origin feature-branch
- Create a pull request to merge your changes into the main branch
- After review and approval, merge the pull request
- Delete the feature branch (optional):
git branch -d feature-branch
Git Best Practices
To make the most of Git and maintain a clean, efficient workflow, consider these best practices:
- Commit often: Make small, frequent commits that represent logical units of change.
- Write meaningful commit messages: Use clear, concise messages that describe what changes were made and why.
- Use branches: Create separate branches for different features or bug fixes to keep your main branch stable.
- Pull before you push: Always pull the latest changes from the remote repository before pushing your own changes to avoid conflicts.
- Review your changes: Use
git diff
to review your changes before committing. - Use .gitignore: Create a .gitignore file to exclude unnecessary files (like build artifacts or sensitive information) from version control.
- Keep your repository clean: Regularly delete merged branches and use
git clean
to remove untracked files. - Use meaningful branch names: Name your branches descriptively, e.g., “feature/user-authentication” or “bugfix/login-error”.
- Learn to use Git’s advanced features: Familiarize yourself with rebasing, cherry-picking, and other advanced Git operations.
Troubleshooting Common Issues
Even experienced developers encounter Git-related issues from time to time. Here are some common problems and their solutions:
1. Merge Conflicts
When Git can’t automatically merge changes, you’ll encounter a merge conflict. To resolve it:
- Open the conflicting file(s) and look for the conflict markers (<<<<<<<, =======, >>>>>>>).
- Manually edit the file to resolve the conflict.
- Stage the resolved file(s) using
git add
. - Complete the merge by committing the changes.
2. Accidentally Committed to the Wrong Branch
If you’ve committed changes to the wrong branch, you can fix it using these steps:
- Stash your changes:
git stash
- Switch to the correct branch:
git checkout correct-branch
- Apply the stashed changes:
git stash pop
- Commit the changes to the correct branch
3. Undoing the Last Commit
If you need to undo your last commit but keep the changes:
git reset --soft HEAD~1
If you want to completely discard the last commit and its changes:
git reset --hard HEAD~1
4. Forgot to Add a File to the Last Commit
If you forgot to include a file in your last commit:
- Stage the forgotten file:
git add forgotten-file.txt
- Amend the last commit:
git commit --amend --no-edit
Advanced Git Features
As you become more comfortable with Git, you may want to explore some of its more advanced features:
1. Interactive Rebase
Interactive rebase allows you to modify your commit history. It’s useful for cleaning up your commits before pushing to a shared repository:
git rebase -i HEAD~3
This command opens an interactive rebase for the last 3 commits, allowing you to reorder, edit, or squash commits.
2. Cherry-picking
Cherry-picking allows you to apply specific commits from one branch to another:
git cherry-pick <commit-hash>
3. Submodules
Submodules allow you to keep a Git repository as a subdirectory of another Git repository. This is useful for including external dependencies in your project:
git submodule add <repository-url> <path>
4. Git Hooks
Git hooks are scripts that Git executes before or after events such as commit, push, and receive. They’re useful for automating tasks or enforcing policies:
cd .git/hooks
cp pre-commit.sample pre-commit
chmod +x pre-commit
You can then edit the pre-commit
file to include your custom scripts.
5. Git Bisect
Git bisect is a powerful debugging tool that uses binary search to find the commit that introduced a bug:
git bisect start
git bisect bad # Current version is bad
git bisect good <known-good-commit>
# Git will then checkout commits for you to test
# Mark each commit as good or bad until the culprit is found
git bisect good # or git bisect bad
# When finished
git bisect reset
Conclusion
Congratulations! You’ve now learned how to set up Git, create repositories, use basic commands, and even explored some advanced features. Git is an incredibly powerful tool that will greatly enhance your development workflow and collaboration abilities.
Remember, mastering Git takes time and practice. Don’t be afraid to experiment with different commands and workflows to find what works best for you and your team. As you continue your journey with AlgoCademy, you’ll find that strong Git skills are invaluable for tackling complex coding challenges and collaborating on projects.
Keep exploring, keep learning, and happy coding!
For more tutorials on coding, algorithms, and preparing for technical interviews, check out our other resources here at AlgoCademy. We’re here to support you on your journey to becoming a skilled programmer and acing those FAANG interviews!