Functional Programming in Python: A Comprehensive Guide
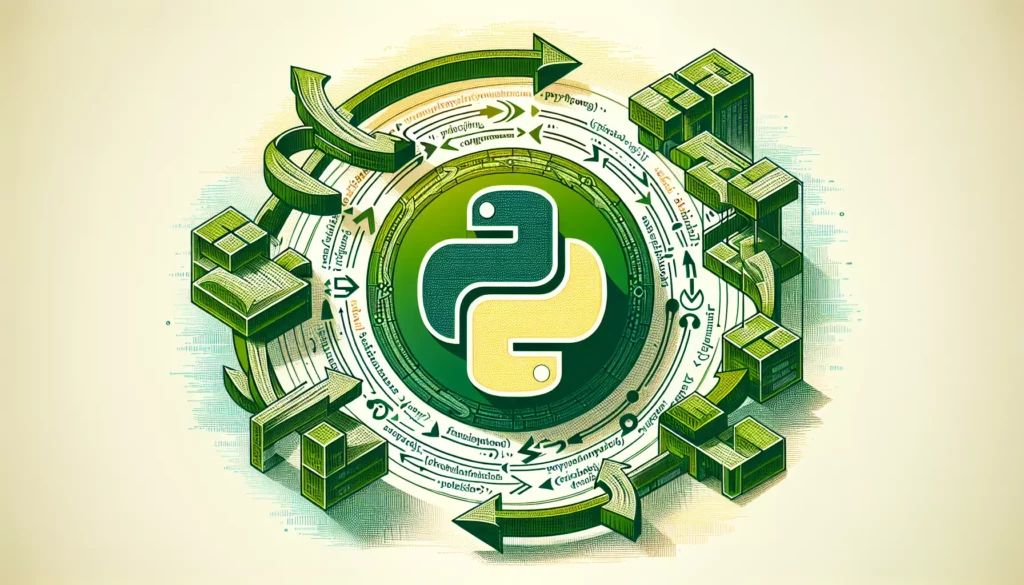
In the world of coding education and programming skills development, understanding different paradigms is crucial. One such paradigm that has gained significant traction in recent years is functional programming. In this comprehensive guide, we’ll explore functional programming in Python, a language known for its versatility and readability. Whether you’re a beginner looking to expand your coding horizons or an experienced developer preparing for technical interviews at major tech companies, this article will provide valuable insights into functional programming concepts and their implementation in Python.
Table of Contents
- Introduction to Functional Programming
- Core Principles of Functional Programming
- Python Features for Functional Programming
- First-Class Functions in Python
- Pure Functions and Immutability
- Higher-Order Functions
- Lambda Functions
- Map, Filter, and Reduce
- List Comprehensions and Generator Expressions
- Recursion in Functional Programming
- Decorators: Enhancing Functions
- Partial Functions and Currying
- Benefits and Challenges of Functional Programming
- Practical Examples and Use Cases
- Preparing for Technical Interviews with Functional Programming
- Conclusion
1. Introduction to Functional Programming
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. It emphasizes the application of functions to inputs to produce outputs without modifying state. This approach can lead to more predictable, testable, and maintainable code.
While Python is not a pure functional programming language like Haskell or Lisp, it does support many functional programming concepts. This makes Python an excellent language for learning and applying functional programming principles, especially for those coming from an object-oriented or procedural background.
2. Core Principles of Functional Programming
Before diving into Python-specific implementations, let’s review the core principles of functional programming:
- Immutability: Once created, data should not be changed. Instead of modifying existing data, new data structures are created.
- Pure Functions: Functions should always produce the same output for the same input and have no side effects.
- First-Class and Higher-Order Functions: Functions are treated as first-class citizens, meaning they can be assigned to variables, passed as arguments, and returned from other functions.
- Recursion: Iterative processes are often replaced with recursive functions.
- Declarative Programming: Code focuses on what to do, rather than how to do it.
3. Python Features for Functional Programming
Python provides several features that support functional programming:
- Lambda functions for creating anonymous functions
- Built-in functions like
map()
,filter()
, andreduce()
- List comprehensions and generator expressions
- Decorators for modifying or enhancing functions
- The
functools
module for higher-order functions and operations on callable objects
4. First-Class Functions in Python
In Python, functions are first-class citizens, which means they can be treated like any other object. This is a fundamental concept in functional programming. Here’s an example:
def greet(name):
return f"Hello, {name}!"
# Assigning a function to a variable
welcome = greet
# Using the function through the variable
print(welcome("Alice")) # Output: Hello, Alice!
# Passing a function as an argument
def apply_function(func, value):
return func(value)
result = apply_function(greet, "Bob")
print(result) # Output: Hello, Bob!
This capability allows for powerful abstractions and flexible code structures.
5. Pure Functions and Immutability
Pure functions are a cornerstone of functional programming. They always produce the same output for the same input and have no side effects. Here’s an example of a pure function in Python:
def add(a, b):
return a + b
result = add(3, 5)
print(result) # Output: 8
This function is pure because it always returns the same result for the same inputs and doesn’t modify any external state.
Immutability is closely related to pure functions. In Python, we can use immutable data types like tuples and frozensets to enforce immutability:
# Using a tuple (immutable) instead of a list (mutable)
point = (3, 4)
# point[0] = 5 # This would raise an error
# Creating a new tuple instead of modifying the existing one
new_point = (5, point[1])
print(new_point) # Output: (5, 4)
6. Higher-Order Functions
Higher-order functions are functions that can take other functions as arguments or return functions. They are a powerful tool in functional programming. Python’s built-in sorted()
function is an example of a higher-order function:
def by_length(word):
return len(word)
words = ["python", "functional", "programming", "is", "powerful"]
sorted_words = sorted(words, key=by_length)
print(sorted_words)
# Output: ['is', 'python', 'powerful', 'functional', 'programming']
In this example, sorted()
takes the by_length
function as an argument to determine the sorting order.
7. Lambda Functions
Lambda functions are small, anonymous functions that can have any number of arguments but can only have one expression. They are particularly useful in functional programming for creating quick, throwaway functions. Here’s an example:
# Using a lambda function with map()
numbers = [1, 2, 3, 4, 5]
squared = list(map(lambda x: x**2, numbers))
print(squared) # Output: [1, 4, 9, 16, 25]
# Using a lambda function with filter()
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
print(even_numbers) # Output: [2, 4]
8. Map, Filter, and Reduce
The map()
, filter()
, and reduce()
functions are powerful tools in functional programming:
from functools import reduce
numbers = [1, 2, 3, 4, 5]
# Map: Apply a function to all items in an input list
squared = list(map(lambda x: x**2, numbers))
print(squared) # Output: [1, 4, 9, 16, 25]
# Filter: Create a list of elements for which a function returns True
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
print(even_numbers) # Output: [2, 4]
# Reduce: Apply a function of two arguments cumulatively to the items of a sequence
sum_of_numbers = reduce(lambda x, y: x + y, numbers)
print(sum_of_numbers) # Output: 15
9. List Comprehensions and Generator Expressions
List comprehensions and generator expressions provide a concise way to create lists and generators based on existing lists or iterables. They align well with the functional programming paradigm:
# List comprehension
squares = [x**2 for x in range(10)]
print(squares) # Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
# Generator expression
even_squares = (x**2 for x in range(10) if x % 2 == 0)
print(list(even_squares)) # Output: [0, 4, 16, 36, 64]
10. Recursion in Functional Programming
Recursion is often used in functional programming instead of loops. Here’s an example of a recursive function to calculate the factorial of a number:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n - 1)
print(factorial(5)) # Output: 120
While Python has a default recursion limit, it’s important to note that excessive recursion can lead to stack overflow errors. In practice, tail recursion optimization or iteration might be preferred for performance reasons.
11. Decorators: Enhancing Functions
Decorators are a powerful feature in Python that allow you to modify or enhance functions. They are often used in functional programming to add functionality to existing functions without modifying their code. Here’s an example:
def uppercase_decorator(func):
def wrapper():
result = func()
return result.upper()
return wrapper
@uppercase_decorator
def greet():
return "hello, world!"
print(greet()) # Output: HELLO, WORLD!
12. Partial Functions and Currying
Partial functions and currying are techniques used in functional programming to create new functions from existing ones by fixing some arguments. Python’s functools.partial
can be used for this:
from functools import partial
def multiply(x, y):
return x * y
double = partial(multiply, 2)
print(double(4)) # Output: 8
triple = partial(multiply, 3)
print(triple(4)) # Output: 12
13. Benefits and Challenges of Functional Programming
Benefits of functional programming include:
- Improved code readability and maintainability
- Easier testing and debugging due to the absence of side effects
- Better support for parallel and concurrent programming
- Reduced bugs and errors in complex systems
Challenges include:
- Learning curve for developers used to imperative programming
- Potential performance overhead in some cases
- Limited support in some programming languages
14. Practical Examples and Use Cases
Let’s look at a practical example of using functional programming concepts in Python to solve a real-world problem. Suppose we want to process a list of transactions and calculate the total amount spent on each category:
from collections import defaultdict
transactions = [
("groceries", 50),
("entertainment", 30),
("groceries", 20),
("utilities", 100),
("entertainment", 45)
]
# Using a functional approach
def categorize_expenses(transactions):
expenses = defaultdict(int)
for category, amount in transactions:
expenses[category] += amount
return dict(expenses)
# Using reduce for a more functional approach
from functools import reduce
def add_expense(expenses, transaction):
category, amount = transaction
expenses[category] = expenses.get(category, 0) + amount
return expenses
categorized_expenses = reduce(add_expense, transactions, {})
print(categorized_expenses)
# Output: {'groceries': 70, 'entertainment': 75, 'utilities': 100}
This example demonstrates how functional programming concepts can be applied to solve data processing problems in a clean and efficient manner.
15. Preparing for Technical Interviews with Functional Programming
Understanding functional programming can be beneficial for technical interviews, especially at major tech companies. Here are some tips:
- Practice solving common algorithmic problems using functional programming techniques.
- Be prepared to explain the benefits and trade-offs of functional programming.
- Understand how to use functional programming to improve code readability and maintainability.
- Be familiar with functional programming features in the language you’re using (e.g., Python’s
map
,filter
,reduce
, and lambda functions). - Practice explaining your thought process when using functional programming concepts to solve problems.
16. Conclusion
Functional programming in Python offers a powerful paradigm for writing clean, maintainable, and efficient code. By embracing concepts such as pure functions, immutability, and higher-order functions, developers can create more robust and scalable applications. While Python is not a purely functional language, its support for functional programming concepts makes it an excellent choice for learning and applying these principles.
As you continue your journey in coding education and skills development, incorporating functional programming techniques into your toolkit can significantly enhance your problem-solving abilities and prepare you for technical interviews at major tech companies. Remember that mastering functional programming, like any skill, requires practice and patience. Keep coding, experimenting, and pushing the boundaries of what you can do with functional programming in Python!